-

@ dbb19ae0:c3f22d5a
2025-02-21 20:38:43
``` python
#!/usr/bin/env python3
import asyncio
from nostr_sdk import Metadata, Client, NostrSigner, Keys, Filter, PublicKey, Kind, init_logger, LogLevel
from datetime import timedelta
async def main():
init_logger(LogLevel.INFO)
secret_key = "nsec1........Replace with your actual nsec secret key"
keys = Keys.parse(secret_key)
signer = NostrSigner.keys(keys)
client = Client(signer)
await client.add_relay("wss://relay.damus.io")
await client.connect()
# Update metadata
new_metadata = Metadata().set_name( "MyName")\
.set_nip05("MyName@example.com")\
.set_lud16("MyName@lud16.com")
await client.set_metadata(new_metadata)
print("Metadata updated successfully.")
# Get updated metadata
npub = "npub1....Replace with your actual npub"
pk = PublicKey.parse(npub)
print(f"\nGetting profile metadata for {npub}:")
metadata = await client.fetch_metadata(pk, timedelta(seconds=15))
print(metadata)
if __name__ == '__main__':
asyncio.run(main())
```
-

@ 4c96d763:80c3ee30
2025-02-21 20:31:55
# Changes
## Ethan Tuttle (1):
- feat: ctrl+enter when creating a new note, sends the note, the same way clicking the "Post Now" button.
## Ken Sedgwick (11):
- drive-by compiler warning fixes
- drive-by clippy fixes
- add derive Debug to some things
- panic on unknown CLI arguments
- move RelayDebugView to notedeck crate and restore --relay-debug
- add diagnostic string to DecodeFailed
- improve relay message parsing unit tests
- fix event error return when decoding fails
- fix OK message parser to include last message component
- fix EOSE parsing to handle extra whitespace
- check message length before prefix comparisons
## William Casarin (5):
- clippy: fix enum too large issue
- changelog: add unreleased section
- clippy: fix lint
- args: skip creation of vec
- nix: fix android build
## jglad (4):
- fix: handle missing file [#715]
- refactor
- fix compilation
- hide nsec in account panel
pushed to [notedeck:refs/heads/master](http://git.jb55.com/notedeck/commit/32c7f83bd7a807155de6a13b66a7d43f48c25e13.html)
-

@ 266815e0:6cd408a5
2025-02-21 17:54:15
I've been working on the applesauce libraries for a while now but I think this release is the first one I would consider to be stable enough to use
A lot of the core concepts and classes are in place and stable enough where they wont change too much next release
If you want to skip straight to the documentation you can find at [hzrd149.github.io/applesauce](https://hzrd149.github.io/applesauce/) or the typescript docs at [hzrd149.github.io/applesauce/typedoc](https://hzrd149.github.io/applesauce/typedoc)
## Whats new
### Accounts
The `applesauce-accounts` package is an extension of the `applesauce-signers` package and provides classes for building a multi-account system for clients
Its primary features are
- Serialize and deserialize accounts so they can be saved in local storage or IndexededDB
- Account manager for multiple accounts and switching between them
- Account metadata for things like labels, app settings, etc
- Support for NIP-46 Nostr connect accounts
see [documentation](https://hzrd149.github.io/applesauce/accounts/manager.html) for more examples
### Nostr connect signer
The `NostrConnectSigner` class from the `applesauce-signers` package is now in a stable state and has a few new features
- Ability to create `nostrconnect://` URIs and waiting for the remote signer to connect
- SDK agnostic way of subscribing and publishing to relays
For a simple example, here is how to create a signer from a `bunker://` URI
```js
const signer = await NostrConnectSigner.fromBunkerURI(
"bunker://266815e0c9210dfa324c6cba3573b14bee49da4209a9456f9484e5106cd408a5?relay=wss://relay.nsec.app&secret=d9aa70",
{
permissions: NostrConnectSigner.buildSigningPermissions([0, 1, 3, 10002]),
async onSubOpen(filters, relays, onEvent) {
// manually open REQ
},
async onSubClose() {
// close previouse REQ
},
async onPublishEvent(event, relays) {
// Pubilsh an event to relays
},
},
);
```
see [documentation](https://hzrd149.github.io/applesauce/signers/nostr-connect.html) for more examples and other signers
### Event Factory
The `EventFactory` class is probably what I'm most proud of. its a standalone class that can be used to create various types of events from templates ([blueprints](https://hzrd149.github.io/applesauce/typedoc/modules/applesauce_factory.Blueprints.html)) and is really simple to use
For example:
```js
import { EventFactory } from "applesauce-factory";
import { NoteBlueprint } from "applesauce-factory/blueprints";
const factory = new EventFactory({
// optionally pass a NIP-07 signer in to use for encryption / decryption
signer: window.nostr
});
// Create a kind 1 note with a hashtag
let draft = await factory.create(NoteBlueprint, "hello world #grownostr");
// Sign the note so it can be published
let signed = await window.nostr.signEvent(draft);
```
Its included in the `applesauce-factory` package and can be used with any other nostr SDKs or vanilla javascript
It also can be used to modify existing replaceable events
```js
let draft = await factory.modifyTags(
// kind 10002 event
mailboxes,
// add outbox relays
addOutboxRelay("wss://relay.io/"),
addOutboxRelay("wss://nostr.wine/"),
// remove inbox relay
removeInboxRelay("wss://personal.old-relay.com/")
);
```
see [documentation](https://hzrd149.github.io/applesauce/overview/factory.html) for more examples
### Loaders
The `applesauce-loaders` package exports a bunch of loader classes that can be used to load everything from replaceable events (profiles) to timelines and NIP-05 identities
They use [rx-nostr](https://penpenpng.github.io/rx-nostr/) under the hood to subscribe to relays, so for the time being they will not work with other nostr SDKs
I don't expect many other developers or apps to use them since in my experience every nostr client requires a slightly different way or loading events
*They are stable enough to start using but they are not fully tested and they might change slightly in the future*
