-

@ fc481c65:e280e7ba
2025-02-22 02:53:44
A matrix is a rectangular array of numbers, symbols, or expressions, arranged in rows and columns. The individual items in a matrix are called its elements or entries. They are foundational element in many areas of [[Mathematics]] and [[Engineering]], including [[Electronics]], [[Computer Science]], [[Finances]], and more.
### Notation and Terms
- **Dimensions**: The size of a matrix is defined by its number of rows and columns and is often referred to as `m x n`, where `m` is the number of rows and `n` is the number of columns.
- **Square Matrix**: A matrix with the same number of rows and columns (`n x n`).
- **Diagonal Matrix**: A square matrix where all elements off the main diagonal are zero.
- **Identity Matrix**: A diagonal matrix where all the elements on the main diagonal are 1. It's denoted as `I`.
- **Zero Matrix**: A matrix all of whose entries are zero.
### Basic Matrix Operations
1. **Addition and Subtraction**
- Matrices must be of the same dimensions to be added or subtracted.
- Add or subtract corresponding elements.
- Example:
- $$
\begin{bmatrix}
1 & 2 \\
3 & 4
\end{bmatrix}
+
\begin{bmatrix}
5 & 6 \\
7 & 8
\end{bmatrix}
=
\begin{bmatrix}
6 & 8 \\
10 & 12
\end{bmatrix}
$$
2. **Scalar Multiplication**
- Multiply every element of a matrix by a scalar (a single number).
- Example:
- $$
2 \times
\begin{bmatrix}
1 & 2 \\
3 & 4
\end{bmatrix}
=
\begin{bmatrix}
2 & 4 \\
6 & 8
\end{bmatrix}
$$
3. **Matrix Multiplication**
- The number of columns in the first matrix must be equal to the number of rows in the second matrix.
- The product of an `m x n` matrix and an `n x p` matrix is an `m x p` matrix.
- Multiply rows by columns, summing the products of the corresponding elements.
- Example:
- $$
\begin{bmatrix}
1 & 2 \\
3 & 4
\end{bmatrix}
\times
\begin{bmatrix}
2 & 0 \\
1 & 2
\end{bmatrix}
=
\begin{bmatrix}
(1 \times 2 + 2 \times 1) & (1 \times 0 + 2 \times 2) \\
(3 \times 2 + 4 \times 1) & (3 \times 0 + 4 \times 2)
\end{bmatrix}
=
\begin{bmatrix}
4 & 4 \\
10 & 8
\end{bmatrix}
$$
### Special Matrix Operations
1. **Determinant**
- Only for square matrices.
- A scalar value that can be computed from the elements of a square matrix and encodes certain properties of the matrix.
- Example for a 2x2 matrix:
- $$
\text{det}
\begin{bmatrix}
a & b \\
c & d
\end{bmatrix}
= ad - bc
$$
2. **Inverse**
- Only for square matrices.
- The matrix that, when multiplied by the original matrix, results in the identity matrix.
- Not all matrices have inverses; a matrix must be "nonsingular" to have an inverse.
-
![[Attachments/71ec50617bc4cc58018cf33db28167ab_MD5.png]]
### Practical Applications
- **Solving Systems of Linear Equations**
- Matrices are used to represent and solve systems of linear equations using methods like Gaussian elimination.$$X=A^{-1}\times B$$
- **Transformations in Computer Graphics**
- Matrix multiplication is used to perform geometric transformations such as rotations, translations, and scaling.$$R(\theta) = \begin{bmatrix} \cos(\theta) & -\sin(\theta) \\ \sin(\theta) & \cos(\theta) \end{bmatrix}$$
##### Example System of Linear Equations
Suppose we have the following system of linear equations:
$$
\begin{align*}
3x + 4y &= 5 \\
2x - y &= 1
\end{align*}
$$
This system can be expressed as a matrix equation $AX=B$ where:
- $A$ is the matrix of coefficients,
- $X$ is the column matrix of variables,
- $B$ is the column matrix of constants.
* ***Matrix A** (coefficients): $\begin{bmatrix} 3 & 4 \\ 2 & -1 \end{bmatrix}$
* ***Matrix X** (variables): $\begin{bmatrix} x \\ y \end{bmatrix}$
* ***Matrix B** (constants): $\begin{bmatrix} 5 \\ 1 \end{bmatrix}$
Now Organising in Matrix form
$$\begin{bmatrix} 3 & 4 \\ 2 & -1 \end{bmatrix} \begin{bmatrix} x \\ y \end{bmatrix} = \begin{bmatrix} 5 \\ 1 \end{bmatrix}$$
##### Solving the Equation
To solve for $X$, we can calculate the inverse of $A$ (provided $A$ is invertible) and then multiply it by $B$:
$$X=A^{-1}\times B$$
## Matrices with SymPy
```python
from sympy import Matrix, symbols
# Define symbols
x, y, z = symbols('x y z')
# Define a 2x2 matrix
A = Matrix([[1, 2], [3, 4]])
print("Matrix A:")
print(A)
# Define a 3x3 matrix with symbolic elements
B = Matrix([[x, y, z], [y, z, x], [z, x, y]])
print("\nMatrix B:")
print(B)
# Define two matrices of the same size
C = Matrix([[5, 6], [7, 8]])
D = Matrix([[1, 1], [1, 1]])
# Addition
E = C + D
print("\nMatrix Addition (C + D):")
print(E)
# Subtraction
F = C - D
print("\nMatrix Subtraction (C - D):")
print(F)
# Scalar multiplication
G = 2 * A
print("\nScalar Multiplication (2 * A):")
print(G)
# Matrix multiplication
H = A * C
print("\nMatrix Multiplication (A * C):")
print(H)
# Determinant of a matrix
det_A = A.det()
print("\nDeterminant of Matrix A:")
print(det_A)
# Inverse of a matrix
inv_A = A.inv()
print("\nInverse of Matrix A:")
print(inv_A)
# Define the coefficient matrix A and the constant matrix B
A_sys = Matrix([[3, 4], [2, -1]])
B_sys = Matrix([5, 1])
# Solve the system AX = B
X = A_sys.inv() * B_sys
print("\nSolution to the system of linear equations:")
print(X)
# Compute eigenvalues and eigenvectors of a matrix
eigenvals = A.eigenvals()
eigenvects = A.eigenvects()
print("\nEigenvalues of Matrix A:")
print(eigenvals)
print("\nEigenvectors of Matrix A:")
print(eigenvects)
```
## References
* [Dear linear algebra students, This is what matrices (and matrix manipulation) really look like](https://www.youtube.com/watch?v=4csuTO7UTMo)
* [Essence of linear algebra](https://www.youtube.com/playlist?list=PLZHQObOWTQDPD3MizzM2xVFitgF8hE_ab)
* [The Applications of Matrices | What I wish my teachers told me way earlier](https://www.youtube.com/watch?v=rowWM-MijXU)
* [Inverse of 2x2 Matrix](https://www.cuemath.com/algebra/inverse-of-2x2-matrix/)
* [Matrices Tutorial](https://www.cuemath.com/algebra/solve-matrices/)
-

