-

@ f33c8a96:5ec6f741
2024-11-27 00:09:03
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/WaPLVUS81EM?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ 5d4b6c8d:8a1c1ee3
2024-11-27 00:06:51
Wizards and Bulls are about to tip.
Who's watching the games tonight?
I've got a little action on the Bucks, who are pretty large underdogs, for reasons I can't begin to fathom.
originally posted at https://stacker.news/items/784245
-

@ f33c8a96:5ec6f741
2024-11-26 16:35:01
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><video src="https://plebdevs-bucket.nyc3.cdn.digitaloceanspaces.com/starter-lesson-4.mp4" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" controls></video></div>
# CSS Fundamentals: Styling Your First Webpage
## Introduction
In our previous lesson, we created the structure of our webpage with HTML. Now, we'll learn how to style it using CSS (Cascading Style Sheets). While HTML provides the bones of our webpage, CSS adds the visual presentation - the colors, layouts, spacing, and overall aesthetics.
## What is CSS?
### Definition
CSS (Cascading Style Sheets) is a stylesheet language that controls the visual presentation of HTML documents. Think of it like the paint, decorations, and interior design of a house - it determines how everything looks and is arranged.
### Key Concepts
1. **Styling Capabilities**
- Fonts and typography
- Colors and backgrounds
- Margins and padding
- Element sizes
- Visual effects
- Layout and positioning
2. **Cascading Nature**
- Styles can be inherited from parent elements
- Multiple styles can apply to the same element
- Specificity determines which styles take precedence
- Styles "cascade" down through your document
## Basic CSS Syntax
```css
selector {
property: value;
}
```
### Example:
```css
h1 {
color: blue;
font-size: 24px;
margin-bottom: 20px;
}
```
## Connecting CSS to HTML
### Method 1: External Stylesheet (Recommended)
```html
<link rel="stylesheet" href="style.css">
```
### Method 2: Internal CSS
```html
<style>
h1 {
color: blue;
}
</style>
```
### Method 3: Inline CSS (Use Sparingly)
```html
<h1 style="color: blue;">Title</h1>
```
## The Box Model
Every HTML element is treated as a box in CSS, with:
```
┌──────────────────────┐
│ Margin │
│ ┌──────────────┐ │
│ │ Border │ │
│ │ ┌──────────┐ │ │
│ │ │ Padding │ │ │
│ │ │ ┌──────┐ │ │ │
│ │ │ │ │ │ │ │
│ │ │ │Content│ │ │ │
│ │ │ │ │ │ │ │
│ │ │ └──────┘ │ │ │
│ │ └──────────┘ │ │
│ └──────────────┘ │
└──────────────────────┘
```
- **Content**: The actual content of the element
- **Padding**: Space between content and border
- **Border**: The border around the padding
- **Margin**: Space outside the border
## CSS Units
### Absolute Units
- `px` - pixels
- `pt` - points
- `cm` - centimeters
- `mm` - millimeters
- `in` - inches
### Relative Units
- `%` - percentage relative to parent
- `em` - relative to font-size
- `rem` - relative to root font-size
- `vh` - viewport height
- `vw` - viewport width
## Practical Example: Styling Our Webpage
### 1. Basic Page Setup
```css
body {
min-height: 100vh;
margin: 0;
font-family: Arial, sans-serif;
background-color: #f0f0f0;
display: flex;
flex-direction: column;
}
```
### 2. Header Styling
```css
header {
background-color: #333;
color: white;
padding: 20px;
text-align: center;
}
```
### 3. Main Content Area
```css
main {
max-width: 800px;
margin: 0 auto;
padding: 20px;
flex: 1;
}
```
### 4. Footer Styling
```css
footer {
background-color: #333;
color: white;
padding: 10px;
text-align: center;
}
```
## Layout with Flexbox
### Basic Concept
Flexbox is a modern layout system that makes it easier to create flexible, responsive layouts.
### Key Properties
```css
.container {
display: flex;
flex-direction: row | column;
justify-content: center | space-between | space-around;
align-items: center | flex-start | flex-end;
}
```
### Common Use Cases
1. Centering content
2. Creating navigation bars
3. Building responsive layouts
4. Equal-height columns
5. Dynamic spacing
## Best Practices
### 1. Organization
- Use consistent naming conventions
- Group related styles together
- Comment your code for clarity
- Keep selectors simple and specific
### 2. Performance
- Avoid unnecessary specificity
- Use shorthand properties when possible
- Minimize redundant code
- Consider load time impact
### 3. Maintainability
- Use external stylesheets
- Follow a consistent formatting style
- Break large stylesheets into logical files
- Document important design decisions
## Debugging CSS
### Common Tools
1. Browser Developer Tools
- Element inspector
- Style inspector
- Box model viewer
### Common Issues
1. Specificity conflicts
2. Inheritance problems
3. Box model confusion
4. Flexbox alignment issues
## Exercises
### 1. Style Modifications
Try modifying these properties in your stylesheet:
```css
/* Change colors */
header {
background-color: #4a90e2;
}
/* Adjust spacing */
main {
padding: 40px;
}
/* Modify typography */
h1 {
font-size: 32px;
font-weight: bold;
}
```
### 2. Layout Challenge
Create a card layout using Flexbox:
```css
.card-container {
display: flex;
justify-content: space-between;
gap: 20px;
}
.card {
flex: 1;
padding: 20px;
background: white;
border-radius: 8px;
box-shadow: 0 2px 4px rgba(0,0,0,0.1);
}
```
## Additional Resources
### Learning Tools
1. [Flexbox Froggy](https://flexboxfroggy.com/) - Interactive Flexbox learning game
2. [CSS-Tricks](https://css-tricks.com) - Excellent CSS reference and tutorials
3. [MDN CSS Documentation](https://developer.mozilla.org/en-US/docs/Web/CSS)
### Practice Projects
1. Style your personal webpage
2. Create a responsive navigation menu
3. Build a flexible card layout
4. Design a custom button style
Remember: CSS is both an art and a science. Don't be afraid to experiment and break things - that's how you'll learn the most. The key is to start simple and gradually add complexity as you become more comfortable with the basics.
Next up, we'll dive into JavaScript to add interactivity to our webpage! 🚀
-

@ f33c8a96:5ec6f741
2024-11-26 15:41:31
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><video src="https://plebdevs-bucket.nyc3.cdn.digitaloceanspaces.com/starter-lesson-4.mp4" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" controls></video></div>
# CSS Fundamentals: Styling Your First Webpage
## Introduction
In our previous lesson, we created the structure of our webpage with HTML. Now, we'll learn how to style it using CSS (Cascading Style Sheets). While HTML provides the bones of our webpage, CSS adds the visual presentation - the colors, layouts, spacing, and overall aesthetics.
## What is CSS?
### Definition
CSS (Cascading Style Sheets) is a stylesheet language that controls the visual presentation of HTML documents. Think of it like the paint, decorations, and interior design of a house - it determines how everything looks and is arranged.
### Key Concepts
1. **Styling Capabilities**
- Fonts and typography
- Colors and backgrounds
- Margins and padding
- Element sizes
- Visual effects
- Layout and positioning
2. **Cascading Nature**
- Styles can be inherited from parent elements
- Multiple styles can apply to the same element
- Specificity determines which styles take precedence
- Styles "cascade" down through your document
## Basic CSS Syntax
```css
selector {
property: value;
}
```
### Example:
```css
h1 {
color: blue;
font-size: 24px;
margin-bottom: 20px;
}
```
## Connecting CSS to HTML
### Method 1: External Stylesheet (Recommended)
```html
<link rel="stylesheet" href="style.css">
```
### Method 2: Internal CSS
```html
<style>
h1 {
color: blue;
}
</style>
```
### Method 3: Inline CSS (Use Sparingly)
```html
<h1 style="color: blue;">Title</h1>
```
## The Box Model
Every HTML element is treated as a box in CSS, with:
```
┌──────────────────────┐
│ Margin │
│ ┌──────────────┐ │
│ │ Border │ │
│ │ ┌──────────┐ │ │
│ │ │ Padding │ │ │
│ │ │ ┌──────┐ │ │ │
│ │ │ │ │ │ │ │
│ │ │ │Content│ │ │ │
│ │ │ │ │ │ │ │
│ │ │ └──────┘ │ │ │
│ │ └──────────┘ │ │
│ └──────────────┘ │
└──────────────────────┘
```
- **Content**: The actual content of the element
- **Padding**: Space between content and border
- **Border**: The border around the padding
- **Margin**: Space outside the border
## CSS Units
### Absolute Units
- `px` - pixels
- `pt` - points
- `cm` - centimeters
- `mm` - millimeters
- `in` - inches
### Relative Units
- `%` - percentage relative to parent
- `em` - relative to font-size
- `rem` - relative to root font-size
- `vh` - viewport height
- `vw` - viewport width
## Practical Example: Styling Our Webpage
### 1. Basic Page Setup
```css
body {
min-height: 100vh;
margin: 0;
font-family: Arial, sans-serif;
background-color: #f0f0f0;
display: flex;
flex-direction: column;
}
```
### 2. Header Styling
```css
header {
background-color: #333;
color: white;
padding: 20px;
text-align: center;
}
```
### 3. Main Content Area
```css
main {
max-width: 800px;
margin: 0 auto;
padding: 20px;
flex: 1;
}
```
### 4. Footer Styling
```css
footer {
background-color: #333;
color: white;
padding: 10px;
text-align: center;
}
```
## Layout with Flexbox
### Basic Concept
Flexbox is a modern layout system that makes it easier to create flexible, responsive layouts.
### Key Properties
```css
.container {
display: flex;
flex-direction: row | column;
justify-content: center | space-between | space-around;
align-items: center | flex-start | flex-end;
}
```
### Common Use Cases
1. Centering content
2. Creating navigation bars
3. Building responsive layouts
4. Equal-height columns
5. Dynamic spacing
## Best Practices
### 1. Organization
- Use consistent naming conventions
- Group related styles together
- Comment your code for clarity
- Keep selectors simple and specific
### 2. Performance
- Avoid unnecessary specificity
- Use shorthand properties when possible
- Minimize redundant code
- Consider load time impact
### 3. Maintainability
- Use external stylesheets
- Follow a consistent formatting style
- Break large stylesheets into logical files
- Document important design decisions
## Debugging CSS
### Common Tools
1. Browser Developer Tools
- Element inspector
- Style inspector
- Box model viewer
### Common Issues
1. Specificity conflicts
2. Inheritance problems
3. Box model confusion
4. Flexbox alignment issues
## Exercises
### 1. Style Modifications
Try modifying these properties in your stylesheet:
```css
/* Change colors */
header {
background-color: #4a90e2;
}
/* Adjust spacing */
main {
padding: 40px;
}
/* Modify typography */
h1 {
font-size: 32px;
font-weight: bold;
}
```
### 2. Layout Challenge
Create a card layout using Flexbox:
```css
.card-container {
display: flex;
justify-content: space-between;
gap: 20px;
}
.card {
flex: 1;
padding: 20px;
background: white;
border-radius: 8px;
box-shadow: 0 2px 4px rgba(0,0,0,0.1);
}
```
## Additional Resources
### Learning Tools
1. [Flexbox Froggy](https://flexboxfroggy.com/) - Interactive Flexbox learning game
2. [CSS-Tricks](https://css-tricks.com) - Excellent CSS reference and tutorials
3. [MDN CSS Documentation](https://developer.mozilla.org/en-US/docs/Web/CSS)
### Practice Projects
1. Style your personal webpage
2. Create a responsive navigation menu
3. Build a flexible card layout
4. Design a custom button style
Remember: CSS is both an art and a science. Don't be afraid to experiment and break things - that's how you'll learn the most. The key is to start simple and gradually add complexity as you become more comfortable with the basics.
Next up, we'll dive into JavaScript to add interactivity to our webpage! 🚀
-

@ f33c8a96:5ec6f741
2024-11-26 15:11:37
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><video src="https://plebdevs.com/api/get-video-url?videoKey=starter-lesson-0.mp4" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" controls></video></div>
# Welcome to the PlebDevs Starter Course
## Course Mission
Welcome to the PlebDevs starter course! I'm Austin, the founder of PlebDevs, and I'll be walking you through this short intro starter course that will get you up and running and interacting with the rest of the content on the platform. If you're here, I'm assuming you're new to coding or just starting out. If you already have experience and have your bearings down, there's lots more intermediate and advanced content on the platform. This course is designed for absolute beginners.
## Course Goals
### Overall PlebDevs Goals
1. Learn how to code
2. Build Bitcoin/Lightning/Nostr apps
3. Become a developer
This is a big journey with a lot of pieces and things to learn. The starter course is going to get you on that path as quickly as possible and make it a smooth journey.
### Starter Course Objectives
1. Give you an easy, high-level overview of the dev journey
2. Get you set up and comfortable in a development environment where you'll write and ship code
3. Give you basic experience in the languages covered in our courses and workshops
There's lots of material out there that will just tell you exactly what to do and not explain why but I want you to actually understand for yourself. I want you to have a good mental model of what this journey is going to be like, what it's going to be like actually writing and pushing code. And then we're going to start getting our hands dirty and gain real experience.
## What is a PlebDev?
### Origins and Philosophy
I started using the term "PlebDev" a few years ago to describe a unique approach to learning development in the Bitcoin space. It represents:
- **Inclusive Learning**: Anyone can become a developer, regardless of background
- **Growth Mindset**: Embracing the journey from beginner to professional
- **Practical Focus**: Emphasizing real-world application development
- **Community Support**: Learning and growing together
### Key Characteristics
- 🌱 **Growth-Focused**: PlebDevs are always learning and improving
- 🎯 **App-Centric**: Focus on building applications rather than protocol development
- 🆕 **Embrace Being New**: Being a new developer is infinitely better than being on the sidelines
- 🤝 **Community-Driven**: Bitcoin/Lightning/Nostr ecosystem needs more plebdevs like you!
## Our Learning Approach
### Core Principles
1. **Lower Barriers**
- Simplify complex concepts
- Focus on practical understanding
- Build confidence through action
2. **Project-Based Learning**
- Learn by doing
- Create real applications
- Build a portfolio as you learn
3. **MVP (Minimum Viable Product) Focus**
- Start with core functionality
- Get things working first
- Iterate and improve
4. **Actionable Knowledge**
- Focus on the 20% that delivers 80% of results
- Learn what you can use right away
- Build practical skills
### Teaching Methods
- Detailed concept breakdowns
- Line-by-line code explanations
- Interactive learning
- 1:1 support available
- Community-driven progress
## Course Structure
### The Learning Path
Instead of the traditional bottom-up approach, we use a project-focused method:
```
🏔️ Advanced Skills
🏔️ Projects & Practice
🏔️ Core Concepts
🏔️ Development Environment
🏔️ Getting Started
```
We'll create checkpoints through projects, allowing you to:
- Verify your understanding
- Build your portfolio
- See real progress
- Have reference points for review
## Student Expectations
### What We Expect From You
- **High Agency**: Take ownership of your learning journey
- **Active Participation**: Engage with the material and community
- **Persistence**: Push through challenges
- **Curiosity**: Ask questions and explore concepts
### What You Can Expect From Us
- Clear, practical instruction
- Comprehensive support
- Real-world applications
- Community backing
## Getting Started
### Next Steps
1. Ensure you're ready to commit to learning
2. Set up your development environment (next lesson)
3. Join our community
4. Start building!
## Resources and Support
### Where to Get Help
- plebdevs.com
### Tips for Success
1. Push code daily, even if it is small.
2. Focus on understanding rather than memorizing.
3. Build projects that interest you.
4. Engage with the community.
5. Don't be afraid to ask questions.
## Remember
You don't need to become a "10x developer" overnight. The goal is to start writing code, build useful things, and gradually improve. Every expert was once a beginner, and the journey of a thousand miles begins with a single line of code.
Ready to begin? Let's dive into the next lesson where we'll set up your development environment! 🚀
-

@ f33c8a96:5ec6f741
2024-11-25 23:52:42
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/BgKagRu_n2M?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ f33c8a96:5ec6f741
2024-11-25 23:47:16
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/RK40cIY8t3E?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ 5d4b6c8d:8a1c1ee3
2024-11-25 23:46:05
We're moving on up! The Raiders are sitting on the third pick, with no prospect of winning again in sight.
This is by far the most hopeless Raiders team I've ever seen. Not necessarily the worst, but I've never been so sure that they're going to lose each week.
# Picks
3rd - QB Shedeur Sanders
34th - WR Isaiah Bond
65th - CB Mansoor Delane
69th (nice) - RB Kaleb Johnson
104th - DT Omarr Norman-Lott
140th - DE Dani Dennis-Sutton
177th - S Dante Trader Jr.
212th - G Jonah Monheim
216th - LB Chris Paul Jr.
219th - OT Drew Shelton
Finally! We got our QB. There are a lot of signs that Shedeur is angling to land in Vegas and the interest is definitely mutual.
It would have been nice to take care of the lines a little earlier, but a high-end WR would be very helpful.
Also, Chris Paul's son falls to us in the 7th.
originally posted at https://stacker.news/items/782909
-

@ f33c8a96:5ec6f741
2024-11-25 23:43:20
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/HLhjV813rqc?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ f33c8a96:5ec6f741
2024-11-25 23:41:06
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/qeKZj-DZ6iU?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ f33c8a96:5ec6f741
2024-11-25 23:27:06
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/_G49vKrKbsU?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ 468f729d:5ab4fd5e
2024-11-25 21:46:31
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><video src="http://localhost:3000/api/get-video-url?videoKey=starter-lesson-0.mp4" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" controls></video></div>
## Course Mission
Welcome to the PlebDevs starter course! I'm Austin, the founder of PlebDevs, and I'll be walking you through this short intro starter course that will get you up and running and interacting with the rest of the content on the platform. If you're here, I'm assuming you're new to coding or just starting out. If you already have experience and have your bearings down, there's lots more intermediate and advanced content on the platform. This course is designed for absolute beginners.
## Course Goals
### Overall PlebDevs Goals
1. Learn how to code
2. Build Bitcoin/Lightning/Nostr apps
3. Become a developer
This is a big journey with a lot of pieces and things to learn. The starter course is going to get you on that path as quickly as possible and make it a smooth journey.
### Starter Course Objectives
1. Give you an easy, high-level overview of the dev journey
2. Get you set up and comfortable in a development environment where you'll write and ship code
3. Give you basic experience in the languages covered in our courses and workshops
There's lots of material out there that will just tell you exactly what to do and not explain why but I want you to actually understand for yourself. I want you to have a good mental model of what this journey is going to be like, what it's going to be like actually writing and pushing code. And then we're going to start getting our hands dirty and gain real experience.
## What is a PlebDev?
### Origins and Philosophy
I started using the term "PlebDev" a few years ago to describe a unique approach to learning development in the Bitcoin space. It represents:
- **Inclusive Learning**: Anyone can become a developer, regardless of background
- **Growth Mindset**: Embracing the journey from beginner to professional
- **Practical Focus**: Emphasizing real-world application development
- **Community Support**: Learning and growing together
### Key Characteristics
- 🌱 **Growth-Focused**: PlebDevs are always learning and improving
- 🎯 **App-Centric**: Focus on building applications rather than protocol development
- 🆕 **Embrace Being New**: Being a new developer is infinitely better than being on the sidelines
- 🤝 **Community-Driven**: Bitcoin/Lightning/Nostr ecosystem needs more plebdevs like you!
## Our Learning Approach
### Core Principles
1. **Lower Barriers**
- Simplify complex concepts
- Focus on practical understanding
- Build confidence through action
2. **Project-Based Learning**
- Learn by doing
- Create real applications
- Build a portfolio as you learn
3. **MVP (Minimum Viable Product) Focus**
- Start with core functionality
- Get things working first
- Iterate and improve
4. **Actionable Knowledge**
- Focus on the 20% that delivers 80% of results
- Learn what you can use right away
- Build practical skills
### Teaching Methods
- Detailed concept breakdowns
- Line-by-line code explanations
- Interactive learning
- 1:1 support available
- Community-driven progress
## Course Structure
### The Learning Path
Instead of the traditional bottom-up approach, we use a project-focused method:
```
🏔️ Advanced Skills
🏔️ Projects & Practice
🏔️ Core Concepts
🏔️ Development Environment
🏔️ Getting Started
```
We'll create checkpoints through projects, allowing you to:
- Verify your understanding
- Build your portfolio
- See real progress
- Have reference points for review
## Student Expectations
### What We Expect From You
- **High Agency**: Take ownership of your learning journey
- **Active Participation**: Engage with the material and community
- **Persistence**: Push through challenges
- **Curiosity**: Ask questions and explore concepts
### What You Can Expect From Us
- Clear, practical instruction
- Comprehensive support
- Real-world applications
- Community backing
## Getting Started
### Next Steps
1. Ensure you're ready to commit to learning
2. Set up your development environment (next lesson)
3. Join our community
4. Start building!
## Resources and Support
### Where to Get Help
- plebdevs.com
### Tips for Success
1. Push code daily, even if it is small.
2. Focus on understanding rather than memorizing.
3. Build projects that interest you.
4. Engage with the community.
5. Don't be afraid to ask questions.
## Remember
You don't need to become a "10x developer" overnight. The goal is to start writing code, build useful things, and gradually improve. Every expert was once a beginner, and the journey of a thousand miles begins with a single line of code.
Ready to begin? Let's dive into the next lesson where we'll set up your development environment! 🚀
-

@ 468f729d:5ab4fd5e
2024-11-25 21:44:44
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><video src="http://localhost:3000/api/get-video-url?videoKey=testr.mp4" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" controls></video></div>
## Course Mission
Welcome to the PlebDevs starter course! I'm Austin, the founder of PlebDevs, and I'll be walking you through this short intro starter course that will get you up and running and interacting with the rest of the content on the platform. If you're here, I'm assuming you're new to coding or just starting out. If you already have experience and have your bearings down, there's lots more intermediate and advanced content on the platform. This course is designed for absolute beginners.
## Course Goals
### Overall PlebDevs Goals
1. Learn how to code
2. Build Bitcoin/Lightning/Nostr apps
3. Become a developer
This is a big journey with a lot of pieces and things to learn. The starter course is going to get you on that path as quickly as possible and make it a smooth journey.
### Starter Course Objectives
1. Give you an easy, high-level overview of the dev journey
2. Get you set up and comfortable in a development environment where you'll write and ship code
3. Give you basic experience in the languages covered in our courses and workshops
There's lots of material out there that will just tell you exactly what to do and not explain why but I want you to actually understand for yourself. I want you to have a good mental model of what this journey is going to be like, what it's going to be like actually writing and pushing code. And then we're going to start getting our hands dirty and gain real experience.
## What is a PlebDev?
### Origins and Philosophy
I started using the term "PlebDev" a few years ago to describe a unique approach to learning development in the Bitcoin space. It represents:
- **Inclusive Learning**: Anyone can become a developer, regardless of background
- **Growth Mindset**: Embracing the journey from beginner to professional
- **Practical Focus**: Emphasizing real-world application development
- **Community Support**: Learning and growing together
### Key Characteristics
- 🌱 **Growth-Focused**: PlebDevs are always learning and improving
- 🎯 **App-Centric**: Focus on building applications rather than protocol development
- 🆕 **Embrace Being New**: Being a new developer is infinitely better than being on the sidelines
- 🤝 **Community-Driven**: Bitcoin/Lightning/Nostr ecosystem needs more plebdevs like you!
## Our Learning Approach
### Core Principles
1. **Lower Barriers**
- Simplify complex concepts
- Focus on practical understanding
- Build confidence through action
2. **Project-Based Learning**
- Learn by doing
- Create real applications
- Build a portfolio as you learn
3. **MVP (Minimum Viable Product) Focus**
- Start with core functionality
- Get things working first
- Iterate and improve
4. **Actionable Knowledge**
- Focus on the 20% that delivers 80% of results
- Learn what you can use right away
- Build practical skills
### Teaching Methods
- Detailed concept breakdowns
- Line-by-line code explanations
- Interactive learning
- 1:1 support available
- Community-driven progress
## Course Structure
### The Learning Path
Instead of the traditional bottom-up approach, we use a project-focused method:
```
🏔️ Advanced Skills
🏔️ Projects & Practice
🏔️ Core Concepts
🏔️ Development Environment
🏔️ Getting Started
```
We'll create checkpoints through projects, allowing you to:
- Verify your understanding
- Build your portfolio
- See real progress
- Have reference points for review
## Student Expectations
### What We Expect From You
- **High Agency**: Take ownership of your learning journey
- **Active Participation**: Engage with the material and community
- **Persistence**: Push through challenges
- **Curiosity**: Ask questions and explore concepts
### What You Can Expect From Us
- Clear, practical instruction
- Comprehensive support
- Real-world applications
- Community backing
## Getting Started
### Next Steps
1. Ensure you're ready to commit to learning
2. Set up your development environment (next lesson)
3. Join our community
4. Start building!
## Resources and Support
### Where to Get Help
- plebdevs.com
### Tips for Success
1. Push code daily, even if it is small.
2. Focus on understanding rather than memorizing.
3. Build projects that interest you.
4. Engage with the community.
5. Don't be afraid to ask questions.
## Remember
You don't need to become a "10x developer" overnight. The goal is to start writing code, build useful things, and gradually improve. Every expert was once a beginner, and the journey of a thousand miles begins with a single line of code.
Ready to begin? Let's dive into the next lesson where we'll set up your development environment! 🚀
-

@ e968e50b:db2a803a
2024-11-25 17:46:20
# Home bitcoin mining is arbitrage across time.
As I understand it, industrial miners pay as they go. In fact, I believe there are whole companies that are dedicated to mitigating risk to the energy providers by providing direct, real-time payment rails between the miners and providers.
This is not true for me. I pay my energy bill once a month, LONG after the bitcoin has been mined, the heat recycled, and the sn pontifications posted. What this means is that the "profitability" of home miners on any given day is actually related to the current "price" of bitcoin on the date that one has to sell it to pay the electric bill. For example:
[Here's a website I like to go to when I'm too lazy to do the math on a miner's profitability myself.](https://www.asicminervalue.com) Whenever I've checked it, it's been basically correct. BUT, that's if I'm assuming that I have to pay my electric bill in fiat the same day that I do my mining it sats. This isn't the case though. In reality, if I have a miner that makes 250 (pic below) sats a day, I can get those sats from my pool immediately through the lightning network. According to the calculator, I'm paying 20 cents for those sats, so I'm paying 210 sats in electricity as of the time of this writing. BUT that's not really the case. I'm actually going to pay for that electricity on January 1st. So, by the time I'm paying, if the "price" of bitcoin has risen, I'm selling less sats out of my earnings.
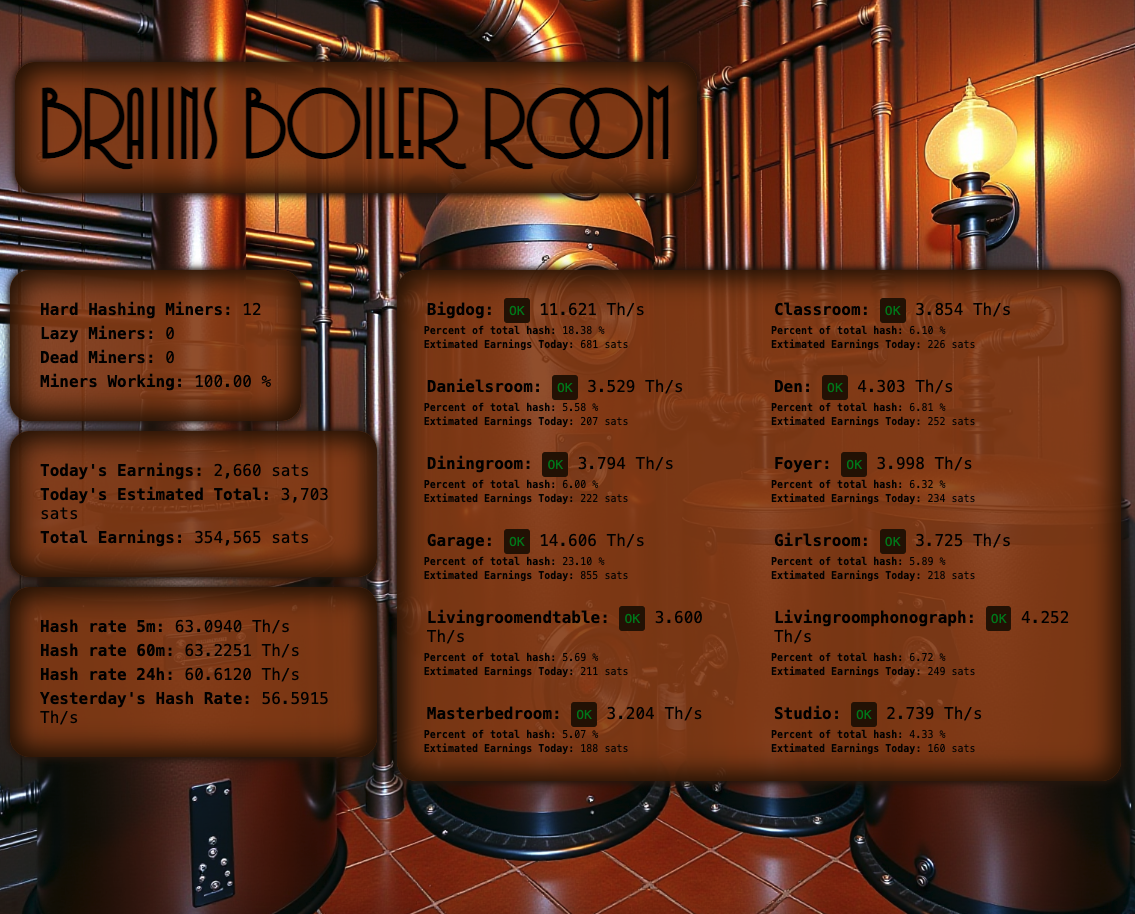
What's the point? You should be home mining. It's not just good for the network, it's a way to be a real-life time bandit! You'll be like the Terminator, but with arbitrage. ...like of like a Robin Hood Terminator.
## Actual Picture of Home Miner in 2024 - The Robinator
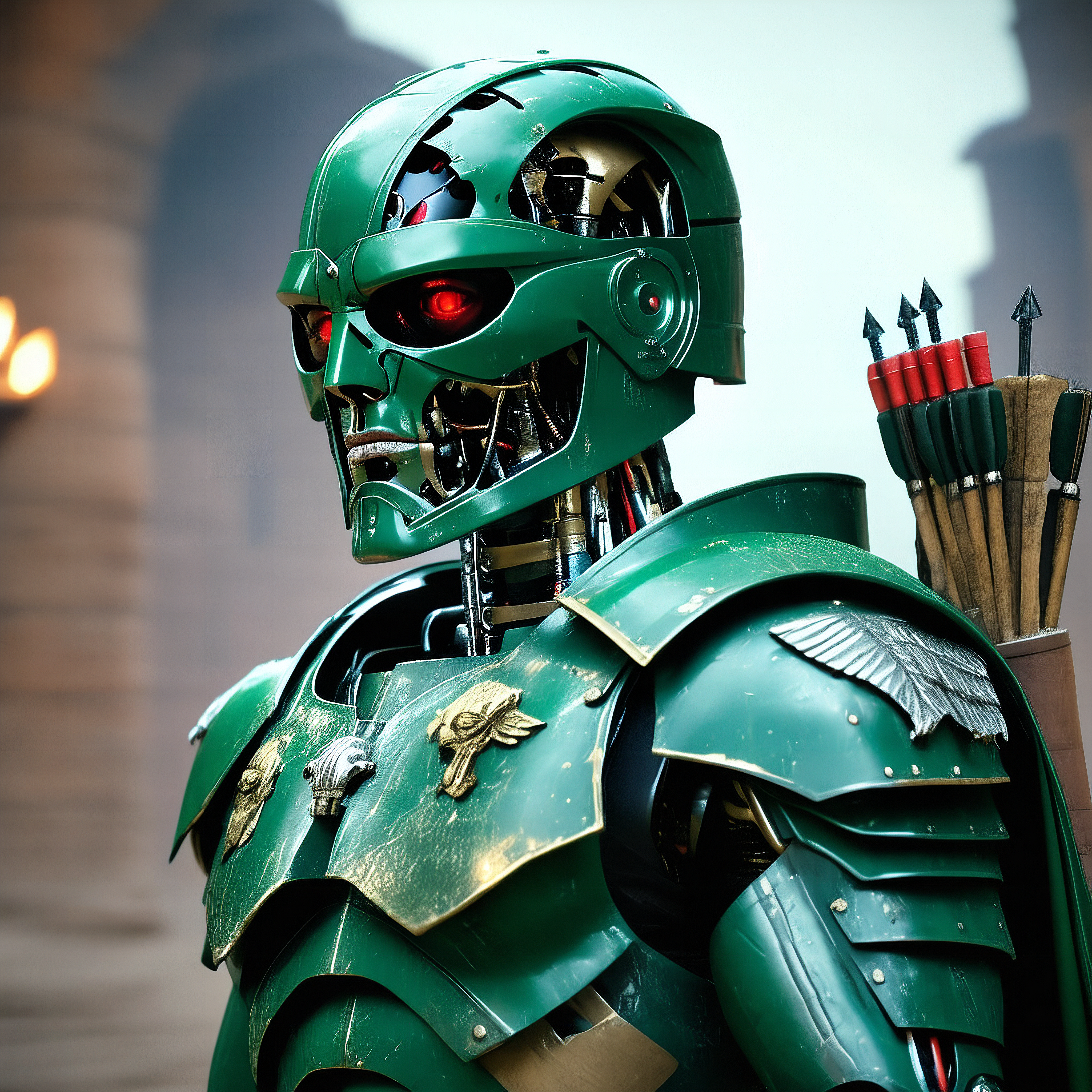
Also, my [Braiins Boiler Room](https://github.com/ttooccooll/braiinsboilerroom) now lets you see how many sats each of your miners are specifically contributing to your mining revenue.
Also, also, after watching [this video](https://www.youtube.com/watch?v=L2hE-kAnlvs), I've just been stuffing my s9 into my air intake after everyone goes to bed at night. I remove it in the morning to put back in the garage. It's a much cheaper alternative to the s19 he demonstrates with here and the noise doesn't disturb anyone since it's just become my after-hours furnace.
originally posted at https://stacker.news/items/782522
-

@ 005bc4de:ef11e1a2
2024-11-25 15:51:33
I edited the book "Kicking the Hornet's Nest" with all of Satoshi's writings. It's free, but there's a printed version also on Lulu and Amazon. Of course, they only take fiat. I want to offer "Kicking" for sale via bitcoin.
https://crrdlx.vercel.app/kicking/index.html
Here's what I want to happen:
1. Simple web form...person clicks buy-with-bitcoin. They enter their shipping address, they send BTC.
2. I get notified of shipping address and proof of payment.
3. I ship book.
I understand that btcpay is likely the gold standard for this and I've watched a few videos on it. But, that might be getting ahead of things at this point. Maybe in the future.
I'm looking for simple...I'm considering doing this just via dm and trust (trust is required anyway when buying online). I'd be saying, "You send me X sats and include your shipping address, I promise I'll send you the book." However, I'm sure there are more professional options out there.
SN has all the smart bitcoin kids, so I'm asking, "What are my options?"
originally posted at https://stacker.news/items/782304
-

@ 5d4b6c8d:8a1c1ee3
2024-11-25 14:36:10
Here are today's picks using my proprietary betting strategy at [Freebitcoin](https://freebitco.in/?r=51325722). For details about what Risk Balanced Odds Arbitrage is and why it works see https://stacker.news/items/342765/r/Undisciplined.
For a hypothetical 1k wager on each match, distribute your sats as follows:
| Outcome 1 | Outcome 2 | Outcome 3 | Bet 1 | Bet 2 | Bet 3 |
|--------------|-------------|--------------|-------|-------|-------|
| Real Betis| Barcelona | Draw | 220| 520 | 260|
| Leganes| Real Sociedad| Draw | 270| 420| 310|
| Girona| Real Madrid| Draw | 200| 580| 220|
| Atletico Madrid| Sevilla | Draw | 650| 140| 210|
| Napoli| Lazio| Draw | 500| 230| 270|
| Juventus| Bologna| Draw | 550| 190| 260|
| Inter Milan| Parma| Draw | 820| 60| 120|
| Atalanta| AC Milan| Draw | 480| 270| 250|
The most recent RBOA post that is entirely in the books is https://stacker.news/items/743312/r/Undisciplined. I won over 28k on these picks with just over 26k in wagers.
originally posted at https://stacker.news/items/782203
-

@ c11cf5f8:4928464d
2024-11-25 14:26:54
Are you a merchant? Having a shop? Doing commerce? Selling stuff for BTC? Or even you have friends with shops that start accepting Bitcoin?
After a year, I'd be interested to bring up an [old conversation](https://stacker.news/items/248871/r/AG), as it was really valuable! Share here your story (no need to give specific location details if you do not want to dox yourself), just explain how was your process and what would like to be better:
- type of shop your have (online or pupusas)
- type of commerce (retail, grocery, bar etc)
- how was the onboarding process
- what tools / apps you used, in the beginning and later
- how you improved your use of bitcoin apps
- how you consider bitcoin in your accounting (cash or "investment")
- anything you would like to share
We need more voice from Bitcoin merchants!
Speak loud!
originally posted at https://stacker.news/items/782196
-