The following is a short list of the loaders and what they can be used for
- `ReplaceableLoader` loads any replaceable events (0, 3, 1xxxx, 3xxxx)
- `SingleEventLoader` loads single events based on ids
- `TimelineLoader` loads a timeline of events from multiple relays based on filters
- `TagValueLoader` loads events based on a tag name (like "e") and a value, can be used to load replies, zaps, reactions, etc
- `DnsIdentityLoader` loads NIP-05 identities and supports caching
- `UserSetsLoader` loads all lists events for users
see [documentation](https://hzrd149.github.io/applesauce/overview/loaders.html) for more examples
### Real tests
For all new features and a lot of existing ones I'm trying to write tests to ensure I don't leave unexpected bugs for later
I'm not going to pretend its 100% tests coverage or that it will ever get close to that point, but these tests cover some of the core classes and help me prove that my code is doing what it says its supposed to do
At the moment there are about 230 tests covering 45 files. not much but its a start
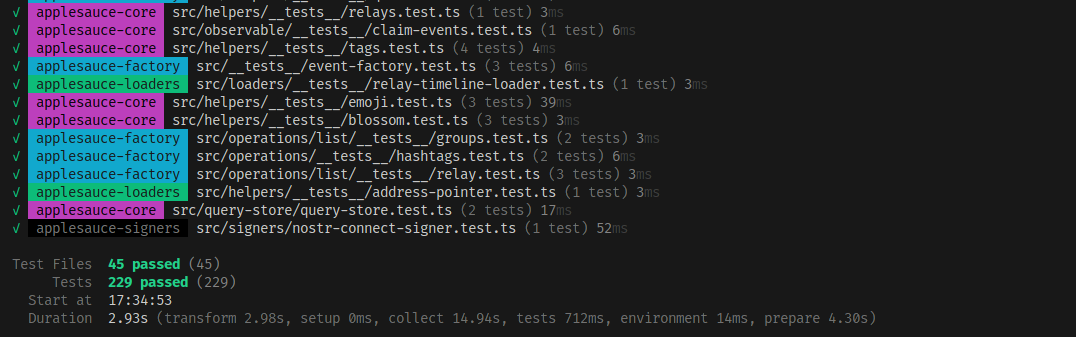
## Apps built using applesauce
If you want to see some examples of applesauce being used in a nostr client I've been testing a lot of this code in production on the apps I've built in the last few months
- [noStrudel](https://github.com/hzrd149/nostrudel) The main app everything is being built for and tested in
- [nsite-manager](https://github.com/hzrd149/nsite-manager) Still a work-in-progress but supports multiple accounts thanks to the `applesauce-accounts` package
- [blossomservers.com](https://github.com/hzrd149/blossomservers) A simple (and incomplete) nostr client for listing and reviewing public blossom servers
- [libretranslate-dvm](https://github.com/hzrd149/libretranslate-dvm) A libretranslate DVM for nostr:npub1mkvkflncllnvp3adq57klw3wge6k9llqa4r60g42ysp4yyultx6sykjgnu
- [cherry-tree](https://github.com/hzrd149/cherry-tree) A chunked blob uploader / downloader. only uses applesauce for boilerplate
- [nsite-homepage](https://github.com/hzrd149/nsite-homepage) A simple landing page for [nsite.lol](https://nsite.lol)
Thanks to nostr:npub1cesrkrcuelkxyhvupzm48e8hwn4005w0ya5jyvf9kh75mfegqx0q4kt37c for teaching me more about rxjs and consequentially making me re-write a lot of the core observables to be faster
-

@ dbb19ae0:c3f22d5a
2025-02-21 17:46:58
Tested and working with nostr_sdk version 0.39
``` python
from nostr_sdk import Metadata, Client, Keys, Filter, PublicKey
from datetime import timedelta
import argparse
import asyncio
import json
async def main(npub):
client = Client()
await client.add_relay("wss://relay.damus.io")
await client.connect()
pk = PublicKey.parse(npub)
print(f"\nGetting profile metadata for {npub}:")
metadata = await client.fetch_metadata(pk, timedelta(seconds=15))
# Printing each field of the Metadata object
print(f"Name: {metadata.get_name()}")
print(f"Display Name: {metadata.get_display_name()}")
print(f"About: {metadata.get_about()}")
print(f"Website: {metadata.get_website()}")
print(f"Picture: {metadata.get_picture()}")
print(f"Banner: {metadata.get_banner()}")
print(f"NIP05: {metadata.get_nip05()}")
print(f"LUD06: {metadata.get_lud06()}")
print(f"LUD16: {metadata.get_lud16()}")
#print(f"Custom: {metadata.get_custom()}")
if __name__ == '__main__':
parser = argparse.ArgumentParser(description='Fetch all metadata for a given npub')
parser.add_argument('npub', type=str, help='The npub of the user')
args = parser.parse_args()
asyncio.run(main(args.npub))
```
-

@ 378562cd:a6fc6773
2025-02-21 16:55:39
Hiking in nature is more than just a leisurely activity—it’s a powerful way to boost physical health, mental well-being, and overall quality of life. Whether you’re trekking through lush forests, climbing rugged mountains, or strolling along a peaceful riverbank (which we really love doing), the benefits of hiking are undeniable.
Here are some points to think about along these lines.
#### **Physical Health Benefits**
- Strengthens muscles and improves cardiovascular health
- Enhances balance, coordination, and endurance
- Supports bone density and joint health
- Burns calories and aids in weight management
#### **Mental and Emotional Well-Being**
- Reduces stress, anxiety, and depression
- Increases endorphin levels, boosting mood and relaxation
- Improves focus, creativity, and cognitive function
- Encourages mindfulness and mental clarity
#### **Connection with Nature**
- Provides an escape from screens and digital distractions
- Encourages appreciation for the environment and wildlife
- Helps regulate sleep cycles by syncing with natural light
- Instills a sense of peace and awe
#### **Social and Personal Growth**
- Strengthens relationships when hiking with friends or family
- Encourages teamwork and problem-solving on group hikes
- Builds confidence and resilience for solo hikers
- Creates lasting memories and a sense of accomplishment
### **A Typical Day on the Trail**
A day of hiking often starts with an early morning wake-up, packing essentials like water, snacks, and a map (if you're old-fashioned like me, LOL). After reaching the trailhead, the journey begins with fresh air, birds singing, and the crunch of dirt underfoot. You might stop to admire a stunning viewpoint, take photos of wildflowers, or rest beside a babbling stream. Lunch could be a simple picnic with a breathtaking backdrop. As the afternoon sun filters through the trees, you finish the hike feeling refreshed, accomplished, and ready to do it all again.
We love to go hiking, and I'm no expert, but when I take the time to get us out there somewhere and the weather is wonderful, it just adds up to an amazing day!
Whether you're a beginner or an experienced hiker, exploring nature on foot provides countless benefits for both body and mind. It’s an accessible, enjoyable, and fulfilling way to stay healthy while reconnecting with the natural world. So, lace up your hiking boots, hit the trails, and experience the transformative power of hiking in nature!