@ ec965405:63996966
2025-02-22 02:33:04
In the 1960s, [IBM coined the term “word processing”](https://web.stanford.edu/~bkunde/fb-press/articles/wdprhist.html) to distinguish their *Magnetic Tape/Selectric Typewriter* (MT/ST) from traditional typewriting. This marketing term evolved to describe software used for digitally composing, editing, formatting, and printing text as technology progressed from electronic typewriters to personal computers.
Despite the convenience of modern word processing software, writing essays in school always overwhelmed me. Opening Microsoft Word and similar programs would *trigger an instant flight response in my brain*. The screen was lined with toolbars stuffed with unnecessary buttons and dropdown menus that I never used. Why force something like this for a 500-word reflection on a short reading?
<figure>
<img src="https://www.versionmuseum.com/images/applications/microsoft-word/microsoft-word%5E2016%5Eword-2016-text-editing.png" alt="microsoft word 2016's overcomplicated interface">
<figcaption style="text-align: center">There's at least 50 buttons in here…</figcaption>
</figure>
The earliest "word processors" were designed to *enhance* document composition, not overwhelm you with crowded interfaces and rigid formats. The worst part was that there was no escape! Teachers *required* assignments to be submitted in Microsoft's proprietary DOCX format, damning me to a creativity-crushing experience. The ensuing mental stress outweighed the occasional praise I received about my writing skills from my professors whenever I managed to put out a decent essay. Am I composing a thesis, I used to think, or just *processing words*?
I graduated university with a sour taste in my mouth for *“word processing”* that hindered me in future job roles. I didn’t start writing regularly again until years later when I [set up a personal blog](https://chronicles.miguelalmodo.com) with a [built-in text editor](https://simplemde.com/). That's when I was introduced to an exciting new way to compose texts that healed the trauma I endured from years of "processing words" in school.
<figure>
<img src="https://www.bludit.com/img/bludit_1_en.png?version=3.9.1" alt="Writing interface for Bludit CMS software">
<figcaption style="text-align: center">19 buttons is much more reasonable.</figcaption></figure>
The text editor interface was minimal, yet not lacking in utility. It gives big MT/ST vibes. The revolutionary feature for me was that it saved my blogs not in a DOCX file, but in a portable plain text format called *Markdown* (.md).
### CommonMark
Created in 2004, Markdown [(or CommonMark](https://commonmark.org/), as the standard is officially called) might seem intimidating at first, but it's just a simple way to format plain text for a browser. Markdown makes your writing digitally legible for [5.5 billion web surfers.](https://www.itu.int/en/ITU-D/Statistics/pages/stat/default.aspx)
<figure>
<img src="https://scientificallysoundorg360.files.wordpress.com/2021/02/markdown_simple_example.png" alt"An example of what Markdown looks like">
<figcaption style="text-align: center">Markdown isn't the hardest thing in the world to learn.</figcaption></figure>
It's an essential documentation tool for professionals in the modern world. News organizations, bloggers, and academics use it to draft articles and it's the standard for software documentation. You can even [format your chat messages in Discord](https://support.discord.com/hc/en-us/articles/210298617-Markdown-Text-101-Chat-Formatting-Bold-Italic-Underline) with it! Markdown is widely adopted and makes your writing portable and convertible to other formats. Whenever I wanted to try a new blog hosting solution, I could simply copy over each blog.md file for a browser-native reading experience. You can't do that with DOCX.
Markdown isn't just for technical people—it's an intuitive way for anyone to write that can bring us back from the brink of ultra-processed words. ***Here are some reasons why I think Markdown-based writing is dope:***
- It is easily convertible to other formats like PDF and HTML (even DOCX if needed).
- It future-proofs your writing.
- A whole genre of minimal text editors becomes available to use that reduces distractions and helps you focus on crafting ideas.
- Plain text puts you in control of formatting. There is no rigid document structure in markdown editors that warps pasted content like with Microsoft Word, preventing unnecessary friction in the creative process.
- Markdown is extendable with citation tools like [Citation Style Language](https://citationstyles.org/developers/) files.
### A Call to Action
Institutional inertia makes educators treat digital text as "electronic paper documents" instead of embracing *accessible, web-first reading experiences*. The ironic part is that *educational institutions pioneered information sharing on the early internet* — so why do they impose overstimulating and proprietary work flows on students and workers now? With Markdown, a return to web-first work flows aligns with the Internet's original purpose! ***Academia is overdue for a shift back to web-first text formatting.***
<figure>
<img src="https://slideplayer.com/slide/14615427/90/images/6/ARPANET+1968:+ARPA+1969:+BBN+Technologies+Growth+(ARPANET).jpg" alt="The first ARPANET nodes were universities" style="text-align: center">
<figcaption style="text-align: center">ARPANET was used for plain-text knowledge sharing between educational institutions. </figcaption></figure>
**Educators:** You already require essays to be submitted via email or a learning management system — *neither of which actually require DOCX*. Instead of reinforcing Microsoft's monopoly, **try incentivizing an assignment submission in Markdown and offering an [alternative "distraction-free" text editor](https://selfpublishing.com/distraction-free-writing-apps/) to see how students respond.**
**Students:** Regularly writing out your thoughts is a valuable workout for your brain. **Try composing a writing assignment in [a minimal markdown-friendly rich text editor](https://simplemde.com/) to see if you enjoy it more than a word processor.**
For fun, you could self host a blog with [Bludit](https://bludit.com) or publish content on a blogging site like [habla.news](https://habla.news) or [tumblr](https://tumblr.com) (tumblr's rich text editor is my favorite).
-