@ 30ceb64e:7f08bdf5
2024-11-25 14:07:17
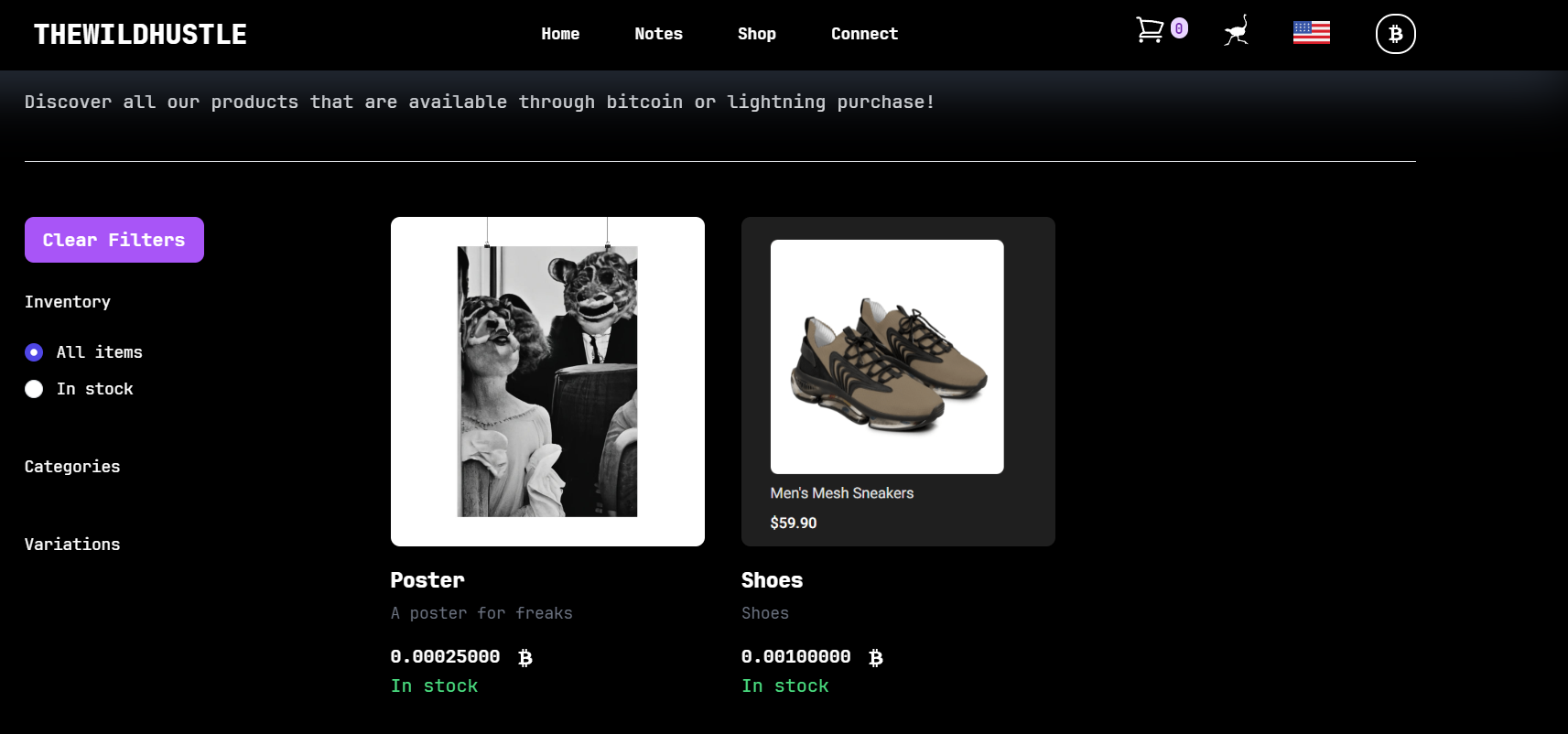
## Introduction
As Nostr continues to evolve, exciting opportunities are emerging for creators looking to build shops online . The age of the nostr shop is just begining. I'm staring at a the inevitability of extremely easy to setup shops from your most trusted npubs and creators, offering you cool products and services.
## Background
My bitcoin rabbit hole journey (which is probably another article I should write) coincided with me setting up a drop shipping Shopify store, selling remote work gear and merch to people who found themselves making that transition during the plandemic. Funnily enough, I find myself attempting to do similar things, but on top of bitcoin and nostr.
## Present
The combination of cypher.space and printify, allow me to sell custom products to freaks. Currently I have a pair of running shoes and a poster showing artwork from my album Animal Bus. Both of these items appear on Amethyst and Shopstr, and once i've tested the quality of the products and have ironed out any kinks, i'll advertise the shop to the wider nostr ecosystem. For the time being, the shop being a bit directionless is probably fine, over time i'll hone in on what my audience would be willing to buy, and offer pretty cool stuff.
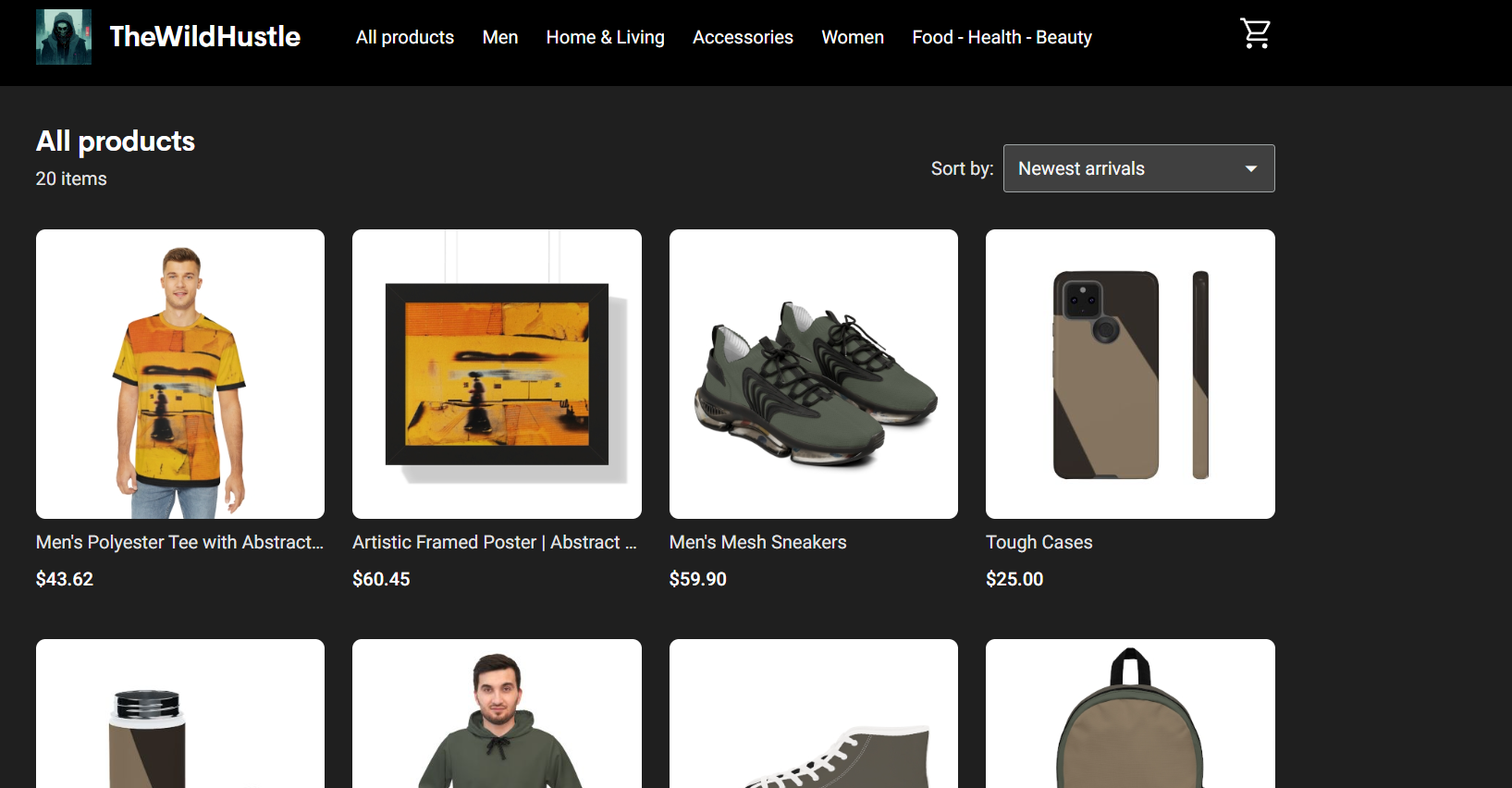
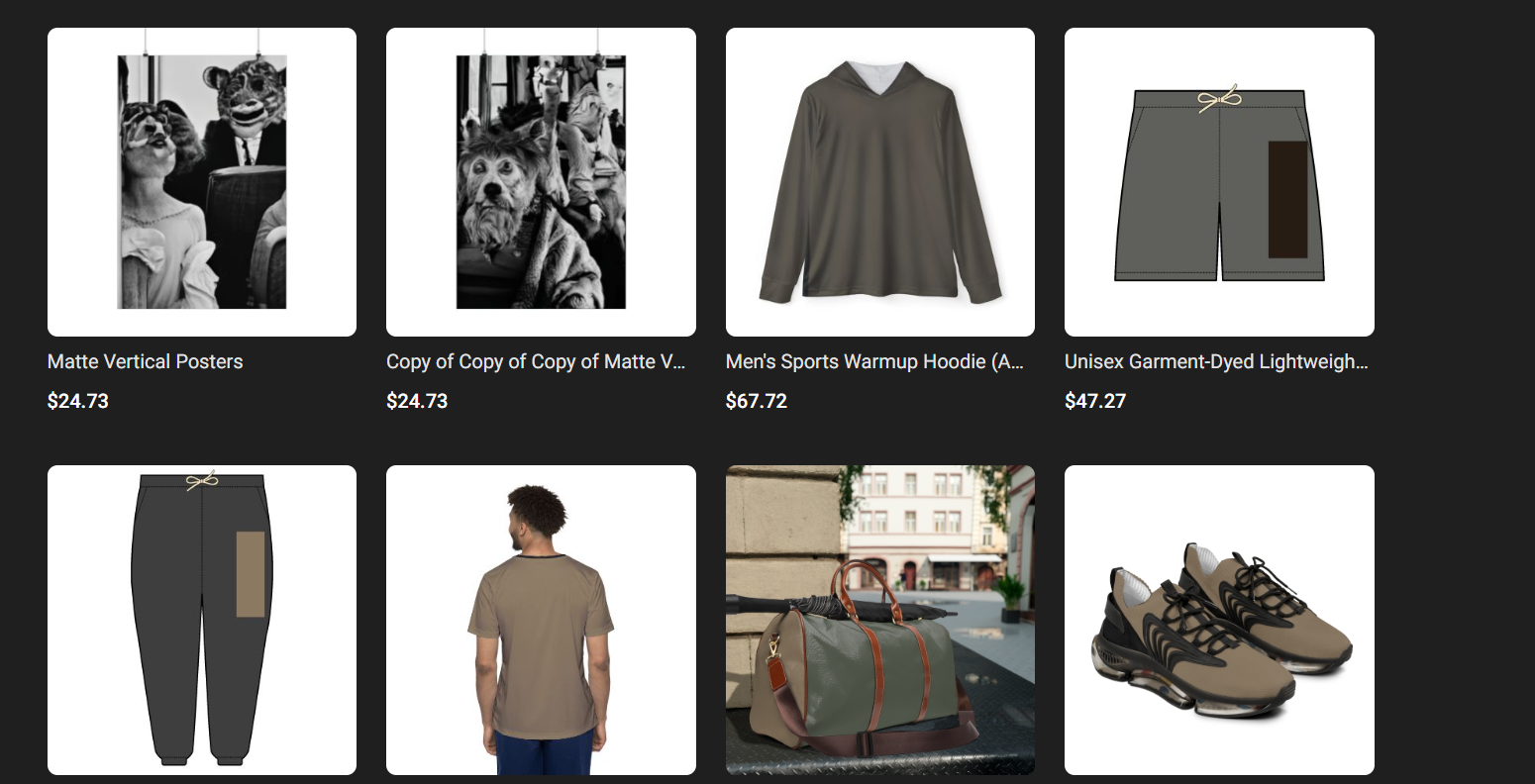
## Rough Roadmap
- Album Artwork as Posters
- Vinyl Records
- Notebooks
- Backpacks
- Clothes
- Shoes
I have all of these pretty much ready to go, but I'd want to re-do designs and test the quality of the products before promoting the stuff too heavily.
I'll sell a bunch of the stuff at a discount, as to generate orders so that I can increase my WOT score, and selling the products below the cost of production would probably market well in the short term.
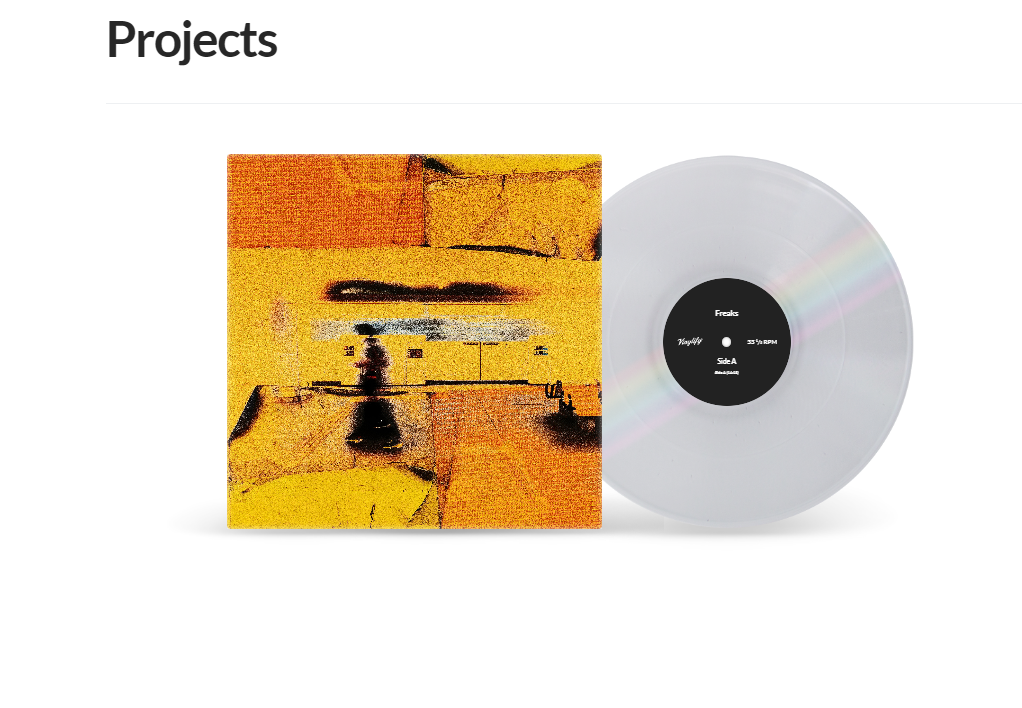

## Would be cool to sell
- Seed Signer Parts
- Blockstream Jades
- Meshtastic Stuff
- Bitaxe things
I could wait for an order and buy most of the stuff from a local computer store. Contributing to the decentralization of Free Open Source hardware sourcing would be pretty cool, and probably more important or attractive than selling t-shirts.
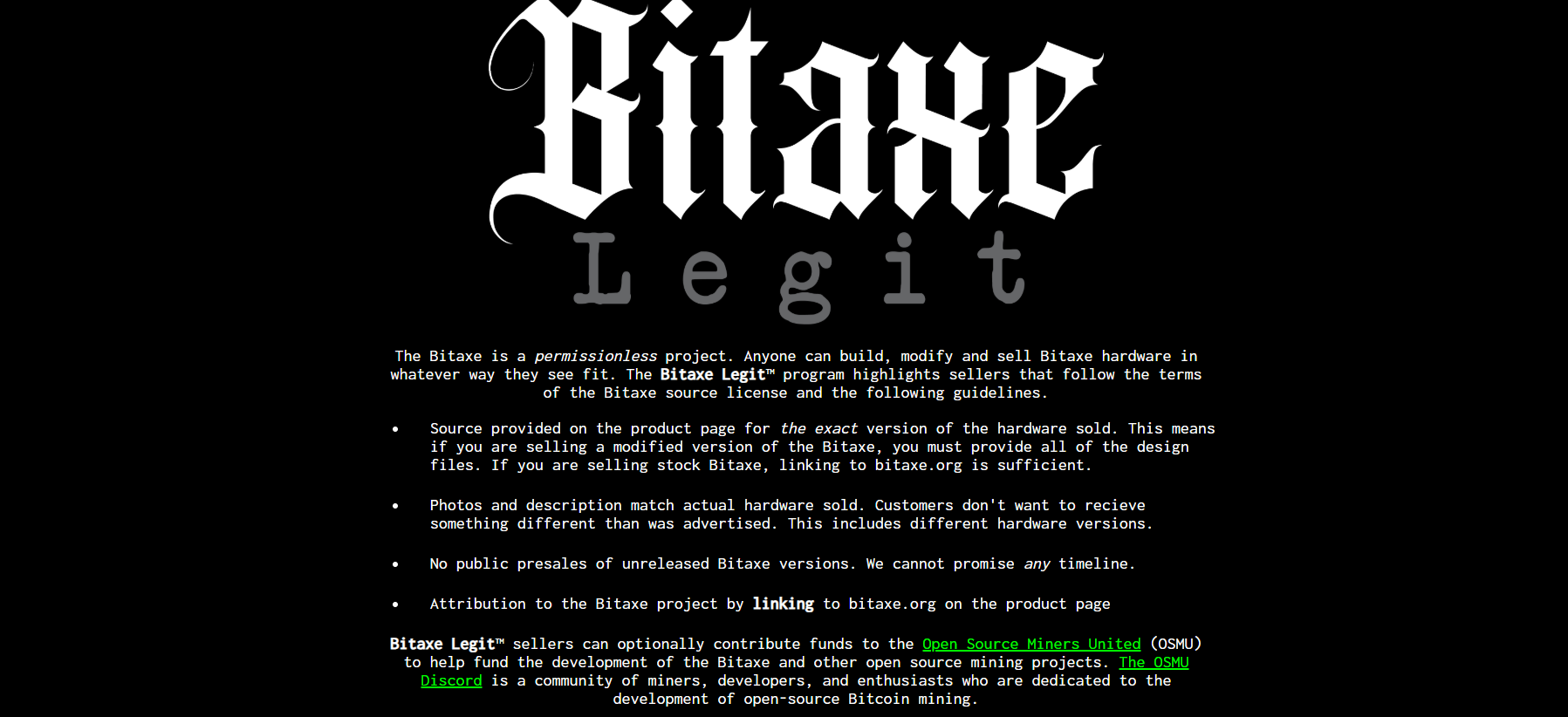
## Interoperability and Opportunity
Its pretty wild how Amethyst has Shopstr integrated into their client. Its pretty much the most popular non apple client on Nostr and as such, creates excellent visibility for sellers to showcase products. Right now the demand and listings aren't too great (which is probably great for people just starting out like me). I see Nostr as the new digital frontier with 100x growth over the horizon. I'm building a digital homestead and the shop hustle might be a few of the bricks in the wall.
## Intentions matter
I've been thinking a bit if my intentions were pure. I'm having fun creating these things and setting up some of this stuff, but I think its more important to do things for the right reason. I would hate to find myself larping or aiming for social media fame as an InFluEncer. Serving the community while getting zaps seems to be a primary motivator, I want to make zaps but I don't want to do it by selling my soul, grifting or scamming. I'll keep hustling wildly and if I think I've gone too far, ill try reeling it back I suppose.
## Conclusion
In Conclusion, this wild hustle is about they joy of building and contributing to a community, while enjoying a deeper sense of ownership, censorship resistance, and freedom. I'm looking forward to growing sustainably while providing value and receiving value in return.
Thanks,
Hustle
originally posted at https://stacker.news/items/782173
-

@ 5d4b6c8d:8a1c1ee3
2024-11-24 17:13:17
We're coming down the home stretch of the Group Play rounds. Remember that you won't be able to use your rebuys once we enter the Knockout Round.
# Tuesday's Games
- Bulls 1-1 @ Wizards 0-2
- Bucks 2-0 @ Heat 1-1
- Rockets 2-0 @ T-Wolves 1-1
- Spurs 1-1 @ Jazz 0-2
- Lakers 2-0 @ Suns 1-1
I need to decide between the Spurs and the Bulls. The Spurs might actually make it to the Knockout Rounds, but it's hard to imagine wanting to pick them then.
I'll post the segment of our podcast where we talk about the Emirates Cup before Tuesday, just in case our analysis sways any opinions.
# Prize: Almost 60k!
Thanks to recent zaps and @Carresan buying back in, we're closing in on a 60k prize.
originally posted at https://stacker.news/items/781193
-

@ 30ceb64e:7f08bdf5
2024-11-24 16:48:15
## A Personal Journey
I recently created a blog using npub.pro to consolidate my Nostr and Stacker News longform posts in one place. It's been a fun experiment, with surprising results.
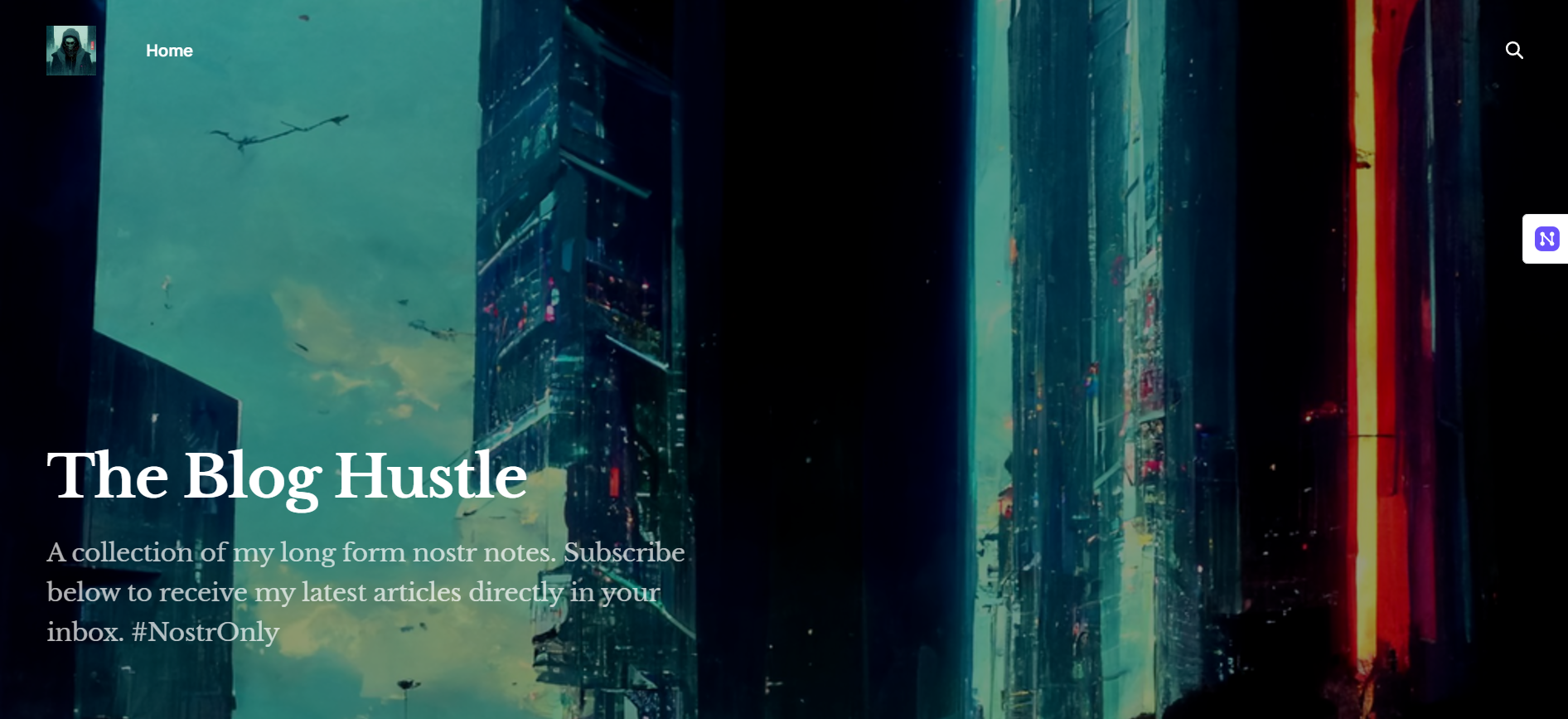
https://thebloghustle.npub.pro/
Primal now displays my SN and Nostr posts through a selection on my profile screen, and users are able to highlight, zap, and comment on the highlights of my articles.
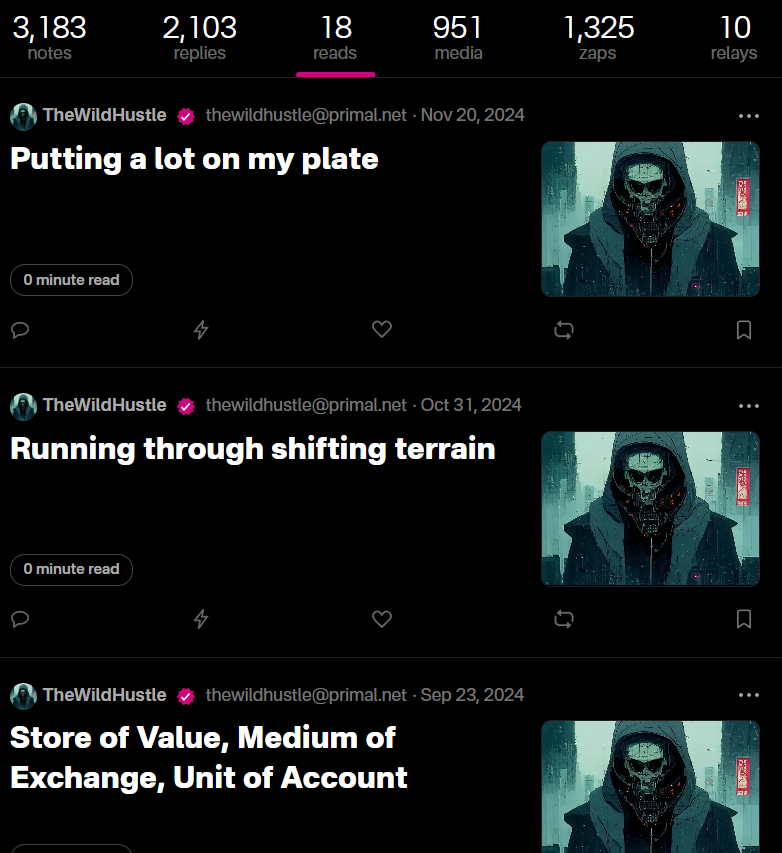
I need to write more, the blog will be something of an open diary, detailing my personal experience with Nostr and bitcoin.
## Why Nostr Outshines Substack and Medium
Nostr in my opinion has already surpassed the legacy blogging platforms. Functionalities like zapping, highlighting, and commenting, are fun and cool, but when added to Nostr's interconnectivity something magical appears. I had no idea my cross posted SN longform posts would be found in npub.pro, and I didn't know npub.pro had added longform support. Primal 2.0 was pretty much the nail in the coffin, the most popular Nostr client just added a reads section, supporting longform content. Nostr freaks like to talk about sovereignty and decentralization and owning your online identity, but the main attraction will probably be zaps, interconnectivity, and a more optimal UX.
Npub.pro, Cypher.space, Blogspot.io, Highlighter, Stacker News, Primal 2.0 and Yakihonne, are just the beginnings, but offer an amazing glimpse at what the future has in store. Keep in mind that these sites are sharing an average of around 10k daily active users, we'll see an explosion of content and a diversity of content because these platforms offer incentives and functionalities that you don't see elsewhere, as bitcoin becomes less taboo, and as the early adopter phase of these products comes to a close, we'll probably see v4v censorship resistance and digital ownership immensely supporting the underdog, the same underdog that just came over for the ease of use and hype.
So with that being said, highlight away. Let me know what you guys think about blogging on Nostr and if you think these are just the ramblings of a madman, or bland and obvious observations.
Thanks,
Hustle
originally posted at https://stacker.news/items/781159
-

@ 9358c676:9f2912fc
2024-11-24 16:44:55
### OBJECTIVE
Establish a hospital guide for thromboembolism prophylaxis in patients admitted to our center, aiming to increase safety in its indication, reduce the incidence of venous thrombosis events in at-risk patients, and minimize adverse effects related to thromboprophylaxis.
### SCOPE
All patients over 16 years of age admitted to our institution.
### RESPONSIBILITIES
Institution physicians from Hematology Services, Surgical Services, Medical Clinic Service, Intensive Care Unit (ICU), and Coronary Unit (CCU). Nursing Coordination. Nursing Department Head. Pharmacy Service.
### REFERENCES AND BIBLIOGRAPHY
1. Updated recommendations for prophylaxis of venous thromboembolic disease in Argentina. Fernando J. Vazquez et al. MEDICINA (Buenos Aires) 2020; 80: 69-80.
2. American Society of Hematology 2018 guidelines for management of venous thromboembolism: prophylaxis for hospitalized and nonhospitalized medical patients. Blood Advances. Holger J. Schünemann et al. DOI 10.1182/bloodadvances.2018022954
3. High-dose versus low-dose venous thromboprophylaxis in hospitalized patients with COVID-19: a systematic review and meta-analysis. Emanuele Valeriani et al. Internal and Emergency Medicine (2022) 17:1817–1825. https://doi.org/10.1007/s11739-022-03004-x
4. Recommendations for the use of thromboprophylaxis in hospitalized COVID-19 patients in Argentina. Fernando J. Vazquez et al. MEDICINA (Buenos Aires) 2020; Vol. 80 (Suppl. III): 65-66. ISSN 1669-9106
### INTRODUCTION
Thromboembolic prophylaxis in hospitalized patients is a critical intervention to reduce the risk of thrombotic events, one of the leading causes of morbidity and mortality in hospitals. Prolonged immobilization, along with other risk factors such as surgeries, trauma, comorbidities, and specific treatments, significantly increases the possibility of developing deep vein thrombosis (DVT) or pulmonary embolism (PE), which are serious conditions requiring rapid intervention and can threaten the patient's life.
In Argentina, the incidence of venous thromboembolism in hospitalized patients is high and represents a significant clinical challenge. It is estimated that hospitalization increases the likelihood of a thromboembolic event by 10 times compared to the general population. Implementing effective thromboprophylaxis strategies can reduce this incidence, optimizing patient safety and reducing both the costs associated with complications and the duration of hospitalization.
This guide aims to standardize the use of thromboprophylaxis in our institution, improving the identification of at-risk patients and promoting safe interventions based on updated scientific evidence.
### GENERAL RECOMMENDATIONS FOR THROMBOPROPHYLAXIS
General indications for thromboprophylaxis are essential to ensure safety and effectiveness in preventing thromboembolic events. The following general recommendations are provided:
Patients without prior anticoagulation: Recommendations apply only to patients who were not receiving oral anticoagulation before hospitalization.
Initial Evaluation: Prior to starting prophylaxis, a complete blood count, prothrombin time, aPTT, platelet count, and an estimation of renal function through creatinine clearance should be obtained.
Conditions for Surgical Patients: All recommendations for surgical patients assume no intraoperative hemorrhagic complications and adequate hemostasis.
Special Cases Evaluation: Prophylaxis in patients with extreme weight (under 40 kg or over 100 kg) or renal insufficiency with a clearance of less than 30 ml/min should be evaluated by a Hematology specialist.
Early Mobilization: Routine early mobilization is recommended for all patients, as long as it is safe.
### RISK SCALE APPLICATION
The assessment of thromboembolic event risk should begin with correct categorization of the patient.
Risk Factors for Venous Thromboembolism (VTE) during hospitalization include: prolonged immobility (more than 2 days in bed), active neoplasms, stroke, heart failure, chronic obstructive pulmonary disease, acute infections, rheumatoid arthritis, ANCA-positive vasculitis, and inflammatory bowel disease. These clinical conditions account for approximately 75% of fatal pulmonary thromboembolisms (PE), highlighting the importance of adequate antithrombotic prophylaxis.
Risk scales are designed to categorize both thromboembolic risk and bleeding risk associated with thromboprophylaxis. The bleeding risk, whether major or clinically relevant, increases with the use of pharmacological prophylaxis. However, fatal bleeding is 10 times less frequent than fatal PE.
### CONTRAINDICATIONS FOR THROMBOPROPHYLAXIS
General contraindications for pharmacological thromboprophylaxis in hospitalized patients aim to prevent hemorrhagic complications. The main contraindications are:
Clinically significant active hemorrhage.
Platelet count less than 30,000 platelets/ml.
Major bleeding disorders.
Active intracranial hemorrhage.
Recent peri-spinal hemorrhage.
Recent surgery with a high risk of bleeding.
It is crucial to carefully evaluate these contraindications before initiating any thromboprophylaxis protocol.
### INDICATION FOR THROMBOPROPHYLAXIS IN NON-SURGICAL HOSPITALIZED PATIENTS
Validated scales help categorize the risk and indication for venous thromboembolism (VTE) in hospitalized patients with non-surgical conditions: the IMPROVE-VTE scale, which considers a high risk for scores above 2 and very high risk for scores above 4, with additional factors such as elevated D-dimer, advanced age, cancer history, or previous VTE.
To assess bleeding risk, the IMPROVE-Bleed scale identifies high risk with scores of 7 or higher. Patients with high VTE risk and low bleeding risk should receive prophylaxis until discharge, and in high-risk cases, it should be extended afterward.
Improve VTE Scale
Risk Factors and Points
Patient History
Previous VTE: 3 points
Recent surgery (within 30 days): 2 points
Active cancer: 2 points
History of trauma: 1 point
Clinical Factors
Age 40-60 years: 1 point
Age over 60 years: 2 points
Obesity (BMI > 30): 1 point
Prolonged immobility (e.g., bed rest for more than 3 days): 2 points
Laboratory Findings
Elevated D-dimer: 1 point
Medication Use
Hormonal therapy (e.g., oral contraceptives, hormone replacement therapy): 1 point
Recent chemotherapy: 2 points
Physical Examination
Signs of deep vein thrombosis (DVT) such as swelling, pain, or tenderness in the legs: 2 points
Scoring and Risk Levels
Total Score Calculation: Add the points from all applicable categories.
Risk Levels:
0-2 points: Low Risk - Standard care; consider early mobilization.
3-5 points: Moderate Risk - Consider mechanical prophylaxis (e.g., compression stockings) and/or pharmacologic prophylaxis.
6+ points: High Risk - Strongly consider pharmacologic prophylaxis (e.g., anticoagulants) and close monitoring.
IMPROVE-Bleed Scale for Bleeding Risk Assessment
Risk Factors and Points
Creatinine clearance < 60 ml/min: 1 point
Creatinine clearance < 30 ml/min: 2.5 points
Male sex: 1 point
Age > 40: 1.5 points
Age > 85: 3.5 points
Active cancer: 2 points
Rheumatic disease: 2 points
Central venous catheter: 2 points
Admission to Intensive Care Unit or Coronary Care Unit: 2.5 points
Liver failure (INR > 1.5): 2.5 points
Platelets < 50,000/µl: 4 points
Bleeding in the last 3 months: 4 points
Active gastroduodenal ulcer: 4.5 points
Score Interpretation
Total Score:
7 or more: High bleeding risk, with a bleeding probability of 4% or more.
General Recommendations for Thromboprophylaxis in Hospitalized Patients with Medical Conditions Without Surgery
Population: High or moderate risk of thrombosis and low risk of bleeding
Recommendation: Critical: Enoxaparin 40 mg every 24 hours; Non-critical: Enoxaparin 40 mg every 24 hours.
Alternative: Unfractionated heparin 5000 U every 8 or 12 hours.
Comments: Until discharge; may consider prolonging in very high-risk patients.
Population: High risk of thrombosis and high risk of bleeding
Recommendation: IPC or CG.
Alternative: -
Comments: -
Population: Patients with creatinine clearance less than 30 ml/min
Recommendation: Unfractionated heparin 5000 U every 8 or 12 hours.
Alternative: -
Comments: Reassess pharmacological prophylaxis when there is low bleeding risk. Until discharge; may consider prolonging in very high-risk patients.
Population: Patients with ischemic stroke
Recommendation: Enoxaparin 40 mg every 24 hours.
Alternative: IPC.
Comments: Initiate within the first 24 hours. Until discharge; may consider prolonging in very high-risk patients.
Population: Patients with intracranial hemorrhage (ICH)
Recommendation: IPC or CG for 48 to 72 hours after stabilization of bleeding, then Enoxaparin 40 mg every 24 hours.
Alternative: IPC.
Comments: Hemorrhage control should be monitored with imaging and evolution. Until discharge; may consider prolonging in very high-risk patients.
Population: Patients with subarachnoid hemorrhage (SAH)
Recommendation: IPC or CG for 48 to 72 hours after vascular exclusion or surgical resolution of the aneurysm, then Enoxaparin 40 mg every 24 hours.
Alternative: IPC.
Comments: Hemorrhage control should be monitored with imaging and evolution. Until discharge; may consider prolonging in very high-risk patients.
References
UFH: unfractionated heparin
IPC: intermittent pneumatic compression
CG: graduated compression stockings
CVA: cerebrovascular accident
ICH: intracranial hemorrhage
SAH: subarachnoid hemorrhage
### RECOMMENDATIONS FOR THROMBOPROPHYLAXIS IN HOSPITALIZED PATIENTS WITH NON-SURGICAL CLINICAL DISEASES
Population Recommendation Alternative Comments Duration
High or moderate VTE risk with low bleeding risk Enoxaparin 40 mg every 24 hours (critical); Enoxaparin 40 mg every 24 hours (non-critical) Unfractionated heparin (UFH) 5000 U every 8 or 12 hours Until discharge, may be extended for very high-risk patients
High VTE risk and high bleeding risk CNI or MCG
Creatinine clearance < 30 ml/min UFH 5000 U every 8 or 12 hours Reevaluate when bleeding risk is low Until discharge, may be extended for very high-risk patients
### INDICATION FOR THROMBOPROPHYLAXIS IN SURGICAL PATIENTS WITH NON-ORTHOPEDIC SURGERY
In non-ambulatory surgeries, the risk of venous thromboembolism (VTE) varies depending on the patient's risk factors and the type of surgery. Thus, individual risk stratification using the Caprini scale is imperative. Patients undergoing general surgery with moderate or higher thrombotic risk (3 points or more) and no increased bleeding risk should receive pharmacological thromboprophylaxis.
### CAPRINI SCALE
Caprini Risk Assessment Model
Risk Factors and Points
History of VTE
Previous VTE: 3 points
Active Cancer
Current or recent cancer (within 6 months): 3 points
Receiving treatment for cancer: 3 points
Surgery/Trauma
Major surgery (e.g., orthopedic, abdominal) with general anesthesia: 2 points
Trauma with hospitalization: 2 points
Minor surgery with general anesthesia: 1 point
Age
Age 41-60 years: 1 point
Age 61-74 years: 2 points
Age 75 years or older: 3 points
Obesity
BMI 30-39: 1 point
BMI 40 or greater: 2 points
Immobility
Bed rest for more than 3 days: 2 points
Paraplegia or quadriplegia: 2 points
Hormonal Therapy
Current use of estrogen-containing medications (e.g., oral contraceptives, hormone replacement therapy): 1 point
Other Risk Factors
History of heart failure or chronic lung disease: 1 point
History of inflammatory bowel disease: 1 point
History of stroke: 1 point
Varicose veins: 1 point
Recent travel (long-distance travel > 4 hours): 1 point
Scoring and Risk Levels
Total Score Calculation: Add the points from all applicable categories.
Risk Levels:
0-1 points: Low Risk - Standard care; consider early mobilization.
2-3 points: Moderate Risk - Consider mechanical prophylaxis (e.g., compression stockings).
4-5 points: High Risk - Consider pharmacologic prophylaxis (e.g., anticoagulants) and mechanical methods.
6+ points: Very High Risk - Strongly consider pharmacologic prophylaxis and close monitoring.
Interpretation of Caprini Scale Score:
Total Score Risk Level Recommendation Comments Duration
0-1 Low Early ambulation Until discharge
2-3 Moderate CNI or MCG Begin before surgery Until discharge
4 or more High Enoxaparin 40 mg every 24 hours or UFH 5000 U every 8 or 12 hours Administer the dose 12 hours before abdominal or pelvic surgery, restart 12 hours post-procedure Minimum 7-10 days; extend up to 28 days post-surgery for oncologic patients or those with low bleeding risk
### INDICATION FOR THROMBOPROPHYLAXIS IN ORTHOPEDIC SURGERY PATIENTS
Major orthopedic surgeries, including total hip arthroplasty (THA), total knee arthroplasty (TKA), and hip fracture surgery (HFS), pose a high risk of thrombotic complications (40%-60% without proper thromboprophylaxis). Pharmacological prophylaxis is always recommended regardless of individual risk factors.
Special Considerations:
In outpatient knee arthroscopy, early ambulation is encouraged. Prophylaxis with low molecular weight heparin (LMWH) for at least 7 days should be considered only in patients with high thrombotic risk (e.g., cancer, prior VTE, obesity, pregnancy, or postpartum).
For immobilization lasting more than 7 days due to fractures, tendinous/cartilaginous ruptures, or soft tissue injuries below the patella with risk factors, pharmacological prophylaxis with LMWH is recommended.
In spinal surgeries, mechanical prophylaxis with graduated compression stockings (GCS) and early ambulation are advised. For prolonged procedures (> 1 hour) with risk factors, pharmacological prophylaxis (Enoxaparin 40 mg/day or UFH 5000 U every 8-12 hours) is recommended 24 hours post-surgery until discharge.
Major trauma patients should receive mechanical prophylaxis (CNI or GCS) combined with Enoxaparin 40 mg/day, provided there is no increased bleeding risk, from admission to discharge, including the rehabilitation period.
Recommendations for Orthopedic Surgery:
Population Recommended Indication Alternative Comments Duration
Scheduled hip and knee surgery Apixaban 2.5 mg every 12 hours None Start 12 hours after surgery 28-35 days (hip), 14 days (knee), extend up to 35 days
Dabigatran 220 mg every 24 hours None Start 110 mg 4 hours after surgery, then continue with 220 mg
Enoxaparin 40 mg every 24 hours None Start 12 hours after surgery
Rivaroxaban 10 mg every 24 hours None Start 6 hours after surgery
Hip fracture Enoxaparin 40 mg every 24 hours UFH 5000 U every 8 or 12 hours Administer from admission and 8-12 hours before surgery; restart 8-12 hours post-surgery 14-35 days
### INDICATION FOR THROMBOPROPHYLAXIS IN OBSTETRICS
Postpartum hemorrhage is the leading cause of maternal death in developing countries, while venous thromboembolic disease (VTE) is the leading cause in developed countries. Pregnant women are 4-10 times more likely to develop VTE than non-pregnant women. Although the incidence of VTE during pregnancy is low (1-2 per 1000 pregnancies), it is considered a preventable cause of maternal death.
### INDICATION FOR THROMBOPROPHYLAXIS IN COVID-19 HOSPITALIZED PATIENTS
COVID-19 infection is associated with an increased risk of venous thromboembolism, particularly in ICU patients. These patients are considered at high thrombotic risk during hospitalization, with significantly elevated D-dimer levels linked to greater risk of severe complications, including death and disseminated intravascular coagulation.
Recommendations:
Thromboprophylaxis with subcutaneous Enoxaparin 40 mg/day is suggested for all hospitalized patients with severe COVID-19, provided no contraindications exist, throughout their hospital stay.
-

@ 5d4b6c8d:8a1c1ee3
2024-11-24 16:07:15
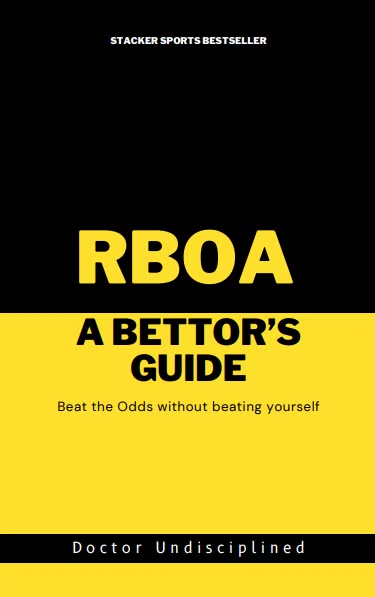
Here are today's picks using my proprietary betting strategy at [Freebitcoin](https://freebitco.in/?r=51325722). For details about what Risk Balanced Odds Arbitrage is and why it works see https://stacker.news/items/342765/r/Undisciplined.
For a hypothetical 1k wager on each match, distribute your sats as follows:
| Outcome 1 | Outcome 2 | Outcome 3 | Bet 1 | Bet 2 | Bet 3 |
|--------------|-------------|--------------|-------|-------|-------|
| Torino| Napoli| Draw | 210| 500| 290|
| Lecce| Juventus| Draw | 180| 560| 260|
| Fiorentina| Inter Milan| Draw | 260| 480| 260|
| Tottenham| Fullham| Draw | 580| 200| 220|
| Southampton| Chelsea| Draw | 160| 620| 220|
| Newcastle| Liverpool| Draw | 250| 490| 260|
| Man United| Everton| Draw | 640| 150| 210|
| Man City| Nottingham Forest| Draw | 800| 60| 140|
| Liverpool| Man City| Draw | 440| 300| 260|
| Chelsea| Aston Villa| Draw | 530| 240| 230|
| Bournemouth| Tottenham| Draw | 310| 440| 250|
| Arsenal| Man United| Draw | 600| 180| 220|
Yesterday's soccer and Formula 1 events brought in almost 28k on 24k of wagers. The soccer matches came out slightly ahead, but Formula 1 was a huge winner.
originally posted at https://stacker.news/items/781101
-

@ c11cf5f8:4928464d
2024-11-24 15:35:48
Let's hear some of your latest Bitcoin purchases, feel free to include links to the shops or merchants you bought from too.
If you missed our last thread, [here](https://stacker.news/items/771187/r/AG) are some of the items stackers recently spent their sats on.
originally posted at https://stacker.news/items/781052
-

@ 6f170f27:711e26dd
2024-11-24 10:30:08
> Google to sell Chrome AND be banned from re-entering the browser market for five years, recommends US Department of Justice. ([source](https://www.pcgamer.com/hardware/Google-should-sell-chrome-and-more-recommends-US-DoJ/?utm_campaign=socialflow))
What now? Despite Alphabet probably battling this in court I'm interested in how this might affect the internet and literally the world. Here are a few of my unorganized early thoughts, **feel free to share your predictions too**
1. Chrome itself isn't profitable. Alphabet might try to **sell it to another tech firm**. Microsoft, Apple, Meta, Amazon have probably no interest in running it. Second row tech firms like Oracle, IBM, Atlassian, Salesforce, SAP might have big interest in this but will probably not allowed by regulators.
2. Chrome will IPO independently. It's not unprecedented for a divested spinoff to IPO. Then Chrome will have to make money by itself. Would you be willing to **buy Chrome premium subscription**? Or do you think corporate customers would be willing to pay while private usage stays free?
3. Making all Users pay would fundamentally transform the internet. **Imagine a world were Web access costs monthly**. The direction in which money flows from user to browser to website would be fundamentally different from the ad-infrastructure
4. Mozilla **Firefox** might not survive this. If Google is forced to stop paying all browser companies we might end up with a 90% Chrome monopoly and 10% Apple adopting the features Chromium forces them to adopt.
5. **Tor Browser** is in deep trouble. Without relying on Firefox we might need to rewrite Tor ontop of Chromium. Depressing thought.
6. App internet vs Web internet. The balance might shift. If Chrome costs money and Firefox dies we might see a decline in Web usage and rise of Apps using APIs instead. It's not a prison but can you already feel the walls getting closer?
originally posted at https://stacker.news/items/780740
-