-

@ d34e832d:383f78d0
2025-02-21 16:00:08
[npub16d8gxt2z4k9e8sdpc0yyqzf5gp0np09ls4lnn630qzxzvwpl0rgq5h4rzv]
Data Storage via Blobs in a Decentralized Manner
Blobs (Binary Large Objects) offer a flexible method of storing large chunks of data, and in the context of decentralized systems, they allow for secure, distributed storage solutions. In a decentralized world, where privacy and autonomy are key, managing data in a distributed manner ensures data isn't controlled or censored by a single entity. Here are three key systems enabling decentralized blob storage:
1. **Blossom Server**
[Blossom Server](https://github.com/hzrd149/blossom-server?tab=readme-ov-file) provides a decentralized platform for storing and sharing large blobs of data. Blossom Server allows users to host their own data and retrieve it from a decentralized network, ensuring that data is not stored in centralized servers. This platform is open-source, offering flexibility and security through peer-to-peer data storage.
2. **Perkeep**
[Perkeep](https://perkeep.org/) (formerly known as Camlistore) is a decentralized data storage system that allows for storing blobs of data in a distributed manner. It focuses on the long-term storage of large data sets, such as personal collections of photos, videos, and documents. By using Perkeep, users can ensure that their data remains private and is not controlled by any central authority. The system uses a unique identifier to access data, promoting both privacy and integrity.
3. **IPFS (InterPlanetary File System)**
[IPFS](https://ipfs.io/) is another popular decentralized file storage system that uses the concept of blobs to store and share data. IPFS allows users to store and access data in a decentralized manner by using a peer-to-peer network. Each piece of data is given a unique hash, ensuring that it is verifiable and tamper-proof. By leveraging IPFS, users can store everything from simple files to large applications, all without relying on centralized servers.
By using these decentralized data storage solutions, individuals and organizations can safeguard their information, increase privacy, and contribute to a more resilient and distributed internet infrastructure.
**Higher-Level Goals for Blob Storage**
Blob storage via Blossom ,Perkeep and IPFS has goals to become a decentralized, self-sufficient protocol for data storage, management, and sharing. While some of these features are already being implemented, they represent a broader vision for the future of decentralized personal data management.
1. **Filesystem Backups**
Allows for easy, incremental backups. Using the **`pk-put`** tool, users can back up files and directories quickly and efficiently. Incremental backups, which save only the changes made since the last backup, are essentially free, making Perkeep an efficient choice for backup solutions. This initial use case has already been implemented, providing seamless and secure backups for personal data.
2. **Efficient Remote Filesystem**
The goal is to create a highly efficient, aggressively caching remote filesystem using Perkeep. A **read-only** version of this filesystem is already trivial to implement, while **read-write** functionality remains an area of active development. Every modification in the filesystem would be snapshotted implicitly, providing version control as a default. This would enable users to interact with their data in a remote environment while ensuring that every change is tracked and recoverable.
3. **Decentralized Sharing System**
Perkeep is working towards enabling users to share data in a decentralized manner. The system will allow individuals to share anything with anyone or everyone, with privacy being the default setting. This decentralized sharing is already starting to work, and users can now share data with others while retaining control over who sees their information.
4. **Blog / Photo Hosting / Document Management CMS**
Perkeep aims to replace traditional blog platforms, photo hosting services, and document management software. By running a **personal blog**, **photo gallery**, and **document management system (CMS)** on Perkeep, users will have full control over their content. Permissions will be configurable, allowing for personal or public sharing. The author intends to use Perkeep for his own blog, gallery, and document management needs, further demonstrating its versatility.
5. **Decentralized Social Networking**
While still a lofty goal, decentralized social networking is a persistent aim for Perkeep. By implementing features like **comments** and **tagging**, users could attach metadata to images and content. Through claims, users could sign data and verify identities, promoting trust in social interactions. This would allow for decentralized social networking where users control their own data and interactions.
6. **Import/Export Adapters for Hosted Web Services**
Perkeep intends to bridge the gap between decentralized storage and traditional hosted web services. This feature would allow users to **mirror data** between hosted services and their private Perkeep storage. Whether content is created in Perkeep or hosted services, the goal is to ensure that data is always backed up privately, ensuring users' data is theirs forever.
### Combined Goals for Blossom and IPFS
Both Blossom and IPFS share common goals of decentralizing data storage, enhancing privacy, and providing users with greater control over their data. Together, these technologies enable:
- **Self-Sovereign Data Management**: Empowering users to store and manage their data without relying on centralized platforms.
- **Resilient and Redundant Storage**: Offering decentralized and redundant data storage that ensures availability and security.
- **Private and Permissioned Sharing**: Enabling secure, private data sharing where the user controls who has access to their content.
By focusing on these goals, both Blossom and IPFS are contributing to a future where individuals control their own data, collaborate more efficiently in decentralized networks and P4P protocols, and ensure the privacy and security of their digital assets.
These technologies in conjunction with nostr lead one to discover user agency and autonomy, where you can actually own and interface with your own data allowing for value creation and content creation strategies.
Donations via
- lightninglayerhash@getalby.com
-

@ d34e832d:383f78d0
2025-02-21 15:32:49
Decentralized Publishing: ChainScribe: How to Approach Studying NIPs (Nostr Improvement Proposals)
[npub16d8gxt2z4k9e8sdpc0yyqzf5gp0np09ls4lnn630qzxzvwpl0rgq5h4rzv]
How to Approach Studying NIPs (Nostr Improvement Proposals)
NIPs (Nostr Improvement Proposals) provide a framework for suggesting and discussing improvements to the Nostr protocol, a decentralized network for open communication. Studying NIPs is crucial for understanding the evolution of Nostr and its underlying principles. To effectively approach this, it's essential to follow a systematic, structured process.
1. **Familiarize with the Nostr Protocol**: Before diving into the specifics of each NIP, gain a solid understanding of the core Nostr protocol. This includes its goals, architecture, and key components like pubkeys, events, and relays.
2. **Explore the NIP Catalog**: Visit [nostr-nips.com](https://nostr-nips.com/) to browse through the available NIPs. Focus on the most recent proposals and those that align with your interests or areas of expertise.
3. **Review the Proposal Structure**: Each NIP follows a standard structure, typically including a description of the problem, proposed solution, and rationale. Learn to read and evaluate these elements critically, paying attention to how each proposal aligns with Nostr’s decentralized ethos.
4. **Follow Active Discussions**: Many NIPs are actively discussed within the community. Follow relevant channels, such as GitHub issues or dedicated discussion forums, to understand community feedback and potential revisions.
