-
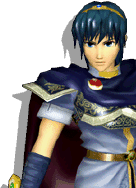
@ Marth
2024-09-03 21:58:26
# Lesson 3: Arrays, Objects, and Callbacks
### Arrays: Lists of Things
Arrays are ordered lists of values. They're perfect for storing multiple related items, like a list of your favorite cat toys:
```javascript
var catToys = ["mouse", "ball", "laser pointer"];
console.log(catToys[0]); // Prints "mouse"
```
### Objects: Grouping Related Information
Objects allow you to group related information together. They're like detailed profiles for each of your cat friends:
```javascript
var myCat = {
name: "Fluffy",
age: 3,
favoriteFood: "tuna"
};
console.log(myCat.name); // Prints "Fluffy"
```
### Callbacks: Doing Things Later
Callbacks are functions that are executed after another function has finished. They're useful for handling asynchronous operations, like waiting for your cat to finish eating before giving it a treat:
```javascript
function feedCat(callback) {
console.log("Feeding the cat...");
setTimeout(function() {
console.log("Cat has finished eating!");
callback();
}, 2000);
}
feedCat(function() {
console.log("Time for a treat!");
});
```
This code simulates feeding a cat, waiting 2 seconds, and then giving it a treat.
Remember, learning JavaScript is a journey. Take your time, practice regularly, and soon you'll be coding like a pro cat!