@ d34e832d:383f78d0
2025-02-22 01:07:13
npub16d8gxt2z4k9e8sdpc0yyqzf5gp0np09ls4lnn630qzxzvwpl0rgq5h4rzv
### **How to Run a Local Matrix Server for Secure Communications**
Running a self-hosted Matrix server allows you to take control of your communications, ensuring privacy and sovereignty. By hosting your own homeserver, you eliminate reliance on centralized services while maintaining encrypted, federated messaging. This guide covers the full installation process for setting up a Matrix homeserver using **Synapse**, the official reference implementation.
---
## **1. Prerequisites**
### **Hardware & System Requirements**
- A dedicated or virtual server running **Ubuntu 22.04 LTS** (or Debian-based OS)
- Minimum **2GB RAM** (4GB+ recommended for production)
- At least **10GB disk space** (more if storing media)
- **A public domain name** (optional for federation)
### **Dependencies**
Ensure your system is updated and install required packages:
```sh
sudo apt update && sudo apt upgrade -y
sudo apt install -y curl wget gnupg2 lsb-release software-properties-common python3-pip virtualenv
```
---
## **2. Install Matrix Synapse**
### **Step 1: Add Matrix Repository**
```sh
sudo apt install -y lsb-release wget apt-transport-https
sudo wget -O /usr/share/keyrings/matrix-keyring.asc https://packages.matrix.org/debian/matrix-org-archive-keyring.gpg
echo "deb [signed-by=/usr/share/keyrings/matrix-keyring.asc] https://packages.matrix.org/debian/ $(lsb_release -cs) main" | sudo tee /etc/apt/sources.list.d/matrix-org.list
sudo apt update
```
### **Step 2: Install Synapse**
```sh
sudo apt install -y matrix-synapse-py3
```
During installation, enter your desired **domain name** (e.g., `matrix.example.com`).
### **Step 3: Start and Enable Synapse**
```sh
sudo systemctl enable --now matrix-synapse
```
---
## **3. Configure Matrix Homeserver**
### **Step 1: Edit the Configuration File**
```sh
sudo nano /etc/matrix-synapse/homeserver.yaml
```
- Set `server_name` to your domain (`example.com`)
- Enable **registration** if needed (`enable_registration: true`)
- Configure **log level** (`log_level: INFO`)
Save and exit (`CTRL+X`, then `Y`).
### **Step 2: Restart Synapse**
```sh
sudo systemctl restart matrix-synapse
```
---
## **4. Set Up a Reverse Proxy (NGINX + Let’s Encrypt SSL)**
### **Step 1: Install NGINX and Certbot**
```sh
sudo apt install -y nginx certbot python3-certbot-nginx
```
### **Step 2: Obtain SSL Certificate**
```sh
sudo certbot --nginx -d matrix.example.com
```
### **Step 3: Configure NGINX**
Create a new file:
```sh
sudo nano /etc/nginx/sites-available/matrix
```
Paste the following configuration:
```nginx
server {
listen 80;
server_name matrix.example.com;
return 301 https://$host$request_uri;
}
server {
listen 443 ssl;
server_name matrix.example.com;
ssl_certificate /etc/letsencrypt/live/matrix.example.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/matrix.example.com/privkey.pem;
location /_matrix/ {
proxy_pass http://localhost:8008;
proxy_set_header X-Forwarded-For $remote_addr;
proxy_set_header Host $host;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
```
Save and exit.
### **Step 4: Enable the Configuration**
```sh
sudo ln -s /etc/nginx/sites-available/matrix /etc/nginx/sites-enabled/
sudo systemctl restart nginx
```
---
## **5. Create an Admin User**
Run the following command:
```sh
register_new_matrix_user -c /etc/matrix-synapse/homeserver.yaml http://localhost:8008
```
Follow the prompts to create an **admin** user.
---
## **6. Test Your Matrix Server**
- Open a browser and go to: `https://matrix.example.com/_matrix/client/versions`
- You should see a JSON response indicating your homeserver is running.
- Use **Element** (https://element.io) to log in with your new user credentials.
---
## **7. Federation (Optional)**
To allow federation, ensure your DNS has an **SRV record**:
```
_matrix._tcp.example.com. 3600 IN SRV 10 5 443 matrix.example.com.
```
You can check your federation status at:
https://federationtester.matrix.org/
---
## **8. Additional Features**
### **Bridges (Connect to Other Platforms)**
- **Discord Bridge**: https://github.com/Half-Shot/matrix-appservice-discord
- **Telegram Bridge**: https://github.com/tulir/mautrix-telegram
### **Hosting a TURN Server (For Calls & Video)**
Install **coturn** for improved call reliability:
```sh
sudo apt install -y coturn
```
Edit `/etc/turnserver.conf` to include:
```
listening-port=3478
fingerprint
use-auth-secret
static-auth-secret=your-random-secret
realm=matrix.example.com
```
Enable and start the service:
```sh
sudo systemctl enable --now coturn
```
---
## **9. Backup & Maintenance**
### **Database Backup**
```sh
sudo systemctl stop matrix-synapse
sudo tar -czvf matrix-backup.tar.gz /var/lib/matrix-synapse/
sudo systemctl start matrix-synapse
```
### **Updating Synapse**
```sh
sudo apt update && sudo apt upgrade -y matrix-synapse-py3
sudo systemctl restart matrix-synapse
```
---
## **Connections**
- **Self-Hosting for Digital Sovereignty**
- **Nostr and Matrix Integration for Decentralized Messaging**
## **Donations via**
- lightninglayerhash@getalby.com
-

@ d34e832d:383f78d0
2025-02-22 00:57:07
[npub16d8gxt2z4k9e8sdpc0yyqzf5gp0np09ls4lnn630qzxzvwpl0rgq5h4rzv]
**Why Signal and Matrix Matter in a World of Mass Surveillance**
In an era where mass surveillance has become the norm, privacy-focused communication tools are essential for digital sovereignty. Signal and Matrix provide individuals and communities with encrypted, censorship-resistant messaging solutions that challenge centralized control over information exchange.
### **1. Signal – End-to-End Encryption and Metadata Resistance**
- **Proven Cryptographic Security**: Signal’s end-to-end encryption, powered by the Signal Protocol, ensures messages remain private even if intercepted.
- **Metadata Protection with Sealed Sender**: Signal minimizes metadata exposure, preventing third parties from knowing who is communicating with whom.
- **Simple and Ubiquitous Privacy**: Designed for ease of use, Signal bridges the gap between security and accessibility, making privacy mainstream.
### **2. Matrix – Decentralized and Federated Communication**
- **Self-Hosted and Federated Infrastructure**: Unlike centralized services, Matrix allows users to host their own servers, reducing reliance on single points of failure.
- **Bridging Across Platforms**: Matrix bridges seamlessly with other communication protocols (IRC, XMPP, Slack, etc.), creating a unified, decentralized network.
- **End-to-End Encryption and Decentralized Identity**: Matrix offers fully encrypted rooms and a decentralized identity system, ensuring control over user data.
### **3. Mass Surveillance vs. Private Communication**
- **Government and Corporate Data Harvesting**: Centralized platforms like WhatsApp, Facebook Messenger, and Gmail log metadata and conversations, creating massive surveillance databases.
- **The Threat of AI-Driven Censorship**: Machine learning algorithms analyze user behavior, making privacy-invasive censorship and social credit scoring systems a reality.
- **Decentralization as a Resistance Strategy**: By adopting Signal and Matrix, individuals reclaim their right to private conversations without interference from state or corporate actors.
### **Integration Potential and Future Developments**
- **Bitcoin and Encrypted Messaging**: Signal and Matrix can be combined with Bitcoin for private transactions and communication, furthering financial sovereignty.
- **P2P and Nostr Integration**: Future developments could see Matrix and Nostr integrated for even more robust censorship-resistant communication.
### **Donations via**
- lightninglayerhash@getalby.com
-