@ 07907690:d4e015f6
2024-11-24 09:38:58
Karena orang tuanya yang berasal dari Hongaria telah melarikan diri dari rezim Soviet pascaperang untuk menetap di Amerika Serikat, Nick Szabo menganggap daerah Teluk California pada tahun 1990-an sebagai rumahnya. Di sana, ia termasuk orang pertama yang sering menghadiri pertemuan tatap muka "Cypherpunk" yang diselenggarakan oleh Timothy May, Eric Hughes, dan anggota pendiri kolektif kriptografer, programmer, dan aktivis privasi lainnya yang berpusat di sekitar milis tahun 90-an dengan nama yang sama.
Seperti Cypherpunk lainnya, Szabo khawatir dengan jaminan privasi yang semakin berkurang di era digital yang akan datang dan mengambil tindakan untuk membendung gelombang tersebut semampunya. Misalnya, di milis Cypherpunk, Szabo memimpin penentangan terhadap "[_Chip Clipper_](https://en.wikipedia.org/wiki/Clipper_chip)", sebuah chip yang diusulkan untuk disematkan di telepon, yang memungkinkan NSA untuk mendengarkan panggilan telepon. Szabo memiliki bakat khusus untuk menjelaskan risiko pelanggaran privasi tersebut dengan cara yang dapat diterima oleh orang-orang yang tidak memiliki latar belakang teknis, terkadang memberikan ceramah tentang topik tersebut atau bahkan membagikan brosur. (Chip tersebut akhirnya ditolak oleh produsen dan konsumen.)
Namun seperti Cypherpunk yang lebih berorientasi libertarian, minat Szabo dalam privasi digital adalah bagian dari gambaran yang lebih besar — ini bukan hanya tentang privasi saja. Terinspirasi oleh visi Timothy May sebagaimana yang ditetapkan dalam [_The Crypto Anarchist Manifesto_](https://activism.net/cypherpunk/crypto-anarchy.html), Szabo melihat potensi untuk menciptakan "Galt's Gulch" di dunia maya: domain tempat individu dapat berdagang dengan bebas, seperti yang dijelaskan oleh novel penulis libertarian Ayn Rand, _Atlas Shrugged_. Medan gaya pseudo-fisika dari cerita tersebut, May dan Szabo percaya, dapat digantikan dengan keajaiban kriptografi kunci publik yang baru-baru ini ditemukan.
“Jika kita mundur sejenak dan mencermati apa yang ingin dicapai oleh banyak cypherpunk, tema idealis utamanya adalah dunia maya Ghana di mana kekerasan hanya bisa menjadi khayalan, entah itu dalam Mortal Komat \[sic\] atau 'perang api',” [tulis](https://cypherpunks.venona.com/date/1995/09/msg01303.html) Szabo dalam milis Cypherpunks.
Namun, Szabo juga menyadari bahwa perusahaan bebas membutuhkan lebih dari sekadar enkripsi sebagai lapisan keamanan. Terinspirasi oleh penulis libertarian lainnya — ekonom Friedrich Hayek — ia menemukan bahwa dasar masyarakat manusia, sebagian besar, didasarkan pada komponen dasar, seperti properti dan kontrak, yang biasanya ditegakkan oleh negara. Untuk menciptakan alternatif dunia maya tanpa negara dan tanpa kekerasan, Szabo tahu bahwa komponen dasar ini harus dipindahkan ke ranah daring.
Beginilah cara Szabo, pada pertengahan 1990-an, mengusulkan sesuatu yang mungkin paling dikenalnya saat ini: _kontrak pintar_. Protokol komputer (yang saat itu masih hipotetis) ini dapat memfasilitasi, memverifikasi, dan menegakkan negosiasi atau pelaksanaan kontrak secara digital, idealnya tanpa memerlukan pihak ketiga mana pun. Seperti yang pernah [dikatakan](http://www.fon.hum.uva.nl/rob/Courses/InformationInSpeech/CDROM/Literature/LOTwinterschool2006/szabo.best.vwh.net/ttps.html) Szabo : "Pihak ketiga yang tepercaya adalah lubang keamanan." Lubang keamanan ini akan menjadi target peretas atau penjahat — serta negara-negara bangsa selama masa ketidakstabilan politik atau penindasan.
Namun kontrak pintar hanyalah sebagian dari teka-teki. Alat kedua yang dibutuhkan Szabo untuk mewujudkan "Galt's Gulch" mungkin bahkan lebih penting. Uang.
Uang Elektronik
---------------
Mata uang digital, uang tunai untuk internet, selalu menjadi [tujuan utama](https://cypherpunks.venona.com/date/1993/08/msg00426.html) Cypherpunk. Namun, hanya sedikit yang mendalami pokok bahasan tersebut seperti yang dilakukan Szabo.
Dalam esainya _"Shelling Out: The Origins of Money,"_ Szabo [menjelaskan](https://nakamotoinstitute.org/shelling-out/) bagaimana — seperti yang [pertama kali dihipotesiskan](https://books.google.nl/books?id=ekonDAAAQBAJ&pg=PA244&lpg=PA244&dq=Richard+Dawkins+money+as+a+formal+token+of+reciprocal+altruism&source=bl&ots=kBbSS-l5AC&sig=fr85YmvwkvFqWcOYLBkL10O7sI4&hl=en&sa=X&redir_esc=y#v=onepage&q=Richard20Dawkins20money20as20a20formal20token20of20reciprocal20altruism&f=false) oleh ahli biologi evolusi Richard Dawkins — penggunaan uang telah tertanam dalam DNA manusia. Setelah menganalisis masyarakat pra-peradaban, Szabo menemukan bahwa orang-orang di berbagai budaya cenderung mengumpulkan benda-benda langka dan mudah dibawa, sering kali untuk dijadikan perhiasan. Benda-benda inilah yang berfungsi sebagai uang, yang pada gilirannya memungkinkan manusia untuk bekerja sama: "altruisme timbal balik" teori permainan melalui perdagangan, dalam skala besar dan lintas waktu.
Szabo juga sangat tertarik pada [perbankan bebas](https://en.wikipedia.org/wiki/Free_banking), sebuah pengaturan moneter yang didukung oleh Hayek, di mana bank swasta menerbitkan mata uang mereka sendiri yang tidak terikat pada negara tertentu. Di bawah sistem seperti itu, pasar bebas sepenuhnya bebas menentukan mata uang mana yang akan digunakan. Meskipun merupakan ide baru saat ini (dan bahkan lebih baru lagi pada tahun-tahun sebelum Bitcoin), perbankan bebas merupakan kenyataan di Amerika Serikat pada tahun 1800-an, serta di beberapa negara lain.
Szabo juga melanjutkan untuk menerapkan minatnya dalam praktik dan menjual keahliannya sebagai _konsultan perdagangan internet_ pada pertengahan 1990-an, jauh sebelum kebanyakan orang melihat potensi perdagangan daring. Yang paling menonjol, ia menghabiskan waktu bekerja di perusahaan rintisan DigiCash milik David Chaum, yang berkantor pusat di Amsterdam. Perusahaan Chaum memperkenalkan uang digital pertama yang pernah ada di dunia dalam bentuk [eCash](https://bitcoinmagazine.com/articles/genesis-files-how-david-chaums-ecash-spawned-cypherpunk-dream): sarana untuk melakukan pembayaran daring yang sama rahasianya dengan uang tunai di dunia nyata.
Namun, di DigiCash pula Szabo mengetahui risiko solusi Chaum. DigiCash adalah perusahaan terpusat, dan Szabo merasa terlalu mudah baginya dan orang lain untuk mengutak-atik saldo orang lain jika mereka mau. Bagaimanapun, pihak tepercaya adalah celah keamanan, dan risiko ini mungkin tidak lebih besar daripada risiko dalam hal uang.
“Masalahnya, singkatnya, adalah bahwa uang kita saat ini bergantung pada kepercayaan pada pihak ketiga untuk menentukan nilainya,” Szabo [berpendapat](https://unenumerated.blogspot.com/2005/12/bit-gold.html) pada tahun 2005. “Seperti yang ditunjukkan oleh banyak episode inflasi dan hiperinflasi selama abad ke-20, ini bukanlah keadaan yang ideal.”
Bahkan, ia menganggap masalah kepercayaan ini sebagai hambatan yang bahkan solusi perbankan bebas pada umumnya bisa mengalaminya: “\[P\]enerbitan uang kertas swasta, meski memiliki berbagai kelebihan dan kekurangan, juga bergantung pada pihak ketiga yang terpercaya.”
Szabo tahu ia ingin menciptakan bentuk uang baru yang tidak bergantung pada kepercayaan pada pihak ketiga mana pun.
Berdasarkan analisisnya terhadap uang prasejarah, Szabo telah menempuh perjalanan panjang dalam menentukan seperti apa bentuk uang idealnya. Pertama, uang tersebut harus “aman dari kehilangan dan pencurian yang tidak disengaja.” Kedua, nilainya harus “sangat mahal dan tidak dapat dipalsukan, sehingga dianggap berharga.” Dan ketiga: “Nilai ini \[harus\] diperkirakan secara akurat melalui pengamatan atau pengukuran sederhana.”
Dibandingkan dengan logam mulia seperti emas, Szabo ingin menciptakan sesuatu yang digital dan langka, di mana kelangkaan ini tidak bergantung pada kepercayaan pihak ketiga. Ia ingin menciptakan emas digital.
> Logam mulia dan barang koleksi memiliki kelangkaan yang tidak dapat dipalsukan karena mahalnya biaya pembuatannya. Hal ini pernah menghasilkan uang yang nilainya sebagian besar tidak bergantung pada pihak ketiga yang tepercaya. Namun, logam mulia memiliki masalah. \[…\] Jadi, akan sangat bagus jika ada protokol yang memungkinkan bit yang sangat mahal dapat dibuat secara daring dengan ketergantungan minimal pada pihak ketiga yang tepercaya, lalu disimpan, ditransfer, dan diuji dengan aman dengan kepercayaan minimal yang serupa. Bit Gold.
Bit Gold
--------
Szabo pertama kali mencetuskan Bit Gold pada tahun 1998, meskipun ia baru [menjelaskannya secara lengkap](https://unenumerated.blogspot.com/2005/12/bit-gold.html) di depan publik pada [tahun 2005](http://web.archive.org/web/20060329122942/http://unenumerated.blogspot.com/2005/12/bit-gold.html). Skema uang digital yang diusulkannya terdiri dari kombinasi berbagai solusi, beberapa di antaranya terinspirasi oleh (atau menyerupai) konsep uang elektronik sebelumnya.
Properti utama pertama Bit Gold adalah _proof of work_, trik kriptografi yang digunakan oleh Dr. Adam Back dalam "mata uang anti-spam" miliknya, [Hashcash](https://bitcoinmagazine.com/articles/genesis-files-hashcash-or-how-adam-back-designed-bitcoins-motor-block). _Proof of work_ merupakan biaya yang tidak dapat dipalsukan yang dicari Szabo, karena memerlukan sumber daya dunia nyata — daya komputasi — untuk menghasilkan bukti-bukti ini.
Sistem pembuktian kerja Bit Gold dimulai dengan "string kandidat": pada dasarnya angka acak. Siapa pun dapat mengambil string ini dan secara matematis menggabungkannya — "hash" — dengan angka acak lain yang baru dibuat. Berdasarkan sifat hashing, hasilnya akan menjadi string angka baru yang tampak acak: hash. Satu-satunya cara untuk mengetahui seperti apa hash ini adalah dengan benar-benar membuatnya — hash tidak dapat dihitung atau diprediksi dengan cara lain.
Triknya, yang juga digunakan dalam Hashcash, adalah bahwa tidak semua hash dianggap valid dalam protokol Bit Gold. Sebaliknya, hash yang valid harus, misalnya, dimulai dengan sejumlah angka nol yang telah ditentukan sebelumnya. Karena sifat hashing yang tidak dapat diprediksi, satu-satunya cara untuk menemukan hash yang valid adalah dengan coba-coba. Oleh karena itu, hash yang valid membuktikan bahwa pembuatnya telah mengeluarkan daya komputasi.
Hash yang valid ini, pada gilirannya, akan menjadi string kandidat Bit Gold berikutnya. Oleh karena itu, sistem Bit Gold akan berkembang menjadi rantai hash proof-of-work, dan akan selalu ada string kandidat berikutnya untuk digunakan.
Siapa pun yang menemukan hash yang valid akan secara harfiah memiliki hash tersebut, mirip dengan bagaimana orang yang menemukan sedikit bijih emas memilikinya. Untuk menetapkan kepemilikan ini secara digital, Bit Gold menggunakan [registri kepemilikan digital](https://nakamotoinstitute.org/secure-property-titles/#selection-125.47-125.66) : blok penyusun lain yang terinspirasi Hayek yang diusulkan oleh Szabo. Dalam registri ini, hash akan ditautkan ke kunci publik dari masing-masing pembuatnya.
Melalui registri kepemilikan digital ini pula, hash dapat ditransfer ke pemilik baru: Pemilik asli secara harfiah akan menandatangani transaksi dengan tanda tangan kriptografi.
Registri kepemilikan akan dikelola oleh "klub properti" Bit Gold. Klub properti ini terdiri dari "anggota klub" (server) yang akan melacak kunci publik mana yang memiliki hash mana. Solusi ini agak mirip dengan solusi basis data replikasi yang diusulkan Wei Dai untuk b-money; baik Szabo maupun Dai tidak hanya aktif di milis Cypherpunks, tetapi juga di milis tertutup yang membahas topik-topik ini.
Namun, alih-alih sistem proof-of-stake milik Dai untuk menjaga agar sistem tetap mutakhir, Szabo mengusulkan "Sistem Kuorum Bizantium." Mirip dengan sistem yang sangat penting bagi keamanan seperti komputer pesawat terbang, jika hanya satu (atau sebagian kecil) dari komputer ini yang tidak berfungsi, sistem secara keseluruhan akan tetap beroperasi dengan baik. Sistem akan bermasalah hanya jika sebagian besar komputer gagal pada saat yang sama. Yang penting, tidak satu pun dari pemeriksaan ini memerlukan pengadilan, hakim, atau polisi, yang didukung oleh monopoli negara atas kekerasan: Semuanya akan bersifat sukarela.
Meskipun sistem ini sendiri tidak sepenuhnya sangat ketat — misalnya [Serangan Sybil](https://en.wikipedia.org/wiki/Sybil_attack) (_"sock puppet problem"_) — Szabo yakin sistem ini bisa berjalan sendiri. Bahkan dalam skenario di mana mayoritas anggota klub akan mencoba berbuat curang, minoritas yang jujur bisa bercabang ke dalam daftar kepemilikan yang bersaing. Pengguna kemudian dapat memilih daftar kepemilikan mana yang akan digunakan, yang menurut Szabo mungkin adalah yang jujur.
"Jika aturan dilanggar oleh pemilih yang menang, maka pecundang yang benar dapat keluar dari grup dan membentuk grup baru, mewarisi gelar lama," jelasnya. "Pengguna gelar (partai yang mengandalkan) yang ingin mempertahankan gelar yang benar dapat memverifikasi sendiri dengan aman kelompok sempalan mana yang telah mengikuti aturan dengan benar dan beralih ke grup yang benar."
(Sebagai contoh modern, ini mungkin dapat dibandingkan dengan Ethereum Classic, yang [memelihara](https://bitcoinmagazine.com/articles/rejecting-today-s-hard-fork-the-ethereum-classic-project-continues-on-the-original-chain-here-s-why-1469038808) versi buku besar Ethereum asli yang tidak membatalkan kontrak pintar The DAO.)
Inflasi
-------
Masalah berikutnya yang harus dipecahkan Szabo adalah inflasi. Seiring dengan semakin baiknya komputer dari waktu ke waktu, akan semakin mudah untuk menghasilkan hash yang valid. Ini berarti bahwa hash itu sendiri tidak dapat berfungsi sebagai uang dengan baik: hash akan semakin langka setiap tahunnya, sampai pada titik di mana kelimpahan akan melemahkan semua nilai.
Szabo menemukan solusinya. Setelah hash yang valid ditemukan, hash tersebut harus diberi cap waktu, idealnya dengan server cap waktu yang berbeda untuk meminimalkan kepercayaan pada hash tertentu. Cap waktu ini akan memberikan gambaran tentang seberapa sulitnya menghasilkan hash: hash yang lama akan lebih sulit diproduksi daripada hash yang baru. Pasar kemudian akan menentukan berapa nilai hash tertentu relatif terhadap hash lainnya, mungkin menyesuaikan nilainya dengan tanggal ditemukannya. "Hash 2018" yang valid seharusnya bernilai jauh lebih rendah daripada "Hash 2008" yang valid.
Namun solusi ini, tentu saja, menimbulkan masalah baru, Szabo tahu : "bagian-bagian (solusi teka-teki) dari satu periode (mulai dari detik hingga minggu, katakanlah seminggu) ke periode berikutnya tidak dapat dipertukarkan." Kepertukaran — gagasan bahwa setiap unit mata uang sama dengan unit lainnya — sangat penting bagi uang. Seorang pemilik toko ingin menerima pembayaran tanpa harus khawatir tentang tanggal uang tersebut dibuat.
Szabo juga menemukan solusi untuk masalah ini. Ia membayangkan semacam solusi "lapisan kedua" di atas lapisan dasar Bit Gold. Lapisan ini akan terdiri dari sejenis bank, meskipun bank yang dapat diaudit secara aman, karena registri Bit Gold bersifat publik. Bank-bank ini akan mengumpulkan hash yang berbeda dari periode waktu yang berbeda dan, berdasarkan nilai hash ini, menggabungkannya ke dalam paket-paket dengan nilai standar gabungan. "Paket 2018" akan mencakup lebih banyak hash daripada "paket 2008," tetapi kedua paket akan bernilai sama.
Paket-paket ini kemudian harus dipotong-potong menjadi sejumlah unit tertentu. Akhirnya, unit-unit ini dapat diterbitkan oleh "bank" sebagai eCash Chaumian yang bersifat pribadi dan anonim.
“\[P\]ansa pesaing menerbitkan uang kertas digital yang dapat ditukarkan dengan bit solusi yang nilai pasarnya sama dengan nilai nominal uang kertas (yakni mereka menciptakan kumpulan nilai standar),” jelas Szabo .
Dengan demikian, Bit Gold dirancang sebagai lapisan dasar seperti standar emas untuk sistem perbankan bebas di era digital.
Bitcoin
-------
Pada tahun 2000-an, Szabo melanjutkan pendidikannya dengan meraih gelar sarjana hukum untuk memahami hukum dan realitas kontrak yang ingin ia gantikan atau tiru secara daring dengan lebih baik. Ia juga mulai mengumpulkan dan menerbitkan ide-idenya di sebuah blog yang sangat dihormati, “[Unenumerated](https://unenumerated.blogspot.com/),” yang membahas berbagai topik mulai dari ilmu komputer hingga hukum dan politik, tetapi juga sejarah dan biologi. “Daftar topik untuk blog ini \[…\] sangat luas dan beragam sehingga tidak dapat disebutkan satu per satu,” Szabo [menjelaskan](https://unenumerated.blogspot.com/2005/10/unenumerated.html) judulnya.
Pada tahun 2008 — 10 tahun setelah pertama kali mengusulkannya secara pribadi — Szabo mengangkat Bit Gold di blognya sekali lagi, hanya saja kali ini ia ingin mewujudkan implementasi pertama usulannya.
“Bit Gold akan sangat diuntungkan dari sebuah demonstrasi, pasar eksperimental (dengan misalnya pihak ketiga yang tepercaya menggantikan keamanan kompleks yang dibutuhkan untuk sistem nyata). Adakah yang mau membantu saya membuat kodenya?” tanyanya [di bagian komentar](https://unenumerated.blogspot.com/2008/04/bit-gold-markets.html?showComment=1207799580000#c3741843833998921269) blognya.
Jika ada yang menanggapi, tanggapan itu tidak disampaikan di depan umum. Bit Gold, dalam bentuk yang diusulkan Szabo, tidak pernah dilaksanakan.
Namun, Bit Gold tetap menjadi inspirasi utama bagi Satoshi Nakamoto, yang menerbitkan white paper Bitcoin di akhir tahun yang sama.
[“Bitcoin merupakan implementasi dari proposal b-money Wei Dai \[...\] di Cypherpunks \[...\] pada tahun 1998 dan proposal Bitgold milik Nick Szabo,”](https://bitcointalk.org/index.php?topic=342.msg4508#msg4508) tulis penemu Bitcoin dengan nama samaran di forum Bitcointalk pada tahun 2010.
Memang, tidak sulit untuk melihat Bit Gold sebagai rancangan awal Bitcoin. Selain dari basis data bersama catatan kepemilikan berdasarkan kriptografi kunci publik, rangkaian hash bukti kerja memiliki kemiripan yang aneh dengan [blockchain](/2024/07/apa-itu-blockchain.html) Bitcoin . Dan, tentu saja, nama Bit Gold dan Bitcoin juga tidak terlalu jauh.
Namun, tidak seperti sistem seperti Hashcash dan b-money, Bit Gold jelas tidak ada dalam white paper Bitcoin. Beberapa orang bahkan menganggap ketidakhadiran ini begitu penting sehingga mereka menganggapnya sebagai salah satu dari beberapa petunjuk bahwa Szabo pastilah orang di balik julukan Satoshi Nakamoto: Siapa lagi yang akan mencoba menyembunyikan asal-usul Bitcoin seperti ini?
Meski demikian, meski mirip dengan Bit Gold dalam beberapa hal, Bitcoin memang menyertakan beberapa perbaikan atas desain Szabo. Secara khusus, di mana Bit Gold masih bergantung pada pihak tepercaya sampai batas tertentu — server dan layanan stempel waktu harus dipercaya sampai batas tertentu untuk tidak berkolusi — Bitcoin adalah sistem pertama yang memecahkan masalah ini sepenuhnya. Bitcoin memecahkannya dengan sangat elegan, dengan memiliki sistem bukti kerja yang diperlukan yang berfungsi sebagai sistem penghargaan dan mekanisme konsensus dalam satu sistem: Rantai hash dengan bukti kerja terbanyak dianggap sebagai versi sejarah yang valid.
“Nakamoto memperbaiki kekurangan keamanan signifikan yang ada pada desain saya,” Szabo [mengakui pada tahun 2011](https://unenumerated.blogspot.com/2011/05/bitcoin-what-took-ye-so-long.html), “yakni dengan mensyaratkan bukti kerja untuk menjadi simpul dalam sistem peer-to-peer yang tangguh terhadap Byzantine untuk mengurangi ancaman pihak yang tidak dapat dipercaya yang mengendalikan mayoritas simpul dan dengan demikian merusak sejumlah fitur keamanan penting.”
Lebih jauh, Bitcoin memiliki model moneter yang sangat berbeda dari yang diusulkan Szabo, dengan jadwal inflasi tetap yang sama sekali tidak terpengaruh oleh peningkatan daya hash. Seiring meningkatnya daya komputasi pada jaringan Bitcoin, itu artinya semakin sulit menemukan koin baru.
“Alih-alih pasar otomatis saya memperhitungkan fakta bahwa tingkat kesulitan teka-teki sering kali dapat berubah secara radikal berdasarkan peningkatan perangkat keras dan terobosan kriptografi (yaitu menemukan algoritma yang dapat memecahkan bukti kerja lebih cepat), dan ketidakpastian permintaan, Nakamoto merancang algoritma yang disetujui Bizantium yang menyesuaikan tingkat kesulitan teka-teki,” jelas Szabo.
“Saya tidak dapat memutuskan apakah aspek Bitcoin ini lebih banyak fitur atau lebih banyak bug,” tambahnya, “tetapi ini membuatnya lebih sederhana.”
Sumber artikel: [bitcoinmagazine.com](https://bitcoinmagazine.com/culture/genesis-files-bit-gold-szabo-was-inches-away-inventing-bitcoin)
Diterjemahkan oleh: [Abeng](https://abeng.xyz)
-

@ 5d4b6c8d:8a1c1ee3
2024-11-23 21:24:35
Hi Everyone,
Sorry I'm late putting this up this week. I would like to blame @grayruby, but he actually sent me the itinerary early this week.
Here's an outline of topics we're planning on hitting this week:
# Territory things:
- NFL Survivor Pool- tnstacker and wumbo still hanging around (tnstacker with a bit of a surprise pick this week)
- USA vs World NFL pick 'em- what is going on???
- Cricket Pools
- NBA Cup pool
# Blokd shots (NHL)
- why can't Canada win the cup?
# MLB
- Off-season news
- MLB testing ball/strike challenge system
# NFL
- Fantasy football recap
- Current playoff picture
- Who is frontrunner for MVP?
# NBA cup
- Survivor Pool Strategy
- Are the Bucks getting back on track or is Giannis just really good
- Who wins a 7 game series between Cavs/Celtics right now
- Embiid and the 76ers failed Process
# Betting
- Predyx: in-game betting experience and trying to inflate the 49ers odds
originally posted at https://stacker.news/items/780312
-

@ 6f170f27:711e26dd
2024-11-23 16:38:10
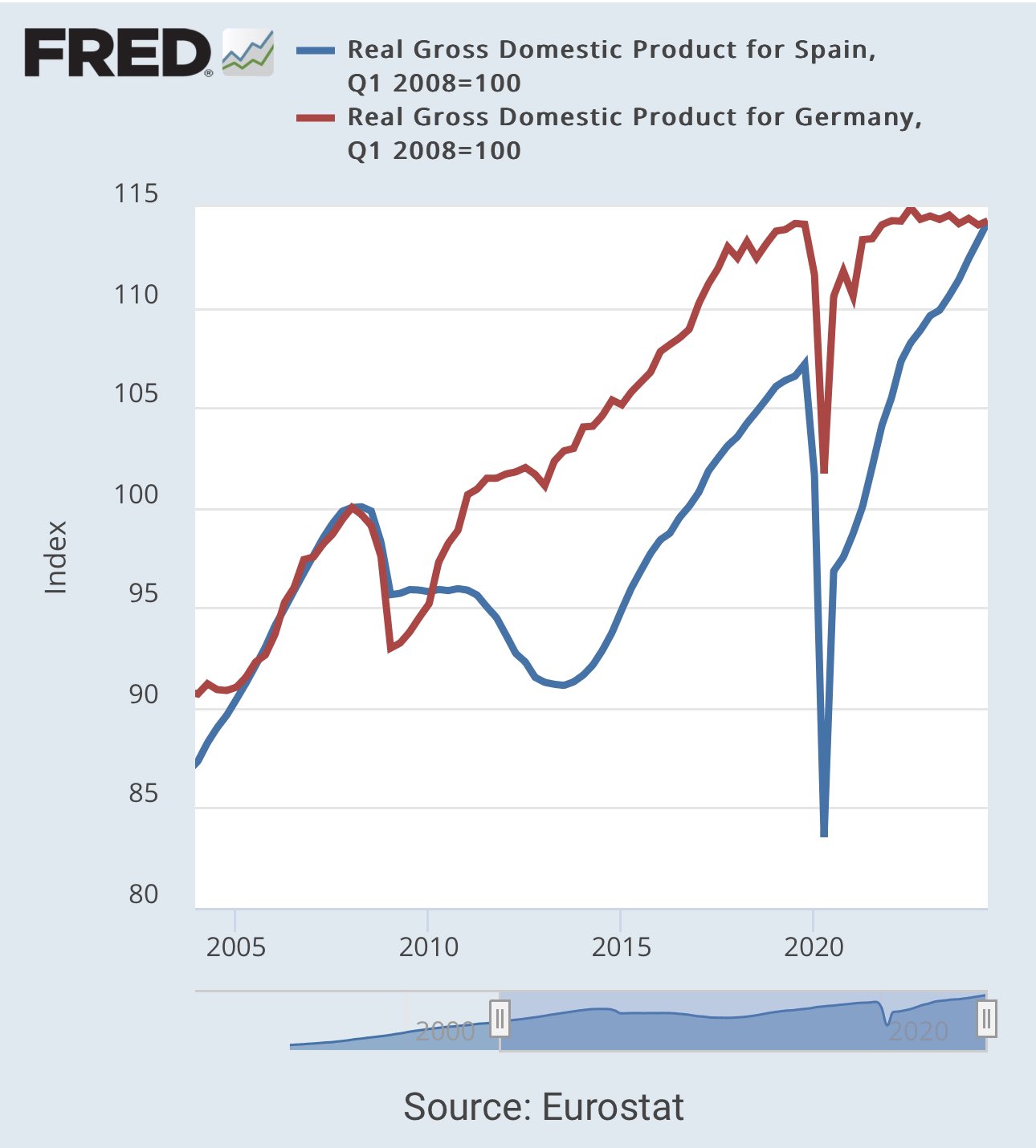
> Here’s a crazy fact for you: Spain and Germany have now seen basically the exact same amount of economic growth post-2008. Would have been unthinkable to people during most of the 2010s. Spain has seen ~10 percentage points more cumulative growth since 2017. ([source](https://x.com/JosephPolitano/status/1860253636762169567))
originally posted at https://stacker.news/items/780007
-

@ a849beb6:b327e6d2
2024-11-23 15:03:47
<img src="https://blossom.primal.net/e306357a7e53c4e40458cf6fa5625917dc8deaa4d1012823caa5a0eefb39e53c.jpg">
\
\
It was another historic week for both bitcoin and the Ten31 portfolio, as the world’s oldest, largest, most battle-tested cryptocurrency climbed to new all-time highs each day to close out the week just shy of the $100,000 mark. Along the way, bitcoin continued to accumulate institutional and regulatory wins, including the much-anticipated approval and launch of spot bitcoin ETF options and the appointment of several additional pro-bitcoin Presidential cabinet officials. The timing for this momentum was poetic, as this week marked the second anniversary of the pico-bottom of the 2022 bear market, a level that bitcoin has now hurdled to the tune of more than 6x despite the litany of bitcoin obituaries published at the time. The entirety of 2024 and especially the past month have further cemented our view that bitcoin is rapidly gaining a sense of legitimacy among institutions, fiduciaries, and governments, and we remain optimistic that this trend is set to accelerate even more into 2025.
Several Ten31 portfolio companies made exciting announcements this week that should serve to further entrench bitcoin’s institutional adoption. AnchorWatch, a first of its kind bitcoin insurance provider offering 1:1 coverage with its innovative use of bitcoin’s native properties, announced it has been designated a Lloyd’s of London Coverholder, giving the company unique, blue-chip status as it begins to write bitcoin insurance policies of up to $100 million per policy starting next month. Meanwhile, Battery Finance Founder and CEO Andrew Hohns appeared on CNBC to delve into the launch of Battery’s pioneering private credit strategy which fuses bitcoin and conventional tangible assets in a dual-collateralized structure that offers a compelling risk/return profile to both lenders and borrowers. Both companies are clearing a path for substantially greater bitcoin adoption in massive, untapped pools of capital, and Ten31 is proud to have served as lead investor for AnchorWatch’s Seed round and as exclusive capital partner for Battery.
As the world’s largest investor focused entirely on bitcoin, Ten31 has deployed nearly $150 million across two funds into more than 30 of the most promising and innovative companies in the ecosystem like AnchorWatch and Battery, and we expect 2025 to be the best year yet for both bitcoin and our portfolio. Ten31 will hold a first close for its third fund at the end of this year, and investors in that close will benefit from attractive incentives and a strong initial portfolio. Visit ten31.vc/funds to learn more and get in touch to discuss participating.\
\
**Portfolio Company Spotlight**
[Primal](http://primal.net/) is a first of its kind application for the Nostr protocol that combines a client, caching service, analytics tools, and more to address several unmet needs in the nascent Nostr ecosystem. Through the combination of its sleek client application and its caching service (built on a completely open source stack), Primal seeks to offer an end-user experience as smooth and easy as that of legacy social media platforms like Twitter and eventually many other applications, unlocking the vast potential of Nostr for the next billion people. Primal also offers an integrated wallet (powered by [Strike BLACK](https://x.com/Strike/status/1755335823023558819)) that substantially reduces onboarding and UX frictions for both Nostr and the lightning network while highlighting bitcoin’s unique power as internet-native, open-source money.
### **Selected Portfolio News**
AnchorWatch announced it has achieved Llody’s Coverholder status, allowing the company to provide unique 1:1 bitcoin insurance offerings starting in [December](https://x.com/AnchorWatch/status/1858622945763131577).\
\
Battery Finance Founder and CEO Andrew Hohns appeared on CNBC to delve into the company’s unique bitcoin-backed [private credit strategy](https://www.youtube.com/watch?v=26bOawTzT5U).
Primal launched version 2.0, a landmark update that adds a feed marketplace, robust advanced search capabilities, premium-tier offerings, and many [more new features](https://primal.net/e/note1kaeajwh275kdwd6s0c2ksvj9f83t0k7usf9qj8fha2ac7m456juqpac43m).
Debifi launched its new iOS app for Apple users seeking non-custodial [bitcoin-collateralized loans](https://x.com/debificom/status/1858897785044500642).
### **Media**
Strike Founder and CEO Jack Mallers [joined Bloomberg TV](https://www.youtube.com/watch?v=i4z-2v_0H1k) to discuss the strong volumes the company has seen over the past year and the potential for a US bitcoin strategic reserve.
Primal Founder and CEO Miljan Braticevic [joined](https://www.youtube.com/watch?v=kqR_IQfKic8) The Bitcoin Podcast to discuss the rollout of Primal 2.0 and the future of Nostr.
Ten31 Managing Partner Marty Bent [appeared on](https://www.youtube.com/watch?v=_WwZDEtVxOE&t=1556s) BlazeTV to discuss recent changes in the regulatory environment for bitcoin.
Zaprite published a customer [testimonial video](https://x.com/ZapriteApp/status/1859357150809587928) highlighting the popularity of its offerings across the bitcoin ecosystem.
### **Market Updates**
Continuing its recent momentum, bitcoin reached another new all-time high this week, clocking in just below $100,000 on Friday. Bitcoin has now reached a market cap of [nearly $2 trillion](https://companiesmarketcap.com/assets-by-market-cap/), putting it within 3% of the market caps of Amazon and Google.
After receiving SEC and CFTC approval over the past month, long-awaited options on spot bitcoin ETFs were fully [approved](https://finance.yahoo.com/news/bitcoin-etf-options-set-hit-082230483.html) and launched this week. These options should help further expand bitcoin’s institutional [liquidity profile](https://x.com/kellyjgreer/status/1824168136637288912), with potentially significant [implications](https://x.com/dgt10011/status/1837278352823972147) for price action over time.
The new derivatives showed strong performance out of the gate, with volumes on options for BlackRock’s IBIT reaching [nearly $2 billion](https://www.coindesk.com/markets/2024/11/20/bitcoin-etf-options-introduction-marks-milestone-despite-position-limits/) on just the first day of trading despite [surprisingly tight](https://x.com/dgt10011/status/1858729192105414837) position limits for the vehicles.
Meanwhile, the underlying spot bitcoin ETF complex had yet another banner week, pulling in [$3.4 billion](https://farside.co.uk/btc/) in net inflows.
New reports [suggested](https://archive.is/LMr4o) President-elect Donald Trump’s social media company is in advanced talks to acquire crypto trading platform Bakkt, potentially the latest indication of the incoming administration’s stance toward the broader “crypto” ecosystem.
On the macro front, US housing starts [declined M/M again](https://finance.yahoo.com/news/us-single-family-housing-starts-134759234.html) in October on persistently high mortgage rates and weather impacts. The metric remains well below pre-COVID levels.
Pockets of the US commercial real estate market remain challenged, as the CEO of large Florida developer Related indicated that [developers need further rate cuts](https://www.bloomberg.com/news/articles/2024-11-19/miami-developer-says-real-estate-market-needs-rate-cuts-badly) “badly” to maintain project viability.
US Manufacturing PMI [increased slightly](https://www.fxstreet.com/news/sp-global-pmis-set-to-signal-us-economy-continued-to-expand-in-november-202411220900) M/M, but has now been in contraction territory (<50) for well over two years.
The latest iteration of the University of Michigan’s popular consumer sentiment survey [ticked up](https://archive.is/fY5j6) following this month’s election results, though so did five-year inflation expectations, which now sit comfortably north of 3%.
### **Regulatory Update**
After weeks of speculation, the incoming Trump administration appointed hedge fund manager [Scott Bessent](https://www.cnbc.com/amp/2024/11/22/donald-trump-chooses-hedge-fund-executive-scott-bessent-for-treasury-secretary.html) to head up the US Treasury. Like many of Trump’s cabinet selections so far, Bessent has been a [public advocate](https://x.com/EleanorTerrett/status/1856204133901963512) for bitcoin.
Trump also [appointed](https://www.axios.com/2024/11/19/trump-commerce-secretary-howard-lutnick) Cantor Fitzgerald CEO Howard Lutnick – another outspoken [bitcoin bull](https://www.coindesk.com/policy/2024/09/04/tradfi-companies-want-to-transact-in-bitcoin-says-cantor-fitzgerald-ceo/) – as Secretary of the Commerce Department.
Meanwhile, the Trump team is reportedly considering creating a new [“crypto czar”](https://archive.is/jPQHF) role to sit within the administration. While it’s unclear at this point what that role would entail, one report indicated that the administration’s broader “crypto council” is expected to move forward with plans for a [strategic bitcoin reserve](https://archive.is/ZtiOk).
Various government lawyers suggested this week that the Trump administration is likely to be [less aggressive](https://archive.is/Uggnn) in seeking adversarial enforcement actions against bitcoin and “crypto” in general, as regulatory bodies appear poised to shift resources and focus elsewhere.
Other updates from the regulatory apparatus were also directionally positive for bitcoin, most notably FDIC Chairman Martin Gruenberg’s confirmation that he [plans to resign](https://www.politico.com/news/2024/11/19/fdics-gruenberg-says-he-will-resign-jan-19-00190373) from his post at the end of President Biden’s term.
Many critics have alleged Gruenberg was an architect of [“Operation Chokepoint 2.0,”](https://x.com/GOPMajorityWhip/status/1858927571666096628) which has created banking headwinds for bitcoin companies over the past several years, so a change of leadership at the department is likely yet another positive for the space.
SEC Chairman Gary Gensler also officially announced he plans to resign at the start of the new administration. Gensler has been the target of much ire from the broader “crypto” space, though we expect many projects outside bitcoin may continue to struggle with questions around the [Howey Test](https://www.investopedia.com/terms/h/howey-test.asp).
Overseas, a Chinese court ruled that it is [not illegal](https://www.benzinga.com/24/11/42103633/chinese-court-affirms-cryptocurrency-ownership-as-legal-as-bitcoin-breaks-97k) for individuals to hold cryptocurrency, even though the country is still ostensibly [enforcing a ban](https://www.bbc.com/news/technology-58678907) on crypto transactions.
### **Noteworthy**
The incoming CEO of Charles Schwab – which administers over $9 trillion in client assets – [suggested](https://x.com/matthew_sigel/status/1859700668887597331) the platform is preparing to “get into” spot bitcoin offerings and that he “feels silly” for having waited this long. As this attitude becomes more common among traditional finance players, we continue to believe that the number of acquirers coming to market for bitcoin infrastructure capabilities will far outstrip the number of available high quality assets.
BlackRock’s 2025 Thematic Outlook notes a [“renewed sense of optimism”](https://www.ishares.com/us/insights/2025-thematic-outlook#rate-cuts) on bitcoin among the asset manager’s client base due to macro tailwinds and the improving regulatory environment. Elsewhere, BlackRock’s head of digital assets [indicated](https://www.youtube.com/watch?v=TE7cAw7oIeA) the firm does not view bitcoin as a “risk-on” asset.
MicroStrategy, which was a sub-$1 billion market cap company less than five years ago, briefly breached a [$100 billion equity value](https://finance.yahoo.com/news/microstrategy-breaks-top-100-u-191842879.html) this week as it continues to aggressively acquire bitcoin. The company now holds nearly 350,000 bitcoin on its balance sheet.
Notably, Allianz SE, Germany’s largest insurer, [spoke for 25%](https://bitbo.io/news/allianz-buys-microstrategy-notes/) of MicroStrategy’s latest $3 billion convertible note offering this week, suggesting [growing appetite](https://x.com/Rob1Ham/status/1860053859181199649) for bitcoin proxy exposure among more restricted pools of capital.
The [ongoing meltdown](https://www.cnbc.com/2024/11/22/synapse-bankruptcy-thousands-of-americans-see-their-savings-vanish.html) of fintech middleware provider Synapse has left tens of thousands of customers with nearly 100% deposit haircuts as hundreds of millions in funds remain missing, the latest unfortunate case study in the fragility of much of the US’s legacy banking stack.
### **Travel**
- [BitcoinMENA](https://bitcoin2024.b.tc/mena), Dec 9-10
- [Nashville BitDevs](https://www.meetup.com/bitcoinpark/events/302533726/?eventOrigin=group_upcoming_events), Dec 10
- [Austin BitDevs](https://www.meetup.com/austin-bitcoin-developers/events/303476169/?eventOrigin=group_upcoming_events), Dec 19
- [Nashville Energy and Mining Summit](https://www.meetup.com/bitcoinpark/events/304092624/?eventOrigin=group_events_list), Jan 30
-

@ 5d4b6c8d:8a1c1ee3
2024-11-23 14:17:35
# Recap
We almost made it through unscathed, but the Nets couldn't pull off the win over superstar-less 76ers. The Ewing Theory is very much in play with Joel Embiid.
The Warriors game came right down to the wire and was unreasonably close throughout.
The Rockets demonstrated why most of us picked them.
Easily the pick of the night was @supercyclone's Bulls. I thought he was a dead man walking with that pick, but that's why we play the games. Great pick!
The Wizards and Nuggets did their best to keep us from watching, with their horrific court designs.
Are the Bucks back on track or are the Pacers just that bad?
I'm glad I didn't take the Kings.
# Prize: 50 kilosats!
@Carresan, you can zap your 5k to this post to buy back in.
originally posted at https://stacker.news/items/779825
-

@ 8dac122d:0fc98d37
2024-11-23 12:11:13
Below are discount codes and holiday deals on various cold storage devices. Now is the perfect time to take custody of your own coins. If you know of any other deals not mentioned here, please leave them in the comments.
**BITBOX**
Get 21% off on the “Black Friday Bundle” (includes BitBox02 hardware wallet; 1 Steelwallet w/automatic puncher; 3 Backup cards).
https://shop.bitbox.swiss/de/products/bitbox-all-inclusive-bundle-29/?ref=gxouqmnm
**COLDCARD**
Get 20% off most products
https://store.coinkite.com/store
**JADE**
Buy One Jade - Get Free Shipping
https://store.blockstream.com/products/blockstream-jade-hardware-wallet
**KEYSTONE**
Get 20% off Keystone Bundle Pack + Extra $10 OFF + Free Shipping. Now thru December 5th
https://keyst.one/shop/products/keystone-bundle-pack
**LEDGER**
Get $70 of Bitcoin with new Ledger. Now thru December 5th
https://shop.ledger.com/pages/black-friday
**PORTAL**
Hardware Wallet - Batch #2
https://store.twenty-two.xyz/products/portal-hardware-wallet
**SEEDSIGNER**
Kits & Assembled Signers
– EU/UK: [GoBrrr](https://www.gobrrr.me), [VULCAN](https://vulcan21.com), [DIYNodes](https://diynodes.com), [BitcoinBrabant](https://bitcoinbrabant.com), [Bitsaga](https://bitsaga.be)
– USA/Canada: [BTC Hardware Solutions](https://btc-hardware-solutions.square.site) (by project leader/creator)
– Brazil: [StackBit](https://stackbit.me/categoria-produto/cases)
– Australia: [HansMade](https://shopbitcoin.com.au/collections/hansmade)
**TREZOR**
Get up to 70% off all Trezor products.
https://trezor.io/store
-50% Trezor Keep Metal 12-word
-40% Trezor Model T
-30% Trezor Model One
-30% Trezor Model Safe 3
originally posted at https://stacker.news/items/779699
-

