-
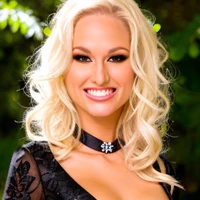
@ ev3-blog
2025-01-22 21:39:38
```perl
#!/usr/bin/perl
use strict;
use warnings;
use Term::ReadKey;
STDOUT->autoflush(1);
# this version run on Windows and use a pwd.txt file created by
# the perl program ; otherwise encoding issue may follow
# following sub needs to be used first by uncommenting the main call
sub write_password {
print ("Enter Password: ") ;
my $password = <STDIN>;
my $file_path = 'pwd.txt';
open(my $fh, '>', $file_path) or die "Cannot open file '$file_path' for writing: $!";
print $fh $password;
close($fh);
print ("\n");
print "Password written to '$file_path'\n";
}
sub printhex {
my $str = shift ;
foreach my $char (split //, $str) {
printf "%02x ", ord($char);
}
print "\n";
}
# Function to read password from file
sub read_password_from_file {
#unecessary
#binmode(STDIN, ':crlf');
my $file_path = shift;
#unecessary
#local $/ = "\r\n" ; # CR, use "\r\n" for CRLF or "\n" for LF
open(my $fh, '<', $file_path) or die "Cannot open file '$file_path' for reading: $!";
my $password = <$fh>;
close($fh);
#printhex ($password) ;
chomp($password);
print "'$password'\n" ;
#$password =~ s/\r?\n$//; # Remove newline character
#$password = substr($password, 2) ; # BOM File starts with FF FE
printhex($password ) ; # "\'$password\'\n" ;
#$password = "abcde" ;
return $password;
}
# Main program
sub main {
#write_password(); # to uncomment for first use
my $correct_password = read_password_from_file("pwd.txt") ;
print "Enter password: ";
ReadMode('noecho'); # Turn off echo
my $entered_password = ReadLine(0);
ReadMode('restore'); # Restore echo
chomp($entered_password);
print "\n";
# Compare entered password with the correct password
if ($entered_password eq $correct_password) {
print "Access granted!\n";
} else {
print "Access denied!\n";
print "'$entered_password' not eq '$correct_password' \n";
printhex ($entered_password);
printhex ($correct_password) ;
print "The end! \n" ;
}
}
# Call main function
main();
```