@ cda24367:8ba5afc3
2025-02-22 00:11:32
Just a test to find out what this Habla.news does. It looks interesting.
And here's an Odysee video to see if the iframe will work.
<iframe id="odysee-iframe" style="width:100%; aspect-ratio:16 / 9;" src="https://odysee.com/$/embed/@RandomStuff:0/Steve-Hughes-on-Charity:0?r=9cn37JvaLAtLK8z8NfZjsqm8ZNCaNuUy" allowfullscreen></iframe>
-

@ 916a7ab7:146bb83e
2025-02-21 23:12:49
tens of thousands of Afghans flee
by often desperate measures,
detained, assaulted, murdered,
martyred
faithless and in fetters.
school girls in Uganda know
they many never have a wedding
when terror rapes
the peace they sow,
punished by beheading.
Chinese mosques, temples, homes
are dismantled brick by brick
for believing something higher than
the state or politic.
the glowing boxes in our hands
or hanging from our walls
manipulate the way we think
becoming passive thralls.
-

@ 916a7ab7:146bb83e
2025-02-21 22:43:35
my eyes
---
are not blurry
---
when they see through lenses
---
of someone's suffering, and then
---
I see.
---
-

@ fc481c65:e280e7ba
2025-02-21 21:30:56
#Algebra is a branch of #Mathematics that uses symbols, known as variables (like x or y), to represent numbers in equations and formulas. It involves operations like addition, subtraction, multiplication, and division, but these operations are performed on variables as well as numbers.
### Key Concepts in Algebra
- **Variables**: Symbols that stand in for unknown values.
- **Constants**: Known values that don’t change.
- **Coefficients**: Numbers used to multiply a variable.
- **Expressions**: Combinations of variables, numbers, and operations (like 3x + 4).
- **Equations**: Statements that assert the equality of two expressions, typically including an equals sign (like 2x + 3 = 7).
### Basic Operations
1. **Adding and Subtracting**: You can add or subtract like terms (terms whose variables and their powers are the same). For example, 2x+3x=5x
2. **Multiplying and Dividing**: You multiply or divide both the coefficients and the variables. For instance, `$$3x \times 2x=6x^2$$`
3. **Solving Equations**: The goal is often to isolate the variable on one side of the equation to find its value. This can involve reversing operations using opposite operations.
### Applications of Algebra
Algebra is used in various fields, from #Engineering and computer science to economics and everyday problem solving. It helps in creating formulas to understand relationships between quantities and in solving equations that model real-world situations.
## Algebra and Electronics
Algebra plays a crucial role in #Electronics engineering, particularly through its application in circuit analysis, signal processing, and control systems. Understanding and utilizing algebraic techniques can significantly enhance problem-solving capabilities in these areas.
### 1. Complex Numbers
In electronic engineering, complex numbers are essential for analyzing AC circuits. They help in representing sinusoidal signals, which are fundamental in communications and power systems.
- **Representation**: z=a+bi or z=reiθ (polar form)
- **Operations**: Addition, subtraction, multiplication, and division in complex form, crucial for understanding the behavior of circuits in the frequency domain.
**Example**: Calculating the impedance of an RLC series circuit at a certain frequency.
- **Circuit Components**: R=50Ω, L=0.1 H, C=10 μF, ω=1000 rad/s
`$$
Z = 50 + j1000 \times 0.1 - \frac{1}{j1000 \times 10 \times 10^{-6}} = 50 + j100 - \frac{1}{j0.01} = 50 + j100 + 100j = 50 + 200j
$$`
### 2. Matrices and Determinants
Matrices are widely used in electronic engineering for handling multiple equations simultaneously, which is common in systems and network analysis.
- **Matrix Operations**: Addition, subtraction, multiplication, and inversion.
- **Determinant and Inverse**: Used in solving systems of linear equations, critical in network theory and control systems.
### 3. Fourier Transforms
Algebraic manipulation is key in applying Fourier transforms, which convert time-domain signals into their frequency components. This is crucial for signal analysis, filtering, and system design.
- **Fourier Series**: Represents periodic signals as a sum of sinusoids.
- **Fourier Transform**: Converts continuous time-domain signals to continuous frequency spectra.
### 4. Laplace Transforms
Laplace transforms are used to simplify the process of analyzing and designing control systems and circuits by converting differential equations into algebraic equations.
- **Transfer Functions**: Represent systems in the s-domain, facilitating easier manipulation and understanding of system dynamics.
### 5. Z-Transforms
Similar to Laplace transforms, Z-transforms are used for discrete systems prevalent in digital signal processing and digital control.
### 6. Algebraic Equations in Filter Design
Algebra is used in the design of filters, both analog and digital, where polynomial equations are used to determine filter coefficients that meet specific frequency response criteria.
### 7. Control Systems
The design and stability analysis of control systems involve solving characteristic equations and manipulating transfer functions, which require a solid understanding of algebra.
### 8. Network Theorems
Theorems like Kirchhoff's laws, Thevenin’s theorem, and Norton’s theorem involve algebraic equations to simplify and analyze circuits.
## Algebra with Sympy
TODO
-