@ 6f170f27:711e26dd
2024-11-23 10:47:24

source: https://www.axios.com/2024/11/22/boomers-gen-z-millennials-financial-success
I wanted to share this because of two observations:
1. **Why the Zoomer expectation is so surprisingly high**
2. **Why the Millennials are so surprisingly low**
Alright, about that first one:
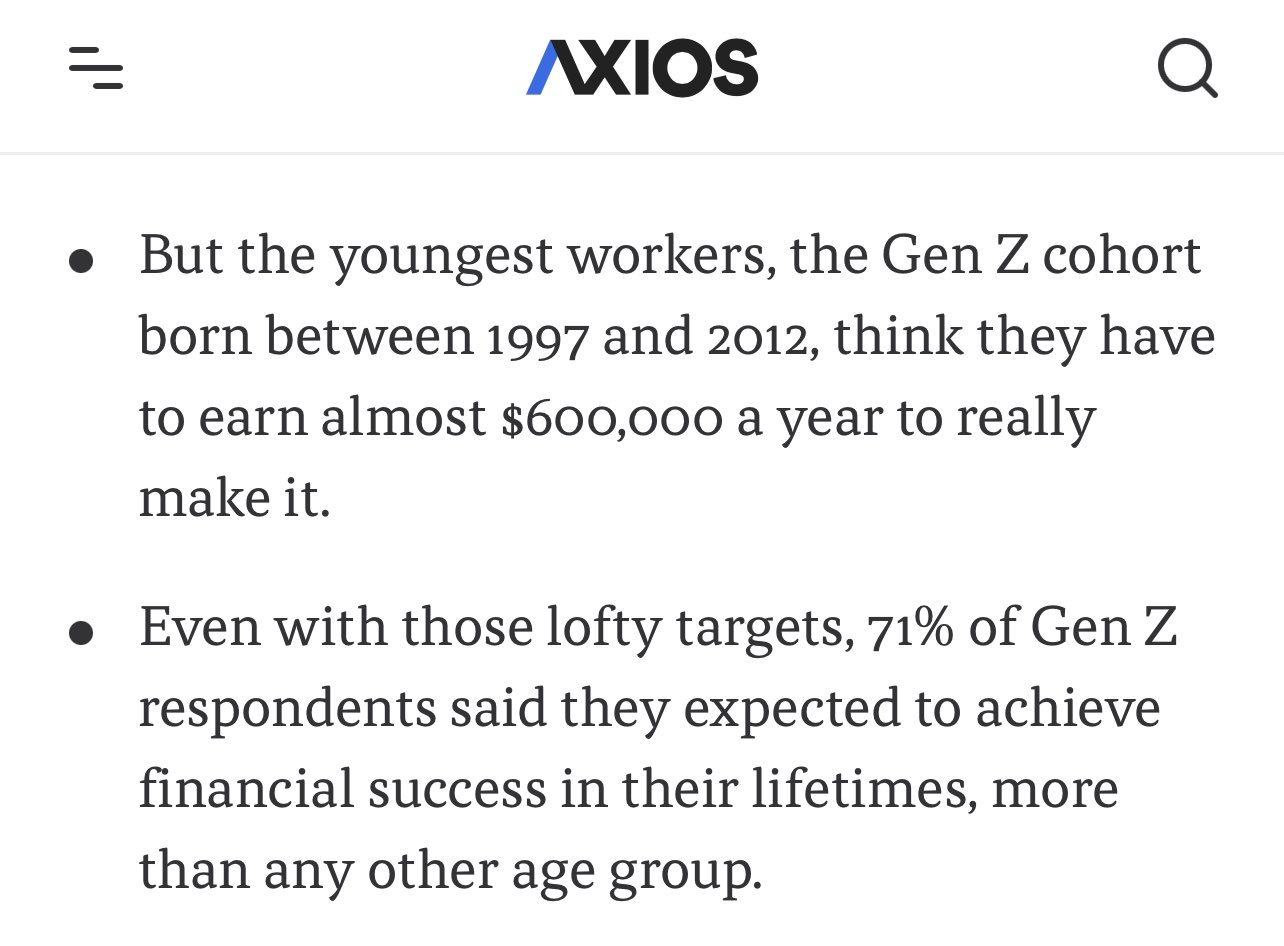
The gut reaction of most people probably is that Zoomers are young, their impressionable years have been marked by inflation. They have more years into the future to think about and their character will be forever stained by this just like great depression babies had a different scarcity mindset even decades after 1929. And thus Zommers extrapolate from there for the next 40 years. 600k. Big number.
I think there must be something true to this. But don't forget that the youngest Zoomers are 12yo. Ask any 12yo about financial success and they will answer ONE MILLION. They would answer every question with one million. That skews the statistic.
Second thought:
Why are Millennials so low? Surely there is a flaw in the graph here if its even lower than genX. Shouldn't it look more like this naturally:
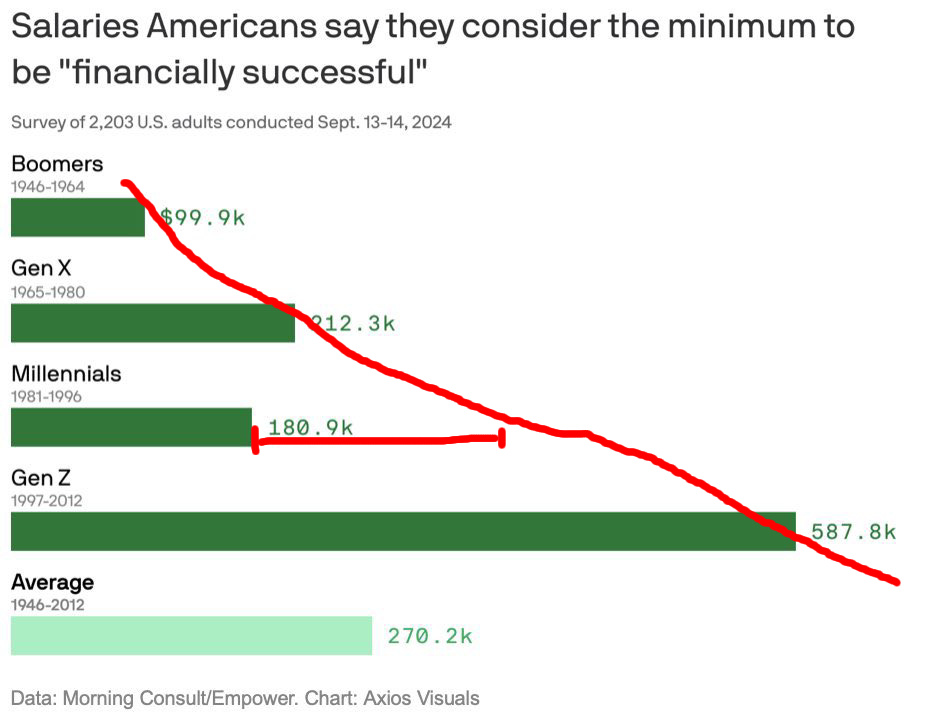
Have a few birthyears been uniquely tainted by 2008? 2008 was bad but there were recessions every few years before that. What's different here?
And even more interesting for me: Will we see an effect of this on genAlpha, the children of Millennials? Maybe the future will hold an alternating pattern of scarcity-abundance generations. Reinforced by a self-fulfilling prophecy.
originally posted at https://stacker.news/items/779653
-

@ 5af07946:98fca8c4
2024-11-23 01:06:57
US natives think $USD is a currency - for the rest of the world it is an asset . Just like #bitcoin.
The way Microstrategy accumulates bitcoin , all central banks collect US debt - a fancier word for US Dollar. As of April 2024, the five countries that hold the most US debt are:
Japan: $1.1 trillion
China: $749.0 billion
United Kingdom: $690.2 billion
Luxembourg: $373.5 billion
Canada: $328.7 billion
Compare this global stake to 15 odd billions that #Microstrategy has in bitcoin. The way Microstrategy wants to protect bitcoin network , central banks want to protect #USD. Which means they all resist any change in capital structure. Not even bitcoin.
Thus the peaceful path for bitcoin is to become the reserve strategic asset for United States and thus for rest of the world. The other path is literally fighting governments worldwide ..
There is however one more opportunity - that is if people all around world adopt #SATs - which is why success of #nostr is so critical ! And other lightning apps - #LNCalendar , #StackerNews , #Fountain
In reality , both these things can happen simultaneously - that would be real freedom - Top down and Bottom up.
originally posted at https://stacker.news/items/779432
-

@ 5d4b6c8d:8a1c1ee3
2024-11-23 00:13:13
More exciting basketball and this time we can live bet one of the matches.
[Predyx](https://beta.predyx.com/market/nets-vs-76ers-nets-win) has a prediction market for the Sixers/Nets game. One of you maniacs picked the Nets. Good luck!
I would have been excited about that Mavs/Nuggets game later, but I don't know if Jokic is even playing and Luka definitely isn't. That probably leaves Bucks/Pacers as the game of the night.
originally posted at https://stacker.news/items/779386
-

@ 6f170f27:711e26dd
2024-11-22 18:44:48
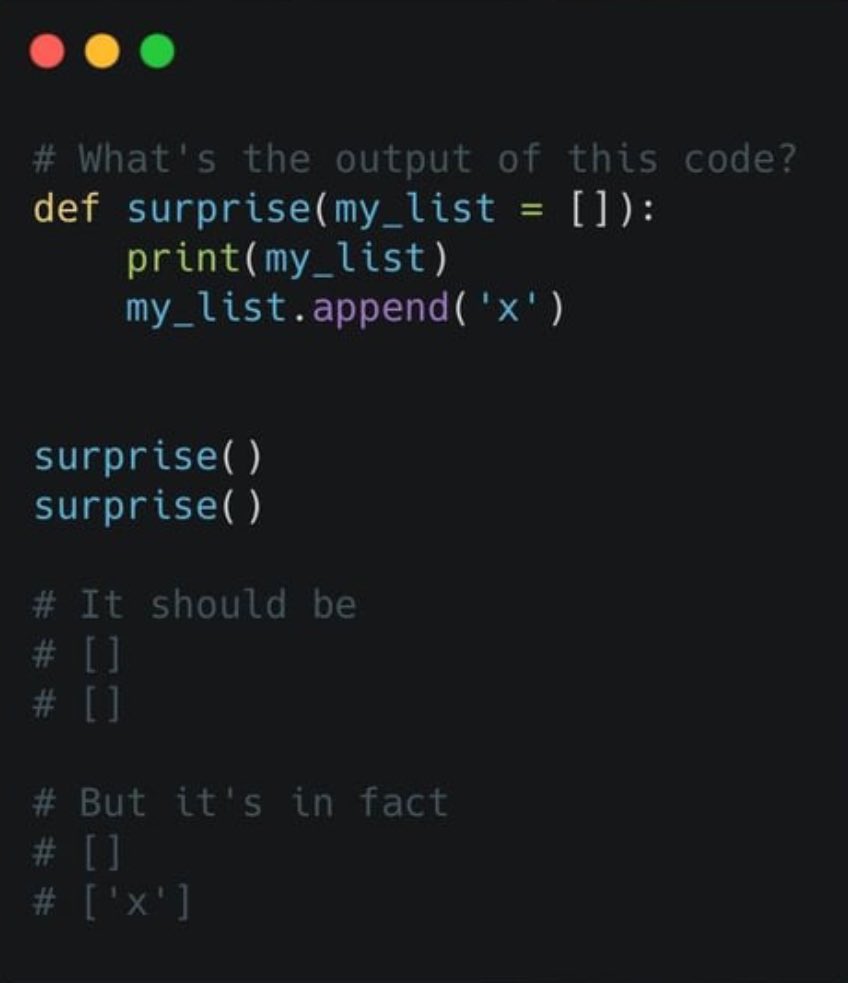
Image [source](https://x.com/hot_girl_spring/status/1859642773059797181)
I like Python a lot. But this fcking design decision is so annoying. I cannot tell you how many times I have tumbled over this now by accident.
originally posted at https://stacker.news/items/778991
-

@ 5d4b6c8d:8a1c1ee3
2024-11-22 13:57:13
Here are today's picks using my proprietary betting strategy at [Freebitcoin](https://freebitco.in/?r=51325722). For details about what Risk Balanced Odds Arbitrage is and why it works see https://stacker.news/items/342765/r/Undisciplined.
There are more matches up, including some Budesliga, but they're too far out to find odds on.
For a hypothetical 1k wager on each match, distribute your sats as follows:
| Outcome 1 | Outcome 2 | Outcome 3 | Bet 1 | Bet 2 | Bet 3 |
|--------------|-------------|--------------|-------|-------|-------|
| Sevilla| Osasuna| Draw | 400| 300| 300|
| Real Sociedad| Real Betis| Draw | 510| 210| 280|
| Real Madrid| Getafe| Draw | 700| 120| 180|
RBOA is up almost 85k now. A ton of our past matches are being played tomorrow, so that will be a big test.
originally posted at https://stacker.news/items/778487
-

@ 0861144c:e68a1caf
2024-11-22 11:31:35
In the days surrounding the US election, here in Stacker but also in other places, there were lots of debates whether is OK to support a politician or not. That's because of the position took by the *crypto*-industry that have [a very active participation](https://x.com/jespow/status/1806569144294556146) in the republican campaign, not only with words but also money and [some proposals](https://x.com/BitcoinMagazine/status/1859618962092216660).
Those days prosecuted also with lots of comments of bitcoiners making arguments about how bad was the take made by the industry because, at the end of the day, Trump isn't a bitcoiner, it's a politician that takes maximum benefits from the *crypto* stuff. Now, having said these things, a couple of notes that I made while I was traveling.
### We were victims of the nirvana fallacy
This I heard in the Lex's interview [with Javier Milei](https://www.youtube.com/watch?v=8NLzc9kobDk), where he ask about his insertion into politics. Milei tells that lots of people, including anarcho-capitalists and liberals, criticized him a lot because he was getting with people not so trustworthy and was building a minarchist-style political campaign. The ones making critics argue that nothing is going to change and be truly free, so why bother to get into it?
Enter the nirvana fallacy, [which states](https://en.wikipedia.org/wiki/Nirvana_fallacy) *"...comparing actual things with unrealistic, idealized alternatives. It can also refer to the tendency to assume there is a perfect solution to a particular problem."* So, what this has to do with us, as bitcoiners?
Well, some notes about where we are right now:
* @suraz puts you context in inflation, [it's bad](https://stacker.news/items/767210).
* @TomK still talking [about economics](https://stacker.news/items/751811) downsides [and new plans](https://stacker.news/items/731370)
So, what can you do?
Well, here is where I stated in the past that [bitcoin is a political tool](https://stacker.news/items/627008). Those who doesn't want to participate in politics, I'm sorry but in the moment you chose to opt-out the fiat system, you made a political act, stating your discomfort to participate in a system which promotes war and terrorism.
And some of them, tired of legislators who tirelessly chase the industry through the well-documented [Operation ChokePoint 2.0](https://x.com/RepFrenchHill/status/1859270268805657058), took a side.
Is the best solution to take a politician as ally? I think not, we don't like political intrusion into our lives but...hey, the [world which we live](https://stacker.news/items/557608) in, either you accept that they want to intrude and try to guide them or leave it to those anti-crypto fascists.
We don't take a clear position, it has consequences, so I think is stupid not to fight against it, even if that means to get into the mud, look the other way and pretend we won because we just use it is to fool ourselves. We won't have hyperbitcoinization[^1] in our generation, maybe my kids would and in order to happen, we need hands in every aspect. I myself doubt that all promises are going to make it.
[^1]: I think hyperbitcoinization as it is, it's just nonsense invented by some dudes
So, yeah, the nirvana fallacy is real, those who claim or criticize bitcoiners for participate in politics behind a screen....fuck you but have a nice day.
originally posted at https://stacker.news/items/778354
-

@ 524314bf:d1e30e55
2024-11-22 05:35:10
Hello Fellow Stackers,
As many of you know, Stacker News will be completely eliminating it's custodial lightning wallet soon. Prior to this change, Stacker News could serve as a 'poor man's' ln proxy as the sats would go to the Stacker News node, then Stacker News would create a new payment to your node (depending on your wallet settings). This was an awesome tool for us Plebs, but I totally understand that it was probably never the intent of Stacker News to serve this purpose ; more of an emergent behavior. So, I mourn it's loss but I am excited for the next phase of the Stacker News value for value experiment.
So, what does this mean for lightning node runners that want to receive sats sent to their Stacker News ln address or want to receive sats from Stacker News activity instead of cowboy credits ? Well, it means that now when you setup a receive option, the receiving node will be exposed when a zap is sent to your Stacker News address. This is a bit of a bummer in comparison to the old setup.
With that said, Stacker News is providing an immediate solution for those users that do not want their node ID exposed. Stacker News now has a setting where you can turn on a proxy for any incoming sats to your Stacker News ln address, albeit at a cost of 10% of the receiving amount. I assume this will work to basically give you the same experience as before, with the Stacker News lightning node acting as the proxy before sats are sent to your node.
That's awesome that the option is available, but the price is pretty steep (I'd imagine that the price is set steep because Stacker News does not want to act in this function for its users ; this is a classy move to provide it at all even though Stacker News is not probably intending to be a leading privacy service provider in the lightning space). So, what is a pleb to do ? Well, there is a solution out there available to all plebs that can give you the proxy receiving privacy you want without paying 10% of your received sats for that privacy: Enter Zeuspay (https://docs.zeusln.app/lightning-address/intro/)
So, Zeuspay is an ln address that any LND node runner can use by setting up their node in the Zeus app via the available options. I won't go into detail how to do this (i'm happy to answer questions in the comments, but not really the point of this post to give a step by step tutorial), but essentially once you have set up your connection in Zeus to your LND node, there is an option in the setting to create your own Zeuspay ln address. This ln address is a bit different than custodial options you have experienced:
1. When sats are sent to this ln address, they actually need to be redeemed by you within the Zeus app within 24 hours. If not redeemed within 24 hours, they are sent back to the sender's wallet. This is how zeus set up the service so that it works best with their embedded mobile node as such nodes will not be online all the time.
2. When you redeem sats, those sats are then actually going first to the Zeus lightning node, and then to your node. Thus, it is a proxy for receiving sats that will not reveal your node to the original sat sender.
So, if a Stacker News user sets up their wallet config in Stacker News to receive sats using the ln address to their Zeuspay ln address, they will now have the privacy benefit of before. AND, what's great is that Zeus just recently dropped the receiving fees to 0 for this service (see the recent nost post here: https://njump.me/nevent1qyt8wumn8ghj7etyv4hzumn0wd68ytnvv9hxgtcpz9mhxue69uhkummnw3ezumrpdejz7qgawaehxw309ahx7um5wghxy6t5vdhkjmn9wgh8xmmrd9skctcpz9mhxue69uhkummnw3ezuamfdejj7qgewaehxw309aex2mrp0yh8xmn0wf6zuum0vd5kzmp0qqsdmf5h6kpzt2pxh0cmgf43uksjhklx07s49n2ydeh82lnqw83p2pqsph5ec). So, as long as you make sure to log into your Zeus app and redeem sats once a day, you can use this option to receive sats to your Stacker News ln address with a privacy proxy that does not utilize Stacker News' proxy service. Now, one caveat I will say which I experienced while testing: if you do not have a channel open with Zeus, it did not work very well for me. However, once I got a channel open with Zeus, and got some inbound liquidity in that channel, it has worked perfectly. Hope that post serves you well fellow Stackers, happy zapping n stacking :)
#bitcoin
#lightning
originally posted at https://stacker.news/items/778172
-

@ 129f5189:3a441803
2024-11-22 03:42:42
Today we will understand how Argentina, when under the control of the Foro de São Paulo, was an important asset for the Chinese Communist Party in South America and how Javier Milei is charting paths to change this scenario.
The Chinese government has been making diplomatic overtures to areas near the polar regions as part of its maritime strategy.
After a "strategic retreat," the Southern Hemisphere has assumed a new dimension for Chinese interests in South America.
Beijing has been increasing its diplomatic engagement with countries in the region, especially Argentina in recent times, through a series of economic, sociocultural, and to a lesser extent, military agreements. This includes the delivery of vaccines and the intention to accelerate an investment plan worth $30 million.
China has focused on several geopolitically sensitive projects in Argentina, all strategic: controlling air and maritime space and strategic facilities in territorial areas monitored by Beijing over Antarctica and the South Atlantic. However, doubts arise about China's intentions...
https://image.nostr.build/f55fc5464d8d09cbbddd0fe803b264a5e885da387c2c6c1702f021875beb18c2.jpg
For Xi Jinping's government, Argentina stands out for its strategic location, the influential posture of its leaders, and its alignment with China's economic and military power expansion. China has made significant investments and infrastructure initiatives in various Argentine regions.
In addition to establishing a presence in the province of Neuquén, China has targeted the port city of Ushuaia in Tierra del Fuego, Antarctica, the South Atlantic islands, and the San Juan region near the Chilean border.
A 2012 agreement between authorities in Argentina's Neuquén province and Beijing allowed the construction of a deep space tracking station near the Chilean border, which caught Washington's attention.
https://image.nostr.build/a3fa7f2c7174ee9d90aaecd9eadb69a2ef82c04c94584165a213b29d2ae8a66e.jpg
In 2014, through a bilateral agreement between the Chinese Lunar Exploration Program, represented by the Satellite Launch and Tracking Control General (CLTC) of the People's Liberation Army (PLA) and Argentina's National Commission on Space Activities (CONAE), the agreement identified the Argentine space station at Bajada del Agrio as the most favorable location for hosting a Chinese base in the Southern Hemisphere.
The project became operational in 2017 on a 200-hectare area and represents the third in a global network and the first outside China. It features a 110-ton, 35-meter-diameter antenna for deep space exploration (telemetry and technology for "terrestrial tracking, command, and data acquisition"), with the CLTC having a special exploration license for a period of 50 years.
https://image.nostr.build/0a469d8bab900c7cefa854594dfdb934febf2758e1a77c7639d394f14cd98491.jpg
The 50-year contract grants the Chinese Communist Party (CCP) the ability to operate freely on Argentine soil. The facility, known as Espacio Lejano, set a precedent for a Chinese ground tracking station in Río Gallegos, on the southeastern coast of Argentina, which was formally announced in 2021.
In 2016, a document issued by the U.S. State Council Information Office raised concerns among the U.S. government and European Union (EU) countries about the potential military and geopolitical uses of the base in the Southern Hemisphere and Antarctica. Another element fueling suspicion is the existence of "secret clauses" in a document signed by the General Directorate of Legal Advisory (DICOL) of Argentina’s Ministry of Foreign Affairs, International Trade, and Worship with the Chinese government.
https://image.nostr.build/1733ba03475755ddf9be4eafc3e9eb838ba8f9fa6e783a4b060f12b89c3f4165.jpg
Since the Espacio Lejano contract was signed, U.S. analysts and authorities have repeatedly expressed concern about China's growing collaboration with Argentina on security and surveillance issues.
In 2023, a general from the U.S. Southern Command stated during a hearing of the House Armed Services Committee: "The PRC [People's Republic of China] has expanded its capacity to extract resources, establish ports, manipulate governments through predatory investment practices, and build potential dual-use space facilities."
https://image.nostr.build/16bbdeae11247d47a97637402866a0414d235d41fe8039218e26c9d11392b487.jpg
The shift in the Argentine government from a leftist spectrum, led by leaders of the São Paulo Forum, to a Milei administration, which has always advocated for libertarian and pro-Western rhetoric, has altered the dynamics of Chinese-Argentine relations in 2024.
Milei assumed office on December 10, 2023, replacing the progressive president Alberto Fernández, who had strengthened ties with China and signed an agreement in 2022 to join the CCP’s Belt and Road Initiative. During his campaign, Milei did not hide his disdain for communist regimes and signaled his intention to move away from socialist policies in favor of a more libertarian direction.
In the nearly seven months since taking office, Milei has implemented major economic reforms and streamlined the government.
https://image.nostr.build/1d534b254529bf10834d81e2ae35ce2698eda2453d5e2b39d98fa50b45c00a59.jpg
Other recent "positive indicators" suggest that the Milei administration is prioritizing defense relations with the United States over China, according to Leland Lazarus, Associate Director of National Security at the Jack D. Gordon Institute for Public Policy at Florida International University.
"The fact is that, in just six months, he has already visited the United States several times. He has met with Secretary [Antony] Blinken, been to the White House... all of this is like absolute music to General Richardson's ears; to President [Joe] Biden's ears," Lazarus told Epoch Times.
General Richardson visited Argentina in April, a trip that included the donation of a C-130H Hercules transport aircraft to the Argentine Air Force and a visit to a naval facility in Ushuaia, Tierra del Fuego, at the southern tip of the country.
"We are committed to working closely with Argentina so that our collaborative security efforts benefit our citizens, our countries, and our hemisphere in enduring and positive ways," she said in a statement at the time.
In Ushuaia, General Richardson met with local military personnel to discuss their role in "safeguarding vital maritime routes for global trade."
https://image.nostr.build/f6d80fee8a7bba03bf11235d86c4f72435ae4be7d201dba81cc8598551e5ed24.jpg
In a statement from the Argentine Ministry of Defense, Milei confirmed that General Richardson also reviewed the progress of an "integrated naval base" at the Ushuaia naval facility. Argentine officials said they also discussed "legislative modernization on defense issues."
Under the previous administration, China had received preferential treatment.
In June 2023, Tierra del Fuego Governor Gustavo Melella approved China's plans to build a "multi-use" port facility near the Strait of Magellan.
The project was met with legislative backlash, as three national deputies and members of the Civic Coalition filed an official complaint against the governor's provincial decree to build the port with Beijing. The same group also accused Melella of compromising Argentina’s national security.
No public records show that the project has progressed since then.
https://image.nostr.build/3b2b57875dc7ac162ab2b198df238cb8479a7d0bbce32b4042e11063b5e2779b.jpg
Argentina's desire for deeper security cooperation with Western partners was also evident in April when Argentine Defense Minister Luis Petri signed a historic agreement to purchase 24 F-16 fighter jets from Denmark.
"Today we are concluding the most important military aviation acquisition since 1983," Petri said in an official statement.
"Thanks to this investment in defense, I can proudly say that we are beginning to restore our air sovereignty and that our entire society is better protected against all the threats we face."
https://image.nostr.build/8aa0a6261e61e35c888d022a537f03a0fb7a963a78bf2f1bec9bf0a242289dba.jpg
The purchase occurred after several media reports in 2022 indicated that the previous Fernández administration was considering buying JF-17 fighter jets made in China and Pakistan. A former minister from ex-president Mauricio Macri's government, who requested anonymity, confirmed to Epoch Times that a deal to acquire JF-17 jets was being considered during the Fernández era.
Chinese investment did not occur only in Argentina. According to a document from the U.S. House Foreign Affairs Committee: "From 2009 to 2019, China transferred a total of $634 million in significant military equipment to five South American countries—Argentina, Bolivia, Ecuador, Peru, and Venezuela. The governments of Venezuela, Ecuador, Bolivia, and Argentina purchased defense equipment from the PRC, cooperated in military exercises, and engaged in educational exchanges and training for their military personnel."
https://image.nostr.build/ed6d8daeea418b7e233ef97c90dee5074be64bd572f1fd0a5452b5960617c9ca.jpg
Access to space plays a crucial role in the CCP's strategic objectives.
Thus, when reports emerged in early April that Milei's government wanted to inspect Espacio Lejano, experts suggested it supported his national security moves away from China.
According to the Espacio Lejano contract, signed under Cristina Fernández de Kirchner's Peronist regime, CCP officials are not required to let anyone—including the Argentine president—enter the facility without prior notice.
According to Article 3, the agreement stipulates that Argentine authorities cannot interfere with or interrupt the "normal activities" of the facility and must explore alternative options and provide an unspecified amount of notice before being granted access.
China has maintained that Espacio Lejano is for deep space exploration, lunar missions, and communication with satellites already in orbit. However, there is deep skepticism that the claim of space exploration alone is highly unlikely.
The big question is: what could this facility do in times of war?
https://image.nostr.build/f46a2807c02c512e70b14981f07a7e669223a42f3907cbddec952d5b27da9895.jpg
Neuquén is just one of 11 ground stations and space research facilities China has in Latin America and the Caribbean. This represents the largest concentration of space equipment China has outside its own country. According to data from the Gordon Institute, the Chinese Espacio Lejano station and the Río Gallegos facility provide an ideal surveillance position near the polar orbit.
The polar orbit is useful for data collection, transmission, and tracking because it allows for observation of the entire planet from space. The resolution of communications is also improved due to the proximity of satellites in orbit to the Earth's surface.
Additionally, it offers strategic advantages for any government involved in espionage.
https://image.nostr.build/39215a4c9f84cbbaf517c4fda8a562bba9e0cd3af3d453a24d3a9b454c5d015d.jpg
Regarding deeper security collaboration with the United States, the trend is that Milei’s government will do as much as possible without jeopardizing its contracts with China, which is currently Argentina's second-largest trading partner.
However, if Argentina's defense cooperation with China cools, the communist regime might wait for another Argentine government to continue its expansion—a government that could be more favorable to the CCP's objectives.
Everything will depend on the continued success of Javier Milei's economic miracle, ensuring his government is re-elected and he can appoint a successor, making it more challenging for China, and avoiding a situation similar to what occurred in Brazil starting in 2023.
https://image.nostr.build/a5dd3e59a703c553be60534ac5a539b1e50496c71904d01b16471086e9843cd4.jpg
-

@ 468f729d:5ab4fd5e
2024-11-21 23:39:06
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/RFA4XJ5HfG4?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
```js
import prisma from "../prisma";
export const getAllDraftsByUserId = async (userId) => {
return await prisma.draft.findMany({
where: { userId },
include: {
user: true,
},
});
}
export const getDraftById = async (id) => {
return await prisma.draft.findUnique({
where: { id },
include: {
user: true,
},
});
};
export const createDraft = async (data) => {
return await prisma.draft.create({
data: {
...data,
user: {
connect: {
id: data.user,
},
},
additionalLinks: data.additionalLinks || [],
},
});
};
export const updateDraft = async (id, data) => {
const { user, additionalLinks, ...otherData } = data;
return await prisma.draft.update({
where: { id },
data: {
...otherData,
user: user ? {
connect: { id: user }
} : undefined,
additionalLinks: additionalLinks || undefined,
},
});
};
export const deleteDraft = async (id) => {
return await prisma.draft.delete({
where: { id },
});
}
```
-

@ 72592ec9:ffa64d2c
2024-11-21 20:47:50
A few months ago, I took a crack at trying to solve a Satoshi puzzle that resurfaced in 2013. Satoshi’s private November 2008 draft code was revealed by Ray Dillinger on BitcoinTalk, receiving acclaim from the likes of @peterktodd and others.
This code was originally shared privately by Satoshi with James Donald, Ray Dillinger and Hal Finney. The code was only a draft work in progress, incomplete. It was not yet polished or ready for deployment. But it was where Satoshi was beginning to form his thoughts on Bitcoin's network details, eventually refined and deployed in January of 2009.
After the 2013 BitcoinTalk thread died down, the code was left unexamined in detail for years, forgotten again. But one day, @francispouliot_ tweeted about the v0.0 code. Being curious, I thought I’d take a look to see if there were any differences in this private v0.0 code versus the Bitcoin code published as v0.1 in January of 2009.
There were huge differences. The most outstanding ones included:
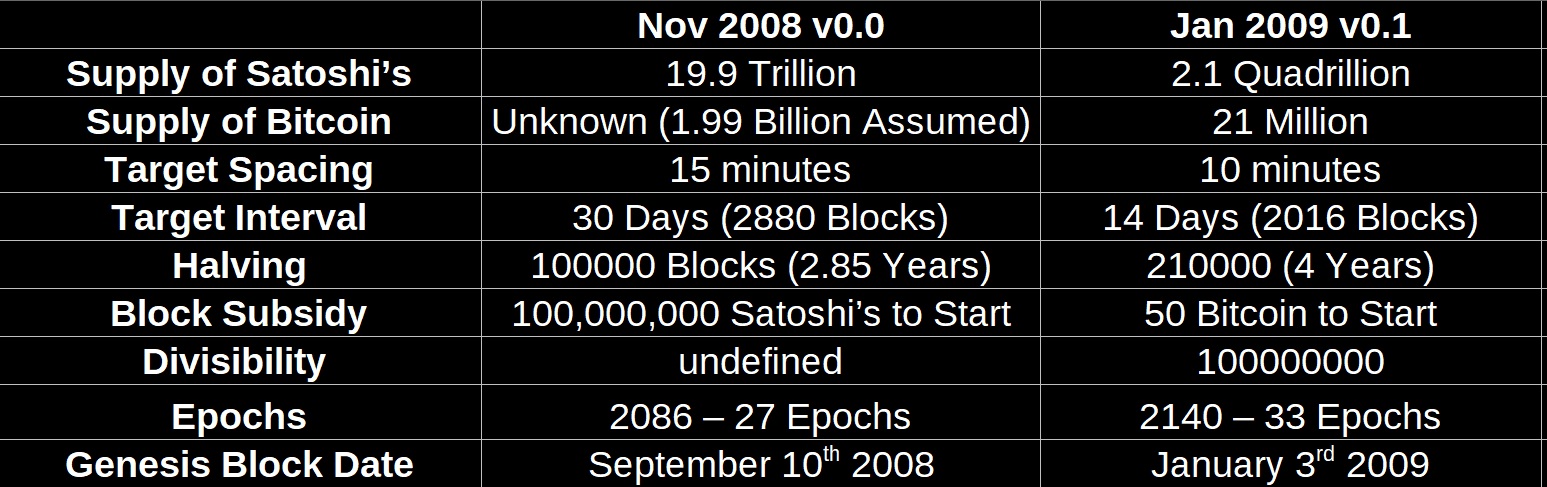
You can see more details about this journey down the rabbit hole of v0.0 code in my earlier part one here: https://medium.com/@Fiach_dubh/1-99-billion-bitcoin-not-21-million-fad9f5550659
Recently, I received some very nice feedback from @lev1tas35619 on this part one, with there interpretation of the Bitcoin v0.0 supply numbers being different from mine. Lev1tas assumed a Bitcoin supply of 20 million units, referring to the COIN variable as supporting evidence for this.
This COIN variable, though defined, is not used at all in the v0.0 code. I've looked for it's usage in the three available files (main.cpp, main.h and node.cpp), and not found it being utilized anywhere.
To Lev1tas’ credit, they sniffed out something I didn’t feel like explaining all that much in my first article.
The fact that bitcoin is not defined at all in v0.0.
Satoshi’s are what are defined in Bitcoin’s code, and from their bitcoin is inferred. In v0.1 this inference is expressed by the following code found in the file util.cpp
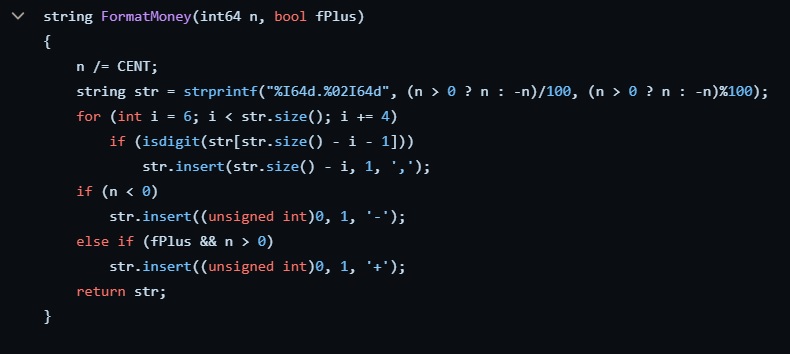
The most important line here is “n /= CENT” which converts the amount from "satoshi’s" to Bitcoin by dividing it by CENT(a constant defined as 1000000). This is used to show "50.000000" bitcoin as the balance in the Bitcoin GUI for someone who successfully mined one of the first Bitcoin blocks.
The issue is that this above code to define the decimal place demarcating Bitcoin’s versus Satoshi’s is nowhere to be found in v0.0 (a lot of code is missing actually). So we are left to assume.
I assumed that if we ported over this above v0.1 code to v0.0, we’d get numbers and decimal places as described in part one. (1.99 Billion Bitcoin & 1.9 Trillion Satoshi’s).
Lev1tas assumes that COIN is used in place of CENT here for v0.0, even though this “n/=COIN” code is missing from v0.0. So there’s kind of two leaps of assumption they’re making here, versus my one assumption. They’re both assuming a port over of this above code, AND and change from the use of CENT to COIN?
But neither of us is really right here, since we don’t really know, the code is missing.
What we can at least say for sure though is that the number of Satoshi’s increased from 19.9 Trillion in v0.0 to 2.1 Quadrillion in v0.1.
The number of bitcoin in v0.0 is unknown, since it was never defined. Or more accurately, the file and lines of code that defined bitcoin for v0.0 was never revealed publicly by Satoshi. Only the Satoshi’s were known.
I want to say a special thank you to Lev1tas for taking the time to engage with me on this interesting bit of Bitcoin history. It’s so fun going through this early Satoshi work, looking at details, and it gets so much better when someone else coinjoins. You mean I’m not the only nerd who likes this stuff?
You sniffed out one of my assumptions, good job! But maybe I’m wrong somewhere and missed something? Please let me know what you all think!
For the medium link of this article: https://medium.com/@Fiach_dubh/satoshis-2008-bitcoin-source-code-v0-0-had-less-bitcoin-4db33fd0e6c9
originally posted at https://stacker.news/items/777712
-

@ b6dcdddf:dfee5ee7
2024-11-21 19:32:10
Hey SN friends!
We built a fun and exciting little thing: Ambassadors.
Ambassadors play an essential role in the Bitcoin ecosystem: they spread the word about valuable Geyser initiatives.
We finally gave them the recognition they deserve on the leaderboards whenever someone contributes through their "hero link".
What do you think? Dope right? The kind of thing you can only/easily do on Lightning ;)
Announcement: https://x.com/geyserfund/status/1859654651148370219
originally posted at https://stacker.news/items/777550
-

@ e968e50b:db2a803a
2024-11-21 17:13:54
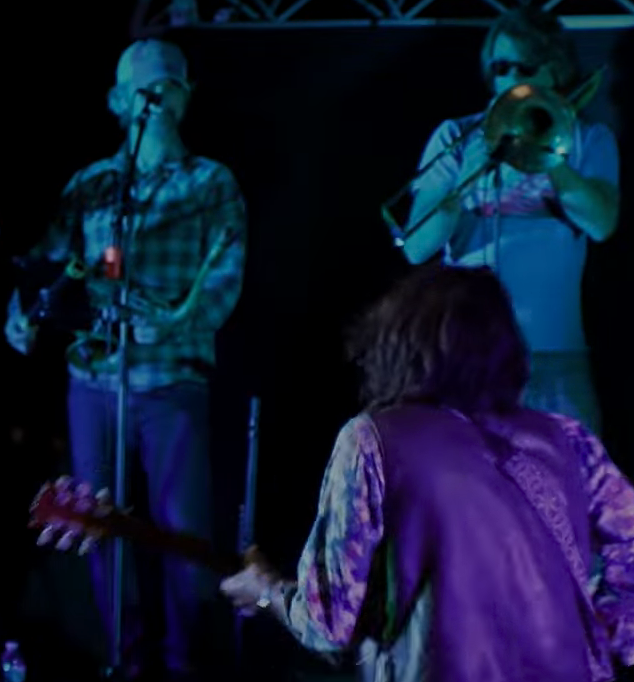
I have a bandmate (not in the group pictured) who, as of last night, has now started telling people, "unless you want an earful, PLEASE don't ask the trombone player what his shirt means."
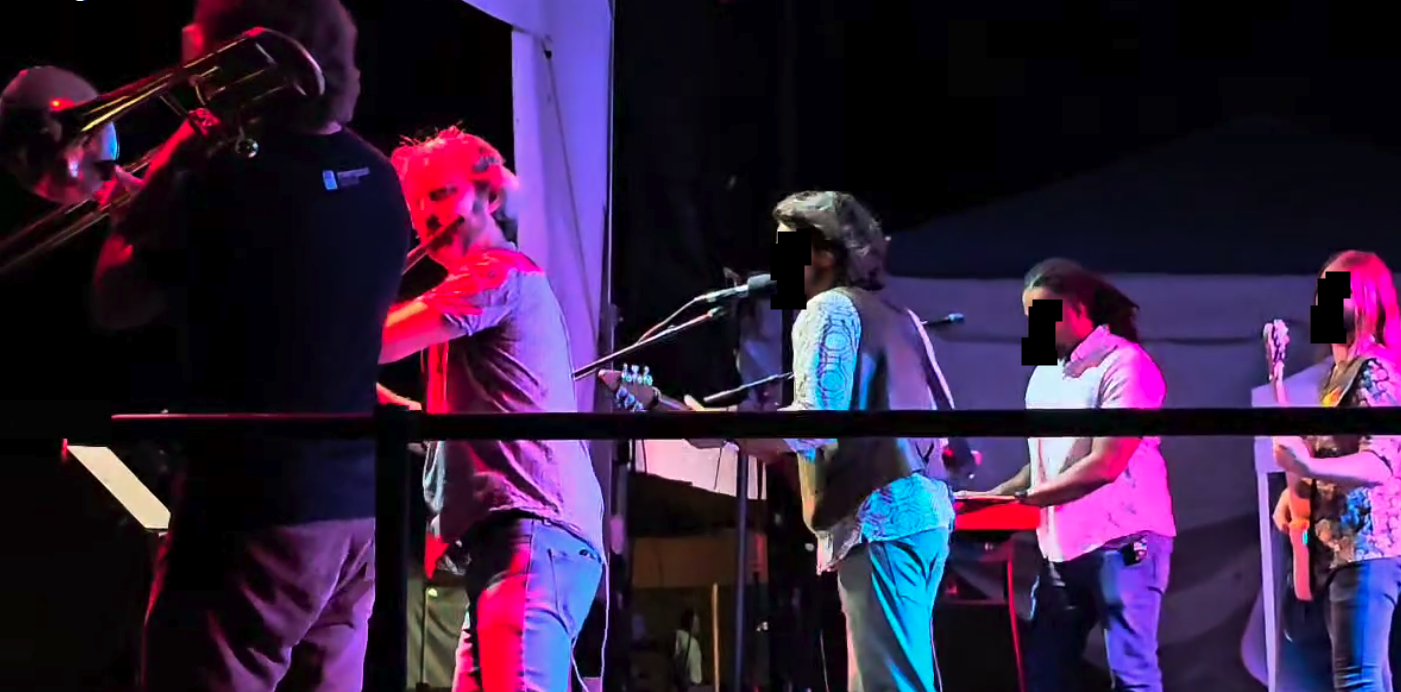
originally posted at https://stacker.news/items/777321
-

@ 7175188c:6382a72c
2024-11-21 16:34:00
It looks like the Megalith LSP node is offline.
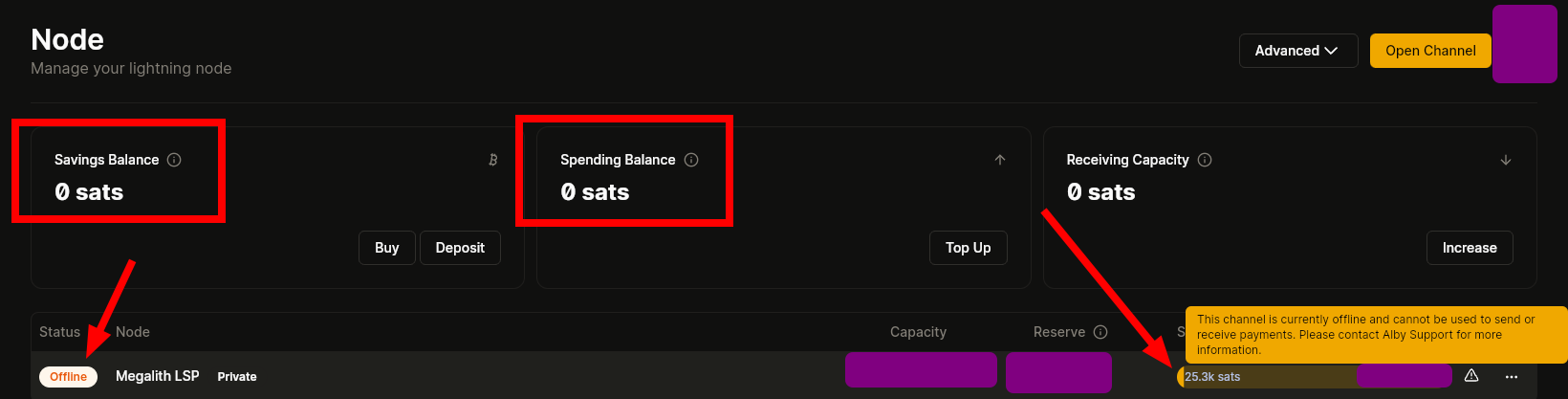
What to do in this situation?
@Alby @getalby
originally posted at https://stacker.news/items/777271
-