5. **Understand Dependencies**: Some NIPs are designed to work in tandem with others or require other technological advancements. Recognize these relationships to better understand the broader implications of any proposal.
6. **Hands-On Testing**: If possible, test NIPs in a development environment to see how they function in practice. Experimenting with proposals will help deepen your understanding and expose potential challenges or flaws.
7. **Contribute to Proposals**: If you have insights or suggestions, contribute to the discussion or propose your own improvements. NIPs thrive on community participation, and your input can help shape the future of Nostr.
Donations via
- lightninglayerhash@getalby.com
-

@ 5d4b6c8d:8a1c1ee3
2025-02-21 14:41:00
Howdy stackers!
Since football season is finally over, we're going to dive into NFL offseason topics.
- There was a huge spike in the salary cap, but lot's of well known players are still going to be cap casualties
- Which free agents are we hoping our teams re-sign and which others should they go after?
The NBA All-Star Game was a huge dud and Wemby has a scary medical condition
- Can the All Star game be fixed?
- What are Wemby's prospects for the future?
- Also, Luka's fat. Were the Mavs right to trade him?
The NHL did manage to fix their All Star event. Is this a winning formula or a one-off?
Degenerate Corner: How are our bets doing?
And, whatever else the stackers want us to talk about.
originally posted at https://stacker.news/items/893005
-

@ a012dc82:6458a70d
2025-02-21 14:20:23
In 2021, El Salvador embarked on an unprecedented financial experiment under President Nayib Bukele's leadership by adopting Bitcoin as legal tender. This decision was not only groundbreaking but also positioned the small Central American nation as a pioneer in the global financial landscape. The move was seen as a step towards modernizing the country's financial system and making it more inclusive and forward-thinking. It was a daring venture into uncharted territory, signaling El Salvador's willingness to embrace technological innovation and challenge traditional economic models. The decision was met with a mix of admiration and skepticism globally, as it represented a significant departure from conventional monetary policy and raised questions about the future of digital currencies in mainstream finance.
**Table of Contents**
- The Genesis of the Bitcoin Law
- Implementation and Challenges
- Economic Impacts and International Response
- Bukele's Vision and Controversies
- The Future of Bitcoin in El Salvador
- Conclusion
- FAQs
**The Genesis of the Bitcoin Law**
The journey to adopting Bitcoin as legal tender was marked by the introduction of the Bitcoin Law, a bold legislative move by President Bukele's administration. This law required all businesses to accept Bitcoin in addition to the US dollar, which had been El Salvador's official currency for two decades. The law aimed to democratize financial access, especially for the 70% of Salvadorans who did not have bank accounts and relied heavily on cash transactions. It also sought to reduce remittance costs for millions of Salvadorans living abroad, who annually send billions of dollars back home. The Bitcoin Law was a part of Bukele's broader strategy to reposition El Salvador as a hub for technological innovation and economic growth. However, the law also raised significant questions about the feasibility and stability of using a highly volatile digital currency in everyday transactions.
**Implementation and Challenges**
Implementing the Bitcoin Law was a complex endeavor. The government's launch of the “Chivo” digital wallet was intended to facilitate Bitcoin transactions and offer a $30 Bitcoin incentive to users. However, the rollout faced technical glitches and public mistrust in cryptocurrency. Many Salvadorans were unfamiliar with Bitcoin, leading to a steep learning curve and reluctance to transition from traditional cash transactions. Additionally, the fluctuating value of Bitcoin posed a risk to users, particularly those living in poverty or on fixed incomes. The government's efforts to promote Bitcoin adoption also faced international scrutiny, with financial experts questioning the impact on El Salvador's financial stability and its relationship with global financial institutions.
**Economic Impacts and International Response**
The economic impact of Bitcoin's adoption in El Salvador has been a subject of intense debate. On one hand, it has attracted significant attention from the global cryptocurrency community, positioning the country as a potential hub for digital finance. On the other hand, the move has been met with caution and criticism from international financial institutions. The International Monetary Fund (IMF), for instance, expressed concern over the potential risks associated with Bitcoin's volatility and its implications for financial stability, governance, and consumer protection. The fluctuating value of Bitcoin also posed a challenge for the national budget and financial planning. Despite these concerns, the Bukele administration remained committed to its digital currency strategy, viewing it as a long-term investment in the country's economic future.
**Bukele's Vision and Controversies**
President Nayib Bukele, a charismatic and controversial leader, has been at the center of El Salvador's Bitcoin experiment. His administration's aggressive push for Bitcoin adoption was part of a broader vision to transform El Salvador into a modern, technologically advanced economy. Bukele's plans included the development of a “Bitcoin City,” funded by Bitcoin-backed bonds, which was envisioned as a tax-free haven for cryptocurrency investors. However, these ambitious projects have not been without controversy. Critics have raised concerns about the lack of transparency in the government's Bitcoin transactions and the potential for financial mismanagement. Bukele's autocratic tendencies, including his clashes with the judiciary and the press, have also raised alarms about the democratic governance of these initiatives.
**The Future of Bitcoin in El Salvador**
As El Salvador continues its journey with Bitcoin, the future of this initiative remains a topic of global interest and speculation. The country is navigating uncharted waters, and its experience will likely serve as a case study for other nations contemplating similar moves. The success of Bitcoin in El Salvador depends on various factors, including technological infrastructure, public education, and global market dynamics. The government's ability to manage the currency's volatility and integrate it into the broader economy will be crucial. Additionally, the impact of this initiative on financial inclusion, remittances, and economic growth will be closely watched. El Salvador's experiment with Bitcoin could pave the way for a new era of digital currency adoption, but it also faces significant challenges that could shape its outcome.
**Conclusion**
El Salvador's adoption of Bitcoin as legal tender under President Bukele's leadership marks a significant moment in the history of digital currency. This bold move has positioned the country as a trailblazer in the cryptocurrency world, but the long-term outcomes of this bold experiment remain uncertain. As El Salvador navigates the challenges and opportunities presented by this initiative, its journey will undoubtedly offer crucial insights into the future intersection of technology, finance, and governance. The world is watching as El Salvador tests the limits of cryptocurrency integration, and its experiences will likely influence global financial policies and the adoption of digital currencies in the years to come. Whether El Salvador's gamble on Bitcoin will pay off or serve as a cautionary tale remains to be seen, but its impact on the global financial landscape is undeniable.
**FAQs**
**What is the Bitcoin Law in El Salvador?**
The Bitcoin Law in El Salvador is a legislation that made Bitcoin a legal tender alongside the US dollar. It mandates all businesses to accept Bitcoin for transactions and was introduced to promote financial inclusion and modernize the economy.