@ 4857600b:30b502f4
2025-02-21 21:15:04
In a revealing development that exposes the hypocrisy of government surveillance, multiple federal agencies including the CIA and FBI have filed lawsuits to keep Samourai Wallet's client list sealed during and after trial proceedings. This move strongly suggests that government agencies themselves were utilizing Samourai's privacy-focused services while simultaneously condemning similar privacy tools when used by ordinary citizens.
The situation bears striking parallels to other cases where government agencies have hidden behind "national security" claims, such as the Jeffrey Epstein case, highlighting a troubling double standard: while average citizens are expected to surrender their financial privacy through extensive reporting requirements and regulations, government agencies claim exemption from these same transparency standards they enforce on others.
This case exemplifies the fundamental conflict between individual liberty and state power, where government agencies appear to be using the very privacy tools they prosecute others for using. The irony is particularly stark given that money laundering for intelligence agencies is considered legal in our system, while private citizens seeking financial privacy face severe legal consequences - a clear demonstration of how the state creates different rules for itself versus the people it claims to serve.
Citations:
[1] https://www.bugle.news/cia-fbi-dnc-rnc-all-sue-to-redact-samourais-client-list-from-trial/
-

@ dbb19ae0:c3f22d5a
2025-02-21 20:38:43
``` python
#!/usr/bin/env python3
import asyncio
from nostr_sdk import Metadata, Client, NostrSigner, Keys, Filter, PublicKey, Kind, init_logger, LogLevel
from datetime import timedelta
async def main():
init_logger(LogLevel.INFO)
secret_key = "nsec1........Replace with your actual nsec secret key"
keys = Keys.parse(secret_key)
signer = NostrSigner.keys(keys)
client = Client(signer)
await client.add_relay("wss://relay.damus.io")
await client.connect()
# Update metadata
new_metadata = Metadata().set_name( "MyName")\
.set_nip05("MyName@example.com")\
.set_lud16("MyName@lud16.com")
await client.set_metadata(new_metadata)
print("Metadata updated successfully.")
# Get updated metadata
npub = "npub1....Replace with your actual npub"
pk = PublicKey.parse(npub)
print(f"\nGetting profile metadata for {npub}:")
metadata = await client.fetch_metadata(pk, timedelta(seconds=15))
print(metadata)
if __name__ == '__main__':
asyncio.run(main())
```
-

@ 4c96d763:80c3ee30
2025-02-21 20:31:55
# Changes
## Ethan Tuttle (1):
- feat: ctrl+enter when creating a new note, sends the note, the same way clicking the "Post Now" button.
## Ken Sedgwick (11):
- drive-by compiler warning fixes
- drive-by clippy fixes
- add derive Debug to some things
- panic on unknown CLI arguments
- move RelayDebugView to notedeck crate and restore --relay-debug
- add diagnostic string to DecodeFailed
- improve relay message parsing unit tests
- fix event error return when decoding fails
- fix OK message parser to include last message component
- fix EOSE parsing to handle extra whitespace
- check message length before prefix comparisons
## William Casarin (5):
- clippy: fix enum too large issue
- changelog: add unreleased section
- clippy: fix lint
- args: skip creation of vec
- nix: fix android build
## jglad (4):
- fix: handle missing file [#715]
- refactor
- fix compilation
- hide nsec in account panel
pushed to [notedeck:refs/heads/master](http://git.jb55.com/notedeck/commit/32c7f83bd7a807155de6a13b66a7d43f48c25e13.html)
-

@ a95c6243:d345522c
2025-02-21 19:32:23
*Europa – das Ganze ist eine wunderbare Idee,* *\
aber das war der Kommunismus auch.* *\
Loriot*  
**«Europa hat fertig», könnte man unken,** und das wäre nicht einmal sehr verwegen. Mit solch einer [Einschätzung](https://transition-news.org/geopolitische-ohnmacht-und-die-last-des-euro-steht-die-eu-vor-der-implosion) stünden wir nicht alleine, denn die Stimmen in diese Richtung mehren sich. Der französische Präsident Emmanuel Macron warnte schon letztes Jahr davor, dass «unser Europa sterben könnte». Vermutlich hatte er dabei andere Gefahren im Kopf als jetzt der ungarische Ministerpräsident Viktor Orbán, der ein «baldiges Ende der EU» prognostizierte. Das Ergebnis könnte allerdings das gleiche sein.
**Neben vordergründigen Themenbereichen wie Wirtschaft, Energie und Sicherheit** ist das eigentliche Problem jedoch die obskure Mischung aus aufgegebener Souveränität und geschwollener Arroganz, mit der europäische Politiker:innende unterschiedlicher Couleur aufzutreten pflegen. Und das Tüpfelchen auf dem i ist die bröckelnde Legitimation politischer Institutionen dadurch, dass die Stimmen großer Teile der Bevölkerung seit Jahren auf vielfältige Weise ausgegrenzt werden.
**Um «UnsereDemokratie» steht es schlecht.** Dass seine Mandate immer schwächer werden, merkt natürlich auch unser «Führungspersonal». Entsprechend werden die Maßnahmen zur Gängelung, Überwachung und Manipulation der Bürger ständig verzweifelter. Parallel dazu [plustern](https://www.bundesregierung.de/breg-de/service/newsletter-und-abos/bundesregierung-aktuell/ausgabe-07-2025-februar-21-2335652?view=renderNewsletterHtml) sich in Paris Macron, Scholz und einige andere noch einmal mächtig in Sachen Verteidigung und [«Kriegstüchtigkeit»](https://transition-news.org/europaische-investitionsbank-tragt-zur-kriegstuchtigkeit-europas-bei) auf.
**Momentan gilt es auch, das Überschwappen covidiotischer und verschwörungsideologischer Auswüchse** aus den USA nach Europa zu vermeiden. So ein «MEGA» (Make Europe Great Again) können wir hier nicht gebrauchen. Aus den Vereinigten Staaten kommen nämlich furchtbare Nachrichten. Beispielsweise wurde einer der schärfsten Kritiker der Corona-Maßnahmen kürzlich zum Gesundheitsminister ernannt. Dieser setzt sich jetzt für eine Neubewertung der mRNA-«Impfstoffe» ein, was durchaus zu einem [Entzug der Zulassungen](https://transition-news.org/usa-zulassungsentzug-fur-corona-impfstoffe-auf-der-tagesordnung) führen könnte.
**Der europäischen Version von** **[«Verteidigung der Demokratie»](https://transition-news.org/eu-macht-freiwilligen-verhaltenskodex-gegen-desinformation-zu-bindendem-recht)** setzte der US-Vizepräsident J. D. Vance auf der Münchner Sicherheitskonferenz sein Verständnis entgegen: «Demokratie stärken, indem wir unseren Bürgern erlauben, ihre Meinung zu sagen». Das Abschalten von Medien, das Annullieren von Wahlen oder das Ausschließen von Menschen vom politischen Prozess schütze gar nichts. Vielmehr sei dies der todsichere Weg, die Demokratie zu zerstören.
**In der Schweiz kamen seine Worte deutlich besser an** als in den meisten europäischen NATO-Ländern. Bundespräsidentin Karin Keller-Sutter lobte die Rede und interpretierte sie als «Plädoyer für die direkte Demokratie». Möglicherweise zeichne sich hier eine [außenpolitische Kehrtwende](https://transition-news.org/schweiz-vor-aussenpolitischer-kehrtwende-richtung-integraler-neutralitat) in Richtung integraler Neutralität ab, meint mein Kollege Daniel Funk. Das wären doch endlich mal ein paar gute Nachrichten.
**Von der einstigen Idee einer europäischen Union** mit engeren Beziehungen zwischen den Staaten, um Konflikte zu vermeiden und das Wohlergehen der Bürger zu verbessern, sind wir meilenweit abgekommen. Der heutige korrupte Verbund unter technokratischer Leitung ähnelt mehr einem Selbstbedienungsladen mit sehr begrenztem Zugang. Die EU-Wahlen im letzten Sommer haben daran ebenso wenig geändert, wie die [Bundestagswahl](https://transition-news.org/bundestagswahl-mehr-aufrustung-statt-friedenskanzler-nach-der-wahl) am kommenden Sonntag darauf einen Einfluss haben wird.
***
Dieser Beitrag ist zuerst auf ***[Transition News](https://transition-news.org/europaer-seid-ihr-noch-zu-retten)*** erschienen.
-

