-
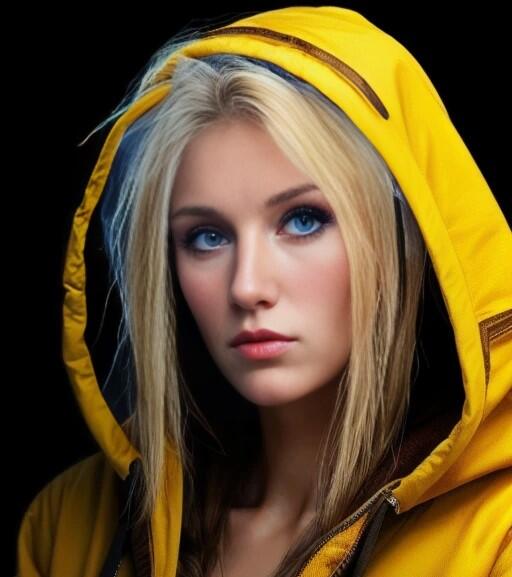
@ ever4st
2025-02-01 09:06:49
### **The Magic of `=()=` in Perl: Counting Matches with Elegance**
Perl is full of powerful and sometimes cryptic features, and one of the lesser-known yet highly useful tricks is the `=()=` operator. This clever construct allows you to count the number of matches in a string without using loops or unnecessary variables.
---
## **Understanding `=()=`: A Smart Counting Trick**
Normally, when you apply a regex with the `/g` (global match) flag, Perl finds all occurrences in the string. However, getting the **count** of those matches isn't as straightforward as you might expect.
Consider this example:
```perl
my $str = "Hello 123, Perl 456!";
my $count = () = $str =~ /\w/g;
print "Matched $count word characters.\n";
```
### **Breaking It Down**
1. **Regex Matching (`$str =~ /\w/g`)**
- This searches for **all** word characters (`\w` → `[A-Za-z0-9_]`) in `$str`.
- The `/g` flag ensures it **captures all occurrences** instead of just the first one.
2. **The Empty List `()`**
- The regex match is assigned to an **empty list `()`**.
- This forces Perl to evaluate the regex in **list context**, where it returns **all matches** instead of just one.
3. **Assigning to a Scalar (`$count`)**
- When a **list is assigned to a scalar**, Perl doesn't store the list—it simply counts its elements.
- The result? `$count` contains the **number of matches**.
### **Expected Output**
```
Matched 15 word characters.
```
If you manually count the `\w` matches (`Hello123Perl456`), you'll find **15**.
---
## **Why Not Use `scalar()` Instead?**
You might think this is equivalent to:
```perl
my $count = scalar($str =~ /\w/g); # will return 1
```
**But this won't work!**
- `scalar($str =~ /\w/g)` only returns the **last match found**, not the count.
- `=()=` is necessary because it **forces list context**, ensuring Perl counts all matches correctly.
---
## **Practical Use Cases**
This trick is useful whenever you need to count occurrences without modifying the original string. Some real-world applications include:
✅ Counting **words, numbers, or special characters** in a string.
✅ Measuring **how many times a pattern appears** in a text.
✅ Quick **validation checks** (e.g., ensuring a string contains at least `n` digits).
Example: Counting words in a sentence
```perl
my $sentence = "Perl is awesome!";
my $word_count = () = $sentence =~ /\b\w+\b/g;
print "Word count: $word_count\n"; # Output: 3
```
---
## **Final Thoughts**
The `=()=` operator might look like magic at first, but it's actually an elegant way to **force list context** and extract match counts without loops or extra variables. Whether processing text or just trying to optimize Perl scripts, this trick is a powerful addition to the Perl toolkit.