@ a39d19ec:3d88f61e
2024-11-21 12:05:09
A state-controlled money supply can influence the development of socialist policies and practices in various ways. Although the relationship is not deterministic, state control over the money supply can contribute to a larger role of the state in the economy and facilitate the implementation of socialist ideals.
## Fiscal Policy Capabilities
When the state manages the money supply, it gains the ability to implement fiscal policies that can lead to an expansion of social programs and welfare initiatives. Funding these programs by creating money can enhance the state's influence over the economy and move it closer to a socialist model. The Soviet Union, for instance, had a centralized banking system that enabled the state to fund massive industrialization and social programs, significantly expanding the state's role in the economy.
## Wealth Redistribution
Controlling the money supply can also allow the state to influence economic inequality through monetary policies, effectively redistributing wealth and reducing income disparities. By implementing low-interest loans or providing financial assistance to disadvantaged groups, the state can narrow the wealth gap and promote social equality, as seen in many European welfare states.
## Central Planning
A state-controlled money supply can contribute to increased central planning, as the state gains more influence over the economy. Central banks, which are state-owned or heavily influenced by the state, play a crucial role in managing the money supply and facilitating central planning. This aligns with socialist principles that advocate for a planned economy where resources are allocated according to social needs rather than market forces.
## Incentives for Staff
Staff members working in state institutions responsible for managing the money supply have various incentives to keep the system going. These incentives include job security, professional expertise and reputation, political alignment, regulatory capture, institutional inertia, and legal and administrative barriers. While these factors can differ among individuals, they can collectively contribute to the persistence of a state-controlled money supply system.
In conclusion, a state-controlled money supply can facilitate the development of socialist policies and practices by enabling fiscal policies, wealth redistribution, and central planning. The staff responsible for managing the money supply have diverse incentives to maintain the system, further ensuring its continuation. However, it is essential to note that many factors influence the trajectory of an economic system, and the relationship between state control over the money supply and socialism is not inevitable.
-

@ b17c5987:85d68928
2024-11-21 10:24:16
Our nation was founded on the basic idea that the people we elect run the government. That isn’t how America functions today. Most legal edicts aren’t laws enacted by Congress but “rules and regulations” promulgated by unelected bureaucrats—tens of thousands of them each year. Most government enforcement decisions and discretionary expenditures aren’t made by the democratically elected president or even his political appointees but by millions of unelected, unappointed civil servants within government agencies who view themselves as immune from firing thanks to civil-service protections.
Our nation was founded on the basic idea that the people we elect run the government. That isn’t how America functions today. Most legal edicts aren’t laws enacted by Congress but “rules and regulations” promulgated by unelected bureaucrats—tens of thousands of them each year. Most government enforcement decisions and discretionary expenditures aren’t made by the democratically elected president or even his political appointees but by millions of unelected, unappointed civil servants within government agencies who view themselves as immune from firing thanks to civil-service protections.
This is antidemocratic and antithetical to the Founders’ vision. It imposes massive direct and indirect costs on taxpayers. Thankfully, we have a historic opportunity to solve the problem. On Nov. 5, voters decisively elected Donald Trump with a mandate for sweeping change, and they deserve to get it.
President Trump has asked the two of us to lead a newly formed Department of Government Efficiency, or DOGE, to cut the federal government down to size. The entrenched and ever-growing bureaucracy represents an existential threat to our republic, and politicians have abetted it for too long. That’s why we’re doing things differently. We are entrepreneurs, not politicians. We will serve as outside volunteers, not federal officials or employees. Unlike government commissions or advisory committees, we won’t just write reports or cut ribbons. We’ll cut costs.
This is antidemocratic and antithetical to the Founders’ vision. It imposes massive direct and indirect costs on taxpayers. Thankfully, we have a historic opportunity to solve the problem. On Nov. 5, voters decisively elected [Donald Trump](https://www.wsj.com/topics/person/donald-trump) with a mandate for sweeping change, and they deserve to get it.
President Trump has asked the two of us to lead a newly formed Department of Government Efficiency, or DOGE, to cut the federal government down to size. The entrenched and ever-growing bureaucracy represents an existential threat to our republic, and politicians have abetted it for too long. That’s why we’re doing things differently. We are entrepreneurs, not politicians. We will serve as outside volunteers, not federal officials or employees. Unlike government commissions or advisory committees, we won’t just write reports or cut ribbons. We’ll cut costs.
We are assisting the Trump transition team to identify and hire a lean team of small-government crusaders, including some of the sharpest technical and legal minds in America. This team will work in the new administration closely with the White House Office of Management and Budget. The two of us will advise DOGE at every step to pursue three major kinds of reform: regulatory rescissions, administrative reductions and cost savings. We will focus particularly on driving change through executive action based on existing legislation rather than by passing new laws. Our North Star for reform will be the U.S. Constitution, with a focus on two critical Supreme Court rulings issued during President Biden’s tenure.
In West Virginia v. Environmental Protection Agency (2022), the justices held that agencies can’t impose regulations dealing with major economic or policy questions unless Congress specifically authorizes them to do so. In Loper Bright v. Raimondo (2024), the court overturned the Chevron doctrine and held that federal courts should no longer defer to federal agencies’ interpretations of the law or their own rulemaking authority. Together, these cases suggest that a plethora of current federal regulations exceed the authority Congress has granted under the law.
DOGE will work with legal experts embedded in government agencies, aided by advanced technology, to apply these rulings to federal regulations enacted by such agencies. DOGE will present this list of regulations to President Trump, who can, by executive action, immediately pause the enforcement of those regulations and initiate the process for review and rescission. This would liberate individuals and businesses from illicit regulations never passed by Congress and stimulate the U.S. economy.
When the president nullifies thousands of such regulations, critics will allege executive overreach. In fact, it will be correcting the executive overreach of thousands of regulations promulgated by administrative fiat that were never authorized by Congress. The president owes lawmaking deference to Congress, not to bureaucrats deep within federal agencies. The use of executive orders to substitute for lawmaking by adding burdensome new rules is a constitutional affront, but the use of executive orders to roll back regulations that wrongly bypassed Congress is legitimate and necessary to comply with the Supreme Court’s recent mandates. And after those regulations are fully rescinded, a future president couldn’t simply flip the switch and revive them but would instead have to ask Congress to do so.
A drastic reduction in federal regulations provides sound industrial logic for mass head-count reductions across the federal bureaucracy. DOGE intends to work with embedded appointees in agencies to identify the minimum number of employees required at an agency for it to perform its constitutionally permissible and statutorily mandated functions. The number of federal employees to cut should be at least proportionate to the number of federal regulations that are nullified: Not only are fewer employees required to enforce fewer regulations, but the agency would produce fewer regulations once its scope of authority is properly limited. Employees whose positions are eliminated deserve to be treated with respect, and DOGE’s goal is to help support their transition into the private sector. The president can use existing laws to give them incentives for early retirement and to make voluntary severance payments to facilitate a graceful exit.
Conventional wisdom holds that statutory civil-service protections stop the president or even his political appointees from firing federal workers. The purpose of these protections is to protect employees from political retaliation. But the statute allows for “reductions in force” that don’t target specific employees. The statute further empowers the president to “prescribe rules governing the competitive service.” That power is broad. Previous presidents have used it to amend the civil service rules by executive order, and the Supreme Court has held—in Franklin v. Massachusetts (1992) and Collins v. Yellen (2021) that they weren’t constrained by the Administrative Procedures Act when they did so. With this authority, Mr. Trump can implement any number of “rules governing the competitive service” that would curtail administrative overgrowth, from large-scale firings to relocation of federal agencies out of the Washington area. Requiring federal employees to come to the office five days a week would result in a wave of voluntary terminations that we welcome: If federal employees don’t want to show up, American taxpayers shouldn’t pay them for the Covid-era privilege of staying home.
Finally, we are focused on delivering cost savings for taxpayers. Skeptics question how much federal spending DOGE can tame through executive action alone. They point to the 1974 Impoundment Control Act, which stops the president from ceasing expenditures authorized by Congress. Mr. Trump has previously suggested this statute is unconstitutional, and we believe the current Supreme Court would likely side with him on this question. But even without relying on that view, DOGE will help end federal overspending by taking aim at the $500 billion plus in annual federal expenditures that are unauthorized by Congress or being used in ways that Congress never intended, from $535 million a year to the Corporation for Public Broadcasting and $1.5 billion for grants to international organizations to nearly $300 million to progressive groups like Planned Parenthood.
The federal government’s procurement process is also badly broken. Many federal contracts have gone unexamined for years. Large-scale audits conducted during a temporary suspension of payments would yield significant savings. The Pentagon recently failed its seventh consecutive audit, suggesting that the agency’s leadership has little idea how its annual budget of more than $800 billion is spent. Critics claim that we can’t meaningfully close the federal deficit without taking aim at entitlement programs like Medicare and Medicaid, which require Congress to shrink. But this deflects attention from the sheer magnitude of waste, fraud and abuse that nearly all taxpayers wish to end—and that DOGE aims to address by identifying pinpoint executive actions that would result in immediate savings for taxpayers.
With a decisive electoral mandate and a 6-3 conservative majority on the Supreme Court, DOGE has a historic opportunity for structural reductions in the federal government. We are prepared for the onslaught from entrenched interests in Washington. We expect to prevail. Now is the moment for decisive action. Our top goal for DOGE is to eliminate the need for its existence by July 4, 2026—the expiration date we have set for our project. There is no better birthday gift to our nation on its 250th anniversary than to deliver a federal government that would make our Founders proud.
Mr. Musk is CEO of SpaceX and Tesla. Mr. Ramaswamy, a businessman, is author, most recently, of “Truths: The Future of America First” and was a candidate for the 2024 Republican presidential nomination. President-elect Trump has named them co-heads of the Department of Government Efficiency.
We are assisting the Trump transition team to identify and hire a lean team of small-government crusaders, including some of the sharpest technical and legal minds in America. This team will work in the new administration closely with the White House Office of Management and Budget. The two of us will advise DOGE at every step to pursue three major kinds of reform: regulatory rescissions, administrative reductions and cost savings. We will focus particularly on driving change through executive action based on existing legislation rather than by passing new laws. Our North Star for reform will be the U.S. Constitution, with a focus on two critical Supreme Court rulings issued during President Biden’s tenure.
In *West Virginia v. Environmental Protection Agency* (2022), the justices held that agencies can’t impose regulations dealing with major economic or policy questions unless Congress specifically authorizes them to do so. In *Loper Bright v. Raimondo* (2024), the court overturned the *Chevron* doctrine and held that federal courts should no longer defer to federal agencies’ interpretations of the law or their own rulemaking authority. Together, these cases suggest that a plethora of current federal regulations exceed the authority Congress has granted under the law.
DOGE will work with legal experts embedded in government agencies, aided by advanced technology, to apply these rulings to federal regulations enacted by such agencies. DOGE will present this list of regulations to President Trump, who can, by executive action, immediately pause the enforcement of those regulations and initiate the process for review and rescission. This would liberate individuals and businesses from illicit regulations never passed by Congress and stimulate the U.S. economy.
When the president nullifies thousands of such regulations, critics will allege executive overreach. In fact, it will be *correcting* the executive overreach of thousands of regulations promulgated by administrative fiat that were never authorized by Congress. The president owes lawmaking deference to Congress, not to bureaucrats deep within federal agencies. The use of executive orders to substitute for lawmaking by adding burdensome new rules is a constitutional affront, but the use of executive orders to roll back regulations that wrongly bypassed Congress is legitimate and necessary to comply with the Supreme Court’s recent mandates. And after those regulations are fully rescinded, a future president couldn’t simply flip the switch and revive them but would instead have to ask Congress to do so.
A drastic reduction in federal regulations provides sound industrial logic for mass head-count reductions across the federal bureaucracy. DOGE intends to work with embedded appointees in agencies to identify the minimum number of employees required at an agency for it to perform its constitutionally permissible and statutorily mandated functions. The number of federal employees to cut should be at least proportionate to the number of federal regulations that are nullified: Not only are fewer employees required to enforce fewer regulations, but the agency would produce fewer regulations once its scope of authority is properly limited. Employees whose positions are eliminated deserve to be treated with respect, and DOGE’s goal is to help support their transition into the private sector. The president can use existing laws to give them incentives for early retirement and to make voluntary severance payments to facilitate a graceful exit.
Conventional wisdom holds that statutory civil-service protections stop the president or even his political appointees from firing federal workers. The purpose of these protections is to protect employees from political retaliation. But the statute allows for “reductions in force” that don’t target specific employees. The statute further empowers the president to “prescribe rules governing the competitive service.” That power is broad. Previous presidents have used it to amend the civil service rules by executive order, and the Supreme Court has held—in *Franklin v. Massachusetts* (1992) and *Collins v. Yellen* (2021) that they weren’t constrained by the Administrative Procedures Act when they did so. With this authority, Mr. Trump can implement any number of “rules governing the competitive service” that would curtail administrative overgrowth, from large-scale firings to relocation of federal agencies out of the Washington area. Requiring federal employees to come to the office five days a week would result in a wave of voluntary terminations that we welcome: If federal employees don’t want to show up, American taxpayers shouldn’t pay them for the Covid-era privilege of staying home.
Finally, we are focused on delivering cost savings for taxpayers. Skeptics question how much federal spending DOGE can tame through executive action alone. They point to the 1974 Impoundment Control Act, which stops the president from ceasing expenditures authorized by Congress. Mr. Trump has previously suggested this statute is unconstitutional, and we believe the current Supreme Court would likely side with him on this question. But even without relying on that view, DOGE will help end federal overspending by taking aim at the $500 billion plus in annual federal expenditures that are unauthorized by Congress or being used in ways that Congress never intended, from $535 million a year to the Corporation for Public Broadcasting and $1.5 billion for grants to international organizations to nearly $300 million to progressive groups like Planned Parenthood.
The federal government’s procurement process is also badly broken. Many federal contracts have gone unexamined for years. Large-scale audits conducted during a temporary suspension of payments would yield significant savings. The Pentagon recently failed its seventh consecutive audit, suggesting that the agency’s leadership has little idea how its annual budget of more than $800 billion is spent. Critics claim that we can’t meaningfully close the federal deficit without taking aim at entitlement programs like Medicare and Medicaid, which require Congress to shrink. But this deflects attention from the sheer magnitude of waste, fraud and abuse that nearly all taxpayers wish to end—and that DOGE aims to address by identifying pinpoint executive actions that would result in immediate savings for taxpayers.
With a decisive electoral mandate and a 6-3 conservative majority on the Supreme Court, DOGE has a historic opportunity for structural reductions in the federal government. We are prepared for the onslaught from entrenched interests in Washington. We expect to prevail. Now is the moment for decisive action. Our top goal for DOGE is to eliminate the need for its existence by July 4, 2026—the expiration date we have set for our project. There is no better birthday gift to our nation on its 250th anniversary than to deliver a federal government that would make our Founders proud.
*Mr. Musk is CEO of SpaceX and [Tesla](https://www.wsj.com/market-data/quotes/TSLA). Mr. Ramaswamy, a businessman, is author, most recently, of “Truths: The Future of America First” and was a candidate for the 2024 Republican presidential nomination. President-elect Trump has named them co-heads of the Department of Government Efficiency.*
-

@ 1bda7e1f:bb97c4d9
2024-11-21 04:17:08
### Tldr
- Nostr is an open protocol which is interoperable with all kinds of other technologies
- You can use this interoperability to create custom solutions
- Nostr apps define a custom URI scheme handler "nostr:"
- In this blog I use this to integrate Nostr with NFC cards
- I create a Nostr NFC "login card" which allows me to log into Amethyst client
- I create a Nostr NFC "business card" which allows anyone to find my profile with a tap
### Inter-Op All The Things!
Nostr is a new open social protocol for the internet. This open nature is very exciting because it means Nostr can add new capabilities to all other internet-connected technologies, from browsers to web applications. In my view, it achieves this through three core capabilities.
- A lightweight decentralised identity (Nostr keys, "npubs" and "nsecs"),
- A lightweight data distribution network (Nostr relays),
- A set of data interoperability standards (The Nostr Improvement Protocols "NIPs"), including the "nostr:" URI which we'll use in this post.
The lightweight nature is its core strength. Very little is required to interoperate with Nostr, which means many existing technologies can be easily used with the network.
Over the next few blog posts, I'll explore different Nostr inter-op ideas, and also deliver my first small open source projects to the community. I'll cover–
- NFC cards integrated with Nostr (in this post),
- Workflow Automations integrated with Nostr,
- AI LLMs integrated with Nostr.
#### The "Nostr:" URI
One feature of Nostr is it defines a custom URI scheme handler "nostr:". What is that?
A URI is used to identify a resource in a system. A system will have a protocol handler registry used to store such URI's, and if a system has a URI registered, then it knows what to do when it sees it. You are probably already familiar with some URI schemes such as "http:" and "mailto:". For example, when you click an http link, the system knows that it describes an http resource and opens a web browser to fetch the content from the internet.
A nostr: link operates in the same way. The nostr: prefix indicates a custom URI scheme specifically designed for the Nostr protocol. If a system has a Nostr application installed, that application may have registered "nostr:" in the protocol handler registry. On that system when a "nostr:" URI is clicked, the system will know that it describes a nostr resource and open the Nostr client to fetch the content from the nostr relay network.
This inter-op with the protocol handler registry gives us the power to do nice and exciting things with other technologies.
### Nostr and NFC
Another technology that uses URIs is NFC cards. NFC (Near Field Communication) is a wireless technology that enables devices to exchange data over a few centimeters. It’s widely used in contactless payments, access control, and information sharing.
NFC tags are small chips embedded in cards or stickers which can store data like plain text, URLs, or custom URIs. They are very cheap (cents each) and widely available (Amazon with next day delivery).
When an NFC tag contains a URI, such as a http: (or nostr:) link, it acts as a trigger. Tapping the tag with an NFC-enabled device launches the associated application and processes the URI. For example, tapping a tag with "nostr:..." could open a Nostr client, directing it to a specific login page, public profile, or event.
This inter-op allows us to bridge the physical world to Nostr with just a tap.
#### Many useful ideas
There are many interesting ways to use this. Too many for me to explore. Perhaps some of these are interesting for your next side hustle?
- Nostr NFC "login cards" – tap to log into Amethyst on Android,
- Nostr NFC "business cards" – give to connections so they can tap to load your npub,
- Nostr NFC "payment cards" – integrating lightning network or ecash,
- Nostr NFC "doorbells", "punch cards", "drop boxes", or "dead drops" – put a tag in a specific place and tap to open a location-specific message or chat,
- Integrations with other access control systems,
- Integrations with other home automation systems,
- Many more ...
To start with I have built and use the "login card" and "business card" solutions. This blog post will show you how to do the same.
### Nostr Login Card
You can use an NFC card to log into your Nostr client.
Most Nostr clients accept a variety of login methods, from posting your nsec into the app (insecure) to using a remote signer (more secure). A less known but more secure method is to sign into a session with a tap of a specially-configured NFC card. Amethyst is a Nostr client on Android which supports this type of login.
- A secure method for logging in
- Optionally keeps no log in history on the device after logout
- Does not require users to know or understand how keys work
- Keys are kept secure on a physically-separated card to reduce risk of compromise
Nostr devs think that this is useful for anti-establishment actors–Fair enough. For me, I am interested in this login card pattern as it could be useful for rolling out identities within an organisation context with less training (office workers are already familiar with door access cards). This pattern potentially abstracts away key management to the IT or ops team who provision the cards.
I first discovered this when [Kohei](nostr:npub16lrdq99ng2q4hg5ufre5f8j0qpealp8544vq4ctn2wqyrf4tk6uqn8mfeq) demonstrated it in [his video](nostr:nevent1qqstx0jy5jvzgh7wr6entjuw7h58d7mapupfdpt9hkf7s4gze34a0vspremhxue69uhkummnw3ez6ur4vgh8wetvd3hhyer9wghxuet59upzp47x6q2txs5ptw3fcj8ngj0y7qrnm7z0ft2cptshx5uqgxn2hd4ckqyqd3).
Here's how you set it up at a high level–
1. Buy yourself some NFC cards
2. Get your Nostr key ready in an encrypted, password protected format called "nencryptsec"
3. Write the nencryptsec to the NFC card as a custom URI
4. Tap to load the login screen, and enter your password to login
Here it is in detail–
#### Buy yourself some NFC cards
I found no specific requirements. As usual with Nostr so far, I tried to the cheapest possible route and it worked. Generic brand NFC cards shipped from China, I believe it was 50X for $15 from Amazon. Your mileage may vary.
#### Get your Nostr key ready
Your key will be saved to the NFC card in an encrypted password-protected format called "nencryptsec". Several applications support this. As we'll be using this to login to Amethyst, we will use Amethyst to output the nencryptsec for us.
1. Login to Amethyst with your nsec,
2. Open the sidebar and click "Backup Keys",
3. Enter a password, and click "Encrypt and my secret key",
4. It will add the password-protected key to your clipboard in the format "ncryptsec1...",
5. Remember to backup your password.
#### Write the ncryptsec to the NFC card
1. Download the free [NFC Tools app](https://play.google.com/store/apps/details?id=com.wakdev.wdnfc) to your device, and open it,
2. Click "Write" and "Add a record", then click "Custom URL / URI",
3. Paste your nencryptsec with the nostr URI in front, i.e. "nostr:ncryptsec1..." and click OK,
4. Click "Write". NFC Tools will prompt you to "Approach an NFC tag",
5. Place your NFC card against your phone, and it will write to the card,
6. Your card is ready.
#### Tap to load the login screen
Tap the card against your phone again, and your phone should open the login screen of Amethyst and prompt you for your password.
Once you enter your password, Amethyst will decrypt your nsec and log you in.
Optionally, you can also set the app to forget you once you log out.
You have created a Nostr NFC "login card".
### Nostr Business Card
You can use another NFC card to give anyone you meet a link straight to your Nostr profile.
I attended [Peter McCormack](nostr:npub14mcddvsjsflnhgw7vxykz0ndfqj0rq04v7cjq5nnc95ftld0pv3shcfrlx)'s #CheatCode conference in Sydney and gave a few of these out following the Nostr panel, notably to [Preston Pysh](nostr:npub1s5yq6wadwrxde4lhfs56gn64hwzuhnfa6r9mj476r5s4hkunzgzqrs6q7z) where it [got some cut through](nostr:nevent1qqsdx0acma85u9knejnvfnfms9pfv27g97mfnnq9fnxslsa9vtrx73spremhxue69uhkummnw3ez6ur4vgh8wetvd3hhyer9wghxuet59upzppggp5a66uxvmntlwnpf5384twu9e0xnm5xth9ta58fpt0dexysy26d4nm) and found me my first 100 followers. You can do the same.
To create your Nostr NFC "business card" is even easier than your NFC "login card".
1. Buy yourself some NFC cards,
2. Download the free [NFC Tools app](https://play.google.com/store/apps/details?id=com.wakdev.wdnfc) to your device, and open it,
2. Click "Write" and "Add a record", then click "Custom URL / URI",
3. Write your npub to the NFC card as a custom URI in the format "nostr:npub1..." (e.g. for me this is "nostr:npub1r0d8u8mnj6769500nypnm28a9hpk9qg8jr0ehe30tygr3wuhcnvs4rfsft"),
4. Your card is ready.
Give the card to someone who is a Nostr user, and when they tap the card against their phone it will open their preferred Nostr client and go directly to your Nostr profile page.
You have created a Nostr NFC "business card".
### What I Did Wrong
I like to share what I did wrong so you don't have to make the same mistakes. This time, this was very easy, and little went wrong. In general
- When password-protecting your nsec, don't forget the password!
- When writing to the NFC card, make sure to use "Custom URI/URL" as this accepts your "nostr:" URI scheme. If you use generic "URI/URL" it won't work.
### What's Next
Over my first four blogs I have explored creating a good Nostr setup
- [Mined a Nostr pubkey and backed up the mnemonic](nostr:naddr1qqsy66twd9hxwtteda6hyt2kv9hxjare94g82cntv4uj6drfw4cxyeszyqda5lslwwttmgk3a7vsx0dgl5kuxc5pq7gdlxlx9av3qw9mjlzdjqcyqqq823c6e2ekw)
- [Set up Nostr payments with a Lightning wallet plus all the bells and whistles](nostr:naddr1qq34xet5w35kueedw4cz65rp09kk2mn5wvkk7m3dfehhxarj95mk7dnvwvmsygqmmflp7uukhk3drmueqv763lfdcd3gzpusm7d7vt6ezquth97ymypsgqqqw4rsuql6je)
- [Set up NIP-05 and Lighting Address at my own domain](nostr:naddr1qqyx2efcvy6rvcnrqyghwumn8ghj7mn0wd68ytnhd9hx2tczyqda5lslwwttmgk3a7vsx0dgl5kuxc5pq7gdlxlx9av3qw9mjlzdjqcyqqq823c634nh0)
- [Set up a Personal Relay at my own domain](nostr:naddr1qqyx2efcvy6rvcnrqy2hwumn8ghj7un9d3shjtnyv9kh2uewd9hj7q3qr0d8u8mnj6769500nypnm28a9hpk9qg8jr0ehe30tygr3wuhcnvsxpqqqp65wmzpn9e)
Over the next few blogs I will be exploring different types of Nostr inter-op
- NFC cards integrated with Nostr (this post)
- Workflow Automations integrated with Nostr
- AI LLMs integrated with Nostr
Please be sure to let me know if you think there's another Nostr topic you'd like to see me tackle.
GM Nostr.
-

@ 30ceb64e:7f08bdf5
2024-11-21 01:24:36
Damn,
I always put too many projects on my plate.
I really need to focus on one at a time or a couple of them.
I'm starting to feel the risk of not doing everything I wanted to accomplish.
I think with a few days hard push, I'd be able to knock out a lot.
- Article Blog
- Cool Shopstr Site
- Nostr Running App
- Podcast with AI reading my Blogs, with wavlake beats playing in the background
- Radio Show
- Music Videos
- Couple more Wavlake Albums (TheWildHustle, and Jayhawk)
- Viny Record
- Short Story
- A brand/npub to release some of my projects, instead of overloading my one npub.
This week I want to hit coding and writing hard.
I need to a hard push will get me to a good place, considering the steps i've already taken.
The start date for my part time job has been moved to December 9th, Its a weekend job working 14 hour shifts on Saturday and Sunday......Ha! I'll be assisting teens with disabilities like downsyndrome and whatnot. I'm looking forward to doing something more than just clicking fiat buttons on the computer and possibly making a direct difference in a freaks life.
I've proofed of work pretty well in the bear. Now I need to execute in this early bull.
How I plan to execute
- I'm TheWIldHustle so it behooves me to lean into my sporadic nature
- Get alot of work done when I feel the animal spirits take me
- Broadcast my actions and rely on negative emotions (me not wanting to be a larp) to motivate my actions and force me into work with an angry ferver.
- Try to maintain a constructive mood
More specifically
- Autoposting blogposts to nostr would be a good idea, I already have some brain dumps in obsidian i can throw into an llm and edit to get my points across, and the autoposting will consistancy if done right.
- The radio show is probably just me using zap.stream and streaming all of the beats i've come out with on wavlake so far and so setting up a stream shouldnt be too dificult and i'd iterrate over time with different processes
- I know where to get the vinyl done, I have an album mixed and mastered with artwork bought from a graphic design artist to use for the front cover just need to hit the buy button and offer it on shopstr when it arrives
- The running app at this point is about 20 percent away from being released as a noob beta project. I primarily need to work on nostr login, UI/UX and testing.
- Short story rough outline is done, I'll probably throw the thing into Claude and edit just as I will with the blogposts.
- The podcast will be a reading of my blog posts with my wavlake beats playing in the background
- The shopstr site will sell digital projects like my stories ebook, wavlake beats and beat tapes. Will sell physical stuff like vinyl records, Album artwork as posters, printify merch-like stuff.
- Music videos/Shorts will probably come from AnimalSunrise/Wavlake beats
- Might want to do some sort of brand consultation with a freak on nostr or fiverr to develop a brand to push out some of this stuff.
Fun times ahead,
Thanks for reading,
Wish me luck,
Hustle
originally posted at https://stacker.news/items/776493
-

@ 04222fa1:634e9de5
2024-11-20 22:00:58
So much information about US foreign policy, NATO and the installation of the current Ukrainian government.
I'm just saying, is the western legacy media reporting a one sided account?
originally posted at https://stacker.news/items/776252
-

@ dca6e1cc:ea7d1750
2024-11-20 21:10:51
Hi all,
Are there programs out there that offer funding for students pursuing graduate degrees with a focus on Bitcoin?
I'd love to get funding for a program I'm interested in...
originally posted at https://stacker.news/items/776186
-

@ 5d4b6c8d:8a1c1ee3
2024-11-20 19:19:23
We've made it to the middle of the Group Play portion of the Emirates Cup. Sadly, one of our party didn't make it this far and two others have sustained serious wounds.
It's probably worth thinking about who will still be standing come the Knockout Round. Remember that only eight teams advance.
# Games (Tournament Standings)
- Nets (1-1) @ 76ers (0-2)
- Celtics (1-1) @ Wizards (0-1)
- Warriors (2-0) @ Pelicans (1-1)
- Pacers (0-1) @ Bucks (1-0)
- Hawks (2-0) @ Bulls (0-1)
- Blazers (1-0) @ Rockets (1-0)
- Mavs (1-1) @ Nuggets (1-1)
- Kings (0-1) @ Clippers (0-1)
I never could have imagined wishing that I still had the Hawks available for a pick, but here we are. I'm leaning towards the Kings.
# Prize
49,293 sats and counting! (That'll put some inbound liquidity on my channel)
originally posted at https://stacker.news/items/776040
-

@ e968e50b:db2a803a
2024-11-20 19:03:43
# Braiins Boiler Room
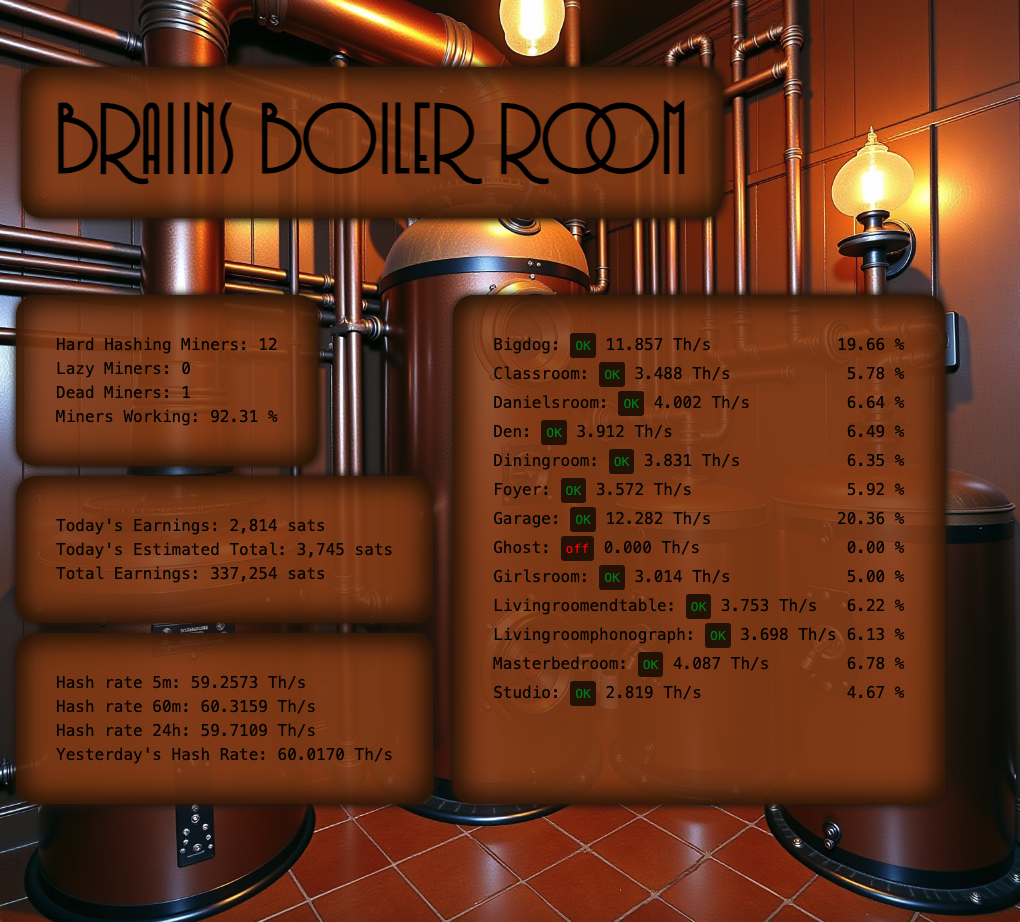
Braiins's mining pool has this API where you can monitor all of your sweet worker stats.
[docs](https://academy.braiins.com/en/braiins-pool/monitoring/#api-configuration)
I started making a boiler room themed dashboard for anybody looking to have all these stats in one spot. You can see how all of your miners are doing and I ran some sophisticated equations (division, multiplication, other hardcore arithmetic) to let you see how your miners are doing in relation to each other.
[Here's the repo for you to use.](https://github.com/ttooccooll/braiinsboilerroom/tree/main)
Use it. Enjoy it. Lemme know what you think. My goal is to turn all of those stats into little retro-future gauges. I want it to look really steam punk. My wife says the Art Deco font isn't steam punk, so maybe it's just going to be 20s themed. IDK Are there any other features you'd want?
**Also, I slapped this together pretty quick, including the README so lemme know if you need help running it.**
Fun fact: If you look at the pic, as of today, my mining revenue (with Braiins) is less than 15,000 sats more than my stacker news revenue. They're neck and neck!
originally posted at https://stacker.news/items/776023
-

@ 000002de:c05780a7
2024-11-20 18:47:13
The 10th amendment to the US Constitution says the following.
> The powers not delegated to the United States by the Constitution, nor prohibited by it to the States, are reserved to the States respectively, or to the people.
When Obama or Biden is in office Democrats seem to believe in a strong federal government. States that oppose the power of the feds over their state's rights are Neo-confederate racists. But something magical occurs when the Democrats lose power. The suddenly discover states rights! This is playing out all over again. Its fun to watch these clowns expose their hypocrisy.
Gavin Newsom is "Trump proofing" California he says. He's also changing his tune on many other issues but that's another topic(in his preparation to run for President in 2028).
The Republicans play the same game. When they hold power many of that party want a nation ban on whatever. They suddenly don't care about the 10th amendment.
If you can't see this hypocrisy in both sides you might need to unplug from the Matrix for a while. Its interesting to me that after over ten years of not being on either side how blind partisans seem to be about reality.
originally posted at https://stacker.news/items/776002
-

@ 5d4b6c8d:8a1c1ee3
2024-11-20 17:31:11
Everyone survived!
That Cavs vs Celtics game was really good. Even though the Cavs historic winning streak came to an end, I was very impressed that they got back into that game. Normally, when the Celtics are shooting the lights out like that, the game is a complete blowout.
@siggy47 barely got out alive, with the Nets only winning by one point. However, that gives him lots of good teams to choose from going forward.
Not having a center finally caught up with OKC. Why don't they use some of those picks to trade for one?
I haven't seen any of the highlights or anything, but the Lakers improved to 2-0 in the tournament, with very beatable Suns and Thunder teams left to play. We'll see if picking the defending champs comes back to bite people in the future.
@grayruby's daughter hit on 4/6 of her picks, which leads me to assume he didn't allow her to participate out of fear of humiliation.
I'll tally up the victory pot for the picks post, but we're still sitting at about 50k for the winner.
originally posted at https://stacker.news/items/775864
-

@ 57d1a264:69f1fee1
2024-11-20 15:06:31
##### _Welcome back to our weekly `JABBB`, **Just Another Bitcoin Bubble Boom**, a comics and meme contest crafted for you, creative stackers!_
If you'd like to learn more, check our welcome post [here](https://stacker.news/items/480740/r/Design_r).
- - -
### This week sticker: `Bitcoin Nostrich`
You can download the source file directly from the [HereComesBitcoin](https://www.herecomesbitcoin.org/) website in [SVG](https://www.herecomesbitcoin.org/assets/Nostrich.svg) and [PNG](https://www.herecomesbitcoin.org/assets/Nostrich.png).
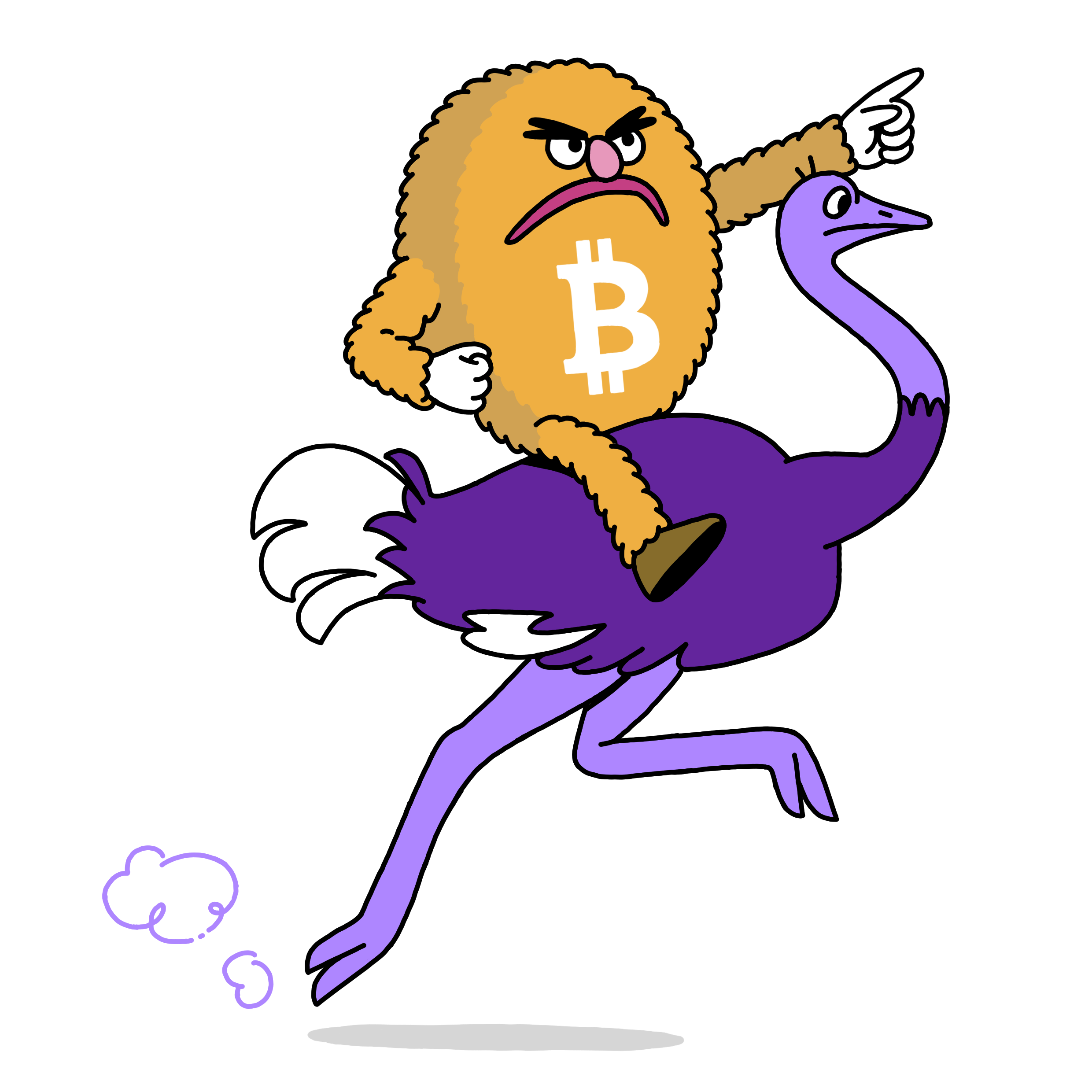
### The task
> Starting from this sticker, design a comic frame or a meme, add a message that perfectly captures the sentiment of the current most hilarious take on the Bitcoin space. You can contextualize it or not, it's up to you, you chose the message, the context and anything else that will help you submit your comic art masterpiece.
> Are you a meme creator? There's space for you too: select the most similar shot from the gifts hosted on the [Gif Station](https://www.herecomesbitcoin.org/) section and craft your best meme... Let's Jabbb!
- - -
If you enjoy designing and memeing, feel free to check our [JABBB archive](https://stacker.news/Design_r#jabbbs--just-another-bitcoin-bubble-boom--comic--meme-contests) and create more to spread Bitcoin awareness to the moon.
Submit each proposal on the relative thread, bounties will be distributed next week together with the release of next JABBB contest.
₿ creative and have fun!
- - -
originally posted at https://stacker.news/items/775646
-

@ 87730827:746b7d35
2024-11-20 09:27:53
Original: https://techreport.com/crypto-news/brazil-central-bank-ban-monero-stablecoins/
Brazilian’s Central Bank Will Ban Monero and Algorithmic Stablecoins in the Country
===================================================================================
Brazil proposes crypto regulations banning Monero and algorithmic stablecoins and enforcing strict compliance for exchanges.
* * *
**KEY TAKEAWAYS**
* The Central Bank of Brazil has proposed **regulations prohibiting privacy-centric cryptocurrencies** like Monero.
* The regulations **categorize exchanges into intermediaries, custodians, and brokers**, each with specific capital requirements and compliance standards.
* While the proposed rules apply to cryptocurrencies, certain digital assets like non-fungible tokens **(NFTs) are still ‘deregulated’ in Brazil**.

In a Notice of Participation announcement, the Brazilian Central Bank (BCB) outlines **regulations for virtual asset service providers (VASPs)** operating in the country.
**_In the document, the Brazilian regulator specifies that privacy-focused coins, such as Monero, must be excluded from all digital asset companies that intend to operate in Brazil._**
Let’s unpack what effect these regulations will have.
Brazil’s Crackdown on Crypto Fraud
----------------------------------
If the BCB’s current rule is approved, **exchanges dealing with coins that provide anonymity must delist these currencies** or prevent Brazilians from accessing and operating these assets.
The Central Bank argues that currencies like Monero make it difficult and even prevent the identification of users, thus creating problems in complying with international AML obligations and policies to prevent the financing of terrorism.
According to the Central Bank of Brazil, the bans aim to **prevent criminals from using digital assets to launder money**. In Brazil, organized criminal syndicates such as the Primeiro Comando da Capital (PCC) and Comando Vermelho have been increasingly using digital assets for money laundering and foreign remittances.
> … restriction on the supply of virtual assets that contain characteristics of fragility, insecurity or risks that favor fraud or crime, such as virtual assets designed to favor money laundering and terrorist financing practices by facilitating anonymity or difficulty identification of the holder.
>
> – [Notice of Participation](https://www.gov.br/participamaisbrasil/edital-de-participacao-social-n-109-2024-proposta-de-regulamentacao-do-)
The Central Bank has identified that **removing algorithmic stablecoins is essential to guarantee the safety of users’ funds** and avoid events such as when Terraform Labs’ entire ecosystem collapsed, losing billions of investors’ dollars.
The Central Bank also wants to **control all digital assets traded by companies in Brazil**. According to the current proposal, the [national regulator](https://techreport.com/cryptocurrency/learning/crypto-regulations-global-view/) will have the **power to ask platforms to remove certain listed assets** if it considers that they do not meet local regulations.
However, the regulations will not include [NFTs](https://techreport.com/statistics/crypto/nft-awareness-adoption-statistics/), real-world asset (RWA) tokens, RWA tokens classified as securities, and tokenized movable or real estate assets. These assets are still ‘deregulated’ in Brazil.
Monero: What Is It and Why Is Brazil Banning It?
------------------------------------------------
Monero ($XMR) is a cryptocurrency that uses a protocol called CryptoNote. It launched in 2013 and ‘erases’ transaction data, preventing the sender and recipient addresses from being publicly known. The Monero network is based on a proof-of-work (PoW) consensus mechanism, which incentivizes miners to add blocks to the blockchain.
Like Brazil, **other nations are banning Monero** in search of regulatory compliance. Recently, Dubai’s new digital asset rules prohibited the issuance of activities related to anonymity-enhancing cryptocurrencies such as $XMR.
Furthermore, exchanges such as **Binance have already announced they will delist Monero** on their global platforms due to its anonymity features. Kraken did the same, removing Monero for their European-based users to comply with [MiCA regulations](https://techreport.com/crypto-news/eu-mica-rules-existential-threat-or-crypto-clarity/).
Data from Chainalysis shows that Brazil is the **seventh-largest Bitcoin market in the world**.