**Why did El Salvador adopt Bitcoin as legal tender?**
El Salvador adopted Bitcoin to increase financial accessibility for its unbanked population, reduce remittance costs, and position itself as a leader in digital currency innovation.
**How does the Chivo wallet work?**
The Chivo wallet is a government-backed digital wallet that facilitates Bitcoin transactions in El Salvador. Users receive a $30 Bitcoin bonus for signing up, aiming to encourage the adoption of Bitcoin.
**What are the challenges faced by El Salvador in adopting Bitcoin?**
Challenges include technical issues with the Chivo wallet, public mistrust and lack of understanding of Bitcoin, and the currency's volatility impacting economic stability.
**How has the international community responded to El Salvador's Bitcoin adoption?**
The international response has been mixed, with some praising the innovative approach, while institutions like the IMF have expressed concerns over financial stability and consumer protection.
**What is Bitcoin City and how is it funded?**
Bitcoin City is a proposed development in El Salvador, envisioned as a tax-free zone for cryptocurrency investors. It is planned to be funded by Bitcoin-backed bonds.
**That's all for today**
**If you want more, be sure to follow us on:**
**NOSTR: croxroad@getalby.com**
**X: [@croxroadnews.co](https://x.com/croxroadnewsco)**
**Instagram: [@croxroadnews.co](https://www.instagram.com/croxroadnews.co/)**
**Youtube: [@croxroadnews](https://www.youtube.com/@croxroadnews)**
**Store: https://croxroad.store**
**Subscribe to CROX ROAD Bitcoin Only Daily Newsletter**
**https://www.croxroad.co/subscribe**
***DISCLAIMER: None of this is financial advice. This newsletter is strictly educational and is not investment advice or a solicitation to buy or sell any assets or to make any financial decisions. Please be careful and do your own research.***
-

@ c43d6de3:a6583169
2025-02-20 23:54:51
## "So, What’s the News?"
Were it not for these very words, we might never have had a modern capitalistic society.
Between 1500 and 1800, coffeehouses were more than just places to grab a quick hit of caffeine—they were the beating heart of local communities. These spaces buzzed with debate, discussion, and the exchange of groundbreaking ideas. Strangers became intellectual sparring partners, not merely reading the news but dissecting and debating it in real-time.
One could easily imagine Adam Smith engaged in animated conversation with David Hume in a coffeehouse on Edinburgh’s Royal Mile—the former outlining the invisible hand of the market, the latter countering that human behavior is driven more by emotion than reason. Such debates weren’t confined to elite scholars. Merchants, craftsmen, and common laborers sat shoulder to shoulder, engaging in discussions that transcended social class.
But then came industrialization.
The rise of factory life replaced the organic rhythms of community engagement with rigid work schedules. As cities expanded and people became busier, the once-thriving coffeehouses—where time was slow, conversation was deep, and debate was central—began to empty. The demand for efficiency left little room for leisurely discussions, and over time, the public square of ideas withered.
-----------
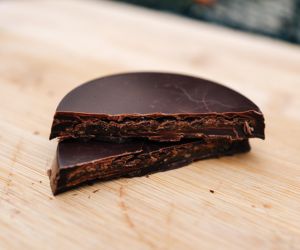
nostr:npub1uzt238htjzpq39dxmltlx60vxym9fetk9czz6kddq6fhvkf4z3usy9qtrh
Mouth watering Hodl rounds- Bitcoin only
https://shopstr.store/listing/84f20c5e1ef94762b60f0d5fb4398594ace6c5589212f94269e9542fbe351732
-----------
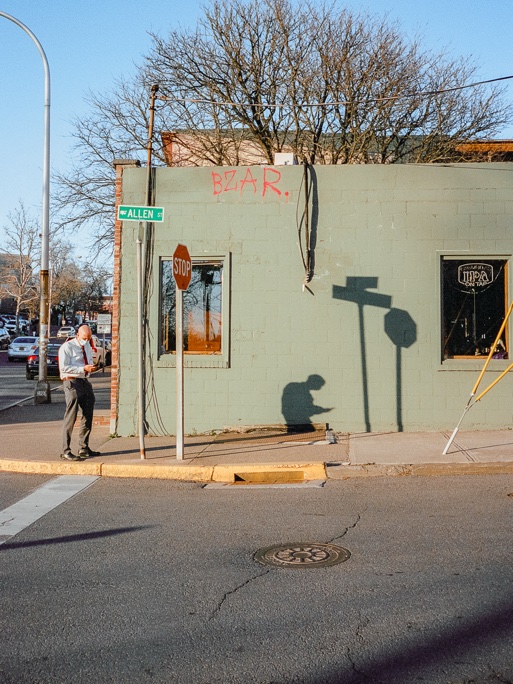
> Photos Courtesy of nostr:npub1c8n9qhqzm2x3kzjm84kmdcvm96ezmn257r5xxphv3gsnjq4nz4lqelne96 Give him a follow and some zap love if you like his art
## Empty Street Corners and Hollow Venues
Adam Smith would lose his mind with how interconnected the world is today.
Yet, he might also notice something unsettling: while we are globally connected, our local communities feel more fragmented than ever before.
He’d have a hell of an online presence but he, just like many do today, might get the sense that something isn't quite right. The world is quieter<a href="https://yakihonne.com/naddr1qvzqqqr4gupzp3padh3au336rew4pzfx78s050p3dw7pmhurgr2ktdcwwxn9svtfqq2kjjzzfpjxvutjg33hjvpcw5cyjezyv9y5k0umm6k">than ever before</a> while online discourse teeters on the verge of violent physical manifestations.
It’s not that public discourse has disappeared. Rather, it has been exiled to the internet. Social media has become our new coffeehouse, but instead of robust face-to-face engagement, we navigate a landscape of curated algorithms, viral outrage, and fragmented conversations. The once-public square of ideas has transformed into isolated echo chambers, where avatars shape narratives that must be adhered to lest one be accused of inconsistency and lose credibility.
The result? People live layered lives with layered personas—some even attaining secret fame in online communities while remaining anonymous in their own neighborhoods. Meanwhile, the street corners remain empty, and the venues that once thrived with the fervor of intellectual thought stand hollow.