@ 86a82cab:b5ef38a0
2025-02-21 18:42:50
This morning, I decided to look into the NIP-23 specialized clients, and try to publish long-form content on the Nostr network of relays: https://habla.news/ https://blogstack.io First, I encourage you to read the NIP, and the first thing that you will notice is that the kind id used to publish is 30023 instead of 1, soThis morning, I decided to look into the NIP-23 specialized clients, and try to publish **long-form** content on the **Nostr** network of relays: https://habla.news/ https://blogstack.io First, I encourage you to read the NIP, and the first thing that you will notice is that the kind id used to publish is 30023 instead of 1, so
-

@ 86a82cab:b5ef38a0
2025-02-21 18:32:52
asdasdasdasdasdNIP-23 Long-form Content. draft optional author:fiatjaf. This NIP defines kind:30023 (a parameterized replaceable event) for long-form text content, generally referred to as "articles" or "blog posts".kind:30024 has the same structure as kind:30023 and is used to save long form drafts. "Social"NIP-23 **Long-form** Content. draft optional author:fiatjaf. This NIP defines kind:30023 (a parameterized replaceable event) for **long-form** text content, generally referred to as "articles" or "blog posts".kind:30024 has the same structure as kind:30023 and is used to save **long** **form** drafts. "Social"
-

@ 6e0ea5d6:0327f353
2025-02-21 18:15:52
"Malcolm Forbes recounts that a lady, wearing a faded cotton dress, and her husband, dressed in an old handmade suit, stepped off a train in Boston, USA, and timidly made their way to the office of the president of Harvard University. They had come from Palo Alto, California, and had not scheduled an appointment. The secretary, at a glance, thought that those two, looking like country bumpkins, had no business at Harvard.
— We want to speak with the president — the man said in a low voice.
— He will be busy all day — the secretary replied curtly.
— We will wait.
The secretary ignored them for hours, hoping the couple would finally give up and leave. But they stayed there, and the secretary, somewhat frustrated, decided to bother the president, although she hated doing that.
— If you speak with them for just a few minutes, maybe they will decide to go away — she said.
The president sighed in irritation but agreed. Someone of his importance did not have time to meet people like that, but he hated faded dresses and tattered suits in his office. With a stern face, he went to the couple.
— We had a son who studied at Harvard for a year — the woman said. — He loved Harvard and was very happy here, but a year ago he died in an accident, and we would like to erect a monument in his honor somewhere on campus.
— My lady — said the president rudely —, we cannot erect a statue for every person who studied at Harvard and died; if we did, this place would look like a cemetery.
— Oh, no — the lady quickly replied. — We do not want to erect a statue. We would like to donate a building to Harvard.
The president looked at the woman's faded dress and her husband's old suit and exclaimed:
— A building! Do you have even the faintest idea of how much a building costs? We have more than seven and a half million dollars' worth of buildings here at Harvard.
The lady was silent for a moment, then said to her husband:
— If that’s all it costs to found a university, why don’t we have our own?
The husband agreed.
The couple, Leland Stanford, stood up and left, leaving the president confused. Traveling back to Palo Alto, California, they established there Stanford University, the second-largest in the world, in honor of their son, a former Harvard student."
Text extracted from: "Mileumlivros - Stories that Teach Values."
Thank you for reading, my friend!
If this message helped you in any way,
consider leaving your glass “🥃” as a token of appreciation.
A toast to our family!
-

@ 6e0ea5d6:0327f353
2025-02-21 18:08:19
When talking about the tumbles we experience in our early years, it’s almost impossible not to try to compare the degree of distress that each stumble has provided us.
Even if harmless, the first accident that comes to mind is one that, in some way, left its mark. Whether it’s a scraped knee, a gash on the forehead, or a broken arm: having that first conscious encounter with pain is an unforgettable terror.
For in one moment, we are sheltered in the loving arms of our parents, feeling invulnerable, and moments later there’s a thick mixture of blood and dirt streaming from a cut that doesn’t seem all that exaggerated to someone looking from the outside — but only to those looking from the outside.
However, this is not what makes that first contact with pain so terrifying. It’s the discovery of how lonely we are when facing an individual agony, even under the care of an adult.
Ultimately, what follows the shock can vary from the sting of cleaning the wound to the warning slap. After the understanding is digested, and we learn how wounds are made, we become cautious. A slip is met with laughter, and we grow tougher than the ground.
That is, until we discover the free fall that holds the world’s problems…
“Consciousness, not age, leads to wisdom.”
— Publilius Syrus
Thank you for reading, my friend!
If this message helped you in any way,
consider leaving your glass “🥃” as a token of appreciation.
A toast to our family!
-