In Latin America, **Brazil is the largest market for digital assets**. Globally, it leads in the innovation of RWA tokens, with several companies already trading this type of asset.
In Closing
----------
Following other nations, Brazil’s regulatory proposals aim to combat illicit activities such as money laundering and terrorism financing.
Will the BCB’s move safeguard people’s digital assets while also stimulating growth and innovation in the crypto ecosystem? Only time will tell.
References
----------
Cassio Gusson is a journalist passionate about technology, cryptocurrencies, and the nuances of human nature. With a career spanning roles as Senior Crypto Journalist at CriptoFacil and Head of News at CoinTelegraph, he offers exclusive insights on South America’s crypto landscape. A graduate in Communication from Faccamp and a post-graduate in Globalization and Culture from FESPSP, Cassio explores the intersection of governance, decentralization, and the evolution of global systems.
[View all articles by Cassio Gusson](https://techreport.com/author/cassiog/)
-

@ 5d4b6c8d:8a1c1ee3
2024-11-20 00:13:15
Anyone else tuning in?
I've got Celtics/Cavs on.
originally posted at https://stacker.news/items/774889
-

@ 468f729d:5ab4fd5e
2024-11-19 20:16:15
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/aWsZX7247cc?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ 6f170f27:711e26dd
2024-11-19 19:36:57
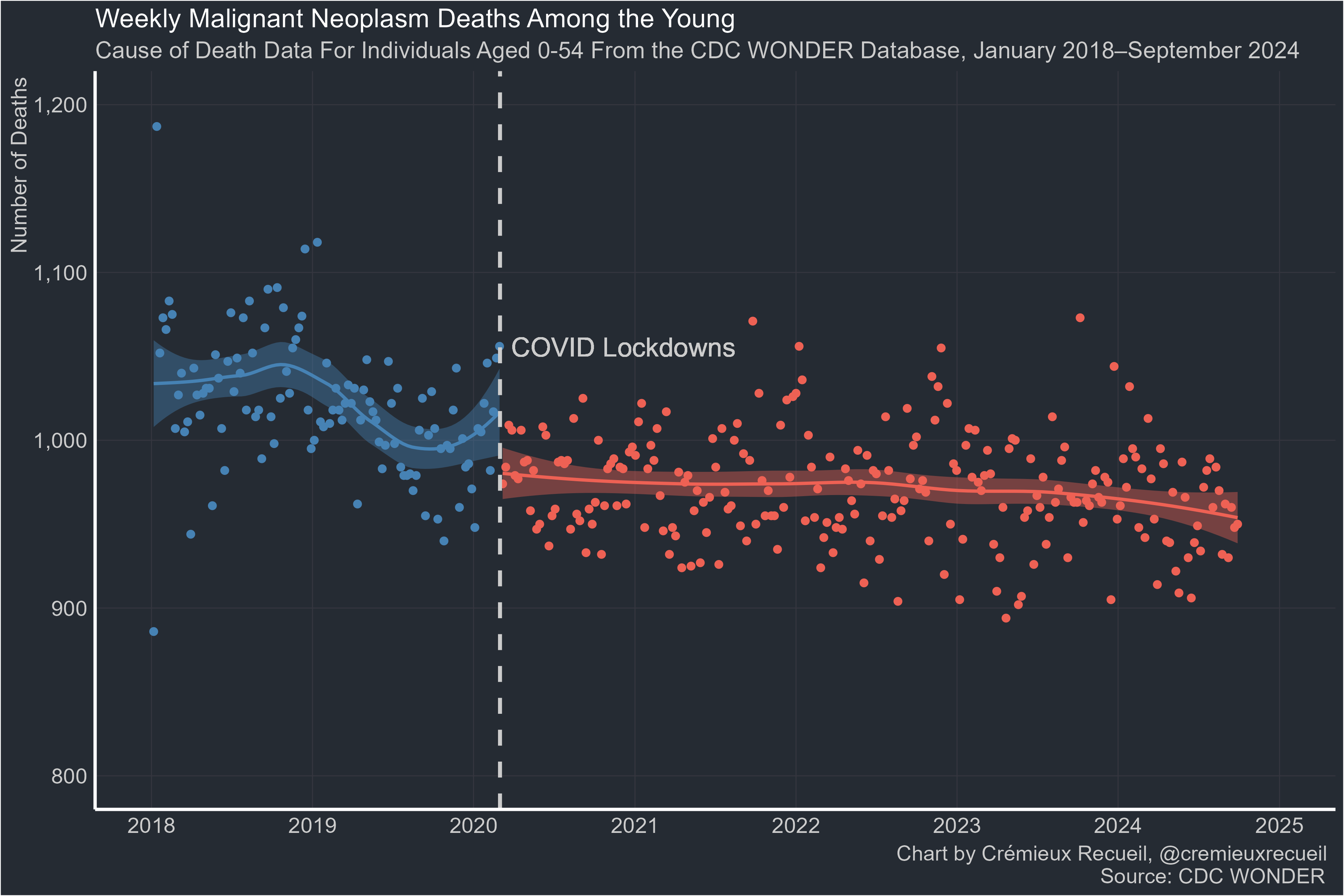
originally posted at https://stacker.news/items/774585
-

@ 57d1a264:69f1fee1
2024-11-19 16:39:49
📙 Sharing the Research plan + Interview Script, lets learn from each other and improve the **[Bitcoin Design Guide](https://bitcoin.design/guide/)**.
🍏 [Previous research](https://github.com/BitcoinDesign/Meta/issues/320) has been done on the guide.
We'll use the [Jobs to be Done framework](https://bitcoinresearch.xyz/jobs-to-be-done) to understand:
- Understand how the Bitcoin Design Guide is being used by builders
- Understand what users typically love about the Bitcoin Design Guide + Community resources
- Understand what friction points exist when using the guide
- Collect a list of key takeaways as to how the Bitcoin Design Guide is useful as a resource to builders as well as how it can be improved
Want to help out? Here's how you can help:
- Feel free to drop comments on the google doc[^1][^2]
- Process the data? These interviews will be done throughout the year and if you're keen to help sort through the data in the end you're most welcome
- Know any builder who has used the guide? Please feel free to send them [our way](https://bitcoin.design)
[^1]: Bitcoin Design Guide Interviews 2024 - Jobs to be done https://docs.google.com/document/d/199wXQYTM75PAIRpApfHpa8KVOo1_veMFhdGgZnRmASw/edit?usp=sharing,
[^2]: Interview script - Jobs to be done https://docs.google.com/document/d/1uFBoZ1nv0HOYBcop2jhqUE4lEEklZT_u5tHeUSqtdNk/edit?tab=t.0#heading=h.c3akgkgb195j
originally posted at https://stacker.news/items/774300
-

@ 8dac122d:0fc98d37
2024-11-19 16:10:43
On this day, 21st day of October, in the year of our Lord 2024 (Gregorian calendar), the nation of Deos Terrum was proclaimed.
Deos Terrum is a nation of self-governing, sui juris, men and women.
https://www.deosterrum.com/
originally posted at https://stacker.news/items/774248
-

@ 5e5fc143:393d5a2c
2024-11-19 10:20:25
Now test old reliable front end
Stay tuned more later
Keeping this as template long note for debugging in future as come across few NIP-33 post edit issues
-

@ 266815e0:6cd408a5
2024-11-19 00:27:34
## Applesauce
I've been working on cleanup up a lot of the old crufty logic in noStrudel and as part of that process I've started breaking out a lot of the "event interpretation" layer into a new library [applesauce](https://github.com/hzrd149/applesauce)
The goal with the library isn't to build another "nostr SDK" but instead to only tackle the problem of how the UI layer of web apps should get data from events and update when new events are detected. Its still a work-in-progress but if your interested check out the [docs](https://hzrd149.github.io/applesauce/modules/applesauce_core.html) (hopefully ill write some examples soon)
## nsite
After SEC-02 I noticed nostr:npub1elta7cneng3w8p9y4dw633qzdjr4kyvaparuyuttyrx6e8xp7xnq32cume created a project built on top of blossom called [nsite](https://github.com/lez/nsite) which was a simple static website CDN using nostr to store the object paths and blossom to store the objects themselves
Because I don't know python I decided to write my own implementation of it in typescript [nsite-ts](https://github.com/hzrd149/nsite-ts) and started hosting it at https://nsite.lol
nostr:npub1klr0dy2ul2dx9llk58czvpx73rprcmrvd5dc7ck8esg8f8es06qs427gxc also created super easy cli tool for uploading static sites to blossom servers called [nsite-cli](https://www.npmjs.com/package/nsite-cli). If your a developer of a nostr web app I would encourage you to give it a try
I also opened my first NIP PR for [static websites](https://github.com/nostr-protocol/nips/pull/1538) :D
## Blossom
Thanks to nostr:npub138s5hey76qrnm2pmv7p8nnffhfddsm8sqzm285dyc0wy4f8a6qkqtzx624, nostr:npub180cvv07tjdrrgpa0j7j7tmnyl2yr6yr7l8j4s3evf6u64th6gkwsyjh6w6, nostr:npub1v0lxxxxutpvrelsksy8cdhgfux9l6a42hsj2qzquu2zk7vc9qnkszrqj49, and nostr:npub1a6we08n7zsv2na689whc9hykpq4q6sj3kaauk9c2dm8vj0adlajq7w0tyc for opening PRs and contributing to the blossom spec. also thanks to everyone else who has shown interest in it. its awesome to watch it slowly grow
There are two more optional BUDs in the spec now
- [BUD-06](https://github.com/hzrd149/blossom/blob/master/buds/06.md) Upload requirements
- [BUD-08](https://github.com/hzrd149/blossom/blob/master/buds/08.md) Nostr File Metadata Tags
And a two breaking changes
- [X-Reason](https://github.com/hzrd149/blossom/pull/32) for all error messages
- Authorization events may have multiple `x` tags with hashes [PR](https://github.com/hzrd149/blossom/pull/24)
## noStrudel
I'm continuing to work on noStrudel and add new features and iron out bugs. I can't list everything here but the next release of the app is shaping up nicely. you can see the changes [here](https://github.com/hzrd149/nostrudel/pull/236)
## Hyper SOCKS5 proxy
Mostly a weekend project but I created a SOCKS5 proxy for hyperDHT and holesail called [hyper-socks5-proxy](https://github.com/hzrd149/hyper-socks5-proxy) that uses a bech32 encoded version of the holesail "connection strings" (public keys) to make them compatible with traditional URLs [hyper-address](https://github.com/hzrd149/hyper-address)
If you want to give it a try you can run it using `npx hyper-socks5-proxy start` to run it locally and have a SOCKS5 proxy that can connect to any hyperDHT or holesail node
Full post here:
nostr:nevent1qvzqqqqqqypzqfngzhsvjggdlgeycm96x4emzjlwf8dyyzdfg4hefp89zpkdgz99qyghwumn8ghj7mn0wd68ytnhd9hx2tcpzfmhxue69uhkummnw3e82efwvdhk6tcqypl8dftpuvdlzfekjd5nc0lmtsldgttv3f0c3uqy3xa3jnqdz4qkw9nptvs
## Other experiments
### CherryTree
A small app for uploading and downloading chunked files on blossom servers [repo](https://github.com/hzrd149/cherry-tree)
### Blossom HLS uploader
A half working cli tool to upload HSL videos to blossom servers [repo](https://github.com/hzrd149/blossom-hls-upload)
### PoW note DVM
A simple content recommendation DVM that recommends notes with the most proof of work [repo](https://github.com/hzrd149/pow-content-dvm)
## Plans for Q4
- Release a working v1 of applesauce packages with documentation
- Release v0.42.0 of noStrudel and start next phase of refactoring the networking code
- Remove "app relays" from noStrudel and make it work entirely on the outbox model
- Try to make WebRTC relays work one last time (before I give up on it forever)
- Work with quentin and leo to release multiple types of paid blossom servers. and also build my own
- More noStrudel features? highlights? NIP-29? cashu wallet?
-

@ af9c48b7:a3f7aaf4
2024-11-18 20:26:07
## Chef's notes
This simple, easy, no bake desert will surely be the it at you next family gathering. You can keep it a secret or share it with the crowd that this is a healthy alternative to normal pie. I think everyone will be amazed at how good it really is.
## Details
- ⏲️ Prep time: 30
- 🍳 Cook time: 0
- 🍽️ Servings: 8
## Ingredients
- 1/3 cup of Heavy Cream- 0g sugar, 5.5g carbohydrates
- 3/4 cup of Half and Half- 6g sugar, 3g carbohydrates
- 4oz Sugar Free Cool Whip (1/2 small container) - 0g sugar, 37.5g carbohydrates
- 1.5oz box (small box) of Sugar Free Instant Chocolate Pudding- 0g sugar, 32g carbohydrates
- 1 Pecan Pie Crust- 24g sugar, 72g carbohydrates
## Directions
1. The total pie has 30g of sugar and 149.50g of carboydrates. So if you cut the pie into 8 equal slices, that would come to 3.75g of sugar and 18.69g carbohydrates per slice. If you decided to not eat the crust, your sugar intake would be .75 gram per slice and the carborytrates would be 9.69g per slice. Based on your objective, you could use only heavy whipping cream and no half and half to further reduce your sugar intake.
2. Mix all wet ingredients and the instant pudding until thoroughly mixed and a consistent color has been achieved. The heavy whipping cream causes the mixture to thicken the more you mix it. So, I’d recommend using an electric mixer. Once you are satisfied with the color, start mixing in the whipping cream until it has a consistent “chocolate” color thorough. Once your satisfied with the color, spoon the mixture into the pie crust, smooth the top to your liking, and then refrigerate for one hour before serving.
-

@ b98139a6:eb269255
2024-11-18 20:15:16
I've been suddenly having trouble posting comments that are priced at 1 sat. I also tested with zaps, and 1sat zaps fail as well. They seem to fail instantly, and show canceled in the wallet history & on AlbyHub (running on my Start9). However, there is no record of the TX failing (or even attempting) in the lightning node itself.
Bigger payments do work, and I tried a 2sat zap which did work. I don't comment too often, but I zap every day, and my zaps are well above 1sat, so I didn't notice until I wanted to write some comments today and they all came back as failing...
Has anyone else seen this? Is there a setting that I somehow messed up? It's odd that the 2sat zap went through fine, and much bigger payments have been working without issue (both outgoing and incoming), so it's not a liquidity issue.
originally posted at https://stacker.news/items/773077
-

@ 5d4b6c8d:8a1c1ee3
2024-11-18 17:13:32
Another OC, another loss. There were some encouraging signs (Bowers might be a generational TE) and we get some clarity on what's wrong.
Thanks to this loss, the Raiders are now picking 4th and it seems that Shedeur is angling to play for the Silver and Black. The stars are aligning a little bit. Also, the Jets continue being a trainwreck, so the pick we got for Davante is moving up the board.
# 2025 Mock Draft
- 4th Pick: QB Jalen Milroe
- 35th Pick: OT Cameron Williams
- 66th Pick: RB Quinshon Judkins
- 71st Pick: DT T.J. Sanders
- 106th Pick: WR Jayden Higgins
- 142nd Pick: IOL Armand Membou
- 179th Pick: CB Zy Alexander
- 212th Pick: QB Dillon Gabriel
- 215th Pick: IOL Seth McLaughlin
- 217th Pick: OT Jude Bowry
It ended up being funny how this board fell, but I don't mind doubling up on all the positions of need. It's maddening watching this team fail to upgrade at QB and on the O-Line.
The Raiders defense is already fine and they'll be getting back two very good D-Linemen next season. They just can't go into next season without addressing the problems on offense.
When are the rest of you going to look to the draft?
originally posted at https://stacker.news/items/772891
-

@ 5d4b6c8d:8a1c1ee3
2024-11-18 15:04:05
Now that we have a month of basketball, we can start reflecting on our predictions. If you want to move on from one of your picks, let me know. Remember that older correct picks are worth more than newer correct picks.
We had a couple new participants join the contest in October. The contest is still open to new folks as the season rolls on.
Here's the current state of the competition with your max possible score next to your name:
| Contestant | MVP | Champ | All NBA | | | | |
|--------------|------|---------|----------|-|-|-|-|
| @Undisciplined 69 | Luka | OKC | Jokic | Giannis | Luka | Ant | SGA |
| @grayruby 70 | Giannis| Pacers| Jokic | Giannis | Luka | Mitchell| Brunson|
| @gnilma 70 | SGA| OKC| Jokic | Wemby| Luka | SGA| Brunson|
| @BitcoinAbhi 70 | Luka| Denver| Jokic | Giannis | Luka | Ant| SGA|
| @Bell_curve 70 | Luka| Celtics| Jokic | Giannis | Luka | Ant| SGA|
| @0xbitcoiner 70 | Jokic| Pacers| Jokic | Giannis | Luka | Ant| Brunson|
| @Coinsreporter 49| Giannis| Pacers| Jokic | Giannis | Luka | Ant| Brunson|
| @TheMorningStar 49| Luka| Celtics| Jokic | Giannis | Luka | Ant| SGA|
| @onthedeklein 49| Luka| T-Wolves| Jokic | Giannis | Luka | Wemby| SGA|
| @Carresan 49| Luka| Mavs| Jokic | Giannis | Luka | Wemby| SGA|
| @BTC_Bellzer 49| Luka| Celtics| Embiid| Giannis | Luka | Tatum| SGA|
| @realBitcoinDog 49| Luka| Lakers| Jokic | Giannis | Luka | Ant| SGA|
| @SimpleStacker 42| SGA| Celtics| Jokic| Tatum| Luka | Brunson| SGA|
| @BlokchainB 42| SGA| Knicks| AD| Giannis | Ant| Brunson| SGA|
I'm not quite ready to move on from Luka, but it's not looking great for Dallas. The nice thing is that Giannis and Jokic are also on struggling teams.
The pot is still only about 4k, but I'm sure it will continue to grow.
If you want to join this contest, just leave your predictions for MVP, Champion, and All-NBA 1st team in the comments. See the [June post](https://stacker.news/items/585231/r/Undisciplined) for more details.
originally posted at https://stacker.news/items/772746
-

@ a79bb203:dde63268
2024-11-18 09:37:20
https://primal.b-cdn.net/media-cache?s=o&a=1&u=https%3A%2F%2Fm.primal.net%2FMZsK.jpg
Find me on Nostr [here](https://njump.me/npub157dmyq6ehpra5xatrn0sssz03qe0y6k69v02xmzyqcrf0h0xxf5qxaw6u8)
originally posted at https://stacker.news/items/772474
-

@ 41e6f20b:06049e45
2024-11-17 17:33:55
Let me tell you a beautiful story. Last night, during the speakers' dinner at Monerotopia, the waitress was collecting tiny tips in Mexican pesos. I asked her, "Do you really want to earn tips seriously?" I then showed her how to set up a Cake Wallet, and she started collecting tips in Monero, reaching 0.9 XMR. Of course, she wanted to cash out to fiat immediately, but it solved a real problem for her: making more money. That amount was something she would never have earned in a single workday. We kept talking, and I promised to give her Zoom workshops. What can I say? I love people, and that's why I'm a natural orange-piller.
-

@ 5d4b6c8d:8a1c1ee3
2024-11-17 16:29:42
We continue on having lost one of our contestants and two others hanging on by a thread.
# Round 3 Games
- Cavs @ Boston
- Hornets @ Nets
- Nuggets @ Grizzlies
- Pelicans @ Mavs
- Thunder @ Spurs
- Jazz @ Lakers
We don't have odds for these games yet and there are injuries galore, so proceed with caution. I'm leaning towards the Mavs, who should make easy work of the Pelicans.
Picks due by tip-off on Tuesday.
# Prize
Let's say 50k, since I know we'll get there soon.
originally posted at https://stacker.news/items/771479
-

@ ac6f9572:8a6853dd
2024-11-17 09:14:36
The Asian Bitcoin Technical Unconference is set!
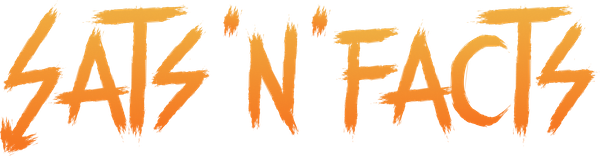
8—10th February 2025 - Chang Mai, Thailand 🇹🇭
- - -
We finally got (nearly) everything ready!
✅ Agenda? NO agenda!
✅ Venue is booked,
✅ Attendees confirmed,
✅ [Registration open](https://satsnfacts.btc.pub/#register) and...
✅ Merch now available!
If you are around Asia this coming Febrary, come and **join us** in Chang Mai, Thailand 🇹🇭
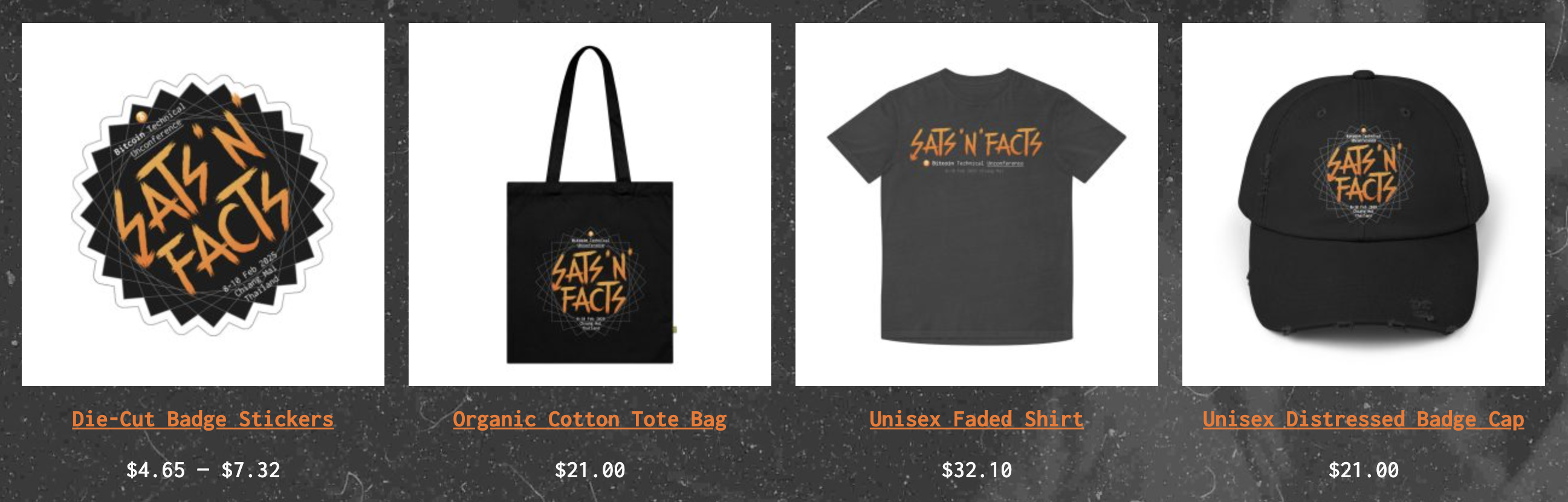
Book your **seat** --> https://formstr.app/#/fill/b3b9bd115fe936848a5e03134fefff945287285d51b1dee4731fef6a1070c597
Submit an **idea** --> https://formstr.app/#/forms/cf02333ea3c0d0c21080df599373e289fa343a55e63a1abdc7f633d1594078ff
Then...
Buy your **ticket** --> https://satsnfacts.btc.pub/p/ticket/
And...
Add some **merch** at check out --> https://satsnfacts.btc.pub/merch/
- - -
If you like to support the event, and the participants joining, consider visit https://geyser.fund/project/satsnfacts
- - -
#SATSnFACTS #event #Biitcoin #Nostr #Lightning #ecash #health #creativity #unconference
originally posted at https://stacker.news/items/771203
-

@ c11cf5f8:4928464d
2024-11-17 08:35:39
Let's hear some of your latest Bitcoin purchases, feel free to include links to the shops or merchants you bought from too.
If you missed our last thread, [here](https://stacker.news/items/761231/r/AG) are some of the items stackers recently spent their sats on.
originally posted at https://stacker.news/items/771187
-

@ a79bb203:dde63268
2024-11-16 21:38:18
I was quite happy with the result (about 3 hours of work)
https://primal.b-cdn.net/media-cache?s=o&a=1&u=https%3A%2F%2Fm.primal.net%2FMYNb.jpg
Bumblebees symbolize hard work, diligence, and productivity.
originally posted at https://stacker.news/items/770779
-

@ 5d4b6c8d:8a1c1ee3
2024-11-16 17:07:19
We almost all survived!
Sorry, @BlokchainB. You took what should have been a very safe Denver team and then neither their very good head coach nor the best player in the world showed up.
**Ode to the fallen**:
> In peace, may you leave this shore. In love, may you find the next. Safe passage on your travels, until our final journey to the ground. May we meet again.
Let's all pour one out for Blok.
----------
# Winners' Recap
The Pistons were really trying to get @supercyclone eliminated, but the Raptors were just too pathetic to hold onto the win.
Despite putting up almost 50 points in the first quarter, Cleveland let the lowly Bulls back into the game. Ultimately, I doubt @realBitcoinDog was ever particularly concerned that the now 14-0 Cavs would put Chicago away.
After seeming to have the game in hand, the Knicks somehow allowed cross-town rival Brooklyn back in the game. Thankfully for @gnilma's wallet, they made two big plays to close out the game.
The Magic, sans young star Paolo, clobbered the supposedly contending 76ers. @Carresan really never had to sweat this one.
In a game of which I watched not one second, the Hawks defeated the woeful Wizards and secured survival for the rest of us.
# Other Notable Games
The Jimmy-less Heat put a real whooping on the Pacers, who are looking miserable this season.
OKC continues winning, despite having no centers in their rotation. I think 6'3" Lu Dort might be playing center.
I caught a little bit of the Lakers, Warriors, and T-Wolves, all of whom looked good in their wins.
# Prize
Closing in on 50k!
originally posted at https://stacker.news/items/770488
-

@ 5d4b6c8d:8a1c1ee3
2024-11-16 00:24:01
I've got Heat-Pacers on and I'm excited about some of the later games.
Is anyone else tuning into the second night of the Emirates Cup?
originally posted at https://stacker.news/items/769843
-

@ f33c8a96:5ec6f741
2024-11-15 21:45:12
# Voltage Tipper ⚡
A Simple Lightning tipping app template built with NextJS and Voltage.
[Voltage Tipper Video Walkthrough](https://www.youtube.com/watch?v=y2eFBtRLRUk)
To deploy, click the button below, create a free Vercel account if you don't have one, fill in the required environment variables from your Voltage node, deploy, and everything should work!
[](https://vercel.com/new/clone?repository-url=https%3A%2F%2Fgithub.com%2FAustinKelsay%2Fvoltage-tipper&env=NEXT_PUBLIC_HOST,NEXT_PUBLIC_INVOICE_MACAROON,NEXT_PUBLIC_READ_MACAROON&envDescription=The%20host%20url%20for%20your%20lnd%20lightning%20node%20(not%20including%20port)%2C%20the%20invoice%20macaroon%20and%20read%20only%20macaroon.&envLink=https%3A%2F%2Fdocs.voltage.cloud%2Flnd-node-api&project-name=voltage-tipper&repository-name=voltage-tipper)
**Run on Replit**
[https://replit.com/@voltage-cloud/voltage-tipper](https://replit.com/@voltage-cloud/voltage-tipper)
## Table of Contents
1. [Deployment](#deployment)
2. [Environment Variables](#environment-variables)
3. [API](#api)
4. [Contributing](#contributing)
5. [License](#license)
## Deployment
To deploy, click the button below, create a free Vercel account if you don't have one, fill in the required environment variables from your Voltage node, deploy, and everything should work!
[](https://vercel.com/new/clone?repository-url=https%3A%2F%2Fgithub.com%2FAustinKelsay%2Fvoltage-tipper&env=NEXT_PUBLIC_HOST,NEXT_PUBLIC_INVOICE_MACAROON,NEXT_PUBLIC_READ_MACAROON&envDescription=The%20host%20url%20for%20your%20lnd%20lightning%20node%20(not%20including%20port)%2C%20the%20invoice%20macaroon%20and%20read%20only%20macaroon.&envLink=https%3A%2F%2Fdocs.voltage.cloud%2Flnd-node-api&project-name=voltage-tipper&repository-name=voltage-tipper)
## Environment Variables
- `NEXT_PUBLIC_HOST`: The host URL for your LND lightning node (not including port).
- `NEXT_PUBLIC_INVOICE_MACAROON`: The invoice macaroon.
- `NEXT_PUBLIC_READ_MACAROON`: The read-only macaroon.
#### Host
The host is your Node's 'API endpoint' you can copy it from your Voltage Dashboard, be sure to include 'https://'. Example: `https://plebdev.m.voltageapp.io`
#### Macaroons
The Invoice and Read macaroons are authentication tokens that allows you to interact with your LND node's REST API with permissions for reading data, and creating invoices. You can also grab a "Admin" macaroon for permissions to spend but that is not required here since we are only receiving through this webapp, so your funds are safu.
**You can find your Macaroons in your Voltage Dashboard by visiting Manage Access -> Macaroon Bakery**
## API
- `/api/lnurl`
Returns lnurlPay response object defined in [LUD-06](https://github.com/lnurl/luds/blob/luds/06.md)
- `/api/getlnurl`
Returns bech32 encoded lnurlPay
- `/.well-known/lnurlp/{NodeAlias}`
This is your lightning address endpoint, returns lnurlPay response defined in [LUD-16](https://github.com/lnurl/luds/blob/luds/16.md)
- `/api/callback?amount=21000`
This is your callback endpoint that will return an invoice when `amount` query parameter is passed with millisat value
## Contributing
Contributions are welcome! Please open an issue or submit a pull request.
## License
This project is licensed under the MIT License. See the LICENSE file for details.
-

@ 5d4b6c8d:8a1c1ee3
2024-11-15 21:03:43
This is a requested topic for a future podcast episode. I do have a giant spreadsheet with a bunch of stats and stuff in it to help figure this out, but I'm doing this off the top of my head.
# My Top 10
1. Jordan: 6 titles, 6 Finals MVPs, 5 MVPs, 1 DPOY, and tops by my eye test
2. Lebron: 4 titles, 4 Finals MVPs, 4 MVPs, 20 All-NBAs, holds virtually all the career playoff stats
3. Kareem: 6 titles, 2 Finals MVPs, 6 MVPs, 15 All-NBAs, also the greatest HS and college player ever
4. Wilt: 2 titles, 1 Finals MVP, 4 MVPs, 10 All-NBAs, holds virtually all single game and single season records
5. Magic: 5 titles, 3 Finals MVPs, 3 MVPs, 10 All-NBAs, greatest passer of all time and played every position
6. Bird: 3 titles, 2 Finals MVPs, 3 MVPs, 10 All-NBAs, most well-rounded player ever
7. Russell: 11 titles, 5 MVPs, 11 All-NBAs, greatest winner in the history of professional sports
8. Duncan: 5 titles, 3 Finals MVPs, 2 MVPs, 15 All-NBAs, most underrated player in NBA history
9. Kobe: 5 titles, 2 Finals MVPs, 1 MVP, 15 All-NBAs, 12 All-Defense teams, more All-Star MVPs than anyone
10. Steph: 4 titles, 1 Finals MVP, 2 MVPs, 10 All-NBA, greatest shooter ever
Honorable mentions: Shaq and Jokic
Shaq and Steph are a toss-up to me. I came down on the side of positional balance. You could think of this as an All-time 1st and 2nd team, rather than purely a top 10.
I expect Jokic to make it, but he's right in the middle of his prime and hasn't yet matched the career achievements of these guys.
Let the arguments begin!
originally posted at https://stacker.news/items/769603
-