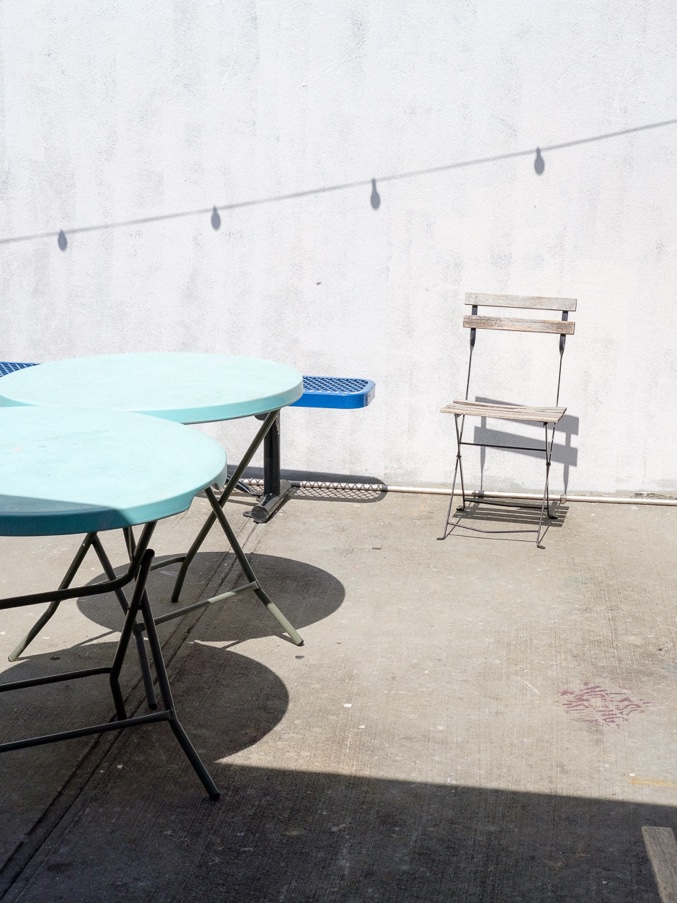
> Photos Courtesy of nostr:npub1c8n9qhqzm2x3kzjm84kmdcvm96ezmn257r5xxphv3gsnjq4nz4lqelne96 Give him a follow and some zap love if you like his art
## The Need for a Modern Coffeehouse
Historically, coffeehouses were revolutionary because they democratized knowledge. They provided an open forum for ideas, allowing philosophy, politics, and commerce to intermingle freely. Unlike monasteries or universities, coffeehouses welcomed anyone who could afford a cup of coffee.
With the rise of digital discourse, have we lost something essential? The depth, accountability, and personal engagement that once defined public debate have been replaced by reactionary commentary and fleeting viral moments. The question is no longer where we discuss ideas—it’s how we engage with them.
We need to ask ourselves: Can we reclaim a physical space for intellectual exchange? Or has the age of meaningful, face-to-face discourse faded, vanishing with the steam of yesterday’s coffee?
Can We Bring Back the Local Forum? Should We Care?
Before coffeehouses, philosophy lived in elite institutions and royal courts. With the rise of coffee culture, it became truly public, shaping modern democracy, scientific thought, and social progress. The internet, for all its wonders, lacks the tactile, immediate, and personal engagement that once made intellectual debate so powerful.
The challenge ahead is not just about nostalgia for coffeehouse debates—it’s about asking whether we can create new spaces, physical or digital, that foster real intellectual exchange. Without them, we risk losing something fundamental: the ability to challenge, refine, and reshape our ideas through genuine human connection. But most of all, we risk the possibility great ideas come but no action is ever taken to make them real in this real world.
So, what’s the news?
-----------
Thank you for reading!
If this article resonated with you, let me know with a zap and share it with friends who might find it insightful.
All zap revenue from this article is shared with nostr:npub1c8n9qhqzm2x3kzjm84kmdcvm96ezmn257r5xxphv3gsnjq4nz4lqelne96 for his photograph contributions.
Your help sends a strong signal for content creators like us to keep making cool shit like this!
Interested in fiction? Follow nostr:npub1j9cmpzhlzeex6y85c2pnt45r5zhxhtx73a2twt77fyjwequ4l4jsp5xd49 for great short stories and serialized fiction.
More photography and articles you might like from nostr:npub1cs7kmc77gcapuh2s3yn0rc868sckh0qam7p5p4t9ku88rfjcx95sq8tqw9 and nostr:npub1c8n9qhqzm2x3kzjm84kmdcvm96ezmn257r5xxphv3gsnjq4nz4lqelne96 :
nostr:naddr1qvzqqqr4gupzps0x2pwq9k5drv99k0tdkmsekt4j9hx4fu8gvvrwez3p8yptx9t7qqxnqvfjxqer2tfh0fekz7r5l270gu
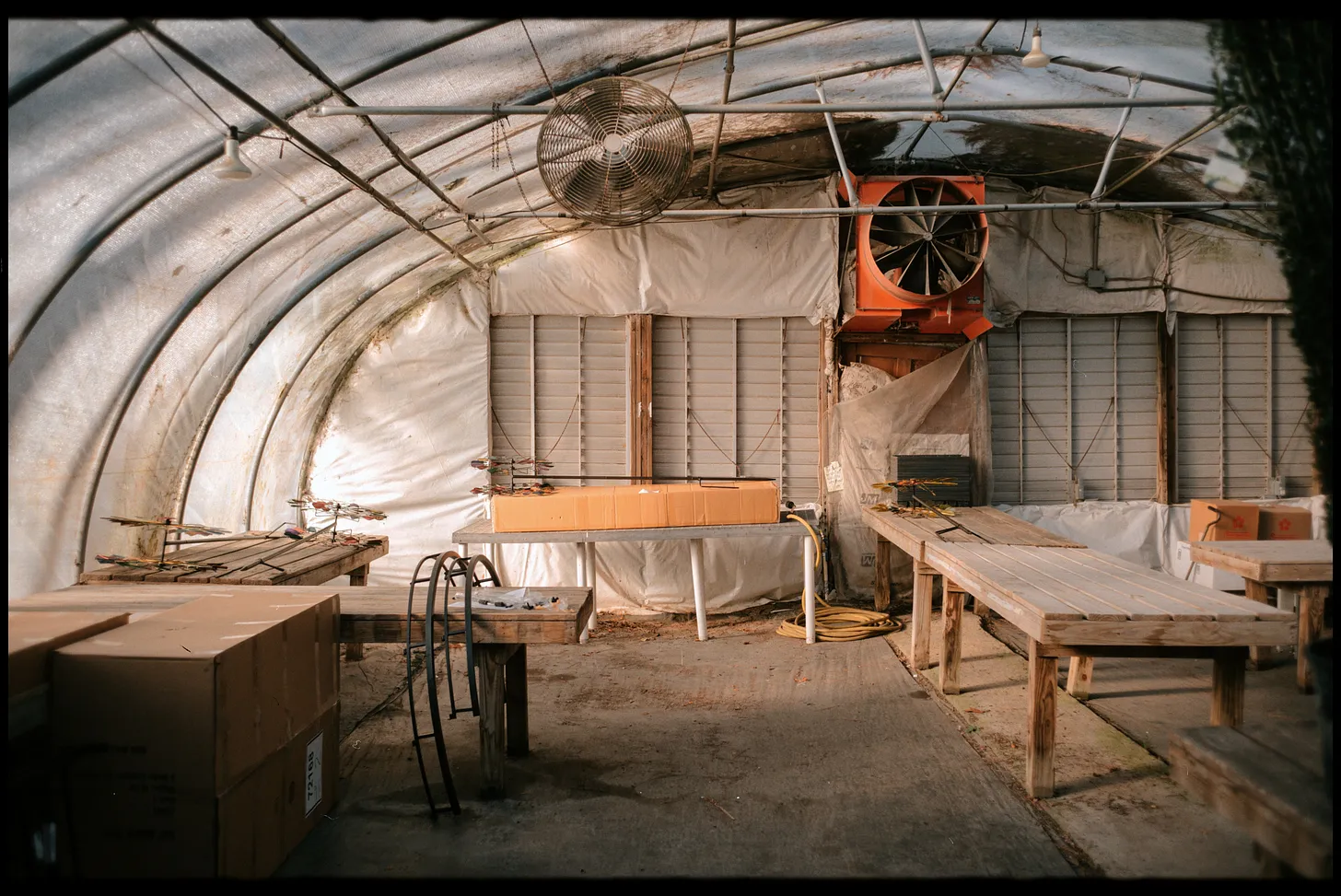
https://pictureroom.substack.com/p/020825
nostr:naddr1qvzqqqr4gupzp3padh3au336rew4pzfx78s050p3dw7pmhurgr2ktdcwwxn9svtfqq24s4mrfdm55utg23z55ufs94argvn523k5yqhkrc3
nostr:naddr1qvzqqqr4gupzp3padh3au336rew4pzfx78s050p3dw7pmhurgr2ktdcwwxn9svtfqq2nvnr5d4nngw2zgd5k5c6etftrzez9fd9kkcl0pzp
-

@ a2eddb26:e2868a80
2025-02-20 20:28:46
In personal finance, the principles of financial independence and time sovereignty (FITS) empower individuals to escape the debt-based cycle that forces them into perpetual work. What if companies could apply the same principles? What if businesses, instead of succumbing to the relentless push for infinite growth, could optimize for real demand?
This case study of the GPU industry aims to show that fiat-driven incentives distort technological progress and imagines an alternative future built on sound money.
### **Fiat Business: Growth or Death**
Tech companies no longer optimize for efficiency, longevity, or real user needs. Instead, under a fiat system, they are forced into a perpetual growth model. If NVIDIA, AMD, or Intel fail to show revenue expansion, their stock price tanks. Let's take NVIDIA's GPUs as an example. The result is predictable:
- GPUs that nobody actually needs but everyone is told to buy.
- A focus on artificial benchmarks instead of real-world performance stability.
- Endless FPS increases that mean nothing for 99% of users.
The RTX 5090 is not for gamers. It is for NVIDIA’s quarterly earnings. This is not a surprise on a fiat standard.