@ 266815e0:6cd408a5
2025-02-21 17:54:15
I've been working on the applesauce libraries for a while now but I think this release is the first one I would consider to be stable enough to use
A lot of the core concepts and classes are in place and stable enough where they wont change too much next release
If you want to skip straight to the documentation you can find at [hzrd149.github.io/applesauce](https://hzrd149.github.io/applesauce/) or the typescript docs at [hzrd149.github.io/applesauce/typedoc](https://hzrd149.github.io/applesauce/typedoc)
## Whats new
### Accounts
The `applesauce-accounts` package is an extension of the `applesauce-signers` package and provides classes for building a multi-account system for clients
Its primary features are
- Serialize and deserialize accounts so they can be saved in local storage or IndexededDB
- Account manager for multiple accounts and switching between them
- Account metadata for things like labels, app settings, etc
- Support for NIP-46 Nostr connect accounts
see [documentation](https://hzrd149.github.io/applesauce/accounts/manager.html) for more examples
### Nostr connect signer
The `NostrConnectSigner` class from the `applesauce-signers` package is now in a stable state and has a few new features
- Ability to create `nostrconnect://` URIs and waiting for the remote signer to connect
- SDK agnostic way of subscribing and publishing to relays
For a simple example, here is how to create a signer from a `bunker://` URI
```js
const signer = await NostrConnectSigner.fromBunkerURI(
"bunker://266815e0c9210dfa324c6cba3573b14bee49da4209a9456f9484e5106cd408a5?relay=wss://relay.nsec.app&secret=d9aa70",
{
permissions: NostrConnectSigner.buildSigningPermissions([0, 1, 3, 10002]),
async onSubOpen(filters, relays, onEvent) {
// manually open REQ
},
async onSubClose() {
// close previouse REQ
},
async onPublishEvent(event, relays) {
// Pubilsh an event to relays
},
},
);
```
see [documentation](https://hzrd149.github.io/applesauce/signers/nostr-connect.html) for more examples and other signers
### Event Factory
The `EventFactory` class is probably what I'm most proud of. its a standalone class that can be used to create various types of events from templates ([blueprints](https://hzrd149.github.io/applesauce/typedoc/modules/applesauce_factory.Blueprints.html)) and is really simple to use
For example:
```js
import { EventFactory } from "applesauce-factory";
import { NoteBlueprint } from "applesauce-factory/blueprints";
const factory = new EventFactory({
// optionally pass a NIP-07 signer in to use for encryption / decryption
signer: window.nostr
});
// Create a kind 1 note with a hashtag
let draft = await factory.create(NoteBlueprint, "hello world #grownostr");
// Sign the note so it can be published
let signed = await window.nostr.signEvent(draft);
```
Its included in the `applesauce-factory` package and can be used with any other nostr SDKs or vanilla javascript
It also can be used to modify existing replaceable events
```js
let draft = await factory.modifyTags(
// kind 10002 event
mailboxes,
// add outbox relays
addOutboxRelay("wss://relay.io/"),
addOutboxRelay("wss://nostr.wine/"),
// remove inbox relay
removeInboxRelay("wss://personal.old-relay.com/")
);
```
see [documentation](https://hzrd149.github.io/applesauce/overview/factory.html) for more examples
### Loaders
The `applesauce-loaders` package exports a bunch of loader classes that can be used to load everything from replaceable events (profiles) to timelines and NIP-05 identities
They use [rx-nostr](https://penpenpng.github.io/rx-nostr/) under the hood to subscribe to relays, so for the time being they will not work with other nostr SDKs
I don't expect many other developers or apps to use them since in my experience every nostr client requires a slightly different way or loading events
*They are stable enough to start using but they are not fully tested and they might change slightly in the future*
The following is a short list of the loaders and what they can be used for
- `ReplaceableLoader` loads any replaceable events (0, 3, 1xxxx, 3xxxx)
- `SingleEventLoader` loads single events based on ids
- `TimelineLoader` loads a timeline of events from multiple relays based on filters
- `TagValueLoader` loads events based on a tag name (like "e") and a value, can be used to load replies, zaps, reactions, etc
- `DnsIdentityLoader` loads NIP-05 identities and supports caching
- `UserSetsLoader` loads all lists events for users
see [documentation](https://hzrd149.github.io/applesauce/overview/loaders.html) for more examples
### Real tests
For all new features and a lot of existing ones I'm trying to write tests to ensure I don't leave unexpected bugs for later
I'm not going to pretend its 100% tests coverage or that it will ever get close to that point, but these tests cover some of the core classes and help me prove that my code is doing what it says its supposed to do
At the moment there are about 230 tests covering 45 files. not much but its a start
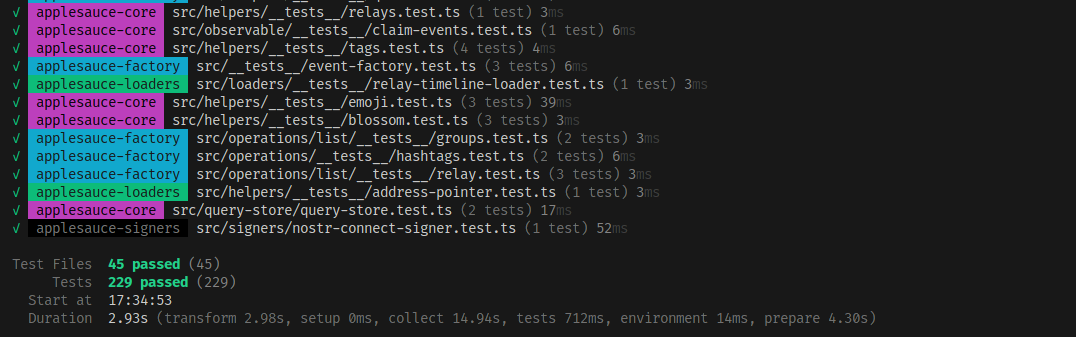
## Apps built using applesauce
If you want to see some examples of applesauce being used in a nostr client I've been testing a lot of this code in production on the apps I've built in the last few months
- [noStrudel](https://github.com/hzrd149/nostrudel) The main app everything is being built for and tested in
- [nsite-manager](https://github.com/hzrd149/nsite-manager) Still a work-in-progress but supports multiple accounts thanks to the `applesauce-accounts` package
- [blossomservers.com](https://github.com/hzrd149/blossomservers) A simple (and incomplete) nostr client for listing and reviewing public blossom servers
- [libretranslate-dvm](https://github.com/hzrd149/libretranslate-dvm) A libretranslate DVM for nostr:npub1mkvkflncllnvp3adq57klw3wge6k9llqa4r60g42ysp4yyultx6sykjgnu
- [cherry-tree](https://github.com/hzrd149/cherry-tree) A chunked blob uploader / downloader. only uses applesauce for boilerplate
- [nsite-homepage](https://github.com/hzrd149/nsite-homepage) A simple landing page for [nsite.lol](https://nsite.lol)
Thanks to nostr:npub1cesrkrcuelkxyhvupzm48e8hwn4005w0ya5jyvf9kh75mfegqx0q4kt37c for teaching me more about rxjs and consequentially making me re-write a lot of the core observables to be faster
-