@ f33c8a96:5ec6f741
2024-11-15 18:44:32
# Setting Up a React App from Scratch: A Minimal Guide
## Prerequisites
- Node.js and npm installed on your machine
- A text editor of your choice
## Step 1: Create a New Project Directory
```bash
mkdir my-react-app
cd my-react-app
```
## Step 2: Initialize the Project
```bash
npm init -y
```
This creates a package.json file with default values.
## Step 3: Install Dependencies
```bash
npm install react react-dom
npm install --save-dev parcel @babel/preset-react
```
## Step 4: Create Project Structure
Create the following files and directories:
```
my-react-app/
├── src/
│ ├── index.html
│ └── index.js
└── package.json
```
## Step 5: Set Up HTML
In src/index.html, add the following content:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My React App</title>
</head>
<body>
<div id="root"></div>
<script src="./index.js"></script>
</body>
</html>
```
## Step 6: Create React Entry Point
In src/index.js, add the following content:
```javascript
import React from 'react';
import ReactDOM from 'react-dom/client';
const App = () => {
return <h1>Hello, React!</h1>;
};
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
```
## Step 7: Configure Babel
Create a .babelrc file in the project root:
```json
{
"presets": ["@babel/preset-react"]
}
```
## Step 8: Update package.json Scripts
Add the following scripts to your package.json:
```json
"scripts": {
"start": "parcel src/index.html",
"build": "parcel build src/index.html"
}
```
## Step 9: Run the Development Server
```bash
npm start
```
Your app should now be running at http://localhost:1234.
## Step 10: Build for Production
When you're ready to deploy:
```bash
npm run build
```
This will create a dist folder with your optimized production build.
---
Congratulations! You've set up a React app from scratch using Parcel. This setup provides a lightweight and modern development environment with minimal overhead.
-

@ f33c8a96:5ec6f741
2024-11-15 18:39:13
# node-backend-walkthrough

A step-by-step walkthrough on how to setup and deploy a nodejs backend built with Express / Knex / SQLite (dev db) / Postgres (production db)
## Step 1: Setting up your project / dependencies
### 1.1 Create a new Node.js project
To set up a new Node.js project, follow these steps:
- Create a new directory for your project by running the following command in the terminal:
`mkdir my-project`
- Navigate into the new directory by running:
`cd my-project`
- Initialize a new Node.js project by running:
`npm init -y`
### 1.2 Install necessary dependencies
To install the necessary dependencies for your project, run the following command in the terminal:
`npm install express knex sqlite3 dotenv pg nodemon helmet morgan`
This will install the following packages:
- express: A popular web framework for Node.js that simplifies the process of building web applications.
- knex: A SQL query builder for Node.js that provides a convenient way to interact with databases.
- sqlite3: A Node.js module that provides an SQLite database driver for use with Knex.
- dotenv: A zero-dependency module that loads environment variables from a .env file into process.env.
- pg: A PostgreSQL database driver for use with Knex, which enables you to connect and interact with PostgreSQL databases from your Node.js application.
- nodemon: A utility that monitors changes to your source code and automatically restarts your server, saving you from manually stopping and starting your server every time you make changes.
- helmet: A middleware that helps secure your Express app by setting various HTTP headers.
- morgan: A middleware that logs HTTP requests and responses.
You can install additional packages as needed for your project. Once the dependencies are installed, you can begin setting up your Node.js app with Express and Knex.
## Step 2: Setting up your Express server
### 2.1 Create an app.js file
To create the basic Express server, follow these steps:
Create a new file called app.js in the root directory of your project by running the following command in the terminal:
`touch app.js`
Open the app.js file in your preferred code editor.
### 2.2 Import necessary modules and middleware
To use Express, Knex, Dotenv, pg, helmet, and morgan in your app.js file, you need to import them at the top of the file:
```
const express = require('express');
const knex = require('knex');
const dotenv = require('dotenv');
const pg = require('pg');
const helmet = require('helmet');
const morgan = require('morgan');
require('dotenv').config();
```
### 2.3 Set up the server
To set up the server, you need to define the necessary routes and middleware, and configure any necessary settings. Here's an example:
```
const app = express();
const port = process.env.PORT || 5500;
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(helmet());
app.use(morgan('common'));
// Routes
app.get('/', (req, res) => {
res.send('Hello, world!');
});
// Listen
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
module.exports = app;
```
In this example, we're creating a new instance of the Express application (app) and setting the port to listen on. We're also setting up some basic middleware to parse incoming requests (using the express.json() and express.urlencoded() middleware), as well as the helmet() and morgan() middleware for security and logging purposes. We're also defining a simple route that sends a "Hello, world!" message as the response. Finally, we're starting the server by calling the listen() method on the app instance.
You can now run `node app.js` in your termnial and start up the server!
You should now see your .listen() message in the terminal `Server running on port 5500` if there are no errors.
Now you can go into Insomnia or Postman and make a get request to `http://localhost:5500` and you should see your welcome message!
## Step 3: Setting up database config with settings for a local SQLite db and a production Postgres db
### 3.1 Create a knexfile.js file
To use Knex to manage your database, you need to create a knexfile.js file in the root directory of your project. This file will contain the configuration settings for your database, including the connection settings for your local SQLite database and your production Postgres database.
Here's an example knexfile.js:
```
module.exports = {
development: {
client: "sqlite3",
connection: {
filename: "./db/dev.sqlite3",
},
useNullAsDefault: true,
migrations: {
directory: "./db/migrations",
},
seeds: {
directory: "./db/seeds",
},
},
production: {
client: "pg",
connection: process.env.DATABASE_URL,
migrations: {
directory: "./db/migrations",
},
seeds: {
directory: "./db/seeds",
},
},
};
```
In this example, we're defining two environments: development and production. For the development environment, we're using SQLite as the database client and specifying a connection to a local file (./dev.sqlite3). We're also specifying the directories for our migrations and seeds.
For the production environment, we're using Postgres as the database client and specifying a connection to a URL stored in the process.env.DATABASE_URL environment variable. We're using the same directories for our migrations and seeds.
### 3.2 Create a database directory and config
To use the settings from your knexfile.js file in your Express server, you need to create a new directory called `db` (the directory our knexfile is referencing) and inside of db add a file called `dbConfig.js` to create a database instance.
Here's an example:
```
const knex = require('knex');
const config = require('../knexfile');
const env = process.env.NODE_ENV || 'development';
const db = knex(config[env]);
module.exports = db;
```
In this example, we're importing the knex library and the knexfile.js configuration settings. We're then setting the environment to use based on the NODE_ENV environment variable, defaulting to 'development' if the variable is not set. Finally, we're creating a new instance of the database using the configuration settings for the current environment and exporting it for use in our Express server.
You can customize this example to fit your specific needs by updating the configuration settings in your knexfile.js file, or using a different method to set the environment (such as using process.env.DB_ENV).
## Step 4: Setting up a user model and migration
### 4.1 Create a new migration
To set up a user model and database table, you will need to create a new migration file. Migrations are scripts that describe how to modify the database schema.
To create a new migration file, run the following command in your terminal:
`npx knex migrate:make create_users_table`
This will generate a new migration file inside the directory specified in the knexfile.js for migrations (e.g. /db/migrations in the example).
### 4.2 Define the user table schema
Open the newly created migration file and add the schema for the user table. Here is an example:
```
exports.up = function (knex) {
return knex.schema.createTable("users", function (table) {
// Creates an auto-incrementing PK column called id
table.increments("id").primary();
// Creates a text column called username which is both required and unique
table.string("username").notNullable().unique();
// Creates a text column called password which is required
table.string("password").notNullable();
// Creates a timestamp column called created_at which is both required and defaults to the current time
table.timestamps(true, true);
});
};
exports.down = function (knex) {
// Drops the entire table if it exists (opposite of createTable)
// This is useful for rolling back migrations if something goes wrong
return knex.schema.dropTableIfExists("users");
};
```
In this example, we are creating a users table with id, name, email, and password columns. The id column is a primary key, which will automatically generate a unique ID for each row added to the table. The email column is set to be unique, which means that it will be enforced as a unique constraint in the database. The timestamps method is used to automatically create created_at and updated_at columns for the table.
The down method describes how to undo the changes made by the up method.
### 4.3 Seed the database
To seed the database with initial data, you need to create seed files that contain the data you want to insert into your tables. Seed files should be named with a descriptive name and should be placed in the directory specified in your knexfile.js.
To create a new seed file, run the following command in your terminal:
`npx knex seed:make 01_users`
This will create a new seed file named 01_users.js in the seeds directory of your project. You can then edit this file to add the data you want to insert.
In your seed files, you can use Knex to insert data into your tables. Here's an example:
```
exports.seed = function(knex) {
// Deletes ALL existing entries
return knex('users').del()
.then(function () {
// Inserts seed entries
return knex('users').insert([
{ username: 'user1', password: 'password1' },
{ username: 'user2', password: 'password2' },
{ username: 'user3', password: 'password3' }
]);
});
};
```
In this example, we're deleting all existing entries in the users table and then inserting new entries. You can modify this code to match the data you want to insert into your table.
### 4.4 Run the migration
To apply the migration and create the users table in your database, run the following command in your terminal:
`npx knex migrate:latest`
This will execute all pending migrations and update your database schema.
### 4.5 Run the seeds
Now that the user table is created in our local database we can test it by running our seeds and attempting to save our dummy user objects into our database
To run your seed files run the following command in your terminal:
`npx knex seed:run`
Great now we have a local database, we have a schema for our users table, and a seed file with dummy data to save to our db and test our user schema!
## Step 5: Creating and testing a User model
### 5.1 Create a User model
Now that the users table has been created in the database, you can create a User model to interact with it.
These will be the methods that our endpoints call to make changes or get data from our database.
Create a new `models` directory in our `db/` directory and add a file called `User.js` in the models directory and add the following code:
```
// require the database configuration module
const db = require("../dbConfig");
module.exports = {
// a function to find a user by id
findById: (id) => {
// query the 'users' table for the user with the given id
return db("users").where({ id }).first();
},
// a function to create a new user
create: (user) => {
// insert the user object into the 'users' table and return the inserted user object
return db("users").insert(user).returning("*");
},
// a function to update an existing user with the given id
update: (id, user) => {
// update the user object in the 'users' table where the id matches and return the updated user object
return db("users").where({ id }).update(user).returning("*");
},
// a function to delete an existing user with the given id
delete: (id) => {
// delete the user from the 'users' table where the id matches
return db("users").where({ id }).del();
},
};
```
This model has four methods:
- findById: finds a user by their ID
- create: creates a new user in the database
- update: updates an existing user in the database
- delete: deletes a user from the database
Each method returns a Knex query object.
## 5.2 Add routes to interact with the User model
To test the User model, you need to add some routes to your Express app that interact with the User model.
- Create a `routers` directory at the root of your repository.
- Create a new file called `userRouter.js` in the routers directory with the following code:
```
const User = require("../db/models/User");
const router = require("express").Router();
// This route is for creating a new user.
router.post("/", async (req, res) => {
// Extracts the name, email, and password from the request body.
const { username, password } = req.body;
// Calls the User.create function and passes in the extracted values to create a new user.
const user = await User.create({ username, password });
// Sends the created user as a JSON response.
res.json(user);
});
// This route is for getting a user by ID.
router.get("/:id", async (req, res) => {
// Extracts the ID parameter from the request.
const { id } = req.params;
// Calls the User.findById function and passes in the ID to retrieve the user with that ID.
const user = await User.findById(id);
// Sends the retrieved user as a JSON response.
res.json(user);
});
// This route is for updating a user by ID.
router.put("/:id", async (req, res) => {
// Extracts the ID parameter from the request.
const { id } = req.params;
// Extracts the updated username, and password from the request body.
const { username, password } = req.body;
// Calls the User.update function and passes in the ID and updated values to update the user with that ID.
const user = await User.update(id, { username, password });
// Sends the updated user as a JSON response.
res.json(user);
});
// This route is for deleting a user by ID.
router.delete("/:id", async (req, res) => {
// Extracts the ID parameter from the request.
const { id } = req.params;
// Calls the User.delete function and passes in the ID to delete the user with that ID.
const user = await User.delete(id);
// Sends the deleted user as a JSON response.
res.json(user);
});
module.exports = router;
```
Now back in app.js, import the userRouter module and add a new /users route
Your updated app.js should look like this:
```
const express = require("express");
const knex = require("knex");
const dotenv = require("dotenv");
const pg = require("pg");
const helmet = require("helmet");
const morgan = require("morgan");
const userRouter = require("./routers/userRouter");
require("dotenv").config();
const app = express();
const port = process.env.PORT || 5500;
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(helmet());
app.use(morgan("common"));
// Routes
app.get("/", (req, res) => {
res.send("Hello, world!");
});
// Points to the userRouter and adds the /users prefix to all routes in the userRouter.
app.use("/users", userRouter);
// Listen
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
module.exports = app;
```
You can now test these routes using a tool like Insomnia or Postman.
## Step 6: Deploying to Heroku
### 6.1 Create a new Heroku app
To deploy your Node.js app to Heroku, you need to create a new Heroku app. Follow these steps to create a new Heroku app:
- Log in to your Heroku account and navigate to the Heroku Dashboard.
- Click the "New" button in the top right corner and select "Create new app" from the dropdown menu.
- Enter a unique name for your app and select a region.
- Click the "Create app" button.
### 6.2 Connect your Heroku app to your GitHub repository
To connect your Heroku app to your GitHub repository, follow these steps:
- In the "Deploy" tab of your Heroku app dashboard, select "GitHub" as the deployment method.
- Connect your Heroku account to your GitHub account by clicking the "Connect to GitHub" button and following the prompts.
- Select the GitHub repository that contains your Node.js app.
- Choose the branch you want to deploy.
- Click the "Enable Automatic Deploys" button.
### 6.3 Set up environment variables on Heroku
To set up environment variables on Heroku, follow these steps:
- In the "Settings" tab of your Heroku app dashboard, click the "Reveal Config Vars" button.
- Enter the name and value for each environment variable you want to set.
- Click the "Add" button for each environment variable.
In this example, you would need to set the following environment variables:
DATABASE_URL: The connection URL for your production Postgres database. You can find this in the Heroku Postgres add-on settings.
NODE_ENV: The environment setting for your app. Set this to "production".
### 6.4 Deploy your app to Heroku
To deploy your app to Heroku, follow these steps:
- In the "Deploy" tab of your Heroku app dashboard, click the "Deploy Branch" button to deploy your app to Heroku.
- Wait for the deployment process to complete.
- Once the deployment is complete, click the "View" button to open your app in a new browser window.
That's it! Your Node.js app should now be deployed and running on Heroku. If you run into any issues, you can view the logs in the "More" tab of your Heroku app dashboard to help diagnose the problem.
-

@ f33c8a96:5ec6f741
2024-11-15 18:36:56
# pleb-node-template
A simple fullstack Lightning App template for learning / development with LND
## Features:
- Vite frontend with simple darkmode styling / form management / data display.
- LND-GRPC library reducing code and making LND methods easier to work with.
- Express server with basic middleware setup / routes for lightning methods.
- Prebuilt LND methods for creating/paying invoices, openeing/closing channels, and adding/removing peers.
## Local Setup (in regtest using Polar):
1. Make sure that you have [Docker Desktop](https://www.docker.com/products/docker-desktop/) and [Polar](https://lightningpolar.com) installed
2. Open Docker Desktop and wait for it to start
<img width="912" alt="image" src="https://user-images.githubusercontent.com/53542748/234646702-30806d33-85e6-42d2-9409-71c42d00ef4d.png">
3. Open Polar and create a new Lightning network
<img width="832" alt="image" src="https://user-images.githubusercontent.com/53542748/234647029-0c79aabf-6448-49f9-821c-dc54a407b637.png">
4. Create your network however you like, though for starting out it's best to have at least 2 LND nodes to talk to each other.
<img width="1426" alt="image" src="https://user-images.githubusercontent.com/53542748/234647812-84a1472d-2464-4874-b8c7-d6151686ca6b.png">
5. Start your network
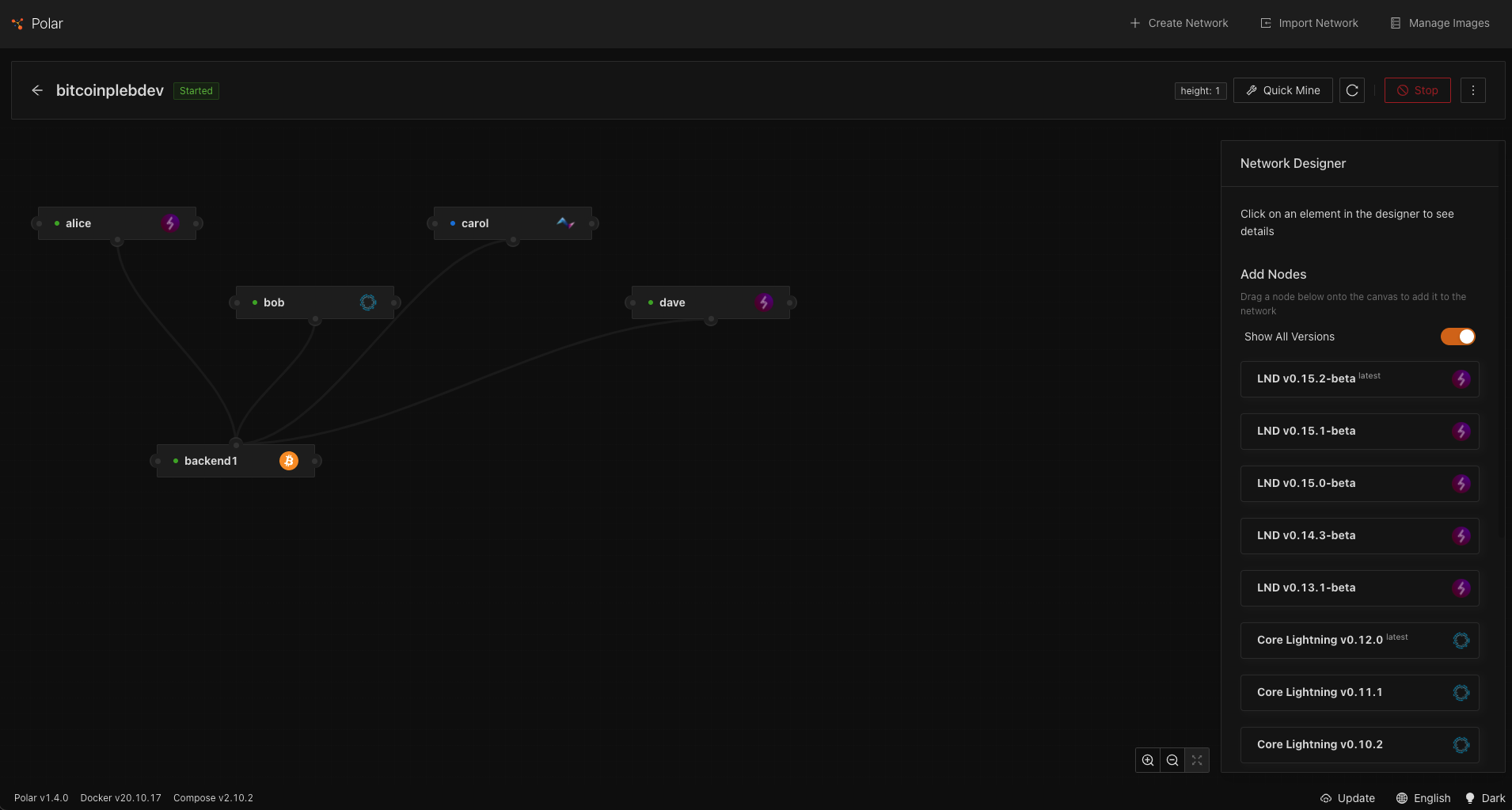
6. Click on the Alice node, visit the connect tab on the right, and copy the 'GRPC Host' value
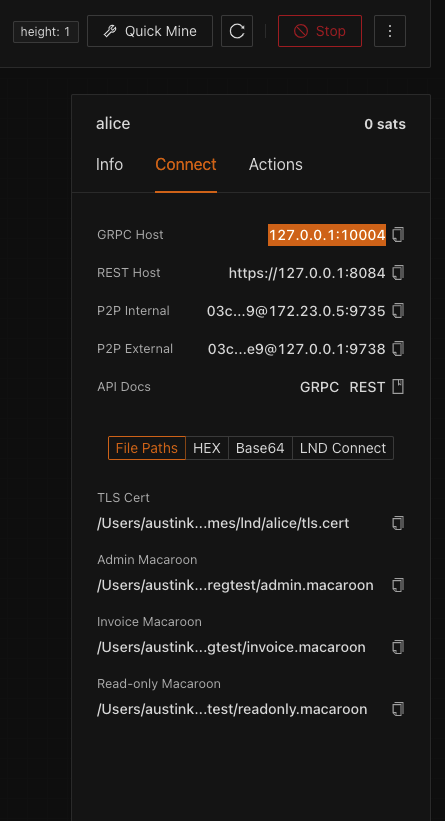
7. Add this as the value to LND_HOST in the .env.sample file
<img width="481" alt="image" src="https://user-images.githubusercontent.com/53542748/235537038-3ba636a8-0931-4db9-91cf-9ad4001781e7.png">
8. Go back to the Connect tab and copy the 'TLS Cert' and 'Admin Macaroon' File Paths and add them as the LND_CERT and LND_MACAROON in .env.sample
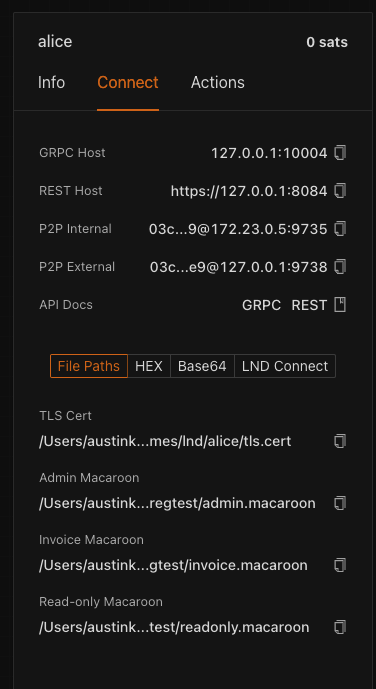
9. Rename the .env.sample to .env
<img width="258" alt="image" src="https://user-images.githubusercontent.com/53542748/235537647-875db3c9-c604-464c-9b61-386d08846a9f.png">
10. Open up the terminal and run `npm i` to install all of the packages and then run `npm run start' to start the server.
<img width="521" alt="image" src="https://user-images.githubusercontent.com/53542748/235538036-0d361a53-c36e-44e0-847c-b439a73172b5.png">
11. Open up a new terminal and run `cd frontend` to navigate to the frontend directory, run `npm i` to install all of the packages, now finally run `npm run dev` to start the frontend.
<img width="657" alt="image" src="https://user-images.githubusercontent.com/53542748/235538322-99afd03b-bc71-4a82-a4ac-fa4753acb57d.png">
12. Visit the Vite local host and you should see pleb-node running. Click 'Connect to your node' and you will see your pubkey and alias immediately populate. Click this button anytime you want to refresh.
<img width="1289" alt="image" src="https://user-images.githubusercontent.com/53542748/235538753-bf63e4e7-de63-4a98-84a0-44fa7e921898.png">
Now in combination with Polar you can open/close channels, create/pay invoices, and add/remove peers.
There is so much more you can do with LND then what is in pleb-node but this should be a great way for you to get started.
Check out lnd.js to see all of the lnd methods being called in pleb-node
-

@ f33c8a96:5ec6f741
2024-11-15 18:33:35
# Rustin Buster Cheatsheet
## Table of Contents
1. [Variable Declaration](#variable-declaration)
2. [Basic Data Types](#basic-data-types)
3. [Structs](#structs)
4. [Implementations (impls)](#implementations-impls)
5. [Enums](#enums)
6. [Pattern Matching](#pattern-matching)
7. [Vectors](#vectors)
8. [Ownership and Borrowing](#ownership-and-borrowing)
9. [Traits](#traits)
10. [Error Handling](#error-handling)
11. [Closures](#closures)
12. [Iterators](#iterators)
13. [Generics](#generics)
14. [Lifetimes](#lifetimes)
15. [Smart Pointers](#smart-pointers)
16. [Concurrency](#concurrency)
17. [Modules](#modules)
## Variable Declaration
In Rust, variables are immutable by default, promoting safer and more predictable code.
```rust
let x = 5; // Immutable variable
let mut y = 10; // Mutable variable
const MAX_POINTS: u32 = 100_000; // Constant
```
- `let` declares an immutable variable.
- `let mut` declares a mutable variable that can be changed later.
- `const` declares a constant, which must have a type annotation and be assigned a constant expression.
## Basic Data Types
Rust has several basic data types to represent numbers, characters, and strings.
```rust
let a: u32 = 42; // Unsigned 32-bit integer
let b: i64 = -100; // Signed 64-bit integer
let c: f32 = 3.14; // 32-bit floating-point number
let d: bool = true; // Boolean
let e: char = 'x'; // Character
let f: &str = "Hello, World!"; // String slice
let g: String = String::from("Hello, World!"); // Owned String
```
- `u32`: Unsigned 32-bit integer (0 to 4,294,967,295)
- `i64`: Signed 64-bit integer (-9,223,372,036,854,775,808 to 9,223,372,036,854,775,807)
- `f32`: 32-bit floating-point number
- `bool`: Boolean (true or false)
- `char`: Unicode scalar value
- `&str`: String slice (borrowed string)
- `String`: Owned string (can be modified)
## Structs
Structs are custom data types that group related data together.
```rust
struct Person {
name: String,
age: u32,
}
// Creating an instance of a struct
let person = Person {
name: String::from("Alice"),
age: 30,
};
// Accessing struct fields
println!("Name: {}", person.name); // Name: Alice
println!("Age: {}", person.age); // Age: 30
// Mutable struct instance
let mut mutable_person = Person {
name: String::from("Bob"),
age: 25,
};
mutable_person.age = 26; // Updating age field
// Tuple Struct
struct Point(i32, i32);
let point = Point(10, 20);
// Unit Struct (marker)
struct Unit;
let unit = Unit;
```
- Regular structs have named fields.
- Tuple structs have unnamed fields, accessed by index.
- Unit structs have no fields and are often used as markers.
## Implementations (impls)
Implementations allow you to define methods and associated functions for structs.
```rust
impl Person {
// Associated function (constructor)
fn new(name: &str, age: u32) -> Person {
Person {
name: String::from(name),
age,
}
}
// Method
fn say_hello(&self) {
println!("Hello, my name is {}", self.name);
}
// Mutable method
fn have_birthday(&mut self) {
self.age += 1;
}
}
// Using the `new` constructor and methods
let mut alice = Person::new("Alice", 30);
alice.say_hello(); // Hello, my name is Alice
alice.have_birthday();
println!("Alice's new age: {}", alice.age); // Alice's new age: 31
```
- Associated functions (like `new`) are called on the struct itself.
- Methods take `&self` or `&mut self` as the first parameter and are called on instances.
## Enums
Enums allow you to define a type that can be one of several variants.
```rust
enum Direction {
North,
South,
East,
West,
}
let direction = Direction::East;
enum OptionalInt {
Value(i32),
Nothing,
}
let x = OptionalInt::Value(42);
let y = OptionalInt::Nothing;
```
- Enums can have variants with no data, like `Direction`.
- Enums can also have variants with associated data, like `OptionalInt`.
## Pattern Matching
Pattern matching is a powerful feature in Rust for destructuring and matching against patterns.
```rust
match direction {
Direction::North => println!("Going North"),
Direction::South => println!("Going South"),
Direction::East => println!("Going East"),
Direction::West => println!("Going West"),
}
match x {
OptionalInt::Value(n) => println!("Value: {}", n),
OptionalInt::Nothing => println!("Nothing"),
}
```
- `match` expressions must be exhaustive, covering all possible cases.
- Pattern matching can destructure enums, tuples, and structs.
## Vectors
Vectors are dynamic arrays that can grow or shrink in size.
```rust
let mut vec = Vec::new();
vec.push(1);
vec.push(2);
vec.push(3);
let first = vec[0]; // 1
// Slices
let slice = &vec[1..3]; // Creates a slice of the vector from index 1 to 2
println!("Slice: {:?}", slice); // Slice: [2, 3]
// Iterating over vectors
for x in &vec {
println!("{}", x);
}
```
- Vectors can store elements of the same type.
- Slices are references to a contiguous sequence of elements in a collection.
- Vectors can be iterated over using a `for` loop.
## Ownership and Borrowing
Rust's ownership system is a key feature that ensures memory safety without a garbage collector.
```rust
let s1 = String::from("hello");
let s2 = s1; // s1 is invalid after this line
let s3 = s2.clone(); // Create a clone
let s4 = String::from("world");
let s5 = &s4; // Borrowing reference
println!("{}, {}", s5, s4); // world, world
```
- When a value is assigned to another variable, ownership is transferred (moved).
- To create a deep copy, use the `clone()` method.
- References allow you to refer to a value without taking ownership.
## Traits
Traits define shared behavior across types.
```rust
trait Summary {
fn summarize(&self) -> String;
}
struct Article {
headline: String,
content: String,
}
impl Summary for Article {
fn summarize(&self) -> String {
format!("{} - {}", self.headline, self.content.split_whitespace().take(5).collect::<Vec<&str>>().join(" "))
}
}
let article = Article {
headline: String::from("Rust Cheatsheet"),
content: String::from("This is a cheatsheet for the Rust programming language."),
};
println!("{}", article.summarize());
```
- Traits are similar to interfaces in other languages.
- Types can implement multiple traits.
- Trait methods can have default implementations.
## Error Handling
Rust uses the `Result` and `Option` types for error handling.
```rust
// Using Result
fn divide(x: f64, y: f64) -> Result<f64, String> {
if y == 0.0 {
Err(String::from("Division by zero"))
} else {
Ok(x / y)
}
}
match divide(10.0, 2.0) {
Ok(result) => println!("Result: {}", result),
Err(e) => println!("Error: {}", e),
}
// Using Option
fn find_user(id: u32) -> Option<String> {
if id == 1 {
Some(String::from("Alice"))
} else {
None
}
}
match find_user(1) {
Some(name) => println!("User found: {}", name),
None => println!("User not found"),
}
```
- `Result<T, E>` represents either success (`Ok(T)`) or failure (`Err(E)`).
- `Option<T>` represents either some value (`Some(T)`) or no value (`None`).
## Closures
Closures are anonymous functions that can capture their environment.
```rust
let add_one = |x: i32| x + 1;
println!("5 + 1 = {}", add_one(5));
```
- Closures can be used as arguments to higher-order functions.
- They can capture variables from their surrounding scope.
## Iterators
Iterators allow you to process sequences of elements.
```rust
let numbers = vec![1, 2, 3, 4, 5];
let doubled: Vec<i32> = numbers.iter().map(|&x| x * 2).collect();
println!("Doubled numbers: {:?}", doubled);
```
- Iterators are lazy and don't compute values until consumed.
- Many methods like `map`, `filter`, and `fold` are available on iterators.
## Generics
Generics allow you to write flexible, reusable code that works with multiple types.
```rust
fn largest<T: PartialOrd>(list: &[T]) -> &T {
let mut largest = &list[0];
for item in list.iter() {
if item > largest {
largest = item;
}
}
largest
}
let numbers = vec![34, 50, 25, 100, 65];
println!("The largest number is {}", largest(&numbers));
```
- `<T: PartialOrd>` specifies that the type `T` must implement the `PartialOrd` trait.
- Generics can be used with functions, structs, and enums.
## Lifetimes
Lifetimes ensure that references are valid for as long as they're used.
```rust
fn longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
let result;
{
let string1 = String::from("short");
let string2 = String::from("longer");
result = longest(string1.as_str(), string2.as_str());
}
println!("The longest string is {}", result);
```
- Lifetimes are denoted with an apostrophe, like `'a`.
- They ensure that references don't outlive the data they refer to.
## Smart Pointers
Smart pointers are data structures that act like pointers but have additional metadata and capabilities.
```rust
use std::rc::Rc;
let s = Rc::new(String::from("Hello, Rust!"));
let s1 = Rc::clone(&s);
let s2 = Rc::clone(&s);
println!("{} has {} strong references", s, Rc::strong_count(&s));
```
- `Rc<T>` (Reference Counted) allows multiple ownership of the same data.
- It keeps track of the number of references to a value.
## Concurrency
Rust provides tools for safe and efficient concurrent programming.
```rust
use std::thread;
use std::time::Duration;
let handle = thread::spawn(|| {
for i in 1..10 {
println!("hi number {} from the spawned thread!", i);
thread::sleep(Duration::from_millis(1));
}
});
handle.join().unwrap();
```
- `thread::spawn` creates a new thread.
- `handle.join()` waits for the spawned thread to finish.
## Modules
Modules help organize and encapsulate code.
```rust
mod math {
pub fn add(a: i32, b: i32) -> i32 {
a + b
}
}
use math::add;
println!("5 + 3 = {}", add(5, 3));
```
- `mod` defines a module.
- `pub` makes items public and accessible outside the module.
- `use` brings items into scope.
This cheatsheet covers many of the fundamental concepts in Rust. Each section provides code examples and explanations to help you understand and use these features effectively in your Rust programs.
-

@ f33c8a96:5ec6f741
2024-11-15 18:31:02
# JavaScript Cheatsheet for Beginners
## Table of Contents
1. [Variable Declaration](#variable-declaration)
2. [Basic Data Types](#basic-data-types)
3. [Operators](#operators)
4. [Objects](#objects)
5. [Functions](#functions)
6. [Arrays](#arrays)
7. [Conditional Statements](#conditional-statements)
8. [Loops](#loops)
9. [Error Handling](#error-handling)
10. [ES6+ Features](#es6-features)
11. [DOM Manipulation](#dom-manipulation)
12. [Event Handling](#event-handling)
13. [Asynchronous JavaScript](#asynchronous-javascript)
14. [Modules](#modules)
## Variable Declaration
In JavaScript, variables can be declared using `var`, `let`, or `const`.
```javascript
var x = 5; // Function-scoped variable (avoid using in modern JavaScript)
let y = 10; // Block-scoped variable
const MAX_POINTS = 100; // Constant (cannot be reassigned)
```
- `var` is function-scoped and hoisted (avoid using in modern JavaScript).
- `let` is block-scoped and can be reassigned.
- `const` is block-scoped and cannot be reassigned (but object properties can be modified).
## Basic Data Types
JavaScript has several basic data types.
```javascript
let num = 42; // Number
let float = 3.14; // Number (JavaScript doesn't distinguish between integers and floats)
let str = "Hello, World!"; // String
let bool = true; // Boolean
let nullValue = null; // Null
let undefinedValue; // Undefined
let symbol = Symbol("unique"); // Symbol (ES6+)
let bigInt = 1234567890123456789012345678901234567890n; // BigInt (ES11+)
```
- `Number`: Represents both integers and floating-point numbers.
- `String`: Represents textual data.
- `Boolean`: Represents `true` or `false`.
- `Null`: Represents a deliberate non-value.
- `Undefined`: Represents a variable that has been declared but not assigned a value.
- `Symbol`: Represents a unique identifier.
- `BigInt`: Represents integers larger than 2^53 - 1.
## Operators
JavaScript includes various types of operators for different operations.
```javascript
// Arithmetic Operators
let sum = 5 + 3; // Addition: 8
let diff = 10 - 4; // Subtraction: 6
let product = 3 * 4; // Multiplication: 12
let quotient = 15 / 3;// Division: 5
let remainder = 17 % 5;// Modulus: 2
let power = 2 ** 3; // Exponentiation: 8
let increment = 5;
increment++; // Increment: 6
let decrement = 5;
decrement--; // Decrement: 4
// Assignment Operators
let x = 10; // Basic assignment
x += 5; // Addition assignment (x = x + 5)
x -= 3; // Subtraction assignment
x *= 2; // Multiplication assignment
x /= 4; // Division assignment
x %= 3; // Modulus assignment
x **= 2; // Exponentiation assignment
// Comparison Operators
console.log(5 == "5"); // Equal to (with type coercion): true
console.log(5 === "5"); // Strictly equal to: false
console.log(7 != "7"); // Not equal to: false
console.log(7 !== "7"); // Strictly not equal to: true
console.log(10 > 5); // Greater than: true
console.log(10 >= 10); // Greater than or equal to: true
console.log(5 < 10); // Less than: true
console.log(5 <= 5); // Less than or equal to: true
// Logical Operators
let a = true, b = false;
console.log(a && b); // Logical AND: false
console.log(a || b); // Logical OR: true
console.log(!a); // Logical NOT: false
// Nullish Coalescing Operator (ES2020)
let nullValue = null;
let defaultValue = nullValue ?? "default"; // "default"
// Optional Chaining Operator (ES2020)
let user = {
address: {
street: "123 Main St"
}
};
console.log(user?.address?.street); // "123 Main St"
console.log(user?.contact?.email); // undefined
// Bitwise Operators
console.log(5 & 3); // Bitwise AND: 1
console.log(5 | 3); // Bitwise OR: 7
console.log(5 ^ 3); // Bitwise XOR: 6
console.log(~5); // Bitwise NOT: -6
console.log(5 << 1); // Left shift: 10
console.log(5 >> 1); // Right shift: 2
console.log(5 >>> 1); // Unsigned right shift: 2
// String Operators
let str1 = "Hello";
let str2 = "World";
console.log(str1 + " " + str2); // Concatenation: "Hello World"
let greeting = "Hello";
greeting += " World"; // Concatenation assignment
// Type Operators
console.log(typeof "Hello"); // "string"
console.log(typeof 123); // "number"
console.log(typeof true); // "boolean"
console.log(obj instanceof Object); // Check if object is instance of a class
```
Key points about operators:
- Arithmetic operators perform mathematical operations
- Assignment operators combine operation with assignment
- Comparison operators return boolean values
- Logical operators work with boolean values and provide short-circuit evaluation
- Nullish coalescing (??) provides a way to specify a default value
- Optional chaining (?.) safely accesses nested object properties
- Bitwise operators perform operations on binary representations
- String operators handle string concatenation
- Type operators help determine variable types
## Objects
Objects are collections of key-value pairs.
```javascript
let person = {
name: "Alice",
age: 30,
sayHello: function() {
console.log("Hello, my name is " + this.name);
}
};
// Accessing object properties
console.log(person.name); // Alice
console.log(person["age"]); // 30
// Calling object methods
person.sayHello(); // Hello, my name is Alice
// Adding a new property
person.job = "Developer";
// Object destructuring (ES6+)
let { name, age } = person;
console.log(name, age); // Alice 30
```
- Objects can contain properties and methods.
- Properties can be accessed using dot notation or bracket notation.
- New properties can be added dynamically.
- Object destructuring allows you to extract multiple properties at once.
## Functions
Functions are reusable blocks of code.
```javascript
// Function declaration
function greet(name) {
return "Hello, " + name + "!";
}
// Function expression
const multiply = function(a, b) {
return a * b;
};
// Arrow function (ES6+)
const add = (a, b) => a + b;
// Default parameters (ES6+)
function power(base, exponent = 2) {
return Math.pow(base, exponent);
}
console.log(greet("Alice")); // Hello, Alice!
console.log(multiply(3, 4)); // 12
console.log(add(5, 3)); // 8
console.log(power(3)); // 9
console.log(power(2, 3)); // 8
```
- Functions can be declared using the `function` keyword or as arrow functions.
- Arrow functions provide a more concise syntax and lexically bind `this`.
- Default parameters allow you to specify default values for function arguments.
## Arrays
Arrays are ordered lists of values.
```javascript
let fruits = ["apple", "banana", "orange"];
// Accessing array elements