### **Fiat Marketing: The Illusion of Need and the Refresh Rate Trap**
Benchmarks confirm that once a GPU maintains 120+ FPS in worst-case scenarios, additional performance gains become irrelevant for most players. This level of capability was reached years ago. The problem is that efficiency does not sell as easily as bigger numbers.
 This extends beyond raw GPU power and into the display market, where increasing refresh rates and resolutions are marketed as critical upgrades, despite diminishing real-world benefits for most users. While refresh rates above 120Hz may offer marginal improvements for competitive esports players, the average user sees little benefit beyond a certain threshold. Similarly, 8K resolutions are pushed as the next frontier, even though 4K remains underutilized due to game optimization and hardware constraints. This is why GPUs keep getting bigger, hotter, and more expensive, even when most gamers would be fine with a card from five years ago. It is why every generation brings another “must-have” feature, regardless of whether it impacts real-world performance. 
Marketing under fiat operates on the principle of making people think they need something they do not. The fiat standard does not just distort capital allocation. It manufactures demand by exaggerating the importance of specifications that most users do not truly need.
The goal is not technological progress but sales volume. True innovation would focus on meaningful performance gains that align with actual gaming demands, such as improving latency, frame-time consistency, and efficient power consumption. Instead, marketing convinces consumers they need unnecessary upgrades, driving them into endless hardware cycles that favor stock prices over user experience.
They need the next-gen cycle to maintain high margins. The hardware is no longer designed for users. It is designed for shareholders. A company operating on sound money would not rely on deceptive marketing cycles. It would align product development with real user needs instead of forcing artificial demand.
### **The Shift to AI**
For years, GPUs were optimized for gaming. Then AI changed everything. OpenAI, Google, and Stability AI now outbid consumers for GPUs. The 4090 became impossible to find, not because of gamers, but because AI labs were hoarding them.
The same companies that depended on the consumer upgrade cycle now see their real profits coming from data centers. Yet, they still push gaming hardware aggressively. However, legitimate areas for improvement do exist. While marketing exaggerates the need for higher FPS at extreme resolutions, real gaming performance should focus on frame stability, low latency, and efficient rendering techniques. These are the areas where actual innovation should be happening. Instead, the industry prioritizes artificial performance milestones to create the illusion of progress, rather than refining and optimizing for the gaming experience itself. Why?
### **Gamers Fund the R&D for AI and Bear the Cost of Scalping**
NVIDIA still needs gamers, but not in the way most think. The gaming market provides steady revenue, but it is no longer the priority. With production capacity shifting toward AI and industrial clients, fewer GPUs are available for gamers. This reduced supply has led to rampant scalping, where resellers exploit scarcity to drive up prices beyond reasonable levels. Instead of addressing the issue, NVIDIA benefits from the inflated demand and price perception, creating an even stronger case for prioritizing enterprise sales. Gaming revenue subsidizes AI research. The more RTX cards they sell, the more they justify pouring resources into data-center GPUs like the H100, which generate significantly higher margins than gaming hardware.
AI dictates the future of GPUs. If NVIDIA and AMD produced dedicated gamer-specific GPUs in higher volumes, they could serve that market at lower prices. But in the fiat-driven world of stockholder demands, maintaining artificially constrained supply ensures maximum profitability. Gamers are left paying inflated prices for hardware that is no longer built with them as the primary customer. That is why GPU prices keep climbing. Gamers are no longer the main customer. They are a liquidity pool.
