-
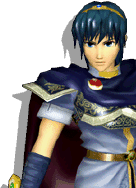
@ Marth
2024-09-03 21:58:26
# Lesson 2: Functions and Loops
### Functions: Your Own Commands
Functions are reusable blocks of code that perform specific tasks. They're like teaching your cat a new trick:
```javascript
function meow(times) {
for (var i = 0; i < times; i++) {
console.log("Meow!");
}
}
meow(3); // This will print "Meow!" three times
```
### Built-in Functions
JavaScript comes with many built-in functions. For example, `console.log()` is a function that prints things to the console:
```javascript
console.log("I'm a cat!");
```
### Loops: Repeating Tasks
Loops allow you to repeat a task multiple times without writing the same code over and over. It's like telling your cat to chase its tail for a specific number of rounds:
```javascript
for (var i = 0; i < 5; i++) {
console.log("Chasing tail, round " + (i + 1));
}
```
This will print "Chasing tail, round X" five times, where X goes from 1 to 5.