@ dbb19ae0:c3f22d5a
2025-02-21 17:46:58
Tested and working with nostr_sdk version 0.39
``` python
from nostr_sdk import Metadata, Client, Keys, Filter, PublicKey
from datetime import timedelta
import argparse
import asyncio
import json
async def main(npub):
client = Client()
await client.add_relay("wss://relay.damus.io")
await client.connect()
pk = PublicKey.parse(npub)
print(f"\nGetting profile metadata for {npub}:")
metadata = await client.fetch_metadata(pk, timedelta(seconds=15))
# Printing each field of the Metadata object
print(f"Name: {metadata.get_name()}")
print(f"Display Name: {metadata.get_display_name()}")
print(f"About: {metadata.get_about()}")
print(f"Website: {metadata.get_website()}")
print(f"Picture: {metadata.get_picture()}")
print(f"Banner: {metadata.get_banner()}")
print(f"NIP05: {metadata.get_nip05()}")
print(f"LUD06: {metadata.get_lud06()}")
print(f"LUD16: {metadata.get_lud16()}")
#print(f"Custom: {metadata.get_custom()}")
if __name__ == '__main__':
parser = argparse.ArgumentParser(description='Fetch all metadata for a given npub')
parser.add_argument('npub', type=str, help='The npub of the user')
args = parser.parse_args()
asyncio.run(main(args.npub))
```
-

@ 0c503f08:4aed05c7
2025-02-21 17:30:53
originally posted at https://stacker.news/items/893208
-

@ 86a82cab:b5ef38a0
2025-02-21 17:26:03
Not everyone wants a super app though, and that’s okay. As with most things in the Nostr ecosystem, flexibility is key. Notedeck gives users the freedom to choose how they engage with it—whether it’s simply following hashtags or managing straightforward feeds. You'll be able to tailor Notedeck to fit your needs, using it as extensively or minimally as you prefer.<meta charset="utf-8"><span style="color: rgb(36, 36, 36); font-family: -apple-system, "system-ui", "Segoe UI", Helvetica, Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe
<img src="https://blossom.primal.net/9c8e97b31353f8c747e628eb5618d9b5bab2aa6176afd0ffa25b2b8246c38301.png">
\
\
UI Symbol"; font-size: 18px; font-style: normal; font-variant-ligatures: normal; font-variant-caps: normal; font-weight: 400; letter-spacing: normal; orphans: 2; text-align: start; text-indent: 0px; text-transform: none; widows: 2; word-spacing: 0px; -webkit-text-stroke-width: 0px; white-space: normal; background-color: rgb(255, 255, 255); text-decoration-thickness: initial; text-decoration-style: initial; text-decoration-color: initial; display: inline !important; float: none;">Not everyone wants a super app though, and that’s okay. As with most things in the Nostr ecosystem, flexibility is key. Notedeck gives users the freedom to choose how they engage with it—whether it’s simply following hashtags or managing straightforward feeds. You'll be able to tailor Notedeck to fit your needs, using it as extensively or minimally as you prefer.</span>
[IMage](https://nostrtips.com/wp-content/uploads/2023/03/nostr-structure-1-1024x576.jpg)
-

@ 86a82cab:b5ef38a0
2025-02-21 17:06:29
Not everyone wants a super app though, and that’s okay. As with most things in the Nostr ecosystem, flexibility is key. Notedeck gives users the freedom to choose how they engage with it—whether it’s simply following hashtags or managing straightforward feeds. You'll be able to tailor Notedeck to fit your needs, using it as extensively or minimally as you prefer.<meta charset="utf-8"><span style="color: rgb(36, 36, 36); font-family: -apple-system, "system-ui", "Segoe UI", Helvetica, Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe
<img src="https://blossom.primal.net/9c8e97b31353f8c747e628eb5618d9b5bab2aa6176afd0ffa25b2b8246c38301.png">
\
\
UI Symbol"; font-size: 18px; font-style: normal; font-variant-ligatures: normal; font-variant-caps: normal; font-weight: 400; letter-spacing: normal; orphans: 2; text-align: start; text-indent: 0px; text-transform: none; widows: 2; word-spacing: 0px; -webkit-text-stroke-width: 0px; white-space: normal; background-color: rgb(255, 255, 255); text-decoration-thickness: initial; text-decoration-style: initial; text-decoration-color: initial; display: inline !important; float: none;">Not everyone wants a super app though, and that’s okay. As with most things in the Nostr ecosystem, flexibility is key. Notedeck gives users the freedom to choose how they engage with it—whether it’s simply following hashtags or managing straightforward feeds. You'll be able to tailor Notedeck to fit your needs, using it as extensively or minimally as you prefer.</span>
[IMage](https://nostrtips.com/wp-content/uploads/2023/03/nostr-structure-1-1024x576.jpg)
-

@ 6e0ea5d6:0327f353
2025-02-21 17:01:17
Your father may have warned you when he saw you hanging out with bad company:
"Remember, you become your friends."
A maxim from Goethe conveys this idea even better:
"Tell me who you walk with, and I’ll tell you who you are."
Be mindful of who you allow into your life—not as an arrogant snob, but as someone striving to cultivate the best possible life.
Ask yourself about the people you know and spend time with:
Are they making me better? Do they encourage me to move forward and hold me accountable? Or do they drag me down to their level?
Now, with that in mind, ask yourself the most important question:
Should I spend more or less time with these people?
The second part of Goethe's quote reminds us of what is at stake in this choice:
"If I know how you spend your time," he said, "then I know what you may become."
"Above all, keep this in mind: never get so attached to your old friends and acquaintances that you are dragged down to their level. If you do not, you will be ruined. [...] You must choose whether you want to be loved by these friends and remain the same or become a better person at the expense of those associations. [...] If you try to do both, you will never make progress nor retain what you once had."
—Epictetus, Discourses
📌 "Remember that if you join someone covered in dirt, you can hardly avoid getting a little dirty yourself."
—Epictetus, Discourses
📚 (Excerpt from The Daily Stoic by Ryan Holiday)
Thank you for reading, my friend!
If this message helped you in any way,
consider leaving your glass “🥃” as a token of appreciation.
A toast to our family!