### **The Financial Reality**
The financial reports confirm this shift: **NVIDIA’s 2024 fiscal year** saw a 126% revenue increase, reaching \$60.9 billion. The data center segment alone grew 217%, generating \$47.5 billion. ([Source](https://investor.nvidia.com/news/press-release-details/2024/NVIDIA-Announces-Financial-Results-for-Fourth-Quarter-and-Fiscal-2024/))
The numbers make it clear. The real money is in AI and data centers, not gaming. NVIDIA has not only shifted its focus away from gamers but has also engaged in financial engineering to maintain its dominance. The company has consistently engaged in substantial stock buybacks, a hallmark of fiat-driven financial practices. In August 2023, NVIDIA announced a \$25 billion share repurchase program, surprising some investors given the stock's significant rise that year. ([Source](https://www.reuters.com/technology/nvidias-25-billion-buyback-a-head-scratcher-some-shareholders-2023-08-25/)) This was followed by an additional \$50 billion buyback authorization in 2024, bringing the total to \$75 billion over two years. ([Source](https://www.marketwatch.com/story/nvidias-stock-buyback-plan-is-one-of-the-biggest-of-2024-is-that-a-good-thing-9beba5c5))
These buybacks are designed to return capital to shareholders and can enhance earnings per share by reducing the number of outstanding shares. However, they also reflect a focus on short-term stock price appreciation rather than long-term value creation. Instead of using capital for product innovation, NVIDIA directs it toward inflating stock value, ultimately reducing its long-term resilience and innovation potential. In addition to shifting production away from consumer GPUs, NVIDIA has also enabled AI firms to use its chips as collateral to secure massive loans. Lambda, an AI cloud provider, secured a \$500 million loan backed by NVIDIA's H200 and Blackwell AI chips, with financing provided by Macquarie Group and Industrial Development Funding. ([Source](https://www.reuters.com/technology/lambda-secures-500-mln-loan-with-nvidia-chips-collateral-2024-04-04/))
This practice mirrors the way Bitcoin miners have used mining hardware as collateral, expecting continuous high returns to justify the debt. GPUs are fast-depreciating assets that lose value rapidly as new generations replace them. Collateralizing loans with such hardware is a high-risk strategy that depends on continued AI demand to justify the debt. AI firms borrowing against them are placing a leveraged bet on demand staying high. If AI market conditions shift or next-generation chips render current hardware obsolete, the collateral value could collapse, leading to cascading loan defaults and liquidations.
This is not a sound-money approach to business. It is fiat-style quicksand financialization, where loans are built on assets with a limited shelf life. Instead of focusing on sustainable capital allocation, firms are leveraging their future on rapid turnover cycles. This further shifts resources away from gamers, reinforcing the trend where NVIDIA prioritizes high-margin AI sales over its original gaming audience. 
At the same time, NVIDIA has been accused of leveraging anti-competitive tactics to maintain its market dominance. The GeForce Partner Program (GPP) launched in 2018 sought to lock hardware partners into exclusive deals with NVIDIA, restricting consumer choice and marginalizing AMD. Following industry backlash, the program was canceled. ([Source](https://en.wikipedia.org/wiki/GeForce_Partner_Program)) 
NVIDIA is not merely responding to market demand but shaping it through artificial constraints, financialization, and monopolistic control. The result is an industry where consumers face higher prices, limited options, and fewer true innovations as companies prioritize financial games over engineering excellence.
On this basis, short-term downturns fueled by stock buybacks and leveraged bets create instability, leading to key staff layoffs. This forces employees into survival mode rather than fostering long-term innovation and career growth. Instead of building resilient, forward-looking teams, companies trapped in fiat incentives prioritize temporary financial engineering over actual product and market development.
### **A Sound Money Alternative: Aligning Incentives**
Under a sound money system, consumers would become more mindful of purchases as prices naturally decline over time. This would force businesses to prioritize real value creation instead of relying on artificial scarcity and marketing hype. Companies would need to align their strategies with long-term customer satisfaction and sustainable engineering instead of driving demand through planned obsolescence.
Imagine an orange-pilled CEO at NVIDIA. Instead of chasing infinite growth, they persuade the board to pivot toward sustainability and long-term value creation. The company abandons artificial product cycles, prioritizing efficiency, durability, and cost-effectiveness. Gaming GPUs are designed to last a decade, not three years. The model shifts to modular upgrades instead of full replacements. Pricing aligns with real user needs, not speculative stock market gains.
Investors initially panic. The stock takes a temporary hit, but as consumers realize they no longer need to upgrade constantly, brand loyalty strengthens. Demand stabilizes, reducing volatility in production and supply chains. Gamers benefit from high-quality products that do not degrade artificially. AI buyers still access high-performance chips but at fair market prices, no longer subsidized by forced consumer churn.
This is not an abstract vision. Businesses could collateralize loans with Bitcoin. Companies could also leverage highly sought-after end products that maintain long-term value. Instead of stock buybacks or anti-competitive practices, companies would focus on building genuine, long-term value. A future where Bitcoin-backed reserves replace fiat-driven financial engineering would stabilize capital allocation, preventing endless boom-bust cycles. This shift would eliminate the speculative nature of AI-backed loans, fostering financial stability for both borrowers and lenders.
Sound money leads to sound business. When capital allocation is driven by real value rather than debt-fueled expansion, industries focus on sustainable innovation rather than wasteful iteration.
### **Reclaiming Time Sovereignty for Companies**
The fiat system forces corporations into unsustainable growth cycles. Companies that embrace financial independence and time sovereignty can escape this trap and focus on long-term value.
GPU development illustrates this distortion. The RTX 3080 met nearly all gaming needs, yet manufacturers push unnecessary performance gains to fuel stock prices rather than improve usability. GPUs are no longer designed for gamers but for AI and enterprise clients, shifting NVIDIA’s priorities toward financial engineering over real innovation.
This cycle of GPU inflation stems from fiat-driven incentives—growth for the sake of stock performance rather than actual demand. Under a sound money standard, companies would build durable products, prioritizing efficiency over forced obsolescence.
Just as individuals can reclaim financial sovereignty, businesses can do the same. Embracing sound money fosters sustainable business strategies, where technology serves real needs instead of short-term speculation.
#Bitcoin
#FITS
#Marketing
#TimeSovereignty
#BitcoinFixesThis
#OptOut
#EngineeringNotFinance
#SoundBusiness