console.log(fruits[0]); // apple
// Array methods
fruits.push("grape"); // Add to the end
fruits.unshift("mango"); // Add to the beginning
let lastFruit = fruits.pop(); // Remove from the end
let firstFruit = fruits.shift(); // Remove from the beginning
// Iterating over arrays
fruits.forEach(fruit => console.log(fruit));
// Array transformation
let upperFruits = fruits.map(fruit => fruit.toUpperCase());
// Filtering arrays
let longFruits = fruits.filter(fruit => fruit.length > 5);
// Reducing arrays
let totalLength = fruits.reduce((sum, fruit) => sum + fruit.length, 0);
// Spread operator (ES6+)
let moreFruits = ["kiwi", "pear"];
let allFruits = [...fruits, ...moreFruits];
```
- Arrays can contain elements of any type.
- Array methods like `push`, `pop`, `shift`, and `unshift` modify the original array.
- Higher-order functions like `map`, `filter`, and `reduce` create new arrays.
- The spread operator `...` can be used to combine arrays.
## Conditional Statements
Conditional statements allow you to execute code based on certain conditions.
```javascript
let age = 18;
// if...else statement
if (age >= 18) {
console.log("You are an adult");
} else {
console.log("You are a minor");
}
// Ternary operator
let status = age >= 18 ? "adult" : "minor";
// switch statement
switch (age) {
case 13:
console.log("You're a teenager");
break;
case 18:
console.log("You're now an adult");
break;
default:
console.log("You're neither 13 nor 18");
}
```
- `if...else` statements allow you to execute different code blocks based on conditions.
- The ternary operator provides a concise way to write simple if-else statements.
- `switch` statements are useful when you have multiple conditions to check against a single value.
## Loops
Loops allow you to repeat code multiple times.
```javascript
// for loop
for (let i = 0; i < 5; i++) {
console.log(i);
}
// while loop
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
// do...while loop
let x = 0;
do {
console.log(x);
x++;
} while (x < 5);
// for...of loop (ES6+)
let numbers = [1, 2, 3, 4, 5];
for (let num of numbers) {
console.log(num);
}
// for...in loop (for object properties)
let person = { name: "Alice", age: 30 };
for (let key in person) {
console.log(key + ": " + person[key]);
}
```
- `for` loops are commonly used when you know how many times you want to iterate.
- `while` loops continue as long as a condition is true.
- `do...while` loops always execute at least once before checking the condition.
- `for...of` loops are used to iterate over iterable objects like arrays.
- `for...in` loops are used to iterate over object properties.
## Error Handling
Error handling allows you to gracefully handle and recover from errors.
```javascript
try {
// Code that might throw an error
throw new Error("Something went wrong");
} catch (error) {
console.error("Caught an error:", error.message);
} finally {
console.log("This always runs");
}
// Custom error
class CustomError extends Error {
constructor(message) {
super(message);
this.name = "CustomError";
}
}
try {
throw new CustomError("A custom error occurred");
} catch (error) {
if (error instanceof CustomError) {
console.log("Caught a custom error:", error.message);
} else {
console.log("Caught a different error:", error.message);
}
}
```
- The `try` block contains code that might throw an error.
- The `catch` block handles any errors thrown in the `try` block.
- The `finally` block always executes, regardless of whether an error was thrown.
- You can create custom error types by extending the `Error` class.
## ES6+ Features
ES6 (ECMAScript 2015) and later versions introduced many new features to JavaScript.
```javascript
// Template literals
let name = "Alice";
console.log(`Hello, ${name}!`);
// Destructuring
let [a, b] = [1, 2];
let { x, y } = { x: 3, y: 4 };
// Default parameters
function greet(name = "Guest") {
console.log(`Hello, ${name}!`);
}
// Rest parameters
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
// Spread operator
let arr1 = [1, 2, 3];
let arr2 = [...arr1, 4, 5];
// Arrow functions
const square = x => x * x;
// Classes
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
// Promises
const fetchData = () => {
return new Promise((resolve, reject) => {
// Asynchronous operation
setTimeout(() => resolve("Data fetched"), 1000);
});
};
// Async/Await
async function getData() {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(error);
}
}
```
- Template literals allow for easy string interpolation.
- Destructuring makes it easy to extract values from arrays or properties from objects.
- Default parameters provide fallback values for function arguments.
- Rest parameters allow functions to accept an indefinite number of arguments as an array.
- The spread operator can be used to expand arrays or objects.
- Arrow functions provide a concise syntax for writing function expressions.
- Classes provide a cleaner syntax for creating objects and implementing inheritance.
- Promises and async/await simplify asynchronous programming.
## DOM Manipulation
DOM manipulation allows you to interact with HTML elements on a web page.
```javascript
// Selecting elements
const element = document.getElementById("myElement");
const elements = document.getElementsByClassName("myClass");
const queryElement = document.querySelector(".myClass");
const queryElements = document.querySelectorAll(".myClass");
// Modifying elements
element.textContent = "New text content";
element.innerHTML = "<strong>New HTML content</strong>";
element.style.color = "red";
element.classList.add("newClass");
element.classList.remove("oldClass");
// Creating and appending elements
const newElement = document.createElement("div");
newElement.textContent = "New element";
document.body.appendChild(newElement);
// Removing elements
element.parentNode.removeChild(element);
// or
element.remove(); // Modern browsers
```
- The DOM (Document Object Model) represents the structure of an HTML document.
- You can select elements using methods like `getElementById`, `getElementsByClassName`, `querySelector`, and `querySelectorAll`.
- Elements can be modified by changing their properties like `textContent`, `innerHTML`, and `style`.
- New elements can be created with `createElement` and added to the DOM with `appendChild`.
- Elements can be removed using `removeChild` or the `remove` method.
## Event Handling
Event handling allows you to respond to user interactions and other events.
```javascript
const button = document.querySelector("#myButton");
// Adding event listeners
button.addEventListener("click", function(event) {
console.log("Button clicked!");
console.log("Event object:", event);
});
// Removing event listeners
function handleClick(event) {
console.log("Button clicked!");
}
button.addEventListener("click", handleClick);
button.removeEventListener("click", handleClick);
// Event delegation
document.body.addEventListener("click", function(event) {
if (event.target.matches("#myButton")) {
console.log("Button clicked using event delegation!");
}
});
// Preventing default behavior
const link = document.querySelector("a");
link.addEventListener("click", function(event) {
event.preventDefault();
console.log("Link click prevented");
});
```
- Event listeners can be added to elements using the `addEventListener` method.
- The event object contains information about the event that occurred.
- Event listeners can be removed using `removeEventListener`.
- Event delegation allows you to handle events for multiple elements with a single listener.
- `preventDefault` can be used to stop the default action of an event.
## Asynchronous JavaScript
Asynchronous JavaScript allows you to perform operations without blocking the main thread.
```javascript
// Callbacks
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched");
}, 1000);
}
fetchData(data => console.log(data));
// Promises
function fetchDataPromise() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched");
}, 1000);
});
}
fetchDataPromise()
.then(data => console.log(data))
.catch(error => console.error(error));
// Async/Await
async function fetchDataAsync() {
try {
const data = await fetchDataPromise();
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchDataAsync();
// Fetch API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
```
- Callbacks are functions passed as arguments to be executed later.
- Promises represent the eventual completion or failure of an asynchronous operation.
- Async/await provides a more synchronous-looking way to write asynchronous code.
- The Fetch API is a modern interface for making HTTP requests.
## Modules
Modules allow you to organize your code into reusable pieces.
```javascript
// math.js
export function add(a, b) {
return a + b;
}
export function multiply(a, b) {
return a * b;
}
// main.js
import { add, multiply } from './math.js';
console.log(add(5, 3)); // 8
console.log(multiply(4, 2)); // 8
// Default export
// utils.js
export default function sayHello(name) {
console.log(`Hello, ${name}!`);
}
// main.js
import sayHello from './utils.js';
sayHello("Alice"); // Hello, Alice!
```
- The `export` keyword is used to expose functions, objects, or primitives from a module.
- The `import` keyword is used to bring functionality from other modules into the current module.
- Default exports can be imported without curly braces and can be given any name when importing.
This cheatsheet covers many of the fundamental concepts in JavaScript. Each section provides code examples and explanations to help you understand and use these features effectively in your JavaScript programs.
-

@ f33c8a96:5ec6f741
2024-11-15 18:25:12
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/kFUIfaCpXzA?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ f33c8a96:5ec6f741
2024-11-15 18:23:58
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/7dsR7um-DxA?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ f33c8a96:5ec6f741
2024-11-15 18:21:00
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/QY6euaT_G_E?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ f33c8a96:5ec6f741
2024-11-15 18:06:42
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/tGVxP4RgyI4?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ 468f729d:5ab4fd5e
2024-11-15 17:52:45
<div style="position:relative;padding-bottom:56.25%;height:0;overflow:hidden;max-width:100%;"><iframe src="https://www.youtube.com/embed/wV4I62NMiQ0?enablejsapi=1" style="position:absolute;top:0;left:0;width:100%;height:100%;border:0;" allowfullscreen></iframe></div>
-

@ 5d4b6c8d:8a1c1ee3
2024-11-15 15:07:59
Heading into episode 6, here's a rough outline of what we're planning on talking about.
~Stacker_Sports contest updates: UEFA, Cricket, NFL, NBA
Bitcoiner Athletes: two sports celebrities were in the bitcoin news recently
Hockey: a hot start for a surprising team
NFL
- our head-to-head fantasy matchup (if @grayruby still wants to talk about it after his team's reprehensible outing),
- which contenders are on the outside looking in,
- Why are the Bears so bad at picking OC's?
Tyson vs Paul
NBA
- By popular demand, KAT Corner makes a return
- Thoughts and expectations for the Emirates CUp
- Can the best player win MVP on a garbage team?
- Our early hits and misses of the season
Degenerate Corner: bets we're excited about, platforms we're excited about, ongoing bets we have with each other.
originally posted at https://stacker.news/items/769019
-

@ 07907690:d4e015f6
2024-11-15 10:10:05
PGP (Pretty Good Privacy) adalah protokol enkripsi yang digunakan untuk memastikan kerahasiaan, integritas, dan otentikasi data dalam komunikasi digital. Diciptakan oleh Phil Zimmermann pada tahun 1991, PGP menggunakan kombinasi teknik kriptografi asimetris (kunci publik dan kunci privat) serta simetris untuk melindungi informasi. Berikut adalah berbagai kegunaan PGP:
## Mengamankan Email (Enkripsi dan Dekripsi)
- Kegunaan: Melindungi isi email agar hanya penerima yang memiliki kunci privat yang benar yang dapat membacanya.
- Cara Kerja:
- Pengirim mengenkripsi email menggunakan kunci publik penerima.
- Hanya penerima yang memiliki kunci privat yang dapat mendekripsi dan membaca email tersebut.
- Contoh: Jurnalis yang berkomunikasi dengan informan atau organisasi yang mengirim data sensitif dapat menggunakan PGP untuk melindungi komunikasi mereka dari penyusup.
## Tanda Tangan Digital (Digital Signature)
- Kegunaan: Memastikan keaslian dan integritas pesan atau dokumen, memastikan bahwa pesan tidak diubah dan benar-benar berasal dari pengirim yang sah.
- Cara Kerja:
- Pengirim membuat tanda tangan digital menggunakan kunci privatnya.
- Penerima dapat memverifikasi tanda tangan tersebut menggunakan kunci publik pengirim.
- Contoh: Digunakan untuk memverifikasi keaslian dokumen hukum, email penting, atau perangkat lunak yang diunduh.
## Melindungi File dan Dokumen
- Kegunaan: Mengenkripsi file sensitif agar hanya orang yang memiliki kunci dekripsi yang benar yang dapat membukanya.
- Cara Kerja: File dienkripsi menggunakan kunci publik penerima, dan penerima menggunakan kunci privatnya untuk mendekripsinya.
- Contoh: Perusahaan dapat menggunakan PGP untuk mengenkripsi laporan keuangan, data pelanggan, atau informasi penting lainnya sebelum membagikannya.
## Mengamankan Backup Data
- Kegunaan: Mengenkripsi cadangan data penting untuk melindunginya dari akses yang tidak sah.
- Cara Kerja: File backup dienkripsi dengan PGP sebelum disimpan, sehingga meskipun backup dicuri, data tetap aman.
- Contoh: Organisasi menyimpan cadangan data klien di server eksternal yang dienkripsi dengan PGP untuk mencegah kebocoran data.
## Perlindungan Identitas dan Anonimitas
- Kegunaan: Melindungi identitas pengirim dan penerima dalam komunikasi online.
- Cara Kerja: Penggunaan enkripsi end-to-end menjamin bahwa hanya pihak yang berwenang yang dapat membaca pesan.
- Contoh: Aktivis atau peneliti yang bekerja di negara dengan pengawasan ketat dapat menggunakan PGP untuk melindungi komunikasi mereka.
## Memverifikasi Integritas Perangkat Lunak
- Kegunaan: Memastikan bahwa perangkat lunak atau paket yang diunduh berasal dari sumber yang terpercaya dan tidak dimodifikasi oleh pihak ketiga.
- Cara Kerja: Pengembang menandatangani perangkat lunak menggunakan kunci privat mereka, dan pengguna dapat memverifikasi tanda tangan menggunakan kunci publik yang disediakan.
- Contoh: Distribusi Linux atau aplikasi open-source sering kali menyertakan tanda tangan PGP untuk memverifikasi keasliannya.
## Komunikasi di Forum atau Jaringan Terdistribusi
- Kegunaan: Memastikan privasi komunikasi di platform terdesentralisasi atau anonim.
- Cara Kerja: Pesan dienkripsi sebelum dikirim dan hanya dapat didekripsi oleh penerima yang sah.
- Contoh: Pengguna di forum yang membahas topik sensitif dapat menggunakan PGP untuk melindungi identitas mereka.
---
## Mengapa PGP Penting?
- Keamanan yang Kuat: Kombinasi kriptografi asimetris dan simetris membuat PGP sangat sulit ditembus oleh peretas.
-Privasi: Menjaga komunikasi Anda tetap aman dan pribadi dari penyadapan atau pengawasan.
- Integritas: Memastikan bahwa pesan atau data yang dikirim tidak diubah selama transmisi.
- Otentikasi: Membuktikan identitas pengirim melalui tanda tangan digital.
---
## Kelebihan PGP
- Sangat Aman: Jika digunakan dengan benar, PGP menawarkan tingkat keamanan yang sangat tinggi.
- Terbuka: Banyak digunakan di kalangan profesional dan komunitas open-source.
- Fleksibel: Dapat digunakan untuk berbagai keperluan, mulai dari komunikasi email hingga penyimpanan data.
## Kekurangan PGP
- Kompleks untuk Pemula: Relatif sulit digunakan oleh pengguna awam.
- Pengelolaan Kunci yang Rumit: Memerlukan pengelolaan kunci publik/privat yang benar.
- Tidak Ada Pemulihan Data: Jika kunci privat hilang, data yang dienkripsi tidak dapat dipulihkan.
---
PGP adalah alat yang sangat berguna untuk memastikan keamanan dan privasi dalam komunikasi dan data. Dengan meningkatnya risiko kejahatan siber, penggunaan PGP menjadi semakin penting, terutama di sektor yang menangani data sensitif.
-

@ bcea2b98:7ccef3c9
2024-11-14 23:38:37
@chess a3
originally posted at https://stacker.news/items/768303
-

@ dbb19ae0:c3f22d5a
2024-11-14 20:39:37
Hello,
As I've registered with Alby a few months ago I told a friend (who complained about the current toxicity of twitter/X) about this new social media Nostr and told him he could get rewarded for posting.
The deal was that I would help him to create his nostr account and get a wallet, https://guides.getalby.com/user-guide/alby-account-and-browser-extension
downloading the extension, creating the nostr account was a breeze, and then ....
As I wanted to send him a few sats to get started
that is when the whole thing went down.
Alby is now forcing its users to get a hub (and a channel), this was not my experience when I started back in March 2024 and I was surprised of this new direction Alby is taking.
Opening a channel costs ~25K sat -- and that was not expected, for a brand new user
Now my friend thinks that this whole new social media and bitcoin stuff is all a big scam.
What can I do?
Is there a way to open a wallet (compatible with Nostr) elsewhere?
originally posted at https://stacker.news/items/768090
-

@ bcea2b98:7ccef3c9
2024-11-14 18:03:14
We all have that one movie or show we turn to when we need a little comfort. Whether it’s for the laughs, the nostalgia, or the familiar characters, these are the ones that never get old.
What’s a movie or series you can watch again and again? What makes it so comforting, and why do you keep coming back to it?
I enjoy The Office with Michael Scott and Dwight Schrute. We watched a lot of that in the past and then again during the pandemic during isolation.
originally posted at https://stacker.news/items/767870
-

@ e968e50b:db2a803a
2024-11-14 17:03:38
<sub>TLDR: No, but if you own bitcoin and can afford to, you should get one anyway.</sub>
# Secure the network.
First off, I'm not mining to heat my house, and I'm not mining to earn bitcoin. I'm not mining out of the goodness of my heart either. I'm mining because I store my family's savings in bitcoin, and mining centralization looks like a real threat to me. I've written about it [here](https://stacker.news/items/629837) and [here](https://stacker.news/items/735779) if you're interested in reading more about why this is so important for average bitcoiners. That being said, I'd like to earn (or at least not lose) bitcoin in the process, and I'd also love to heat my home with it.
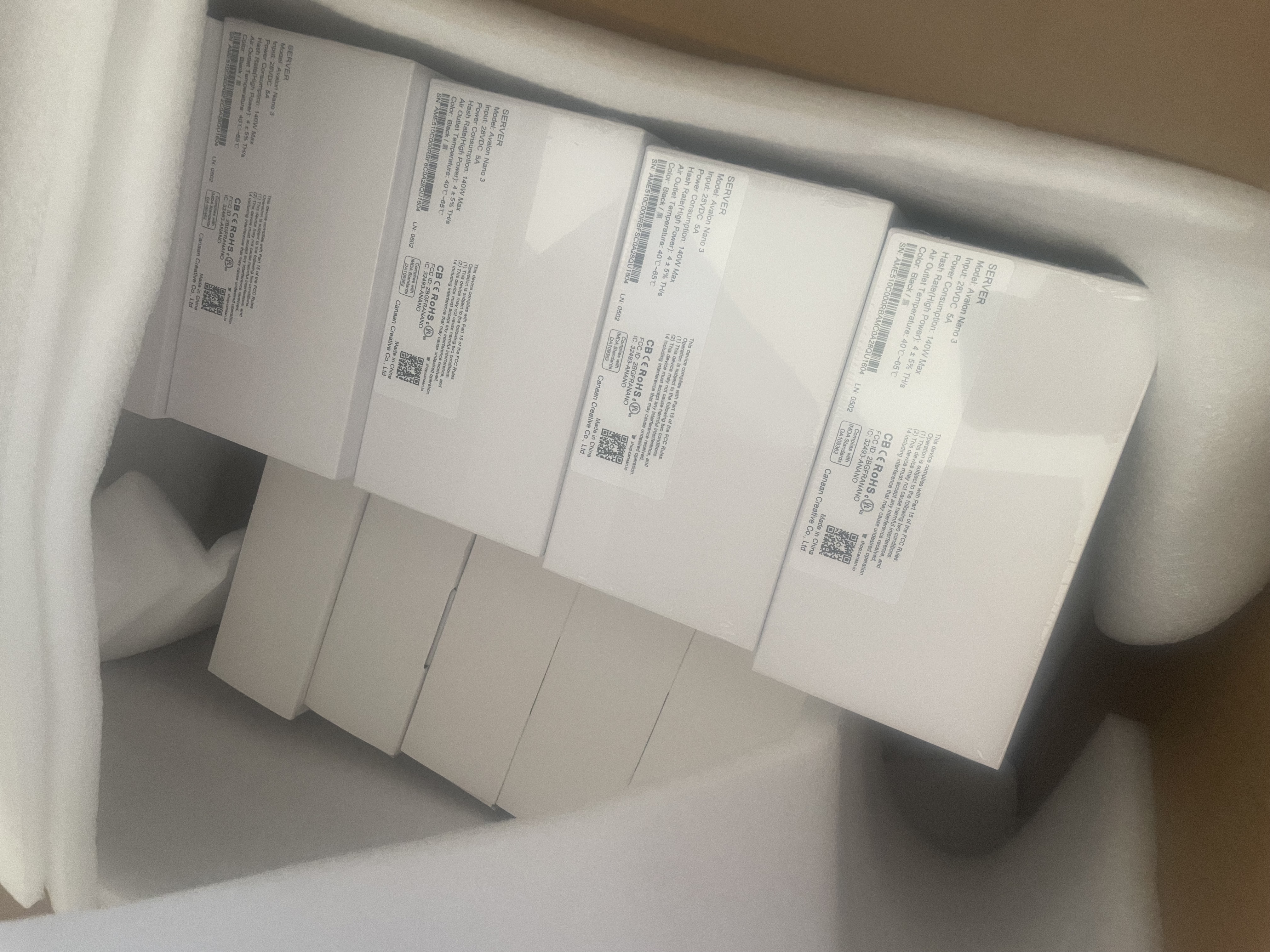
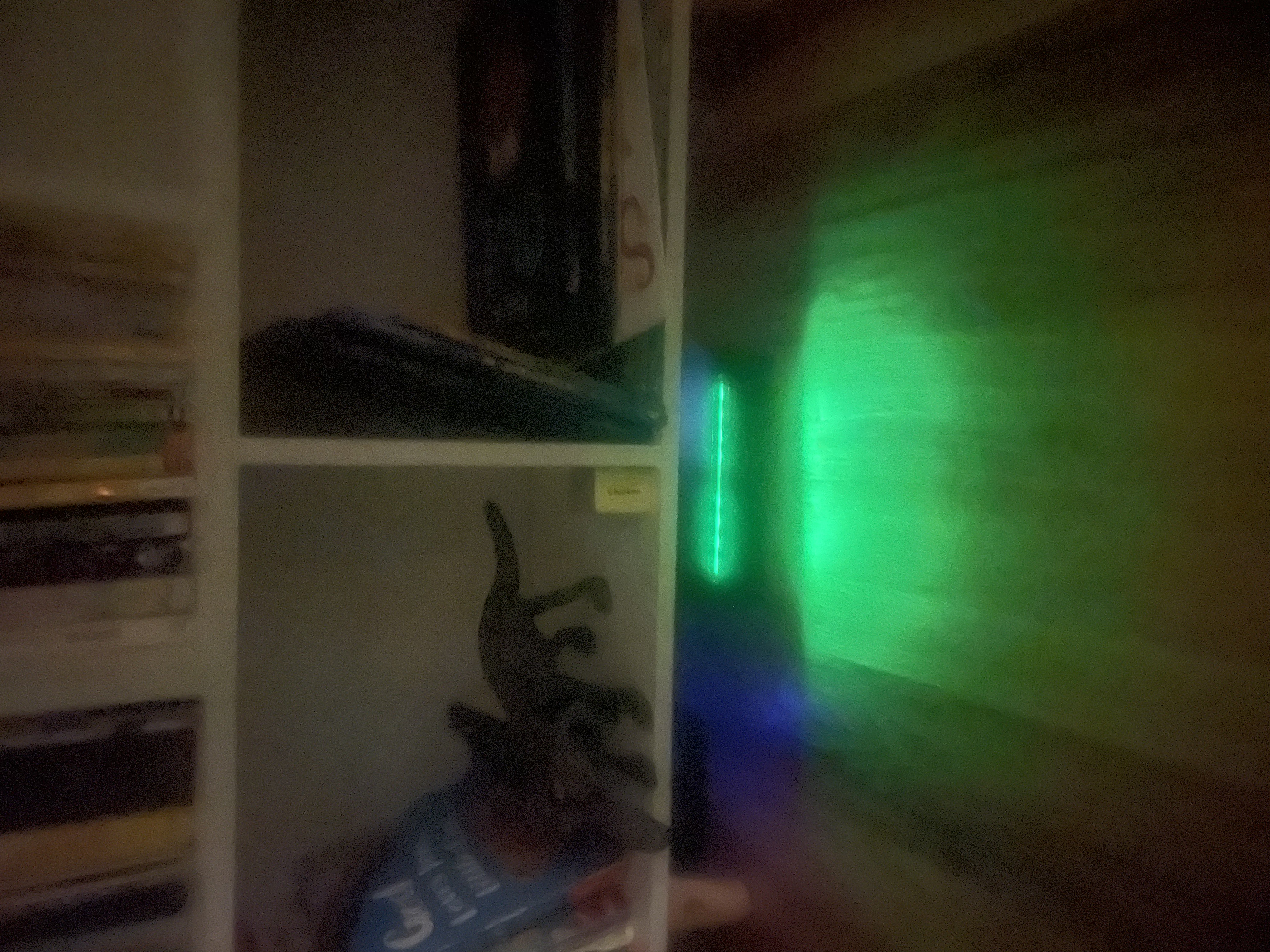
# Nano 3
In one of the aforementioned posts, I made a stink about how little hash one contributes with a lottery miner and @OT called me out on ignoring hardware centralization concerns with Bitmain. Well, Canaan isn't exactly a small company, and they're not open source, but they aren't Bitmain, and I found a miner that's about eight times as powerful as the virtual signaling[^1] solo miners at about the same cost.
My big find was the Nano 3. It's actually marketed as a space heater with three heating settings. At it's warmest, this guy mines at 4TH/s and uses about 140 watts. So it's about as efficient as a midrange s19, hashes about the a third as much as an s9, and costs as much as a Bitaxe. As a tool for decentralization, I'd say that's a great combo.
Ironically, most of the folks I see talking about it online are shitcoiners that use it to solo mine Bitcoin Cash. I wonder if this phenomenon is culturally turning bitcoiners off to a better decentralizing tool.
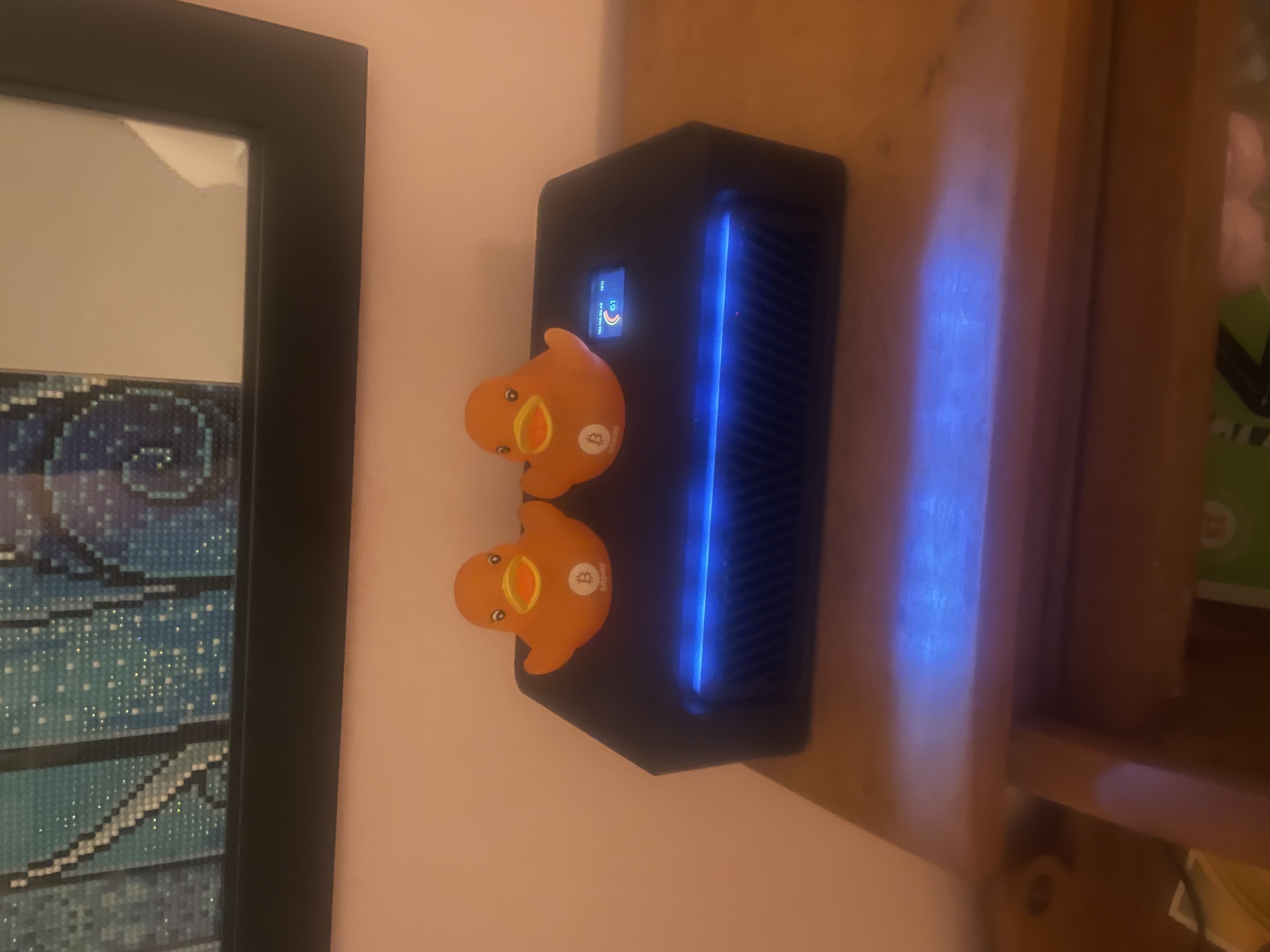
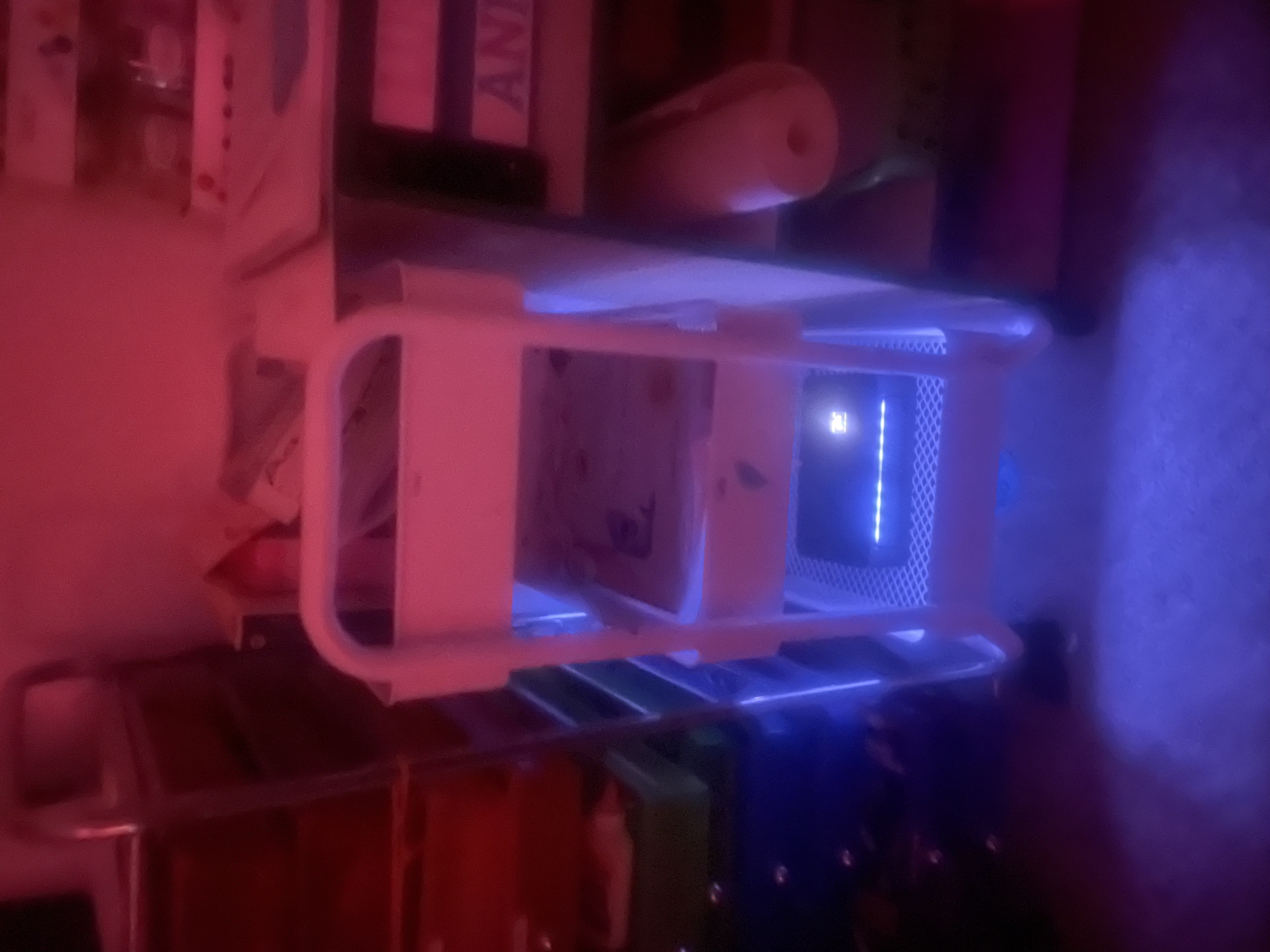
# Recycled Heat
So, now that I have them, I can tell you from personal experience how they're doing as heaters. Well, they are pushing heat out. However, it's a very small fraction of what my s9s do. They look better in house[^2], and they're significantly quieter (but not silent), but there's no way these guys are going to let me go furnace free this winter like a similar amount[^3] of Bitmain products would do.
#Conclusion
So I don't see the Nano 3 as the silver bullet for home miners. I do, however, think it's a great option and am not at all bummed with my purchase. At my current electricity price, I'll pay them off (even denominated in bitcoin) at some point. But I'm also going back to the drawing board about trying to replace my furnace with miners.
[^1]: totally unfair characterization... I get it. Just trying to make a point with some hyperbole
[^2]: The lighting is also pretty cool. You can make it pulse or strobe, and the full color spectrum is available. They're keep my kids' rooms a LITTLE bit warmer this winter and double as a cool night lite that teaches them about bitcoin. The ones in living areas do the same for guests. I consider these non-trivial features.
[^3]: Similar price too! Most s9s online are about the same price as the Nano 3s.
originally posted at https://stacker.news/items/767748
-

@ 5d4b6c8d:8a1c1ee3
2024-11-14 15:30:36
So, I had been maintaining a position of not muting anyone on Stacker News. In part, that's because I don't want SN to become an echo chamber like so much of the rest of social media. I also don't want to risk missing out on something valuable that someone has to say, just because I don't like them.
As I hope is clear to everyone here, and my record is fairly abundant, I'll engage on just about any topic with just about anyone. I've had many civil disagreements with folks (as well as some uncivil ones): sometimes I change my mind, sometimes I don't, but I do always listen to what the other person said and try to understand where they're coming from. I don't argue to win (shifting the goal posts when the other person makes a good point or simply ignoring it), but rather to learn.
High-level civil disagreements are one of the things that sets Stacker News apart from the rest of the internet.
What finally got someone muted was not their opinion (which I do disagree with), but their conduct. We had gone through the same tedious argument several times and the person never engaged with my points, but just kept repeating their assertions. When they attempted to start the same argument again recently, I told them I wasn't interested in rehashing it with them and they could go reread our past exchanges to see what I think. Rather than respect my wishes, the person belligerently insisted on pressing their argument. When I set my terms for continuing the conversation (read our past exchanges and bring up something new), they resorted to name calling. Mute.
As much as I enjoy talking about stuff and having disagreements, I don't owe anyone my time or attention. If someone tells me that they're sick of talking to me about something, I will drop it (or I will at least acknowledge that I should have). I expect the same courtesy from others. Have some respect for the person you're interacting with, if you want them to interact with you.
Have whatever opinion you want, but argue it in good faith and be respectful.
originally posted at https://stacker.news/items/767536
-

@ 7b8a7a4b:910f3874
2024-11-14 10:05:23
123 test test
originally posted at https://stacker.news/items/459399
-

@ 4ba8e86d:89d32de4
2024-11-14 09:17:14
Tutorial feito por nostr:nostr:npub1rc56x0ek0dd303eph523g3chm0wmrs5wdk6vs0ehd0m5fn8t7y4sqra3tk poste original abaixo:
Parte 1 : http://xh6liiypqffzwnu5734ucwps37tn2g6npthvugz3gdoqpikujju525yd.onion/263585/tutorial-debloat-de-celulares-android-via-adb-parte-1
Parte 2 : http://xh6liiypqffzwnu5734ucwps37tn2g6npthvugz3gdoqpikujju525yd.onion/index.php/263586/tutorial-debloat-de-celulares-android-via-adb-parte-2
Quando o assunto é privacidade em celulares, uma das medidas comumente mencionadas é a remoção de bloatwares do dispositivo, também chamado de debloat. O meio mais eficiente para isso sem dúvidas é a troca de sistema operacional. Custom Rom’s como LineageOS, GrapheneOS, Iodé, CalyxOS, etc, já são bastante enxutos nesse quesito, principalmente quanto não é instalado os G-Apps com o sistema. No entanto, essa prática pode acabar resultando em problemas indesejados como a perca de funções do dispositivo, e até mesmo incompatibilidade com apps bancários, tornando este método mais atrativo para quem possui mais de um dispositivo e separando um apenas para privacidade.
Pensando nisso, pessoas que possuem apenas um único dispositivo móvel, que são necessitadas desses apps ou funções, mas, ao mesmo tempo, tem essa visão em prol da privacidade, buscam por um meio-termo entre manter a Stock rom, e não ter seus dados coletados por esses bloatwares. Felizmente, a remoção de bloatwares é possível e pode ser realizada via root, ou mais da maneira que este artigo irá tratar, via adb.
## O que são bloatwares?
Bloatware é a junção das palavras bloat (inchar) + software (programa), ou seja, um bloatware é basicamente um programa inútil ou facilmente substituível — colocado em seu dispositivo previamente pela fabricante e operadora — que está no seu dispositivo apenas ocupando espaço de armazenamento, consumindo memória RAM e pior, coletando seus dados e enviando para servidores externos, além de serem mais pontos de vulnerabilidades.
## O que é o adb?
O Android Debug Brigde, ou apenas adb, é uma ferramenta que se utiliza das permissões de usuário shell e permite o envio de comandos vindo de um computador para um dispositivo Android exigindo apenas que a depuração USB esteja ativa, mas também pode ser usada diretamente no celular a partir do Android 11, com o uso do Termux e a depuração sem fio (ou depuração wifi). A ferramenta funciona normalmente em dispositivos sem root, e também funciona caso o celular esteja em Recovery Mode.
Requisitos:
Para computadores:
• Depuração USB ativa no celular;
• Computador com adb;
• Cabo USB;
Para celulares:
• Depuração sem fio (ou depuração wifi) ativa no celular;
• Termux;
• Android 11 ou superior;
Para ambos:
• Firewall NetGuard instalado e configurado no celular;
• Lista de bloatwares para seu dispositivo;
## Ativação de depuração:
Para ativar a Depuração USB em seu dispositivo, pesquise como ativar as opções de desenvolvedor de seu dispositivo, e lá ative a depuração. No caso da depuração sem fio, sua ativação irá ser necessária apenas no momento que for conectar o dispositivo ao Termux.
## Instalação e configuração do NetGuard
O NetGuard pode ser instalado através da própria Google Play Store, mas de preferência instale pela F-Droid ou Github para evitar telemetria.
F-Droid:
https://f-droid.org/packages/eu.faircode.netguard/
Github: https://github.com/M66B/NetGuard/releases
Após instalado, configure da seguinte maneira:
Configurações → padrões (lista branca/negra) → ative as 3 primeiras opções (bloquear wifi, bloquear dados móveis e aplicar regras ‘quando tela estiver ligada’);
Configurações → opções avançadas → ative as duas primeiras (administrar aplicativos do sistema e registrar acesso a internet);
Com isso, todos os apps estarão sendo bloqueados de acessar a internet, seja por wifi ou dados móveis, e na página principal do app basta permitir o acesso a rede para os apps que você vai usar (se necessário). Permita que o app rode em segundo plano sem restrição da otimização de bateria, assim quando o celular ligar, ele já estará ativo.
## Lista de bloatwares
Nem todos os bloatwares são genéricos, haverá bloatwares diferentes conforme a marca, modelo, versão do Android, e até mesmo região.
Para obter uma lista de bloatwares de seu dispositivo, caso seu aparelho já possua um tempo de existência, você encontrará listas prontas facilmente apenas pesquisando por elas. Supondo que temos um Samsung Galaxy Note 10 Plus em mãos, basta pesquisar em seu motor de busca por:
```
Samsung Galaxy Note 10 Plus bloatware list
```
Provavelmente essas listas já terão inclusas todos os bloatwares das mais diversas regiões, lhe poupando o trabalho de buscar por alguma lista mais específica.
Caso seu aparelho seja muito recente, e/ou não encontre uma lista pronta de bloatwares, devo dizer que você acaba de pegar em merda, pois é chato para um caralho pesquisar por cada aplicação para saber sua função, se é essencial para o sistema ou se é facilmente substituível.
### De antemão já aviso, que mais para frente, caso vossa gostosura remova um desses aplicativos que era essencial para o sistema sem saber, vai acabar resultando na perda de alguma função importante, ou pior, ao reiniciar o aparelho o sistema pode estar quebrado, lhe obrigando a seguir com uma formatação, e repetir todo o processo novamente.
## Download do adb em computadores
Para usar a ferramenta do adb em computadores, basta baixar o pacote chamado SDK platform-tools, disponível através deste link: https://developer.android.com/tools/releases/platform-tools. Por ele, você consegue o download para Windows, Mac e Linux.
Uma vez baixado, basta extrair o arquivo zipado, contendo dentro dele uma pasta chamada platform-tools que basta ser aberta no terminal para se usar o adb.
## Download do adb em celulares com Termux.
Para usar a ferramenta do adb diretamente no celular, antes temos que baixar o app Termux, que é um emulador de terminal linux, e já possui o adb em seu repositório. Você encontra o app na Google Play Store, mas novamente recomendo baixar pela F-Droid ou diretamente no Github do projeto.
F-Droid: https://f-droid.org/en/packages/com.termux/
Github: https://github.com/termux/termux-app/releases
## Processo de debloat
Antes de iniciarmos, é importante deixar claro que não é para você sair removendo todos os bloatwares de cara sem mais nem menos, afinal alguns deles precisam antes ser substituídos, podem ser essenciais para você para alguma atividade ou função, ou até mesmo são insubstituíveis.
Alguns exemplos de bloatwares que a substituição é necessária antes da remoção, é o Launcher, afinal, é a interface gráfica do sistema, e o teclado, que sem ele só é possível digitar com teclado externo. O Launcher e teclado podem ser substituídos por quaisquer outros, minha recomendação pessoal é por aqueles que respeitam sua privacidade, como Pie Launcher e Simple Laucher, enquanto o teclado pelo OpenBoard e FlorisBoard, todos open-source e disponíveis da F-Droid.
Identifique entre a lista de bloatwares, quais você gosta, precisa ou prefere não substituir, de maneira alguma você é obrigado a remover todos os bloatwares possíveis, modifique seu sistema a seu bel-prazer. O NetGuard lista todos os apps do celular com o nome do pacote, com isso você pode filtrar bem qual deles não remover.
Um exemplo claro de bloatware insubstituível e, portanto, não pode ser removido, é o com.android.mtp, um protocolo onde sua função é auxiliar a comunicação do dispositivo com um computador via USB, mas por algum motivo, tem acesso a rede e se comunica frequentemente com servidores externos. Para esses casos, e melhor solução mesmo é bloquear o acesso a rede desses bloatwares com o NetGuard.
MTP tentando comunicação com servidores externos:
## Executando o adb shell
No computador
Faça backup de todos os seus arquivos importantes para algum armazenamento externo, e formate seu celular com o hard reset. Após a formatação, e a ativação da depuração USB, conecte seu aparelho e o pc com o auxílio de um cabo USB. Muito provavelmente seu dispositivo irá apenas começar a carregar, por isso permita a transferência de dados, para que o computador consiga se comunicar normalmente com o celular.
Já no pc, abra a pasta platform-tools dentro do terminal, e execute o seguinte comando:
```
./adb start-server
```
O resultado deve ser:
*daemon not running; starting now at tcp:5037
*daemon started successfully
E caso não apareça nada, execute:
```
./adb kill-server
```
E inicie novamente.
Com o adb conectado ao celular, execute:
```
./adb shell
```
Para poder executar comandos diretamente para o dispositivo. No meu caso, meu celular é um Redmi Note 8 Pro, codinome Begonia.
Logo o resultado deve ser:
begonia:/ $
Caso ocorra algum erro do tipo:
adb: device unauthorized.
This adb server’s $ADB_VENDOR_KEYS is not set
Try ‘adb kill-server’ if that seems wrong.
Otherwise check for a confirmation dialog on your device.
Verifique no celular se apareceu alguma confirmação para autorizar a depuração USB, caso sim, autorize e tente novamente. Caso não apareça nada, execute o kill-server e repita o processo.
## No celular
Após realizar o mesmo processo de backup e hard reset citado anteriormente, instale o Termux e, com ele iniciado, execute o comando:
```
pkg install android-tools
```
Quando surgir a mensagem “Do you want to continue? [Y/n]”, basta dar enter novamente que já aceita e finaliza a instalação
Agora, vá até as opções de desenvolvedor, e ative a depuração sem fio. Dentro das opções da depuração sem fio, terá uma opção de emparelhamento do dispositivo com um código, que irá informar para você um código em emparelhamento, com um endereço IP e porta, que será usado para a conexão com o Termux.
Para facilitar o processo, recomendo que abra tanto as configurações quanto o Termux ao mesmo tempo, e divida a tela com os dois app’s, como da maneira a seguir:
Para parear o Termux com o dispositivo, não é necessário digitar o ip informado, basta trocar por “localhost”, já a porta e o código de emparelhamento, deve ser digitado exatamente como informado. Execute:
```
adb pair localhost:porta CódigoDeEmparelhamento
```
De acordo com a imagem mostrada anteriormente, o comando ficaria “adb pair localhost:41255 757495”.
Com o dispositivo emparelhado com o Termux, agora basta conectar para conseguir executar os comandos, para isso execute:
```
adb connect localhost:porta
```
Obs: a porta que você deve informar neste comando não é a mesma informada com o código de emparelhamento, e sim a informada na tela principal da depuração sem fio.
Pronto! Termux e adb conectado com sucesso ao dispositivo, agora basta executar normalmente o adb shell:
```
adb shell
```
Remoção na prática
Com o adb shell executado, você está pronto para remover os bloatwares. No meu caso, irei mostrar apenas a remoção de um app (Google Maps), já que o comando é o mesmo para qualquer outro, mudando apenas o nome do pacote.
Dentro do NetGuard, verificando as informações do Google Maps:
Podemos ver que mesmo fora de uso, e com a localização do dispositivo desativado, o app está tentando loucamente se comunicar com servidores externos, e informar sabe-se lá que peste. Mas sem novidades até aqui, o mais importante é que podemos ver que o nome do pacote do Google Maps é com.google.android.apps.maps, e para o remover do celular, basta executar:
```
pm uninstall –user 0 com.google.android.apps.maps
```
E pronto, bloatware removido! Agora basta repetir o processo para o resto dos bloatwares, trocando apenas o nome do pacote.
Para acelerar o processo, você pode já criar uma lista do bloco de notas com os comandos, e quando colar no terminal, irá executar um atrás do outro.
Exemplo de lista:
Caso a donzela tenha removido alguma coisa sem querer, também é possível recuperar o pacote com o comando:
```
cmd package install-existing nome.do.pacote
```
## Pós-debloat
Após limpar o máximo possível o seu sistema, reinicie o aparelho, caso entre no como recovery e não seja possível dar reboot, significa que você removeu algum app “essencial” para o sistema, e terá que formatar o aparelho e repetir toda a remoção novamente, desta vez removendo poucos bloatwares de uma vez, e reiniciando o aparelho até descobrir qual deles não pode ser removido. Sim, dá trabalho… quem mandou querer privacidade?
Caso o aparelho reinicie normalmente após a remoção, parabéns, agora basta usar seu celular como bem entender! Mantenha o NetGuard sempre executando e os bloatwares que não foram possíveis remover não irão se comunicar com servidores externos, passe a usar apps open source da F-Droid e instale outros apps através da Aurora Store ao invés da Google Play Store.
Referências:
Caso você seja um Australopithecus e tenha achado este guia difícil, eis uma videoaula (3:14:40) do Anderson do canal Ciberdef, realizando todo o processo: http://odysee.com/@zai:5/Como-remover-at%C3%A9-200-APLICATIVOS-que-colocam-a-sua-PRIVACIDADE-E-SEGURAN%C3%87A-em-risco.:4?lid=6d50f40314eee7e2f218536d9e5d300290931d23
Pdf’s do Anderson citados na videoaula: créditos ao anon6837264
http://eternalcbrzpicytj4zyguygpmkjlkddxob7tptlr25cdipe5svyqoqd.onion/file/3863a834d29285d397b73a4af6fb1bbe67c888d72d30/t-05e63192d02ffd.pdf
Processo de instalação do Termux e adb no celular: https://youtu.be/APolZrPHSms
-

@ b4403b24:83542d4e
2024-11-14 00:55:14
Donald Trump officially announced his nomination of Tucker Carlson for a White House Press Secretary. 🫶
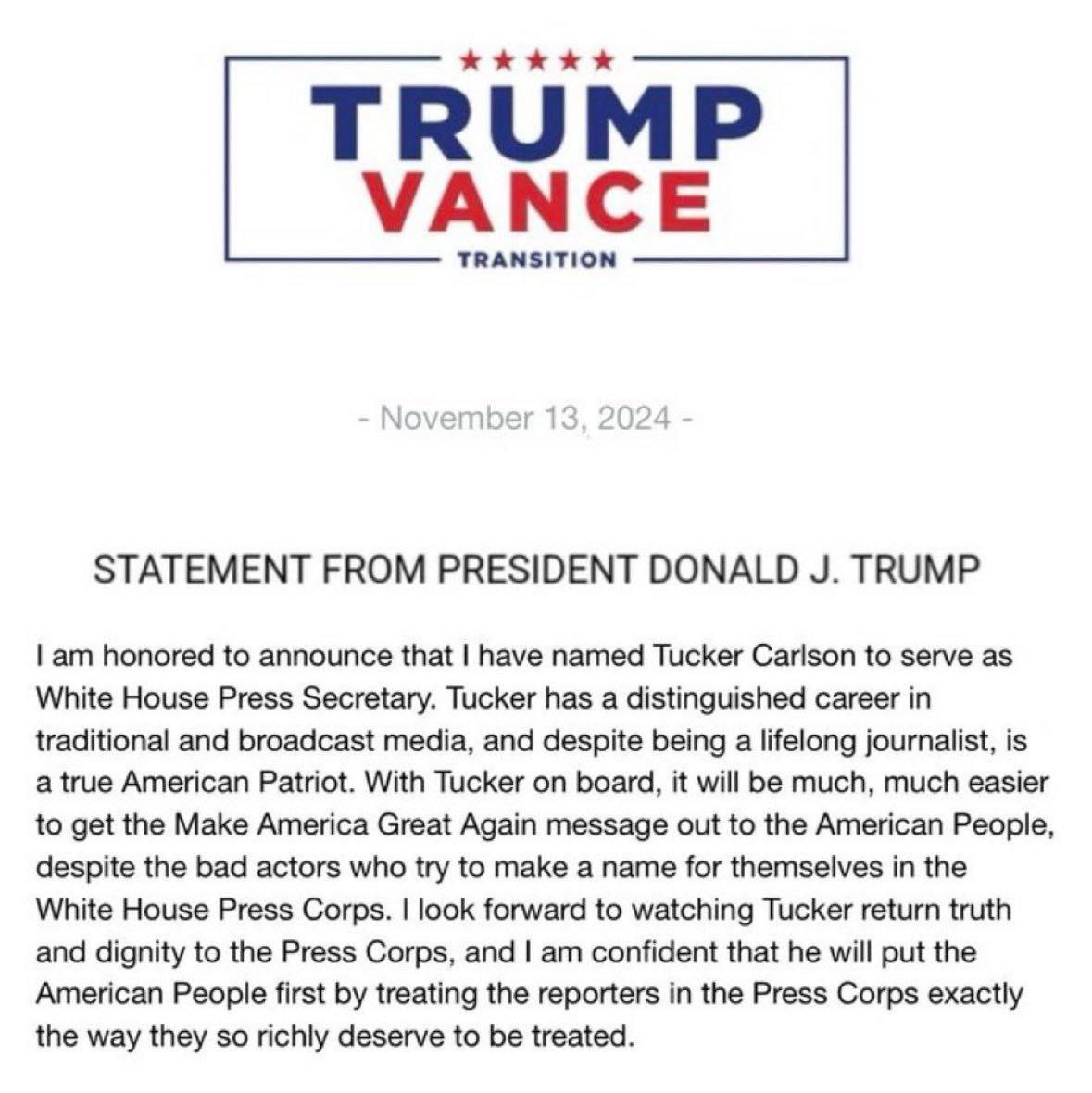
originally posted at https://stacker.news/items/766758
-

@ bcea2b98:7ccef3c9
2024-11-14 00:42:08
Sometimes we come across values that resonate deeply only after going through certain experiences. These values may not have been obvious to us earlier, but now they play a meaningful role in how we approach life.
Is there a value you’ve discovered later in life that’s become important to you?
originally posted at https://stacker.news/items/766754
-

@ b4403b24:83542d4e
2024-11-13 21:00:29
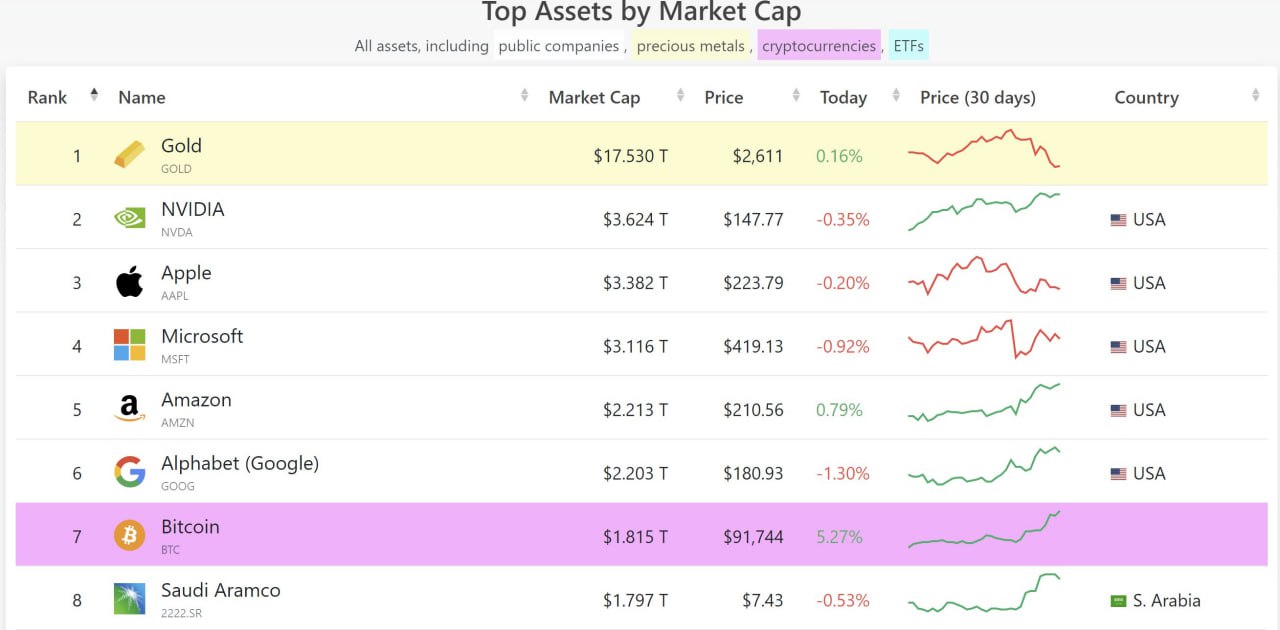
originally posted at https://stacker.news/items/766497
-

@ 000002de:c05780a7
2024-11-13 19:46:44
Here's the official list.
[A-Z index of U.S. government departments and agencies | USAGov](https://www.usa.gov/agency-index).
My answer: Department of Education
Why?
* Its completely unnecessary. Every state has its own.
* It is a mechanism for centralizing thought.
* It wields great influence over schools and states with its massive budget
* If you want to change the future of the nation in a positive direction stop allowing progressives manipulate the minds of the youth.
* Decentralizing to at least the state level puts more power back into the states vs. a single agency that is largely unaccountable.
What do you think?
originally posted at https://stacker.news/items/766383
-

@ 6f170f27:711e26dd
2024-11-13 19:17:52
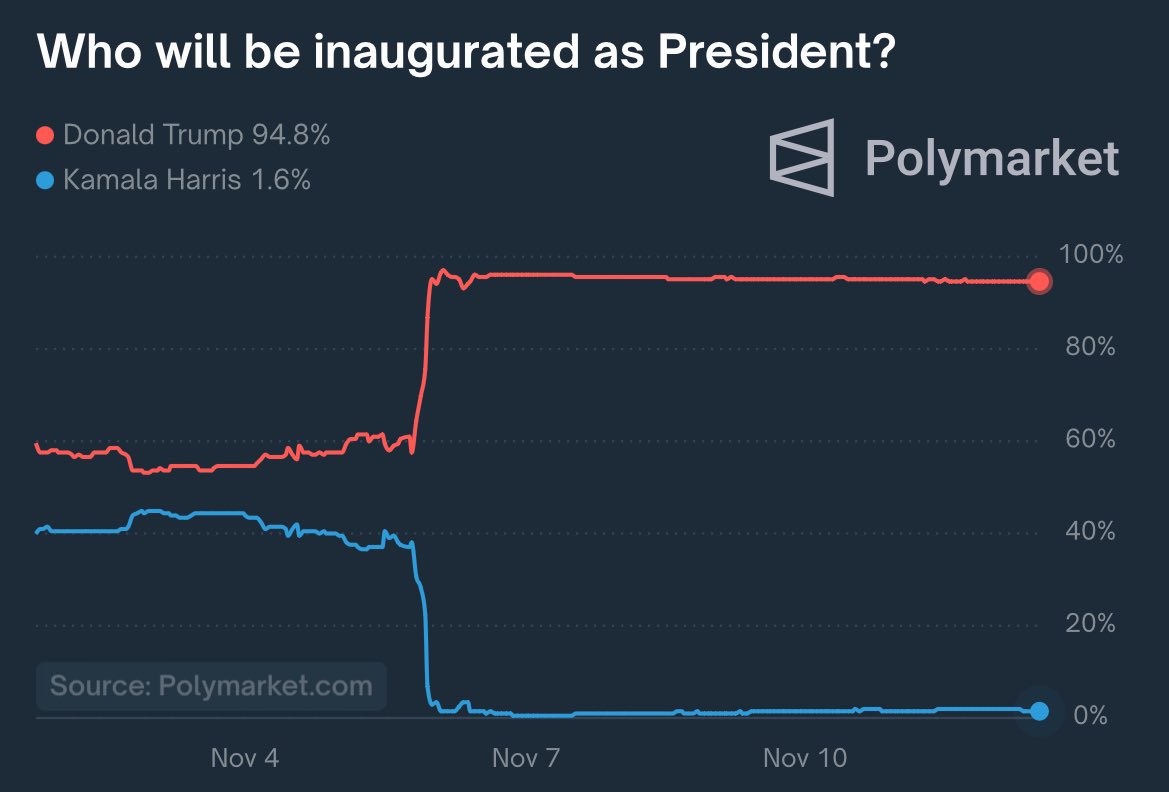
originally posted at https://stacker.news/items/766349